Mouse code for the MacroRat
Platform
Data Structures | |
class | Callback< R()> |
Callback class based on template specialization. More... | |
class | Callback< R(A0)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1, A2)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1, A2, A3)> |
Callback class based on template specialization. More... | |
class | Callback< R(A0, A1, A2, A3, A4)> |
Callback class based on template specialization. More... | |
class | CircularBuffer< T, BufferSize, CounterType > |
Templated Circular buffer class. More... | |
class | CThunk< T > |
Class for created a pointer with data bound to it. More... | |
class | PlatformMutex |
A stub mutex for when an RTOS is not present. More... | |
struct | SingletonPtr< T > |
Utility class for creating an using a singleton. More... | |
struct | transaction_t |
Transaction structure. More... | |
class | Transaction< Class > |
Transaction class defines a transaction. More... | |
Typedefs | |
typedef Callback< void()> * | pFunctionPointer_t |
Group one or more functions in an instance of a CallChain, then call them in sequence using CallChain::call(). | |
typedef void(* | mbed_mem_trace_cb_t )(uint8_t op, void *res, void *caller,...) |
Type of the callback used by the memory tracer. | |
Functions | |
Callback (const Callback< R()> &func) | |
Attach a Callback. | |
template<typename T , typename U > | |
Callback (U *obj, R(T::*method)()) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const U *obj, R(T::*method)() const) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (volatile U *obj, R(T::*method)() volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const volatile U *obj, R(T::*method)() const volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (R(*func)(T *), U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const T *), const U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(volatile T *), volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const volatile T *), const volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename F > | |
Callback (F f, typename detail::enable_if< detail::is_type< R(F::*)(),&F::operator()>::value &&sizeof(F)<=sizeof(uintptr_t) >::type=detail::nil()) | |
Create a Callback with a function object. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
~Callback () | |
Destroy a callback. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(R(*func)()) | |
Attach a static function. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const Callback< R()> &func) | |
Attach a Callback. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(volatile U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const volatile U *obj | |
Attach a static function with a bound pointer. | |
Callback & | operator= (const Callback &that) |
Assign a callback. | |
R | call () const |
Call the attached function. | |
R | operator() () const |
Call the attached function. | |
operator bool () const | |
Test if function has been attached. | |
static R | thunk (void *func) |
Static thunk for passing as C-style function. | |
Callback (const Callback< R(A0)> &func) | |
Attach a Callback. | |
template<typename T , typename U > | |
Callback (U *obj, R(T::*method)(A0)) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const U *obj, R(T::*method)(A0) const) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (volatile U *obj, R(T::*method)(A0) volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const volatile U *obj, R(T::*method)(A0) const volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (R(*func)(T *, A0), U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const T *, A0), const U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(volatile T *, A0), volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const volatile T *, A0), const volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename F > | |
Callback (F f, typename detail::enable_if< detail::is_type< R(F::*)(A0),&F::operator()>::value &&sizeof(F)<=sizeof(uintptr_t) >::type=detail::nil()) | |
Create a Callback with a function object. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
~Callback () | |
Destroy a callback. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(R(*func)(A0)) | |
Attach a static function. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const Callback< R(A0)> &func) | |
Attach a Callback. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(volatile U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const volatile U *obj | |
Attach a static function with a bound pointer. | |
Callback & | operator= (const Callback &that) |
Assign a callback. | |
R | call (A0 a0) const |
Call the attached function. | |
R | operator() (A0 a0) const |
Call the attached function. | |
operator bool () const | |
Test if function has been attached. | |
static R | thunk (void *func, A0 a0) |
Static thunk for passing as C-style function. | |
Callback (const Callback< R(A0, A1)> &func) | |
Attach a Callback. | |
template<typename T , typename U > | |
Callback (U *obj, R(T::*method)(A0, A1)) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const U *obj, R(T::*method)(A0, A1) const) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (volatile U *obj, R(T::*method)(A0, A1) volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const volatile U *obj, R(T::*method)(A0, A1) const volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (R(*func)(T *, A0, A1), U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const T *, A0, A1), const U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(volatile T *, A0, A1), volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const volatile T *, A0, A1), const volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename F > | |
Callback (F f, typename detail::enable_if< detail::is_type< R(F::*)(A0, A1),&F::operator()>::value &&sizeof(F)<=sizeof(uintptr_t) >::type=detail::nil()) | |
Create a Callback with a function object. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
~Callback () | |
Destroy a callback. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(R(*func)(A0 | |
Attach a static function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(volatile U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const volatile U *obj | |
Attach a static function with a bound pointer. | |
Callback & | operator= (const Callback &that) |
Assign a callback. | |
R | call (A0 a0, A1 a1) const |
Call the attached function. | |
R | operator() (A0 a0, A1 a1) const |
Call the attached function. | |
operator bool () const | |
Test if function has been attached. | |
static R | thunk (void *func, A0 a0, A1 a1) |
Static thunk for passing as C-style function. | |
Callback (const Callback< R(A0, A1, A2)> &func) | |
Attach a Callback. | |
template<typename T , typename U > | |
Callback (U *obj, R(T::*method)(A0, A1, A2)) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const U *obj, R(T::*method)(A0, A1, A2) const) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (volatile U *obj, R(T::*method)(A0, A1, A2) volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const volatile U *obj, R(T::*method)(A0, A1, A2) const volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (R(*func)(T *, A0, A1, A2), U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const T *, A0, A1, A2), const U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(volatile T *, A0, A1, A2), volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const volatile T *, A0, A1, A2), const volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename F > | |
Callback (F f, typename detail::enable_if< detail::is_type< R(F::*)(A0, A1, A2),&F::operator()>::value &&sizeof(F)<=sizeof(uintptr_t) >::type=detail::nil()) | |
Create a Callback with a function object. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
~Callback () | |
Destroy a callback. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(R(*func)(A0 | |
Attach a static function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(volatile U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const volatile U *obj | |
Attach a static function with a bound pointer. | |
Callback & | operator= (const Callback &that) |
Assign a callback. | |
R | call (A0 a0, A1 a1, A2 a2) const |
Call the attached function. | |
R | operator() (A0 a0, A1 a1, A2 a2) const |
Call the attached function. | |
operator bool () const | |
Test if function has been attached. | |
static R | thunk (void *func, A0 a0, A1 a1, A2 a2) |
Static thunk for passing as C-style function. | |
Callback (const Callback< R(A0, A1, A2, A3)> &func) | |
Attach a Callback. | |
template<typename T , typename U > | |
