
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
meas.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef _MEAS_H_ 00018 #define _MEAS_H_ 00019 00020 /****************************************************************************** 00021 * Includes 00022 *****************************************************************************/ 00023 00024 #include "mbed.h" 00025 #include "dm_board_config.h" 00026 00027 /****************************************************************************** 00028 * Typedefs and defines 00029 *****************************************************************************/ 00030 00031 // Enable extra measuring pins, at the expense of peripheral access. 00032 // For example MEAS pins 5-9 will prevent use of UART4/SPI2 and some timers. 00033 //#define EXTRA_PINS 00034 00035 // Internal macros, don't use directly 00036 #if defined(DM_BOARD_ENABLE_MEASSURING_PINS) 00037 #define _INTERNAL_SET_MEAS_PIN(__port, __pin) ((LPC_GPIO ## __port)->SET = (1UL << (__pin))) 00038 #define _INTERNAL_CLR_MEAS_PIN(__port, __pin) ((LPC_GPIO ## __port)->CLR = (1UL << (__pin))) 00039 #else 00040 #define _INTERNAL_SET_MEAS_PIN(__port, __pin) do {} while(0) 00041 #define _INTERNAL_CLR_MEAS_PIN(__port, __pin) do {} while(0) 00042 #endif 00043 00044 // Manipulates measurement pin 1 which is GPIO1[24], available on J10-7 00045 #define SET_MEAS_PIN_1() _INTERNAL_SET_MEAS_PIN(1, 24) 00046 #define CLR_MEAS_PIN_1() _INTERNAL_CLR_MEAS_PIN(1, 24) 00047 00048 // Manipulates measurement pin 2 which is GPIO1[23], available on J10-8 00049 #define SET_MEAS_PIN_2() _INTERNAL_SET_MEAS_PIN(1, 23) 00050 #define CLR_MEAS_PIN_2() _INTERNAL_CLR_MEAS_PIN(1, 23) 00051 00052 // Manipulates measurement pin 3 which is GPIO1[20], available on J10-9 00053 #define SET_MEAS_PIN_3() _INTERNAL_SET_MEAS_PIN(1, 20) 00054 #define CLR_MEAS_PIN_3() _INTERNAL_CLR_MEAS_PIN(1, 20) 00055 00056 // Manipulates measurement pin 4 which is GPIO1[19], available on J10-10 00057 #define SET_MEAS_PIN_4() _INTERNAL_SET_MEAS_PIN(1, 19) 00058 #define CLR_MEAS_PIN_4() _INTERNAL_CLR_MEAS_PIN(1, 19) 00059 00060 #ifdef EXTRA_PINS 00061 // Manipulates measurement pin 5 which is GPIO5[0], available on J10-15 00062 #define SET_MEAS_PIN_5() _INTERNAL_SET_MEAS_PIN(5, 0) 00063 #define CLR_MEAS_PIN_5() _INTERNAL_CLR_MEAS_PIN(5, 0) 00064 00065 // Manipulates measurement pin 6 which is GPIO5[1], available on J10-16 00066 #define SET_MEAS_PIN_6() _INTERNAL_SET_MEAS_PIN(5, 1) 00067 #define CLR_MEAS_PIN_6() _INTERNAL_CLR_MEAS_PIN(5, 1) 00068 00069 // Manipulates measurement pin 7 which is GPIO5[2], available on J10-17 00070 #define SET_MEAS_PIN_7() _INTERNAL_SET_MEAS_PIN(5, 2) 00071 #define CLR_MEAS_PIN_7() _INTERNAL_CLR_MEAS_PIN(5, 2) 00072 00073 // Manipulates measurement pin 8 which is GPIO5[3], available on J10-18 00074 #define SET_MEAS_PIN_8() _INTERNAL_SET_MEAS_PIN(5, 3) 00075 #define CLR_MEAS_PIN_8() _INTERNAL_CLR_MEAS_PIN(5, 3) 00076 00077 // Manipulates measurement pin 9 which is GPIO5[4], available on J10-19 00078 #define SET_MEAS_PIN_9() _INTERNAL_SET_MEAS_PIN(5, 4) 00079 #define CLR_MEAS_PIN_9() _INTERNAL_CLR_MEAS_PIN(5, 4) 00080 00081 #define _INTERNAL_EXTRA_INIT_MEAS() do {\ 00082 LPC_IOCON->P5_0 &= ~0x7; \ 00083 LPC_IOCON->P5_1 &= ~0x7; \ 00084 LPC_IOCON->P5_2 &= ~0x7; \ 00085 LPC_IOCON->P5_3 &= ~0x7; \ 00086 LPC_IOCON->P5_4 &= ~0x7; \ 00087 LPC_GPIO5->DIR |= (1<<0) | (1<<1) | (1<<2) | (1<<3) | (1<<4); \ 00088 CLR_MEAS_PIN_5(); \ 00089 CLR_MEAS_PIN_6(); \ 00090 CLR_MEAS_PIN_7(); \ 00091 CLR_MEAS_PIN_8(); \ 00092 CLR_MEAS_PIN_9(); \ 00093 } while(0) 00094 #else 00095 #define _INTERNAL_EXTRA_INIT_MEAS() do {} while(false) 00096 #endif 00097 00098 #if defined(DM_BOARD_ENABLE_MEASSURING_PINS) 00099 #define _INTERNAL_INIT_MEAS() do {\ 00100 LPC_IOCON->P1_19 &= ~0x7; \ 00101 LPC_IOCON->P1_20 &= ~0x7; \ 00102 LPC_IOCON->P1_23 &= ~0x7; \ 00103 LPC_IOCON->P1_24 &= ~0x7; \ 00104 LPC_GPIO1->DIR |= (1<<19) | (1<<20) | (1<<23) | (1<<24); \ 00105 CLR_MEAS_PIN_1(); \ 00106 CLR_MEAS_PIN_2(); \ 00107 CLR_MEAS_PIN_3(); \ 00108 CLR_MEAS_PIN_4(); \ 00109 _INTERNAL_EXTRA_INIT_MEAS(); \ 00110 } while(0) 00111 #else 00112 #define _INTERNAL_INIT_MEAS() do {} while(0) 00113 #endif 00114 00115 /****************************************************************************** 00116 * Global Variables 00117 *****************************************************************************/ 00118 00119 #endif /* end _MEAS_H_ */ 00120
Generated on Tue Jul 12 2022 14:18:31 by
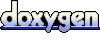