
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
DMBoard.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef DMBOARD_H 00018 #define DMBOARD_H 00019 00020 #include "mbed.h" 00021 #include "dm_board_config.h" 00022 #include "RtosLog.h" 00023 00024 #if defined(DM_BOARD_USE_MCI_FS) 00025 #include "MCIFileSystem.h" 00026 #endif 00027 #if defined(DM_BOARD_USE_QSPI_FS) 00028 #include "SPIFI.h" 00029 #include "QSPIFileSystem.h" 00030 #elif defined(DM_BOARD_USE_QSPI) 00031 #include "SPIFI.h" 00032 #endif 00033 #include "Display.h" 00034 #include "TouchPanel.h" 00035 #if defined(DM_BOARD_USE_REGISTRY) 00036 #include "Registry.h" 00037 #endif 00038 00039 #if defined(DM_BOARD_USE_USB_HOST) && defined(DM_BOARD_USE_ETHERNET) 00040 /* 00041 * It is not possible to use USB Host and Ethernet at the same time due to a 00042 * conflict in memory areas. 00043 * 00044 * In OS 5 memory area AHBSRAM0 is used by the EMAC driver (lpc17_emac.cpp) and 00045 * AHBSRAM1 is used by LWIP as heap (lwip_sys_arch.c). The USB host 00046 * implementation is using AHBSRAM1 (USBHALHost.cpp). 00047 */ 00048 #error "Cannot have both USB Host and Ethernet enabled at the same time. See DMBoard.h" 00049 #endif 00050 00051 00052 /** 00053 * Example of using the Board class: 00054 * 00055 * @code 00056 * #include "mbed.h" 00057 * #include "DMBoard.h" 00058 * 00059 * int main(void) { 00060 * DMBoard* board = &DMBoard::instance(); 00061 * board->init(); 00062 * ... 00063 * board->setLed(1, true); 00064 * } 00065 * @endcode 00066 */ 00067 class DMBoard { 00068 public: 00069 enum Leds { 00070 Led1, 00071 Led2, 00072 Led3, 00073 Led4, 00074 }; 00075 00076 enum BoardError { 00077 Ok = 0, 00078 MemoryError, 00079 SpifiError, 00080 DisplayError, 00081 TouchError, 00082 BiosInvalidError, 00083 BiosVersionError, 00084 BiosStorageError, 00085 RegistryError, 00086 }; 00087 00088 /** Get the only instance of the DMBoard 00089 * 00090 * @returns The DMBoard 00091 */ 00092 static DMBoard& instance() 00093 { 00094 static DMBoard singleton; 00095 return singleton; 00096 } 00097 00098 /** Initializes the wanted features 00099 * 00100 * @returns 00101 * Ok on success 00102 * An error code on failure 00103 */ 00104 BoardError init(); 00105 00106 /** Controls the four LEDs on the Display Module 00107 * 00108 * @param led One of Led1, Led2, Led3 or Led4 00109 * @param on true to turn the LED on regardless of its polarity 00110 */ 00111 void setLED(Leds led, bool on); 00112 00113 /** Controls the buzzer 00114 * 00115 * Examples: 00116 * buzzer() turns it off 00117 * buzzer(440) plays an A4 (440Hz) note forever 00118 * buzzer(200, 25) plays a 200Hz tone for 25ms and then turns it off 00119 * 00120 * Note that if duration_ms is >0 this is a blocking call 00121 * 00122 * @param frequency the frequency of the tone (in Hz) or 0 to turn it off 00123 * @param duration_ms the number of milliseconds to play or 0 for forever 00124 */ 00125 void buzzer(int frequency=0, int duration_ms=0); 00126 00127 /** Test if the USER button is pressed or not 00128 * 00129 * @returns 00130 * True if the button is pressed, false if not 00131 */ 00132 bool buttonPressed(); 00133 00134 /** Returns the TouchPanel interface 00135 * 00136 * @returns 00137 * The touch panel 00138 */ 00139 TouchPanel* touchPanel(); 00140 00141 /** Returns the Display interface 00142 * 00143 * @returns 00144 * The display 00145 */ 00146 Display* display(); 00147 00148 /** Returns the logger interface 00149 * 00150 * @returns 00151 * The logger 00152 */ 00153 RtosLog* logger() { return &_logger; } 00154 00155 #if defined(DM_BOARD_USE_REGISTRY) 00156 /** Returns the Registry interface 00157 * 00158 * @returns 00159 * The registry 00160 */ 00161 Registry* registry() { return &Registry::instance(); } 00162 #endif 00163 00164 #if defined(DM_BOARD_USE_MCI_FS) 00165 /** Returns the MCI File System instance. 00166 * 00167 * Can be used to call e.g. cardInserted(). 00168 * 00169 * @returns 00170 * The file system instance 00171 */ 00172 MCIFileSystem* getMciFS() { return &_mcifs; } 00173 #else 00174 void* getMciFS() { return NULL; } 00175 #endif 00176 #if defined(DM_BOARD_USE_QSPI_FS) 00177 /** Returns the QSPI File System instance. 00178 * 00179 * Can be used to call e.g. isformatted() and format(). 00180 * 00181 * @returns 00182 * The file system instance 00183 */ 00184 QSPIFileSystem* getQspiFS() { return &_qspifs; } 00185 #else 00186 void* getQspiFS() { return NULL; } 00187 #endif 00188 00189 00190 private: 00191 00192 bool _initialized; 00193 00194 #if defined(DM_BOARD_USE_MCI_FS) 00195 MCIFileSystem _mcifs; 00196 FATFileSystem _mciFatFs; 00197 #endif 00198 #if defined(DM_BOARD_USE_QSPI_FS) 00199 QSPIFileSystem _qspifs; 00200 #endif 00201 00202 PwmOut _buzzer; 00203 DigitalIn _button; 00204 DigitalOut _led1; 00205 DigitalOut _led2; 00206 DigitalOut _led3; 00207 DigitalOut _led4; 00208 00209 RtosLog _logger; 00210 00211 explicit DMBoard(); 00212 // hide copy constructor 00213 DMBoard(const DMBoard&); 00214 // hide assign operator 00215 DMBoard& operator=(const DMBoard&); 00216 ~DMBoard(); 00217 }; 00218 00219 #endif
Generated on Tue Jul 12 2022 14:18:31 by
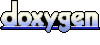