Callback (U *obj, R(T::*method)(A0, A1, A2, A3)) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const U *obj, R(T::*method)(A0, A1, A2, A3) const) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (volatile U *obj, R(T::*method)(A0, A1, A2, A3) volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const volatile U *obj, R(T::*method)(A0, A1, A2, A3) const volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (R(*func)(T *, A0, A1, A2, A3), U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const T *, A0, A1, A2, A3), const U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(volatile T *, A0, A1, A2, A3), volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const volatile T *, A0, A1, A2, A3), const volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename F > | |
Callback (F f, typename detail::enable_if< detail::is_type< R(F::*)(A0, A1, A2, A3),&F::operator()>::value &&sizeof(F)<=sizeof(uintptr_t) >::type=detail::nil()) | |
Create a Callback with a function object. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
~Callback () | |
Destroy a callback. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(R(*func)(A0 | |
Attach a static function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(volatile U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const volatile U *obj | |
Attach a static function with a bound pointer. | |
Callback & | operator= (const Callback &that) |
Assign a callback. | |
R | call (A0 a0, A1 a1, A2 a2, A3 a3) const |
Call the attached function. | |
R | operator() (A0 a0, A1 a1, A2 a2, A3 a3) const |
Call the attached function. | |
operator bool () const | |
Test if function has been attached. | |
static R | thunk (void *func, A0 a0, A1 a1, A2 a2, A3 a3) |
Static thunk for passing as C-style function. | |
Callback (const Callback< R(A0, A1, A2, A3, A4)> &func) | |
Attach a Callback. | |
template<typename T , typename U > | |
Callback (U *obj, R(T::*method)(A0, A1, A2, A3, A4)) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const U *obj, R(T::*method)(A0, A1, A2, A3, A4) const) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (volatile U *obj, R(T::*method)(A0, A1, A2, A3, A4) volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (const volatile U *obj, R(T::*method)(A0, A1, A2, A3, A4) const volatile) | |
Create a Callback with a member function. | |
template<typename T , typename U > | |
Callback (R(*func)(T *, A0, A1, A2, A3, A4), U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const T *, A0, A1, A2, A3, A4), const U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(volatile T *, A0, A1, A2, A3, A4), volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
Callback (R(*func)(const volatile T *, A0, A1, A2, A3, A4), const volatile U *arg) | |
Create a Callback with a static function and bound pointer. | |
template<typename F > | |
Callback (F f, typename detail::enable_if< detail::is_type< R(F::*)(A0, A1, A2, A3, A4),&F::operator()>::value &&sizeof(F)<=sizeof(uintptr_t) >::type=detail::nil()) | |
Create a Callback with a function object. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to Callback(func, arg)") Callback(const volatile U *obj | |
Create a Callback with a static function and bound pointer. | |
~Callback () | |
Destroy a callback. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(R(*func)(A0 | |
Attach a static function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by simple assignment 'Callback cb = func") void attach(const volatile U *obj | |
Attach a member function. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(volatile U *obj | |
Attach a static function with a bound pointer. | |
template<typename T , typename U > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to attach(func, arg)") void attach(const volatile U *obj | |
Attach a static function with a bound pointer. | |
Callback & | operator= (const Callback &that) |
Assign a callback. | |
R | call (A0 a0, A1 a1, A2 a2, A3 a3, A4 a4) const |
Call the attached function. | |
R | operator() (A0 a0, A1 a1, A2 a2, A3 a3, A4 a4) const |
Call the attached function. | |
operator bool () const | |
Test if function has been attached. | |
static R | thunk (void *func, A0 a0, A1 a1, A2 a2, A3 a3, A4 a4) |
Static thunk for passing as C-style function. | |
template<typename R > | |
Callback< R()> | callback (R(*func)()=0) |
Create a callback class with type infered from the arguments. | |
template<typename R > | |
Callback< R()> | callback (const Callback< R()> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (U *obj, R(T::*method)()) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (const U *obj, R(T::*method)() const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (volatile U *obj, R(T::*method)() volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (const volatile U *obj, R(T::*method)() const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (R(*func)(T *), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (R(*func)(const T *), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (R(*func)(volatile T *), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
Callback< R()> | callback (R(*func)(const volatile T *), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","Arguments to callback have been reordered to callback(func, arg)") Callback< R()> callback(U *obj | |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(A0)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 > | |
Callback< R(A0)> | callback (const Callback< R(A0)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (U *obj, R(T::*method)(A0)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (const U *obj, R(T::*method)(A0) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (volatile U *obj, R(T::*method)(A0) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (const volatile U *obj, R(T::*method)(A0) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(T *, A0), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(const T *, A0), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(volatile T *, A0), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 > | |
Callback< R(A0)> | callback (R(*func)(const volatile T *, A0), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(A0, A1)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (const Callback< R(A0, A1)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (U *obj, R(T::*method)(A0, A1)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (const U *obj, R(T::*method)(A0, A1) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (volatile U *obj, R(T::*method)(A0, A1) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (const volatile U *obj, R(T::*method)(A0, A1) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(T *, A0, A1), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(const T *, A0, A1), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(volatile T *, A0, A1), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 > | |
Callback< R(A0, A1)> | callback (R(*func)(const volatile T *, A0, A1), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(A0, A1, A2)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (const Callback< R(A0, A1, A2)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (U *obj, R(T::*method)(A0, A1, A2)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (const U *obj, R(T::*method)(A0, A1, A2) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (volatile U *obj, R(T::*method)(A0, A1, A2) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (const volatile U *obj, R(T::*method)(A0, A1, A2) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(T *, A0, A1, A2), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(const T *, A0, A1, A2), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(volatile T *, A0, A1, A2), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 > | |
Callback< R(A0, A1, A2)> | callback (R(*func)(const volatile T *, A0, A1, A2), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(A0, A1, A2, A3)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (const Callback< R(A0, A1, A2, A3)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (U *obj, R(T::*method)(A0, A1, A2, A3)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (const U *obj, R(T::*method)(A0, A1, A2, A3) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (volatile U *obj, R(T::*method)(A0, A1, A2, A3) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (const volatile U *obj, R(T::*method)(A0, A1, A2, A3) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(T *, A0, A1, A2, A3), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(const T *, A0, A1, A2, A3), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(volatile T *, A0, A1, A2, A3), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 > | |
Callback< R(A0, A1, A2, A3)> | callback (R(*func)(const volatile T *, A0, A1, A2, A3), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(A0, A1, A2, A3, A4)=0) |
Create a callback class with type infered from the arguments. | |
