
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
BiosTouch.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BIOSTOUCH_H 00018 #define BIOSTOUCH_H 00019 00020 #include "mbed.h" 00021 #include "TouchPanel.h" 00022 #include "Display.h" 00023 #include "bios.h" 00024 #include "rtos.h" 00025 00026 class TouchHandler; 00027 00028 /** 00029 * Glue between the BIOS and the TouchPanel interface. 00030 */ 00031 class BiosTouch : public TouchPanel { 00032 public: 00033 00034 /** Get the only instance of the BiosTouch 00035 * 00036 * @returns The display 00037 */ 00038 static BiosTouch& instance() 00039 { 00040 static BiosTouch singleton; 00041 return singleton; 00042 } 00043 00044 /** 00045 * Initialize the touch controller. This method must be called before 00046 * calibrating or reading data from the controller 00047 * 00048 * @returns 00049 * Ok on success 00050 * An error code on failure 00051 */ 00052 TouchError init(); 00053 00054 /** 00055 * Tests if a touch controller is available or not. 00056 * 00057 * Note that this function only returns a valid value 00058 * after the display has been intitialized. 00059 * 00060 * @return true if there is a touch controller 00061 */ 00062 bool isTouchSupported(); 00063 00064 // From the TouchPanel interface 00065 virtual TouchError read(touch_coordinate_t &coord); 00066 virtual TouchError read(touch_coordinate_t* coord, int num); 00067 virtual TouchError info(bool* resistive, int* maxPoints, bool* calibrated); 00068 virtual TouchError calibrateStart(); 00069 virtual TouchError getNextCalibratePoint(uint16_t* x, uint16_t* y, bool* last=NULL); 00070 virtual TouchError waitForCalibratePoint(bool* morePoints, uint32_t timeout); 00071 virtual void setListener(Callback<void()> listener); 00072 00073 private: 00074 00075 bool _initialized; 00076 bool _haveInfo; 00077 bool _poweredOn; 00078 00079 bios_header_t* _bios; 00080 void* _biosData; 00081 00082 Thread* _handlerThread; 00083 TouchHandler* _handler; 00084 00085 bool _supportsTouch; 00086 bool _supportsTouchCalibration; 00087 uint8_t _touchNumFingers; 00088 00089 explicit BiosTouch(); 00090 // hide copy constructor 00091 BiosTouch(const BiosTouch&); 00092 // hide assign operator 00093 BiosTouch& operator=(const BiosTouch&); 00094 ~BiosTouch(); 00095 }; 00096 00097 #endif /* BIOSTOUCH_H */
Generated on Tue Jul 12 2022 14:18:31 by
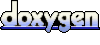