Project Submission (late)
Dependencies: mbed
Drawer.cpp
00001 #include "Drawer.h" 00002 #include "GraphicMatrices.h" 00003 00004 // constructor simply assigns the passed pointers to the 00005 // class' internal fields for storage 00006 Drawer::Drawer(Player* playerPtr, N5110* screenPtr) { 00007 player = playerPtr; 00008 lcd = screenPtr; 00009 } 00010 00011 void Drawer::drawBranch1(Vector2Di loc) { 00012 Vector2Di peekDirection = player->direction; 00013 peekDirection.rotateVector(-PI/2); // rotate vector left 00014 loc.addVector(peekDirection); // then move forward one 00015 00016 lcd->drawSprite(0,3,42,21,(int *)greenBranchG); 00017 if (player->checkLocation(loc, 0, 0)) { 00018 //if the square in front is open 00019 lcd->drawSprite(0,9,30,21,(int *)sideSquare1G); 00020 loc.addVector(player->direction); // move forward 00021 } 00022 else if (player->checkLocation(loc, 0, 2)) { 00023 // if the square in front is the exit 00024 lcd->drawSprite(0,9,30,21,(int *)sideSquare1G); 00025 lcd->drawSprite(0,8,32,21,(int *)exit2); // draw the exit graphic for this position 00026 return; 00027 } 00028 else { 00029 return; 00030 } 00031 if (player->checkLocation(loc, -PI/2, 0)) 00032 lcd->drawSprite(0,12,24,7,(int *)sideBranch1G); 00033 if (player->checkLocation(loc, 0, 0)) 00034 lcd->drawSprite(10,13,22,11,(int *)sideSquare2G); 00035 else if (player->checkLocation(loc, 0, 2)) { 00036 lcd->drawSprite(10,13,22,11,(int *)sideSquare2G); 00037 lcd->drawSprite(15,15,18,6,(int *)exit6); 00038 } 00039 } 00040 00041 void Drawer::drawBranch2(Vector2Di loc) { 00042 Vector2Di peekDirection = player->direction; 00043 peekDirection.rotateVector(PI/2); 00044 loc.addVector(peekDirection); 00045 lcd->drawSprite(63,3,42,21,(int *)greenBranchG); 00046 if (player->checkLocation(loc, 0, 0)) { 00047 lcd->drawSprite(63,9,30,21,(int *)sideSquare6G); 00048 loc.addVector(player->direction); 00049 } 00050 else if (player->checkLocation(loc, 0, 2)) { 00051 //lcd->drawSprite(63,9,30,21,(int *)sideSquare6G); 00052 lcd->drawSprite(63,8,32,21,(int *)exit2); 00053 return; 00054 } 00055 else { 00056 return; 00057 } 00058 if (player->checkLocation(loc, PI/2, 0)) 00059 lcd->drawSprite(77,12,24,7,(int *)sideBranch1G); 00060 if (player->checkLocation(loc, 0, 0)) 00061 lcd->drawSprite(63,13,22,11,(int *)sideSquare7G); 00062 else if (player->checkLocation(loc, 0, 2)) { 00063 lcd->drawSprite(63,13,22,11,(int *)sideSquare7G); 00064 lcd->drawSprite(63,15,18,6,(int *)exit5); 00065 } 00066 } 00067 00068 00069 void Drawer::drawBranch3(Vector2Di loc) { 00070 Vector2Di peekDirection = player->direction; 00071 peekDirection.rotateVector(-PI/2); 00072 loc.addVector(peekDirection); 00073 lcd->drawSprite(22,12,24,8,(int *)orangeBranchG); 00074 if (player->checkLocation(loc, 0, 0)) { 00075 lcd->drawSprite(22,17,14,8,(int *)sideSquare3G); 00076 loc.addVector(player->direction); 00077 } 00078 else if (player->checkLocation(loc, 0, 2)) { 00079 //lcd->drawSprite(22,17,14,8,(int *)sideSquare3G); 00080 lcd->drawSprite(22,15,18,8,(int *)exit4); 00081 return; 00082 } 00083 else { 00084 return; 00085 } 00086 if (player->checkLocation(loc, 0, 0)) { 00087 lcd->drawSprite(28,19,10,2,(int *)sideSquare4G); 00088 } 00089 else { 00090 return; 00091 } 00092 } 00093 void Drawer::drawBranch4(Vector2Di loc) { 00094 Vector2Di peekDirection = player->direction; 00095 peekDirection.rotateVector(PI/2); 00096 loc.addVector(peekDirection); 00097 lcd->drawSprite(54,12,24,8,(int *)orangeBranchG); 00098 if (player->checkLocation(loc, 0, 0)) { 00099 lcd->drawSprite(54,17,14,8,(int *)sideSquare8G); 00100 loc.addVector(player->direction); 00101 } 00102 else if (player->checkLocation(loc, 0, 2)) { 00103 //lcd->drawSprite(54,17,14,8,(int *)sideSquare8G); 00104 lcd->drawSprite(54,15,18,8,(int *)exit4); 00105 return; 00106 } 00107 else { 00108 return; 00109 } 00110 if (player->checkLocation(loc, 0, 0)) { 00111 lcd->drawSprite(54,19,10,2,(int *)sideSquare4G); 00112 } 00113 else { 00114 return; 00115 } 00116 } 00117 void Drawer::drawBranch5(Vector2Di loc) { 00118 Vector2Di