template<typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (const Callback< R(A0, A1, A2, A3, A4)> &func) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (U *obj, R(T::*method)(A0, A1, A2, A3, A4)) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (const U *obj, R(T::*method)(A0, A1, A2, A3, A4) const) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (volatile U *obj, R(T::*method)(A0, A1, A2, A3, A4) volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (const volatile U *obj, R(T::*method)(A0, A1, A2, A3, A4) const volatile) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(T *, A0, A1, A2, A3, A4), U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(const T *, A0, A1, A2, A3, A4), const U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(volatile T *, A0, A1, A2, A3, A4), volatile U *arg) |
Create a callback class with type infered from the arguments. | |
template<typename T , typename U , typename R , typename A0 , typename A1 , typename A2 , typename A3 , typename A4 > | |
Callback< R(A0, A1, A2, A3, A4)> | callback (R(*func)(const volatile T *, A0, A1, A2, A3, A4), const volatile U *arg) |
Create a callback class with type infered from the arguments. | |
pFunctionPointer_t | add (Callback< void()> func) |
Add a function at the end of the chain. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The add function does not support cv-qualifiers. Replaced by ""add(callback(obj, method)).") pFunctionPointer_t add(T *obj | |
Add a function at the end of the chain. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The add_front function does not support cv-qualifiers. Replaced by ""add_front(callback(obj, method)).") pFunctionPointer_t add_front(T *obj | |
Add a function at the beginning of the chain. | |
pFunctionPointer_t | get (int i) const |
Get a function object from the chain. | |
int | find (pFunctionPointer_t f) const |
Look for a function object in the call chain. | |
void | clear () |
Clear the call chain (remove all functions in the chain). | |
bool | remove (pFunctionPointer_t f) |
Remove a function object from the chain. | |
void | call () |
Call all the functions in the chain in sequence. | |
void | push (const T &data) |
Push the transaction to the buffer. | |
bool | pop (T &data) |
Pop the transaction from the buffer. | |
bool | empty () |
Check if the buffer is empty. | |
bool | full () |
Check if the buffer is full. | |
void | reset () |
Reset the buffer. | |
void | mbed_start_application (uintptr_t address) |
Start the application at the given address. | |
void | mbed_assert_internal (const char *expr, const char *file, int line) |
Internal mbed assert function which is invoked when MBED_ASSERT macro failes. | |
bool | core_util_are_interrupts_enabled (void) |
Determine the current interrupts enabled state. | |
void | core_util_critical_section_enter (void) |
Mark the start of a critical section. | |
void | core_util_critical_section_exit (void) |
Mark the end of a critical section. | |
bool | core_util_atomic_cas_u8 (uint8_t *ptr, uint8_t *expectedCurrentValue, uint8_t desiredValue) |
Atomic compare and set. | |
bool | core_util_atomic_cas_u16 (uint16_t *ptr, uint16_t *expectedCurrentValue, uint16_t desiredValue) |
Atomic compare and set. | |
bool | core_util_atomic_cas_u32 (uint32_t *ptr, uint32_t *expectedCurrentValue, uint32_t desiredValue) |
Atomic compare and set. | |
bool | core_util_atomic_cas_ptr (void **ptr, void **expectedCurrentValue, void *desiredValue) |
Atomic compare and set. | |
uint8_t | core_util_atomic_incr_u8 (uint8_t *valuePtr, uint8_t delta) |
Atomic increment. | |
uint16_t | core_util_atomic_incr_u16 (uint16_t *valuePtr, uint16_t delta) |
Atomic increment. | |
uint32_t | core_util_atomic_incr_u32 (uint32_t *valuePtr, uint32_t delta) |
Atomic increment. | |
void * | core_util_atomic_incr_ptr (void **valuePtr, ptrdiff_t delta) |
Atomic increment. | |
uint8_t | core_util_atomic_decr_u8 (uint8_t *valuePtr, uint8_t delta) |
Atomic decrement. | |
uint16_t | core_util_atomic_decr_u16 (uint16_t *valuePtr, uint16_t delta) |
Atomic decrement. | |
uint32_t | core_util_atomic_decr_u32 (uint32_t *valuePtr, uint32_t delta) |
Atomic decrement. | |
void * | core_util_atomic_decr_ptr (void **valuePtr, ptrdiff_t delta) |
Atomic decrement. | |
static void | debug (const char *format,...) |
Output a debug message. | |
static void | debug_if (int condition, const char *format,...) |
Conditionally output a debug message. | |
int | mbed_interface_connected (void) |
Functions to control the mbed interface. | |
int | mbed_interface_reset (void) |
Instruct the mbed interface to reset, as if the reset button had been pressed. | |
int | mbed_interface_disconnect (void) |
This will disconnect the debug aspect of the interface, so semihosting will be disabled. | |
int | mbed_interface_powerdown (void) |
This will disconnect the debug aspect of the interface, and if the USB cable is not connected, also power down the interface. | |
int | mbed_interface_uid (char *uid) |
This returns a string containing the 32-character UID of the mbed interface This is a weak function that can be overwritten if required. | |
void | mbed_mac_address (char *mac) |
This returns a unique 6-byte MAC address, based on the interface UID If the interface is not present, it returns a default fixed MAC address (00:02:F7:F0:00:00) | |
void | mbed_die (void) |
Cause the mbed to flash the BLOD (Blue LEDs Of Death) sequence. | |
void | mbed_error_printf (const char *format,...) |
Print out an error message. | |
void | mbed_error_vfprintf (const char *format, va_list arg) |
Print out an error message. | |
void | mbed_mem_trace_set_callback (mbed_mem_trace_cb_t cb) |
Set the callback used by the memory tracer (use NULL for disable tracing). | |
void * | mbed_mem_trace_malloc (void *res, size_t size, void *caller) |
Trace a call to 'malloc'. | |
void * | mbed_mem_trace_realloc (void *res, void *ptr, size_t size, void *caller) |
Trace a call to 'realloc'. | |
void * | mbed_mem_trace_calloc (void *res, size_t num, size_t size, void *caller) |
Trace a call to 'calloc'. | |
void | mbed_mem_trace_free (void *ptr, void *caller) |
Trace a call to 'free'. | |
void | mbed_mem_trace_default_callback (uint8_t op, void *res, void *caller,...) |
Default memory trace callback. | |
void | set_time (time_t t) |
Implementation of the C time.h functions. | |
void | attach_rtc (time_t(*read_rtc)(void), void(*write_rtc)(time_t), void(*init_rtc)(void), int(*isenabled_rtc)(void)) |
Attach an external RTC to be used for the C time functions. | |
static __INLINE void | sleep (void) |
Send the microcontroller to sleep. | |
static __INLINE void | deepsleep (void) |
Send the microcontroller to deep sleep. | |
void | mbed_stats_heap_get (mbed_stats_heap_t *stats) |
Fill the passed in heap stat structure with heap stats. | |
void | mbed_stats_stack_get (mbed_stats_stack_t *stats) |
Fill the passed in structure with stack stats. | |
size_t | mbed_stats_stack_get_each (mbed_stats_stack_t *stats, size_t count) |
Fill the passed array of stat structures with the stack stats for each available stack. | |
static void | singleton_lock (void) |
Lock the singleton mutex. | |
static void | singleton_unlock (void) |
Unlock the singleton mutex. | |
Class * | get_object () |
Get object's instance for the transaction. | |
transaction_t * | get_transaction () |
Get the transaction. | |
Variables | |
size_t | tx_length |
Length of Tx buffer. | |
void * | rx_buffer |
Rx buffer. | |
size_t | rx_length |
Length of Rx buffer. | |
uint32_t | event |
Event for a transaction. | |
event_callback_t | callback |
User's callback. | |
uint8_t | width |
Buffer's word width (8, 16, 32, 64) | |
Friends | |
bool | operator== (const Callback &l, const Callback &r) |
Test for equality. | |
bool | operator!= (const Callback &l, const Callback &r) |
Test for inequality. | |
bool | operator== (const Callback &l, const Callback &r) |
Test for equality. | |
bool | operator!= (const Callback &l, const Callback &r) |
Test for inequality. | |
bool | operator== (const Callback &l, const Callback &r) |
Test for equality. | |
bool | operator!= (const Callback &l, const Callback &r) |
Test for inequality. | |
bool | operator== (const Callback &l, const Callback &r) |
Test for equality. | |
bool | operator!= (const Callback &l, const Callback &r) |
Test for inequality. | |
bool | operator== (const Callback &l, const Callback &r) |
Test for equality. | |
bool | operator!= (const Callback &l, const Callback &r) |
Test for inequality. | |
bool | operator== (const Callback &l, const Callback &r) |
Test for equality. | |
bool | operator!= (const Callback &l, const Callback &r) |
Test for inequality. |
Typedef Documentation
typedef void(* mbed_mem_trace_cb_t)(uint8_t op, void *res, void *caller,...) |
Type of the callback used by the memory tracer.
