Project Submission (late)
Dependencies: mbed
Drawer.h
00001 #ifndef DRAWER_H 00002 #define DRAWER_H 00003 00004 #include "Player.h" 00005 #include "N5110.h" 00006 /** Drawer Class 00007 * @brief A class responsible for searching the maze matrix relative to the player's location 00008 * @brief and drawing the correct graphics to the LCD accordingly. 00009 * @brief can draw cells up to a distance of 4 in front of the player 00010 * @brief (not including the cell they are currently standing in). 00011 * 00012 * @brief Version 1.0 00013 * @author Thomas Caine 00014 * @date May 2019 00015 */ 00016 class Drawer { 00017 00018 public: 00019 /** Create a Drawer object capable of printing to the lcd 00020 * @param player - pointer to a player object. Player's direction and position is needed 00021 * @param screenPtr - pointer to the LCD object. Important for the lcd object to be shared so it's a pointer. 00022 */ 00023 Drawer(Player* player, N5110* screenPtr); 00024 00025 /** Draw the first branch. 00026 * 00027 * This corresponds to the line of 3 cells in front of the player, 00028 * beginning from the cell immediately to their _left_. 00029 * @param location - vector representing the cell to begin branch search from 00030 */ 00031 void drawBranch1(Vector2Di loc); 00032 /** Draw the second branch. 00033 * 00034 * This corresponds to the line of 3 cells in front of the player, 00035 * beginning from the cell immediately to their _right_. 00036 * @param location - vector representing the cell to begin branch search from 00037 */ 00038 void drawBranch2(Vector2Di loc); 00039 /** Draw the third branch. 00040 * 00041 * This corresponds to the line of 3 cells in front of the player, 00042 * beginning from the cell forward one and left one. 00043 * @param location - vector representing the cell to begin branch search from 00044 */ 00045 void drawBranch3(Vector2Di loc); 00046 /** Draw the fourth branch. 00047 * 00048 * This corresponds to the line of 3 cells in front of the player, 00049 * beginning from the cell forward one and right one. 00050 * @param location - vector representing the cell to begin branch search from 00051 */ 00052 void drawBranch4(Vector2Di loc); 00053 /** Draw the fifth branch. 00054 * 00055 * This corresponds to the line of 3 cells in front of the player, 00056 * beginning from the cell forward two and left one. 00057 * @param location - vector representing the cell to begin branch search from 00058 */ 00059 void drawBranch5(Vector2Di loc); 00060 /** Draw the fifth branch. 00061 * 00062 * This corresponds to the line of 3 cells in front of the player, 00063 * beginning from the cell forward two and right one. 00064 */ 00065 void drawBranch6(Vector2Di loc); 00066 /** 00067 * @brief Draw the player's current view to the screen buffer. 00068 * 00069 * @details drawScreen() will walk the cells in front of the player, checking 00070 * @details which cells are empty or solid. When it finds an empty cell either in 00071 * @details front, to the left or to the right of the current cell, it will draw graphics 00072 * @details and/or call the drawBranch# functions accordingly. 00073 */ 00074 void drawScreen(); 00075 00076 // references to the player and lcd objects 00077 Player* player; 00078 N5110* lcd; 00079 00080 00081 00082 }; 00083 00084 #endif // DRAWER_H
Generated on Thu Jul 14 2022 20:06:28 by
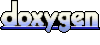