
My ELEC2645 project. Nikollao Sulollari. 200804685
Dependencies: N5110 SoftPWM mbed
Fork of Treasure_Hunt by
main.h
00001 /** 00002 @file main.h 00003 @brief Header file contains functions and variables 00004 @brief Treasure Hunt - Embedded Systems Project 00005 @brief Revision 1.0 00006 @author Nikollao Sulollari 00007 @Date 26/03/2016 00008 */ 00009 00010 #ifndef MAIN_H 00011 #define MAIN_H 00012 #include "mbed.h" 00013 #include "N5110.h" 00014 #include "SoftPWM.h" 00015 00016 #define DIRECTION_TOLERANCE 0.05 00017 /** 00018 @nameSpace r_led 00019 @brief output for status of red LED 00020 */ 00021 DigitalOut r_led(LED_RED); 00022 00023 /** 00024 @namespace g_led 00025 @brief output for status of green LED 00026 */ 00027 DigitalOut g_led(LED_GREEN); 00028 00029 /** 00030 @namespace b_led 00031 @brief output for status of blue LED 00032 */ 00033 DigitalOut b_led(LED_BLUE); 00034 00035 /** 00036 @namespace blue_led 00037 @brief output for status of blue LED, lights when game tool is loaded 00038 */ 00039 DigitalOut blue_led(PTA1); 00040 00041 /** 00042 @namespace led_output 00043 @brief output for status of left tries for the user to win the game 00044 */ 00045 BusOut led_output(PTC2,PTA2,PTB23); 00046 00047 /** 00048 @namespace lcd 00049 @brief object of the N5110 class 00050 */ 00051 N5110 lcd(PTE26 , PTA0 , PTC4 , PTD0 , PTD2 , PTD1 , PTC3); 00052 00053 /** 00054 @namespace pc 00055 @brief serial connection between mbed and pc 00056 */ 00057 Serial pc(USBTX,USBRX); 00058 00059 // K64F on-board switches 00060 InterruptIn sw2(SW2); 00061 InterruptIn sw3(SW3); 00062 00063 00064 /** 00065 @namespace xPot 00066 @brief read x-axis position from the value of the joystick 00067 */ 00068 AnalogIn xPot(PTB2); 00069 00070 /** 00071 @namespace yPot 00072 @brief read y-axis position from the value of the joystick 00073 */ 00074 AnalogIn yPot(PTB3); 00075 00076 /** 00077 @namespace button 00078 @brief interrupt executes an event triggered task 00079 */ 00080 InterruptIn button(PTB10); 00081 00082 /** 00083 @namespace button 00084 @brief interrupt executes an event triggered task 00085 */ 00086 InterruptIn button1(PTB18); 00087 00088 /** 00089 @namespace buzzer 00090 @brief create PWM signal using the SoftPWM library to set buzzer duty cycle and period 00091 */ 00092 SoftPWM buzzer(PTB9); 00093 00094 /** 00095 @namespace ticker 00096 @brief interrupt executes a time-triggered task 00097 */ 00098 Ticker ticker; 00099 00100 /** 00101 @namespace timer 00102 @brief interface is used to measure the time between start and stop 00103 */ 00104 Timer timer; 00105 00106 /** 00107 @namespace game_ticker 00108 @brief interrupt executes a time-triggered task 00109 */ 00110 Ticker game_ticker; 00111 00112 /** 00113 @namespace timeout 00114 @brief interrupt calls a function after a specified amount of time 00115 */ 00116 Timeout timeout; 00117 00118 00119 /** 00120 @namespace DirectionName 00121 @brief define joystick's direction based on its x,y values 00122 */ 00123 enum DirectionName { 00124 UP, 00125 DOWN, 00126 LEFT, 00127 RIGHT, 00128 CENTRE, 00129 UP_LEFT, 00130 UP_RIGHT, 00131 DOWN_LEFT, 00132 DOWN_RIGHT 00133 }; 00134 00135 /** 00136 @namespace Joystick 00137 @brief create strcut Joystick 00138 */ 00139 typedef struct JoyStick Joystick; 00140 struct JoyStick { 00141 double x; /// current x value 00142 double x0; /// 'centred' x value 00143 double y; /// current y value 00144 double y0; /// 'centred' y value 00145 int button; /// button state (assume pull-down used, so 1 = pressed, 0 = unpressed) 00146 DirectionName direction; // current direction 00147 }; 00148 /// create struct variable 00149 Joystick joystick; 00150 00151 /** 00152 The main function where the program takes place 00153 */ 00154 int main(); 00155 00156 /** 00157 set-up serial port 00158 */ 00159 void init_serial(); 00160 00161 /** 00162 set-up the on-board LEDs and switches 00163 */ 00164 void init_K64F(); 00165 00166 /** 00167 Set-up random variables and game menu 00168 */ 00169 void init_game(); 00170 00171 /** 00172 Set-up flag to 1 00173 */ 00174 00175 /** 00176 Set-up flag to 1 00177 */ 00178 void timer_isr(); 00179 00180 /** 00181 Set-up flag to 1 00182 */ 00183 00184 /** 00185 Set-up flag to 1 00186 */ 00187 void game_timer_isr(); 00188 00189 /** 00190 Set-up flag to 1 00191 */ 00192 void sw2_isr(); 00193 00194 /** 00195 Set-up flag to 1 00196 */ 00197 void sw3_isr(); 00198 00199 /** 00200 Set-up flag to 1 00201 */ 00202 void button_isr(); 00203 00204 /** 00205 Set-up flag to 1 00206 */ 00207 void button1_isr(); 00208 00209 /** 00210 Set-up flag to 1 00211 */ 00212 void timeout_isr(); 00213 00214 00215 /** 00216 Set current position to default position of Joystick 00217 */ 00218 void calibrateJoystick(); 00219 00220 /** 00221 Update the values of the joystick to get current position 00222 */ 00223 void updateJoystick(); 00224 00225 /** 00226 Create an enemy rectangular shape 00227 */ 00228 void enemyRect(); 00229 00230 /** 00231 Create an enemy rectangular shape 00232 */ 00233 void enemyRocket(); 00234 00235 /** 00236 Create circle shape enemy 00237 */ 00238 void enemyCircle(); 00239 00240 /** 00241 creates the hero of the game 00242 */ 00243 void hero(); 00244 00245 /** 00246 Shows the right direction 00247 */ 00248 void guidance(); 00249 00250 /** 00251 Locates obstacles in the screen 00252 */ 00253 void obstacles(); 00254 00255 /** 00256 Gets enemies depending on the level 00257 */ 00258 void enemies(); 00259 00260 /** 00261 Implement Menu in the game and display on screen 00262 */ 00263 void menu(); 00264 00265 /** 00266 Check for overlap between hero and enemies or obstacles 00267 */ 00268 void checkOverlap(); 00269 00270 /** 00271 Check for intrersection 00272 @param i loops through x direction 00273 @param j loops through y direction 00274 @returns the number of pixels around the hero detected 00275 */ 00276 int intersection(int i, int j); 00277 00278 00279 //vars 00280 volatile int rectX ; /*!< used to determine x-axis position of the rect enemy */ 00281 00282 volatile int rectY ;/*!< used to determine y-axis position of the rect enemy */ 00283 00284 volatile int circleX ;/*!< used to determine x-axis position of the circular enemy */ 00285 00286 volatile int circleY ;/*!< used to determine y-axis position of the circular enemy */ 00287 00288 volatile int heroX ;/*!< used to move the hero along x-axis */ 00289 00290 volatile int heroY ;/*!< used to move the hero along y-axis */ 00291 00292 volatile int level = 0; /*!< initiate the level difficulty of the game */ 00293 00294 volatile int g_timer_flag = 0; /*!< set timer flag in the isr, timer trigger interrupt */ 00295 00296 volatile int g_game_timer_flag = 0; /*!< set timer flag in the isr, timer trigger interrupt */ 00297 00298 volatile int g_sw2_flag = 0; /*!< set timer flag in the isr, event trigger interrupt */ 00299 00300 volatile int g_sw3_flag = 0; /*!< set timer flag in the isr, event trigger interrupt */ 00301 00302 volatile int g_button_flag = 0; /*!< set flag in the isr, event trigger interrupt */ 00303 00304 volatile int g_button1_flag = 0; /*!< set flag in the isr, event trigger interrupt */ 00305 00306 volatile int option = 0; /*!< select option in menu based on Joystick's movement */ 00307 00308 volatile int play = 0; /*!< counts the number of plays */ 00309 00310 volatile int tries = 3;/*!< counts the number of trials */ 00311 00312 volatile int n ;/*!< number of pixels overlapping */ 00313 00314 volatile float game_speed = 0.05; /*!< value is set to the ticker, allows user to select game speed */ 00315 00316 int fsm [4] = {7,6,4,0}; /*!< array of elements in the FSM, each element is the output of the counter */ 00317 00318 int fsm_state = 0; /*!< variable is used to access the array of states and produce an output */ 00319 00320 int reset = 0; /*!< used to save current level of difficulty */ 00321 00322 int objectX = 0; /*!< used to move obstacles in the x-axis */ 00323 00324 int objectY = 20; /*!< used to move obstacles in the y-axis */ 00325 00326 int state = 0; /*!< controls the direction of the objtacle */ 00327 00328 int objectX1 = 58; /*!< used to move obstacles in the x-axis */ 00329 00330 int state1 = 1; /*!< controls the direction of the objtacle */ 00331 00332 int speed = 1; /*!< controls the speed menu display */ 00333 00334 bool normal =0; /*!< controls the lcd mode selection on menu */ 00335 00336 bool sound = 0; /*!< determines if sound is on or off */ 00337 00338 #endif
Generated on Tue Jul 12 2022 18:32:59 by
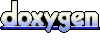