
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
RtosLog.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef RTOSLOG_H 00018 #define RTOSLOG_H 00019 00020 #include "mbed.h" 00021 #include "rtos.h" 00022 #include "dm_board_config.h" 00023 00024 #if defined(DM_BOARD_USE_USBSERIAL_IN_RTOSLOG) 00025 #include "USBSerial.h" 00026 #endif 00027 00028 /** 00029 * All threads can independantly call the printf function in the RtosLog class 00030 * without risk of getting the output tangled up. 00031 * 00032 * The RtosLog class uses a Thread to process the messages one at a time. 00033 * 00034 * 00035 * Example of using the RtosLog class: 00036 * 00037 * @code 00038 * #include "mbed.h" 00039 * #include "DMBoard.h" 00040 * 00041 * void myTask(void const*args) { 00042 * RtosLog* log = DMBoard::instance().logger(); 00043 * ... 00044 * log->printf("Hello world!\n"); 00045 * ... 00046 * } 00047 * @endcode 00048 * 00049 */ 00050 class RtosLog { 00051 public: 00052 enum Constants { 00053 MessageLen = 80, 00054 NumMessages = 16, 00055 }; 00056 00057 RtosLog(); 00058 ~RtosLog(); 00059 00060 /** Starts the logger thread, called from DMBoard::instance().init() 00061 */ 00062 void init(); 00063 00064 /** Printf that works in an RTOS 00065 * 00066 * This function will create a string from the specified format and 00067 * optional extra arguments and put it into a message that the RtosLog 00068 * thread will write to the log. 00069 * 00070 * Note that if the underlying queue is full then this function 00071 * will block until an entry becomes available. This is required to 00072 * make sure that all printf calls actually get printed. If this happens 00073 * too often then increase the priority of the RtosLog thread or reduce 00074 * the number of printed messages. 00075 * 00076 * @param format format string 00077 * @param ... optional extra arguments 00078 */ 00079 int printf(const char* format, ...); 00080 00081 /** Printf that works in an RTOS 00082 * 00083 * This function will create a string from the specified format and 00084 * optional extra arguments and put it into a message that the RtosLog 00085 * thread will write to the log. 00086 * 00087 * Note that if the underlying queue is full then this function 00088 * discards the message and returns immediately. 00089 * 00090 * @param format format string 00091 * @param ... optional extra arguments 00092 */ 00093 int isr_printf(const char* format, ...); 00094 00095 private: 00096 00097 typedef struct { 00098 char* ptr; /* Non-NULL if memory is allocated */ 00099 char msg[MessageLen+1]; /* A counter value */ 00100 } message_t; 00101 00102 MemoryPool<message_t, NumMessages> _mpool; 00103 Queue<message_t, NumMessages> _queue; 00104 Semaphore _sem; 00105 #if defined(DM_BOARD_USE_USBSERIAL_IN_RTOSLOG) 00106 USBSerial _serial; 00107 #else 00108 Serial _serial; 00109 #endif 00110 Thread* _thr; 00111 00112 static void logTask(void const* args); 00113 }; 00114 00115 #endif
Generated on Tue Jul 12 2022 14:18:31 by
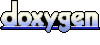