This callback is called when a memory allocation operation (malloc, realloc, calloc, free) is called and tracing is enabled for that memory allocation function.
- Parameters:
-
op the ID of the operation (MBED_MEM_TRACE_MALLOC, MBED_MEM_TRACE_REALLOC, MBED_MEM_TRACE_CALLOC or MBED_MEM_TRACE_FREE). res the result that the memory operation returned (NULL for 'free'). caller the caller of the memory operation. Note that the value of 'caller' might be unreliable.
The rest of the parameters passed 'mbed_mem_trace_cb_t' are the same as the memory operations that triggered its call (see 'man malloc' for details):
- for malloc: cb(MBED_MEM_TRACE_MALLOC, res, caller, size).
- for realloc: cb(MBED_MEM_TRACE_REALLOC, res, caller, ptr, size).
- for calloc: cb(MBED_MEM_TRACE_CALLOC, res, caller, nmemb, size).
- for free: cb(MBED_MEM_TRACE_FREE, NULL, caller, ptr).
Definition at line 60 of file mbed_mem_trace.h.
typedef Callback<void()>* pFunctionPointer_t |
Group one or more functions in an instance of a CallChain, then call them in sequence using CallChain::call().
Used mostly by the interrupt chaining code, but can be used for other purposes.
Synchronization level: Not protected
Example:
#include "mbed.h" CallChain chain; void first(void) { printf("'first' function.\n"); } void second(void) { printf("'second' function.\n"); } class Test { public: void f(void) { printf("A::f (class member).\n"); } }; int main() { Test test; chain.add(second); chain.add_front(first); chain.add(&test, &Test::f); chain.call(); }
Definition at line 65 of file CallChain.h.
Function Documentation
pFunctionPointer_t add | ( | Callback< void()> | func ) | [inherited] |
Add a function at the end of the chain.
- Parameters:
-
func A pointer to a void function
- Returns:
- The function object created for 'func'
Definition at line 28 of file CallChain.cpp.
void attach_rtc | ( | time_t(*)(void) | read_rtc, |
void(*)(time_t) | write_rtc, | ||
void(*)(void) | init_rtc, | ||
int(*)(void) | isenabled_rtc | ||
) |
Attach an external RTC to be used for the C time functions.
Synchronization level: Thread safe
- Parameters:
-
read_rtc pointer to function which returns current UNIX timestamp write_rtc pointer to function which sets current UNIX timestamp, can be NULL init_rtc pointer to funtion which initializes RTC, can be NULL isenabled_rtc pointer to function wich returns if the rtc is enabled, can be NULL
Definition at line 87 of file mbed_rtc_time.cpp.
R call | ( | A0 | a0, |
A1 | a1, | ||
A2 | a2 | ||
) | const [inherited] |
Call the attached function.
Definition at line 2327 of file Callback.h.
void call | ( | ) | [inherited] |
Call all the functions in the chain in sequence.
Definition at line 108 of file CallChain.cpp.
R call | ( | ) | const [inherited] |
Call the attached function.
Definition at line 542 of file Callback.h.
R call | ( | A0 | a0, |
A1 | a1, | ||
A2 | a2, | ||
A3 | a3 | ||
) | const [inherited] |
Call the attached function.
Definition at line 2922 of file Callback.h.
R call | ( | A0 | a0 ) | const [inherited] |
Call the attached function.
Definition at line 1137 of file Callback.h.
R call | ( | A0 | a0, |
A1 | a1 | ||
) | const [inherited] |
Call the attached function.
Definition at line 1732 of file Callback.h.
R call | ( | A0 | a0, |
A1 | a1, | ||
A2 | a2, | ||
A3 | a3, | ||
A4 | a4 | ||
) | const [inherited] |
Call the attached function.
Definition at line 3517 of file Callback.h.
Callback | ( | volatile U * | obj, |
R(T::*)() volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 112 of file Callback.h.
Callback | ( | R(*)(volatile T *) | func, |
volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 148 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0, A1, A2) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4180 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0, A1, A2) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4191 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1, A2) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4202 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(T *, A0, A1, A2) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4213 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(const T *, A0, A1, A2) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4224 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(volatile T *, A0, A1, A2) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4235 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(const volatile T *, A0, A1, A2) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4246 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(A0, A1, A2, A3) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4317 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | const Callback< R(A0, A1, A2, A3)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4327 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | U * | obj, |
R(T::*)(A0, A1, A2, A3) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4338 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0, A1, A2, A3) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4349 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0, A1, A2, A3) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4360 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1, A2, A3) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4371 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(T *, A0, A1, A2, A3) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4382 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(const T *, A0, A1, A2, A3) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4393 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(volatile T *, A0, A1, A2, A3) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4404 of file Callback.h.
Callback<R(A0, A1, A2, A3)> mbed::callback | ( | R(*)(const volatile T *, A0, A1, A2, A3) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4415 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(A0, A1, A2, A3, A4) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4486 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | const Callback< R(A0, A1, A2, A3, A4)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4496 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4507 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4518 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4529 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4540 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(T *, A0, A1, A2, A3, A4) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4551 of file Callback.h.
Callback | ( | U * | obj, |
R(T::*)() | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 94 of file Callback.h.
Callback | ( | const Callback< R(A0, A1, A2, A3)> & | func ) | [inherited] |
Attach a Callback.