peekDirection = player->direction; 00119 peekDirection.rotateVector(-PI/2); 00120 loc.addVector(peekDirection); 00121 lcd->drawSprite(31,18,12,5,(int *)blueBranchG); 00122 if (player->checkLocation(loc, 0, 0)) { 00123 lcd->drawSprite(31,20,8,5,(int *)sideSquare5G); 00124 } 00125 else if (player->checkLocation(loc, 0, 2)) { 00126 //lcd->drawSprite(54,17,14,8,(int *)sideSquare5G); 00127 lcd->drawSprite(31,20,8,5,(int *)exit8); 00128 return; 00129 } 00130 else { 00131 return; 00132 } 00133 } 00134 void Drawer::drawBranch6(Vector2Di loc) { 00135 Vector2Di peekDirection = player->direction; 00136 peekDirection.rotateVector(PI/2); 00137 loc.addVector(peekDirection); 00138 lcd->drawSprite(48,18,12,5,(int *)blueBranchG); 00139 if (player->checkLocation(loc, 0, 0)) { 00140 lcd->drawSprite(48,20,8,5,(int *)sideSquare9G); 00141 } 00142 else if (player->checkLocation(loc, 0, 2)) { 00143 //lcd->drawSprite(48,20,8,5,(int *)sideSquare9G); 00144 lcd->drawSprite(48,20,8,5,(int *)exit8); 00145 return; 00146 } 00147 else { 00148 return; 00149 } 00150 } 00151 00152 void Drawer::drawScreen() { 00153 Vector2Di ghostLocation = player->pos; 00154 // phase 1 00155 lcd->drawSprite(18,0,48,48,(int *)greenSquareG); 00156 if (player->checkLocation(ghostLocation, -PI/2, 0)) // if the left square is empty 00157 drawBranch1(ghostLocation); 00158 if (player->checkLocation(ghostLocation, PI/2, 0)) // left/right are reversed 00159 drawBranch2(ghostLocation); 00160 00161 if (player->checkLocation(ghostLocation, 0, 0)) { // if the front square is empty 00162 ghostLocation.addVector(player->direction); // move forward 00163 } 00164 else if (player->checkLocation(ghostLocation, 0, 2)) { 00165 lcd->drawSprite(22,4,40,40,(int *)orangeSquareG); 00166 lcd->drawSprite(26,8,32,32,(int *)exit1); // if front square is exit 00167 return; 00168 } 00169 else { 00170 return; // drawing is complete 00171 } 00172 // phase 2 00173 lcd->drawSprite(22,4,40,40,(int *)orangeSquareG); 00174 if (player->checkLocation(ghostLocation, -PI/2, 0)) // if the left square is empty 00175 drawBranch3(ghostLocation); 00176 if (player->checkLocation(ghostLocation, PI/2, 0)) 00177 drawBranch4(ghostLocation); 00178 if (player->checkLocation(ghostLocation,0, 0)) { // if the front square is empty 00179 ghostLocation.addVector(player->direction); // move forward 00180 } 00181 else if (player->checkLocation(ghostLocation, 0, 2)) { 00182 lcd->drawSprite(31,13,22,22,(int *)blueSquareG); 00183 lcd->drawSprite(33,15,18,18,(int *)exit3); // if front square is exit 00184 return; 00185 } 00186 else { 00187 return; // drawing is complete 00188 } 00189 // phase 3 00190 lcd->drawSprite(31,13,22,22,(int *)blueSquareG); 00191 if (player->checkLocation(ghostLocation, -PI/2, 0)) // if the left square is empty 00192 drawBranch5(ghostLocation); 00193 if (player->checkLocation(ghostLocation, PI/2, 0)) 00194 drawBranch6(ghostLocation); 00195 00196 if (player->checkLocation(ghostLocation,0, 0)) { // if the front square is empty 00197 ghostLocation.addVector(player->direction); // move forward 00198 } 00199 else if (player->checkLocation(ghostLocation, 0, 2)) { 00200 lcd->drawSprite(37,19,10,10,(int *)yellowSquareG); 00201 lcd->drawSprite(38,20,8,8,(int *)exit7); // if front square is exit 00202 return; 00203 } 00204 else { 00205 return; // drawing is complete 00206 } 00207 // phase 4 00208 lcd->drawSprite(37,19,10,10,(int *)yellowSquareG); 00209 if (player->checkLocation(ghostLocation, -PI/2, 0)) // if the left square is empty 00210 lcd->drawSprite(37,21,6,2,(int *)yellowBranchG); 00211 if (player->checkLocation(ghostLocation, PI/2, 0)) 00212 lcd->drawSprite(45,21,6,2,(int *)yellowBranchG); 00213 00214 if (player->checkLocation(ghostLocation,0, 0)) { // if the front square is empty 00215 ghostLocation.addVector(player->direction); // move forward 00216 } 00217 else { 00218 return; // drawing is complete 00219 } 00220 // phase 5 00221 lcd->drawSprite(40,22,4,4,(int *)purpleSquareG); 00222 }
Generated on Thu Jul 14 2022 20:06:28 by
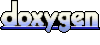