Project Submission (late)
Dependencies: mbed
main.cpp
00001 /* 00002 ELEC2645 Embedded Systems Project 00003 School of Electronic & Electrical Engineering 00004 University of Leeds 00005 Name: Thomas Caine 00006 Username: el175c 00007 Student ID Number: 201127594 00008 Date: 10/05/2019 00009 */ 00010 00011 00012 #include "mbed.h" 00013 #include "Gamepad.h" 00014 #include "N5110.h" 00015 #include "Maze.h" 00016 #include "Vector2Di.h" 00017 #include "Player.h" 00018 #include "Drawer.h" 00019 #include "MainMenu.h" 00020 #include "DefeatMenu.h" 00021 #include "VictoryMenu.h" 00022 00023 /* Note: 00024 If it were up to me almost everything in this file would be in a Game object and main would only have game.init(); game.run(); in it 00025 Unfortunately I have been plagued by L6312W the empty execution region error (not the warning) all the way through this project 00026 and this is as good as I could get it in terms of object orientation. 00027 As far as I'm aware this error is a bug with the mbed compiler of this version but it can be worked around. 00028 Dock marks as you see fit for not encapsulating the other headers into a single class. 00029 (but the other classes are nice and object-oriented, right?) 00030 */ 00031 00032 00033 N5110 lcd(PTC9,PTC0,PTC7,PTD2,PTD1,PTC11); 00034 Gamepad gamepad; 00035 /** Causes the currentMenu's button index to be decremented, moving the cursor to the higher button 00036 */ 00037 void buttonPressedUp() { 00038 if (currentMenu->buttonIndex > 0) { 00039 currentMenu->buttonIndex -= 1; 00040 currentButton = currentMenu->buttons[currentMenu->buttonIndex]; 00041 } 00042 printf("currentMenu->buttonIndex = %d\n", currentMenu->buttonIndex); 00043 } 00044 00045 /** Causes the currentMenu's button index to be incremented, moving the cursor to the lower button 00046 */ 00047 void buttonPressedDown() { 00048 if (currentMenu->buttonIndex < (currentMenu->numOfButtons - 1)) { 00049 currentMenu->buttonIndex += 1; 00050 currentButton = currentMenu->buttons[currentMenu->buttonIndex]; 00051 } 00052 printf("currentMenu->buttonIndex = %d\n", currentMenu->buttonIndex); 00053 } 00054 00055 Ticker arrowFlicker; 00056 Ticker ingameCounter; 00057 bool arrowFlickFlag = 1; 00058 int ingameTime; 00059 00060 /** Funciton for drawing the ingame Timer 00061 */ 00062 void drawTimer() { 00063 lcd.drawRect(1,1,20,8,FILL_WHITE); 00064 std::stringstream ss; 00065 ss << ingameTime; 00066 std::string timeStr = "Time:" + ss.str(); 00067 lcd.printString(timeStr.c_str(), 1,0); 00068 } 00069 /** Timer increment/decrement function 00070 * @brief depending on the timerFlag (toggled on or off) it will count up or down 00071 */ 00072 void timerFunc() { 00073 if (timerFlag) 00074 ingameTime--; 00075 else 00076 ingameTime++; 00077 } 00078 00079 /** Funciton making the button selection arrow flicker 00080 * @brief aesthetically pleasing (i think). 00081 */ 00082 void selectionArrowFunc() { 00083 arrowFlickFlag = !arrowFlickFlag; 00084 }; 00085 00086 /** The main function 00087 * Due to constantly getting Empty Execution region errors this is a lot more full than it should be 00088 * refer to note at the top of main.cpp for more comments on the issue. 00089 */ 00090 int main() { 00091 gamepad.init(); 00092 lcd.init(); 00093 lcd.setContrast(0.5); 00094 lcd.normalMode(); 00095 lcd.setBrightness(0.5); 00096 00097 arrowFlicker.attach(&selectionArrowFunc,0.9); 00098 currentMenu = new MainMenu(&lcd); 00099 00100 // program loop - never exits 00101 while (true) { 00102 // menu loop 00103 gamepad.check_event(Gamepad::START_PRESSED); // consume trailing input from last loop 00104 while (true) { 00105 00106 if (gamepad.check_event(Gamepad::A_PRESSED)) { 00107 buttonPressedDown(); 00108 } 00109 if (gamepad.check_event(Gamepad::Y_PRESSED)) { 00110 buttonPressedUp(); 00111 } 00112 if (gamepad.check_event(Gamepad::X_PRESSED)) { 00113 currentButton->runBack(); 00114 gamepad.check_event(Gamepad::X_PRESSED); // consume second input if it appeared 00115 } 00116 if (gamepad.check_event(Gamepad::B_PRESSED)) { 00117 currentButton->run(); 00118 gamepad.check_event(Gamepad::B_PRESSED); // consume second input if it appeared 00119 } 00120 if (gamepad.check_event(Gamepad::START_PRESSED)) { 00121 currentButton->run(); 00122 