- Parameters:
-
func The Callback to attach
Definition at line 2462 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(const T *, A0, A1, A2, A3, A4) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4562 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(volatile T *, A0, A1, A2, A3, A4) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4573 of file Callback.h.
Callback<R(A0, A1, A2, A3, A4)> mbed::callback | ( | R(*)(const volatile T *, A0, A1, A2, A3, A4) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4584 of file Callback.h.
Callback | ( | U * | obj, |
R(T::*)(A0, A1, A2, A3) | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 2474 of file Callback.h.
Callback | ( | R(*)(const volatile T *) | func, |
const volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 157 of file Callback.h.
Callback | ( | U * | obj, |
R(T::*)(A0, A1) | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 1284 of file Callback.h.
Callback | ( | const U * | obj, |
R(T::*)(A0, A1, A2, A3) const | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 2483 of file Callback.h.
Callback | ( | volatile U * | obj, |
R(T::*)(A0, A1, A2, A3) volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 2492 of file Callback.h.
Callback | ( | const Callback< R(A0, A1)> & | func ) | [inherited] |
Attach a Callback.
- Parameters:
-
func The Callback to attach
Definition at line 1272 of file Callback.h.
Callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1, A2, A3) const volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 2501 of file Callback.h.
Callback | ( | R(*)(T *, A0, A1, A2, A3) | func, |
U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 2510 of file Callback.h.
Callback | ( | const U * | obj, |
R(T::*)(A0, A1) const | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 1293 of file Callback.h.
Callback | ( | R(*)(const T *, A0, A1, A2, A3) | func, |
const U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 2519 of file Callback.h.
Callback | ( | R(*)(volatile T *, A0, A1, A2, A3) | func, |
volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 2528 of file Callback.h.
Callback | ( | volatile U * | obj, |
R(T::*)(A0, A1) volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 1302 of file Callback.h.
Callback | ( | R(*)(const volatile T *, A0, A1, A2, A3) | func, |
const volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 2537 of file Callback.h.
Callback | ( | F | f ) | [inherited] |
Create a Callback with a function object.
- Parameters:
-
func Function object to attach
- Note:
- The function object is limited to a single word of storage
Definition at line 2546 of file Callback.h.
Callback | ( | U * | obj, |
R(T::*)(A0) | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 689 of file Callback.h.
Callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1) const volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 1311 of file Callback.h.
Callback | ( | const Callback< R(A0)> & | func ) | [inherited] |
Attach a Callback.
- Parameters:
-
func The Callback to attach
Definition at line 677 of file Callback.h.
Callback | ( | R(*)(T *, A0, A1) | func, |
U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 1320 of file Callback.h.
Callback | ( | const volatile U * | obj, |
R(T::*)() const volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 121 of file Callback.h.
Callback | ( | const U * | obj, |
R(T::*)(A0) const | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 698 of file Callback.h.
Callback | ( | R(*)(const T *, A0, A1) | func, |
const U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 1329 of file Callback.h.
Callback | ( | R(*)(volatile T *, A0, A1) | func, |
volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 1338 of file Callback.h.
Callback | ( | volatile U * | obj, |
R(T::*)(A0) volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 707 of file Callback.h.
Callback | ( | R(*)(const volatile T *, A0, A1) | func, |
const volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 1347 of file Callback.h.
Callback | ( | F | f ) | [inherited] |
Create a Callback with a function object.
- Parameters:
-
func Function object to attach
- Note:
- The function object is limited to a single word of storage
Definition at line 1356 of file Callback.h.
Callback | ( | F | f ) | [inherited] |
Create a Callback with a function object.
- Parameters:
-
func Function object to attach
- Note:
- The function object is limited to a single word of storage
Definition at line 166 of file Callback.h.
Callback | ( | const volatile U * | obj, |
R(T::*)(A0) const volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 716 of file Callback.h.
Callback | ( | R(*)(T *, A0) | func, |
U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 725 of file Callback.h.
Callback | ( | R(*)(const T *, A0) | func, |
const U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 734 of file Callback.h.
Callback | ( | R(*)(volatile T *, A0) | func, |
volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 743 of file Callback.h.
Callback | ( | const Callback< R(A0, A1, A2, A3, A4)> & | func ) | [inherited] |
Attach a Callback.
- Parameters:
-
func The Callback to attach
Definition at line 3057 of file Callback.h.
Callback | ( | U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 3069 of file Callback.h.
Callback | ( | R(*)(const volatile T *, A0) | func, |
const volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 752 of file Callback.h.
Callback | ( | const U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) const | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 3078 of file Callback.h.
Callback | ( | volatile U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 3087 of file Callback.h.
Callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1, A2, A3, A4) const volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 3096 of file Callback.h.
Callback | ( | R(*)(T *, A0, A1, A2, A3, A4) | func, |
U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 3105 of file Callback.h.
Callback | ( | F | f ) | [inherited] |
Create a Callback with a function object.
- Parameters:
-
func Function object to attach
- Note:
- The function object is limited to a single word of storage
Definition at line 761 of file Callback.h.
Callback | ( | R(*)(const T *, A0, A1, A2, A3, A4) | func, |
const U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 3114 of file Callback.h.
Callback | ( | R(*)(volatile T *, A0, A1, A2, A3, A4) | func, |
volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 3123 of file Callback.h.
Callback | ( | R(*)(const volatile T *, A0, A1, A2, A3, A4) | func, |
const volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 3132 of file Callback.h.
Callback | ( | F | f ) | [inherited] |
Create a Callback with a function object.
- Parameters:
-
func Function object to attach
- Note:
- The function object is limited to a single word of storage
Definition at line 3141 of file Callback.h.
Callback | ( | const U * | obj, |
R(T::*)() const | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 103 of file Callback.h.
Callback | ( | R(*)(T *) | func, |
U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 130 of file Callback.h.
Callback | ( | const Callback< R(A0, A1, A2)> & | func ) | [inherited] |
Attach a Callback.
- Parameters:
-
func The Callback to attach
Definition at line 1867 of file Callback.h.
Callback<R()> mbed::callback | ( | R(*)() | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3641 of file Callback.h.
Callback<R()> mbed::callback | ( | const Callback< R()> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3651 of file Callback.h.
Callback | ( | U * | obj, |
R(T::*)(A0, A1, A2) | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 1879 of file Callback.h.
Callback<R()> mbed::callback | ( | U * | obj, |
R(T::*)() | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3662 of file Callback.h.
Callback<R()> mbed::callback | ( | const U * | obj, |
R(T::*)() const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3673 of file Callback.h.
Callback<R()> mbed::callback | ( | volatile U * | obj, |
R(T::*)() volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3684 of file Callback.h.
