
802.15.4 Power Management using Cyclic Sleep example for mbed XBeeLib By Digi
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * Copyright (c) 2015 Digi International Inc., 00003 * All rights not expressly granted are reserved. 00004 * 00005 * This Source Code Form is subject to the terms of the Mozilla Public 00006 * License, v. 2.0. If a copy of the MPL was not distributed with this file, 00007 * You can obtain one at http://mozilla.org/MPL/2.0/. 00008 * 00009 * Digi International Inc. 11001 Bren Road East, Minnetonka, MN 55343 00010 * ======================================================================= 00011 */ 00012 00013 #include "mbed.h" 00014 #include "XBeeLib.h" 00015 #if defined(ENABLE_LOGGING) 00016 #include "DigiLoggerMbedSerial.h" 00017 using namespace DigiLog; 00018 #endif 00019 00020 using namespace XBeeLib; 00021 00022 /* Configure Sleep Options */ 00023 #define SLEEP_OPTIONS 0 /* Short Sleep */ 00024 00025 /* Configure Sleep Period in mS */ 00026 #define SLEEP_PERIOD_MS (5000 / 10) /* 5000 mS */ 00027 00028 /* Configure Time before sleep */ 00029 #define TIME_BEFORE_SLEEP_MS 500 /* 500 mS */ 00030 00031 Serial *log_serial; 00032 00033 /** Callback function, invoked at packet reception */ 00034 static void receive_cb(const RemoteXBee802& remote, bool broadcast, const uint8_t *const data, uint16_t len) 00035 { 00036 const uint64_t remote_addr64 = remote.get_addr64(); 00037 00038 log_serial->printf("\r\nGot a %s RX packet [%08x:%08x|%04x], len %d\r\nData: ", broadcast ? "BROADCAST" : "UNICAST", 00039 UINT64_HI32(remote_addr64), UINT64_LO32(remote_addr64), remote.get_addr16(), len); 00040 00041 log_serial->printf("%.*s\r\n\r\n", len, data); 00042 } 00043 00044 int main() 00045 { 00046 log_serial = new Serial(DEBUG_TX, DEBUG_RX); 00047 log_serial->baud(9600); 00048 log_serial->printf("Sample application to demo cyclic sleep power management with the XBee802\r\n\r\n"); 00049 log_serial->printf(XB_LIB_BANNER); 00050 00051 #if defined(ENABLE_LOGGING) 00052 new DigiLoggerMbedSerial(log_serial, LogLevelInfo); 00053 #endif 00054 00055 XBee802 xbee = XBee802(RADIO_TX, RADIO_RX, RADIO_RESET, NC, NC, 9600); 00056 00057 /* Register callbacks */ 00058 xbee.register_receive_cb(&receive_cb); 00059 00060 RadioStatus const radioStatus = xbee.init(); 00061 MBED_ASSERT(radioStatus == Success); 00062 00063 /* Configure sleep parameters */ 00064 AtCmdFrame::AtCmdResp cmdresp; 00065 00066 cmdresp = xbee.set_param("A1", 4); /* AutoAssociate = Device attempts Association until success */ 00067 if (cmdresp != AtCmdFrame::AtCmdRespOk) { 00068 log_serial->printf("A1 Failed!!\r\n"); 00069 } 00070 00071 wait_ms(500); 00072 00073 cmdresp = xbee.set_param("SO", (uint32_t)SLEEP_OPTIONS); 00074 if (cmdresp != AtCmdFrame::AtCmdRespOk) { 00075 log_serial->printf("SO Failed!!\r\n"); 00076 } 00077 00078 cmdresp = xbee.set_param("SP", SLEEP_PERIOD_MS); 00079 if (cmdresp != AtCmdFrame::AtCmdRespOk) { 00080 log_serial->printf("SP Failed!!\r\n"); 00081 } 00082 00083 cmdresp = xbee.set_param("ST", TIME_BEFORE_SLEEP_MS); 00084 if (cmdresp != AtCmdFrame::AtCmdRespOk) { 00085 log_serial->printf("ST Failed!!\r\n"); 00086 } 00087 00088 /* Configure Sleep mode */ 00089 cmdresp = xbee.set_param("SM", 4); /* Cyclic Sleep Remote */ 00090 if (cmdresp != AtCmdFrame::AtCmdRespOk) { 00091 log_serial->printf("SM Failed!!\r\n"); 00092 } 00093 00094 /* Start processing frames */ 00095 while (true) { 00096 xbee.process_rx_frames(); 00097 wait_ms(100); 00098 log_serial->printf("."); 00099 } 00100 00101 delete(log_serial); 00102 }
Generated on Mon Jul 18 2022 07:51:49 by
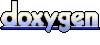