
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
RAMFileSystem.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef RAMFILESYSTEM_H 00018 #define RAMFILESYSTEM_H 00019 00020 #include "mbed.h" 00021 #include "FATFileSystem.h" 00022 #include "sdram.h" 00023 #include <stdint.h> 00024 00025 /** Creates a FAT file system in SDRAM 00026 * 00027 * @code 00028 * #include "mbed.h" 00029 * #include "RAMFileSystem.h" 00030 * 00031 * RAMFileSystem ramfs(0xA0000000, 4*1024*1024, "ram"); // 4MB of ram starting at 0xA... 00032 * 00033 * int main() { 00034 * sdram_init(); 00035 * 00036 * FILE *fp = fopen("/ram/myfile.txt", "w"); 00037 * fprintf(fp, "Hello World!\n"); 00038 * fclose(fp); 00039 * } 00040 * @endcode 00041 */ 00042 class RAMFileSystem : public FATFileSystem { 00043 public: 00044 00045 /** Create the File System in RAM 00046 * 00047 * @param addr Start of memory to use for file system 00048 * @param size Number of bytes to use for file system 00049 * @param name The name used to access the virtual filesystem 00050 */ 00051 RAMFileSystem(uint32_t addr, uint32_t size, const char* name); 00052 virtual int disk_initialize(); 00053 virtual int disk_status(); 00054 virtual int disk_read(uint8_t * buffer, uint64_t sector, uint8_t count); 00055 virtual int disk_write(const uint8_t * buffer, uint64_t sector, uint8_t count); 00056 virtual int disk_sync(); 00057 virtual uint64_t disk_sectors(); 00058 00059 uint64_t disk_size(); 00060 00061 protected: 00062 00063 uint32_t memStart; 00064 uint32_t memSize; 00065 int status; 00066 }; 00067 00068 #endif
Generated on Tue Jul 12 2022 14:18:31 by
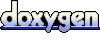