Callback<R()> mbed::callback | ( | const volatile U * | obj, |
R(T::*)() const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3695 of file Callback.h.
Callback | ( | const Callback< R()> & | func ) | [inherited] |
Callback | ( | const U * | obj, |
R(T::*)(A0, A1, A2) const | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 1888 of file Callback.h.
Callback | ( | volatile U * | obj, |
R(T::*)(A0, A1, A2) volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 1897 of file Callback.h.
Callback<R()> mbed::callback | ( | R(*)(T *) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3706 of file Callback.h.
Callback<R()> mbed::callback | ( | R(*)(const T *) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3717 of file Callback.h.
Callback<R()> mbed::callback | ( | R(*)(volatile T *) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3728 of file Callback.h.
Callback<R()> mbed::callback | ( | R(*)(const volatile T *) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3739 of file Callback.h.
Callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1, A2) const volatile | method | ||
) | [inherited] |
Create a Callback with a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
Definition at line 1906 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(A0) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3810 of file Callback.h.
Callback | ( | R(*)(T *, A0, A1, A2) | func, |
U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 1915 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | const Callback< R(A0)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3820 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | U * | obj, |
R(T::*)(A0) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3831 of file Callback.h.
Callback | ( | R(*)(const T *, A0, A1, A2) | func, |
const U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 1924 of file Callback.h.
Callback | ( | R(*)(volatile T *, A0, A1, A2) | func, |
volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 1933 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3842 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3853 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 3864 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(T *, A0) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3875 of file Callback.h.
Callback | ( | R(*)(const volatile T *, A0, A1, A2) | func, |
const volatile U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 1942 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(const T *, A0) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3886 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(volatile T *, A0) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3897 of file Callback.h.
Callback | ( | F | f ) | [inherited] |
Create a Callback with a function object.
- Parameters:
-
func Function object to attach
- Note:
- The function object is limited to a single word of storage
Definition at line 1951 of file Callback.h.
Callback<R(A0)> mbed::callback | ( | R(*)(const volatile T *, A0) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 3908 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | U * | obj, |
R(T::*)(A0, A1, A2) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4169 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(A0, A1) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3979 of file Callback.h.
Callback | ( | R(*)(const T *) | func, |
const U * | arg | ||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
func Static function to attach arg Pointer argument to function
Definition at line 139 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | const Callback< R(A0, A1)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 3989 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | U * | obj, |
R(T::*)(A0, A1) | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4000 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | const U * | obj, |
R(T::*)(A0, A1) const | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4011 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | volatile U * | obj, |
R(T::*)(A0, A1) volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4022 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | const volatile U * | obj, |
R(T::*)(A0, A1) const volatile | method | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function method Member function to attach
- Returns:
- Callback with infered type
Definition at line 4033 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(T *, A0, A1) | func, |
U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4044 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(const T *, A0, A1) | func, |
const U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4055 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(volatile T *, A0, A1) | func, |
volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4066 of file Callback.h.
Callback<R(A0, A1)> mbed::callback | ( | R(*)(const volatile T *, A0, A1) | func, |
const volatile U * | arg | ||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach arg Pointer argument to function
- Returns:
- Callback with infered type
Definition at line 4077 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | R(*)(A0, A1, A2) | func = 0 ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4148 of file Callback.h.
Callback<R(A0, A1, A2)> mbed::callback | ( | const Callback< R(A0, A1, A2)> & | func ) |
Create a callback class with type infered from the arguments.
- Parameters:
-
func Static function to attach
- Returns:
- Callback with infered type
Definition at line 4158 of file Callback.h.
void clear | ( | ) | [inherited] |
Clear the call chain (remove all functions in the chain).
Definition at line 86 of file CallChain.cpp.
bool core_util_are_interrupts_enabled | ( | void | ) |
Determine the current interrupts enabled state.
This function can be called to determine whether or not interrupts are currently enabled.
- Note:
- NOTE: This function works for both cortex-A and cortex-M, although the underlyng implementation differs.
- Returns:
- true if interrupts are enabled, false otherwise
Definition at line 31 of file mbed_critical.c.
bool core_util_atomic_cas_ptr | ( | void ** | ptr, |
void ** | expectedCurrentValue, | ||
void * | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value. : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value. [in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
: In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is still updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
Definition at line 308 of file mbed_critical.c.
bool core_util_atomic_cas_u16 | ( | uint16_t * | ptr, |
uint16_t * | expectedCurrentValue, | ||
uint16_t | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value. : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value. [in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
: In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is still updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
Definition at line 109 of file mbed_critical.c.
bool core_util_atomic_cas_u32 | ( | uint32_t * | ptr, |
uint32_t * | expectedCurrentValue, | ||
uint32_t | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value. : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value. [in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
: In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is still updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
Definition at line 122 of file mbed_critical.c.
bool core_util_atomic_cas_u8 | ( | uint8_t * | ptr, |
uint8_t * | expectedCurrentValue, | ||
uint8_t | desiredValue | ||
) |
Atomic compare and set.
It compares the contents of a memory location to a given value and, only if they are the same, modifies the contents of that memory location to a given new value. This is done as a single atomic operation. The atomicity guarantees that the new value is calculated based on up-to-date information; if the value had been updated by another thread in the meantime, the write would fail due to a mismatched expectedCurrentValue.
Refer to https://en.wikipedia.org/wiki/Compare-and-set [which may redirect you to the article on compare-and swap].
- Parameters:
-
ptr The target memory location. [in,out] expectedCurrentValue A pointer to some location holding the expected current value of the data being set atomically. The computed 'desiredValue' should be a function of this current value. : This is an in-out parameter. In the failure case of atomic_cas (where the destination isn't set), the pointee of expectedCurrentValue is updated with the current value. [in] desiredValue The new value computed based on '*expectedCurrentValue'.
- Returns:
- true if the memory location was atomically updated with the desired value (after verifying that it contained the expectedCurrentValue), false otherwise. In the failure case, exepctedCurrentValue is updated with the new value of the target memory location.
pseudocode: function cas(p : pointer to int, old : pointer to int, new : int) returns bool { if *p != *old { *old = *p return false } *p = new return true }
: In the failure case (where the destination isn't set), the value pointed to by expectedCurrentValue is still updated with the current value. This property helps writing concise code for the following incr:
function incr(p : pointer to int, a : int) returns int { done = false value = *p // This fetch operation need not be atomic. while not done { done = atomic_cas(p, &value, value + a) // *value gets updated automatically until success } return value + a }
Definition at line 97 of file mbed_critical.c.
void* core_util_atomic_decr_ptr | ( | void ** | valuePtr, |
ptrdiff_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented in bytes.
- Returns:
- The new decremented value.
- Note:
- The type of the pointer argument is not taken into account and the pointer is decremented by bytes
Definition at line 319 of file mbed_critical.c.
uint16_t core_util_atomic_decr_u16 | ( | uint16_t * | valuePtr, |
uint16_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented.