gamepad.check_event(Gamepad::START_PRESSED); // consume second input if it appeared 00123 } 00124 if (gamepad.check_event(Gamepad::BACK_PRESSED)) { 00125 delete currentMenu; 00126 currentMenu = new MainMenu(&lcd); 00127 } 00128 00129 lcd.clear(); 00130 currentMenu->draw(); 00131 00132 if (arrowFlickFlag) 00133 lcd.drawSprite((currentButton->x)-2,(currentButton->y),7,4, (int *)selectArrow2); 00134 00135 lcd.refresh(); 00136 if (beginFlag) { 00137 beginFlag = false; 00138 printf("begin flag is true\n"); 00139 break; 00140 } 00141 sleep(); 00142 } 00143 delete currentMenu; 00144 00145 Maze maze; 00146 Player player(&maze); 00147 maze.selectMaze(mazeSize); 00148 player.pos.x = maze.startX; 00149 player.pos.y = maze.startY; 00150 Drawer drawer(&player, &lcd); 00151 00152 if (timerFlag) 00153 ingameTime = maze.timeToFinish; 00154 else 00155 ingameTime = 0; 00156 ingameCounter.attach(&timerFunc, 1); 00157 arrowFlicker.detach(); 00158 bool winFlag = false; 00159 00160 // Main game loop 00161 while (true) { 00162 if (gamepad.check_event(Gamepad::Y_PRESSED)) { 00163 if (player.checkLocation(player.pos, 0, 2)) { 00164 winFlag = true; 00165 } 00166 player.walk(); 00167 wait(0.2); 00168 if (gamepad.check_event(Gamepad::Y_PRESSED)) { 00169 printf("walked but input fired twice\n"); 00170 } 00171 } 00172 if (gamepad.check_event(Gamepad::X_PRESSED)) { 00173 player.turnLeft(); 00174 wait(0.2); 00175 if (gamepad.check_event(Gamepad::X_PRESSED)) { 00176 printf("turned left but input fired twice\n"); 00177 } 00178 } 00179 if (gamepad.check_event(Gamepad::B_PRESSED)) { 00180 player.turnRight(); 00181 wait(0.2); 00182 if (gamepad.check_event(Gamepad::B_PRESSED)) { 00183 printf("turned right but input fired twice\n"); 00184 } 00185 } 00186 if (gamepad.check_event(Gamepad::A_PRESSED)) { 00187 player.stepBack(); 00188 wait(0.2); 00189 if (gamepad.check_event(Gamepad::A_PRESSED)) { 00190 printf("stepped back but input fired twice\n"); 00191 } 00192 } 00193 if (gamepad.check_event(Gamepad::R_PRESSED)) { 00194 player.turnRight(); 00195 wait(0.2); 00196 if (gamepad.check_event(Gamepad::R_PRESSED)) { 00197 printf("turned right but input fired twice\n"); 00198 } 00199 } 00200 if (gamepad.check_event(Gamepad::L_PRESSED)) { 00201 player.turnLeft(); 00202 wait(0.2); 00203 if (gamepad.check_event(Gamepad::L_PRESSED)) { 00204 printf("turned left but input fired twice\n"); 00205 } 00206 } 00207 00208 if (winFlag) { 00209 printf("Player has escaped the maze\n"); 00210 // player has won, end the game loop 00211 winFlag = false; 00212 currentMenu = new VictoryMenu(&lcd); 00213 if (timerFlag) { 00214 currentMenu->score = maze.timeToFinish + (ingameTime * 2); 00215 } else { 00216 currentMenu->score = maze.timeToFinish + ((maze.timeToFinish*10)/ingameTime); 00217 } 00218 break; 00219 } 00220 if (timerFlag && (ingameTime <= 0)) { 00221 printf("Player has ran out of time\n"); 00222 // player has lost, end the game loop 00223 currentMenu = new DefeatMenu(&lcd); 00224 currentMenu->score = 0; 00225 break; 00226 } 00227 printf("========\n"); 00228 00229 lcd.clear(); 00230 drawer.drawScreen(); 00231 drawTimer(); 00232 lcd.refresh(); 00233 00234 sleep(); 00235 } 00236 00237 arrowFlicker.attach(&selectionArrowFunc,0.9); 00238 gamepad.check_event(Gamepad::START_PRESSED); 00239 while (true) { 00240 if (gamepad.check_event(Gamepad::B_PRESSED)) { 00241 buttonPressedDown(); 00242 } 00243 if (gamepad.check_event(Gamepad::X_PRESSED)) { 00244 buttonPressedUp(); 00245 } 00246 if (gamepad.check_event(Gamepad::A_PRESSED)) { 00247 //buttonActivate(*currentButton); 00248 } 00249 if (gamepad.check_event(Gamepad::START_PRESSED)) { 00250 currentButton->run(); 00251 } 00252 lcd.clear(); 00253 currentMenu->draw(); 00254 if (arrowFlickFlag) { 00255 lcd.drawSprite((currentButton->x)-2,(currentButton->y),7,4, 00256 (int *)selectArrow2); 00257 } 00258 if (restartFlag) { 00259 restartFlag = false; 00260 delete currentMenu; 00261 currentMenu = new StartMenu(&lcd); 00262 break; 00263 } 00264 if (menuFlag) { 00265 menuFlag = false; 00266 delete currentMenu; 00267 currentMenu = new MainMenu(&lcd); 00268 break; 00269 } 00270 lcd.refresh(); 00271 sleep(); 00272 } 00273 } 00274 }
Generated on Thu Jul 14 2022 20:06:28 by
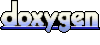