- Returns:
- The new decremented value.
Definition at line 171 of file mbed_critical.c.
uint32_t core_util_atomic_decr_u32 | ( | uint32_t * | valuePtr, |
uint32_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented.
- Returns:
- The new decremented value.
Definition at line 180 of file mbed_critical.c.
uint8_t core_util_atomic_decr_u8 | ( | uint8_t * | valuePtr, |
uint8_t | delta | ||
) |
Atomic decrement.
- Parameters:
-
valuePtr Target memory location being decremented. delta The amount being decremented.
- Returns:
- The new decremented value.
Definition at line 162 of file mbed_critical.c.
void* core_util_atomic_incr_ptr | ( | void ** | valuePtr, |
ptrdiff_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented in bytes.
- Returns:
- The new incremented value.
- Note:
- The type of the pointer argument is not taken into account and the pointer is incremented by bytes.
Definition at line 315 of file mbed_critical.c.
uint16_t core_util_atomic_incr_u16 | ( | uint16_t * | valuePtr, |
uint16_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented.
- Returns:
- The new incremented value.
Definition at line 143 of file mbed_critical.c.
uint32_t core_util_atomic_incr_u32 | ( | uint32_t * | valuePtr, |
uint32_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented.
- Returns:
- The new incremented value.
Definition at line 152 of file mbed_critical.c.
uint8_t core_util_atomic_incr_u8 | ( | uint8_t * | valuePtr, |
uint8_t | delta | ||
) |
Atomic increment.
- Parameters:
-
valuePtr Target memory location being incremented. delta The amount being incremented.
- Returns:
- The new incremented value.
Definition at line 134 of file mbed_critical.c.
void core_util_critical_section_enter | ( | void | ) |
Mark the start of a critical section.
This function should be called to mark the start of a critical section of code.
- Note:
- NOTES: 1) The use of this style of critical section is targetted at C based implementations. 2) These critical sections can be nested. 3) The interrupt enable state on entry to the first critical section (of a nested set, or single section) will be preserved on exit from the section. 4) This implementation will currently only work on code running in privileged mode.
Definition at line 40 of file mbed_critical.c.
void core_util_critical_section_exit | ( | void | ) |
Mark the end of a critical section.
This function should be called to mark the end of a critical section of code.
- Note:
- NOTES: 1) The use of this style of critical section is targetted at C based implementations. 2) These critical sections can be nested. 3) The interrupt enable state on entry to the first critical section (of a nested set, or single section) will be preserved on exit from the section. 4) This implementation will currently only work on code running in privileged mode.
Definition at line 65 of file mbed_critical.c.
static void debug | ( | const char * | format, |
... | |||
) | [static] |
Output a debug message.
- Parameters:
-
format printf-style format string, followed by variables
Definition at line 35 of file mbed_debug.h.
static void debug_if | ( | int | condition, |
const char * | format, | ||
... | |||
) | [static] |
Conditionally output a debug message.
NOTE: If the condition is constant false (== 0) and the compiler optimization level is greater than 0, then the whole function will be compiled away.
- Parameters:
-
condition output only if condition is true (!= 0) format printf-style format string, followed by variables
Definition at line 53 of file mbed_debug.h.
static __INLINE void deepsleep | ( | void | ) | [static] |
Send the microcontroller to deep sleep.
- Note:
- This function can be a noop if not implemented by the platform.
- This function will only put device to sleep in release mode (small profile or when NDEBUG is defined).
This processor is setup ready for deep sleep, and sent to sleep using __WFI(). This mode has the same sleep features as sleep plus it powers down peripherals and clocks. All state is still maintained.
The processor can only be woken up by an external interrupt on a pin or a watchdog timer.
- Note:
- The mbed interface semihosting is disconnected as part of going to sleep, and can not be restored. Flash re-programming and the USB serial port will remain active, but the mbed program will no longer be able to access the LocalFileSystem
Definition at line 70 of file mbed_sleep.h.
bool empty | ( | ) | [inherited] |
Check if the buffer is empty.
- Returns:
- True if the buffer is empty, false if not
Definition at line 79 of file CircularBuffer.h.
int find | ( | pFunctionPointer_t | f ) | const [inherited] |
Look for a function object in the call chain.
- Parameters:
-
f the function object to search
- Returns:
- The index of the function object if found, -1 otherwise.
Definition at line 73 of file CallChain.cpp.
bool full | ( | ) | [inherited] |
Check if the buffer is full.
- Returns:
- True if the buffer is full, false if not
Definition at line 90 of file CircularBuffer.h.
pFunctionPointer_t get | ( | int | i ) | const [inherited] |
Get a function object from the chain.
- Parameters:
-
i function object index
- Returns:
- The function object at position 'i' in the chain
Definition at line 62 of file CallChain.cpp.
Class* get_object | ( | ) | [inherited] |
Get object's instance for the transaction.
- Returns:
- The object which was stored
Definition at line 58 of file Transaction.h.
transaction_t* get_transaction | ( | ) | [inherited] |
Get the transaction.
- Returns:
- The transaction which was stored
Definition at line 66 of file Transaction.h.
void mbed_assert_internal | ( | const char * | expr, |
const char * | file, | ||
int | line | ||
) |
Internal mbed assert function which is invoked when MBED_ASSERT macro failes.
This function is active only if NDEBUG is not defined prior to including this assert header file. In case of MBED_ASSERT failing condition, error() is called with the assertation message.
- Parameters:
-
expr Expresion to be checked. file File where assertation failed. line Failing assertation line number.
Definition at line 22 of file mbed_assert.c.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2, A3, A4)>(func, arg)" | |||
) | const [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | [inherited] |
Attach a static function.
- Parameters:
-
func Static function to attach
Definition at line 274 of file Callback.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2, A3, A4)>(func, arg)" | |||
) | const volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const [inherited] |
Attach a Callback.
- Parameters:
-
func The Callback to attach
Definition at line 286 of file Callback.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1)>(func, arg)" | |||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2, A3)>(func, arg)" | |||
) | const [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2, A3)>(func, arg)" | |||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2, A3)>(func, arg)" | |||
) | volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2, A3)>(func, arg)" | |||
) | const volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | [inherited] |
Attach a static function.
- Parameters:
-
func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1)>(func, arg)" | |||
) | const [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1)>(func, arg)" | |||
) | volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1)>(func, arg)" | |||
) | const volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2, A3, A4)>(func, arg)" | |||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | [inherited] |
Attach a static function.
- Parameters:
-
func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R()>(func, arg)" | |||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | [inherited] |
Attach a static function.
- Parameters:
-
func Static function to attach
Definition at line 869 of file Callback.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The add_front function does not support cv-qualifiers. Replaced by ""add_front(callback(obj, method))." | |||
) | [inherited] |
Add a function at the beginning of the chain.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called
- Returns:
- The function object created for 'tptr' and 'mptr'
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The add function does not support cv-qualifiers. Replaced by ""add(callback(obj, method))." | |||
) | [inherited] |
Add a function at the end of the chain.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called
- Returns:
- The function object created for 'obj' and 'method'
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R()>(func, arg)" | |||
) | const [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0)>(func, arg)" | |||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2, A3, A4)>(func, arg)" | |||
) | volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0)>(func, arg)" | |||
) | const [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0)>(func, arg)" | |||
) | const volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0)>(func, arg)" | |||
) | volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2)>(func, arg)" | |||
) | const volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2)>(func, arg)" | |||
) | volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | [inherited] |
Attach a static function.
- Parameters:
-
func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const [inherited] |
Attach a Callback.
- Parameters:
-
func The Callback to attach
Definition at line 881 of file Callback.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R()>(func, arg)" | |||
) | const volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const volatile [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to callback(func, arg)" | |||
) |
Create a callback class with type infered from the arguments.
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
- Parameters:
-
obj Optional pointer to object to bind to function func Static function to attach
- Returns:
- Callback with infered type
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R()>(func, arg)" | |||
) | volatile [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | const [inherited] |
Attach a member function.
- Parameters:
-
obj Pointer to object to invoke member function on method Member function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to attach(func, arg)" | |||
) | const volatile [inherited] |
Attach a static function with a bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | ) | [inherited] |
Attach a static function.
- Parameters:
-
func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2)>(func, arg)" | |||
) | [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"Arguments to callback have been reordered to Callback< R(A0, A1, A2)>(func, arg)" | |||
) | const [inherited] |
Create a Callback with a static function and bound pointer.
- Parameters:
-
obj Pointer to object to bind to function func Static function to attach
void mbed_die | ( | void | ) |
Cause the mbed to flash the BLOD (Blue LEDs Of Death) sequence.
Definition at line 29 of file mbed_board.c.
void mbed_error_printf | ( | const char * | format, |
... | |||
) |
Print out an error message.
This is typically called when hanlding a crash.
Synchronization level: Interrupt safe
Definition at line 69 of file mbed_board.c.
void mbed_error_vfprintf | ( | const char * | format, |
va_list | arg | ||
) |
Print out an error message.
Similar to mbed_error_printf but uses a va_list.
Synchronization level: Interrupt safe
Definition at line 76 of file mbed_board.c.
int mbed_interface_connected | ( | void | ) |
Functions to control the mbed interface.
mbed Microcontrollers have a built-in interface to provide functionality such as drag-n-drop download, reset, serial-over-usb, and access to the mbed local file system. These functions provide means to control the interface suing semihost calls it supports. Determine whether the mbed interface is connected, based on whether debug is enabled
- Returns:
- 1 if interface is connected, 0 otherwise
Definition at line 28 of file mbed_interface.c.
int mbed_interface_disconnect | ( | void | ) |
This will disconnect the debug aspect of the interface, so semihosting will be disabled.
The interface will still support the USB serial aspect
- Returns:
- 0 if successful, -1 otherwise (e.g. interface not present)
Definition at line 50 of file mbed_interface.c.
int mbed_interface_powerdown | ( | void | ) |
This will disconnect the debug aspect of the interface, and if the USB cable is not connected, also power down the interface.
If the USB cable is connected, the interface will remain powered up and visible to the host
- Returns:
- 0 if successful, -1 otherwise (e.g. interface not present)
Definition at line 62 of file mbed_interface.c.
int mbed_interface_reset | ( | void | ) |
Instruct the mbed interface to reset, as if the reset button had been pressed.
- Returns:
- 1 if successful, 0 otherwise (e.g. interface not present)
Definition at line 32 of file mbed_interface.c.
int mbed_interface_uid | ( | char * | uid ) |
This returns a string containing the 32-character UID of the mbed interface This is a weak function that can be overwritten if required.
- Parameters:
-
uid A 33-byte array to write the null terminated 32-byte string
- Returns:
- 0 if successful, -1 otherwise (e.g. interface not present)
Definition at line 41 of file mbed_interface.c.
void mbed_mac_address | ( | char * | mac ) |
This returns a unique 6-byte MAC address, based on the interface UID If the interface is not present, it returns a default fixed MAC address (00:02:F7:F0:00:00)
This is a weak function that can be overwritten if you want to provide your own mechanism to provide a MAC address.
- Parameters:
-
mac A 6-byte array to write the MAC address
Definition at line 84 of file mbed_interface.c.
void* mbed_mem_trace_calloc | ( | void * | res, |
size_t | num, | ||
size_t | size, | ||
void * | caller | ||
) |
Trace a call to 'calloc'.
- Parameters:
-
res the result of running 'calloc'. nmemb the 'nmemb' argument given to 'calloc'. size the 'size' argument given to 'calloc'. caller the caller of the memory operation. 'res' (the first argument).
Definition at line 62 of file mbed_mem_trace.c.
void mbed_mem_trace_default_callback | ( | uint8_t | op, |
void * | res, | ||
void * | caller, | ||
... | |||
) |
Default memory trace callback.
DO NOT CALL DIRECTLY. It is meant to be used as the second argument of 'mbed_mem_trace_setup'.
The default callback outputs trace data using 'printf', in a format that's easily parsable by an external tool. For each memory operation, the callback outputs a line that begins with '#<op>:<0xresult>;<0xcaller>-':
- 'op' identifies the memory operation ('m' for 'malloc', 'r' for 'realloc', 'c' for 'calloc' and 'f' for 'free').
- 'result' (base 16) is the result of the memor operation. This is always NULL for 'free', since 'free' doesn't return anything. -'caller' (base 16) is the caller of the memory operation. Note that the value of 'caller' might be unreliable.
The rest of the output depends on the operation being traced:
- for 'malloc': 'size', where 'size' is the original argument to 'malloc'.
- for 'realloc': '0xptr;size', where 'ptr' (base 16) and 'size' are the original arguments to 'realloc'.
- for 'calloc': 'nmemb;size', where 'nmemb' and 'size' are the original arguments to 'calloc'.
- for 'free': '0xptr', where 'ptr' (base 16) is the original argument to 'free'.
Examples:
- 'm:0x20003240;0x600d-50' encodes a 'malloc' that returned 0x20003240, was called by the instruction at 0x600D with a the 'size' argument equal to 50.
- 'f:0x0;0x602f-0x20003240' encodes a 'free' that was called by the instruction at 0x602f with the 'ptr' argument equal to 0x20003240.
Definition at line 81 of file mbed_mem_trace.c.
void mbed_mem_trace_free | ( | void * | ptr, |
void * | caller | ||
) |
Trace a call to 'free'.
- Parameters:
-
ptr the 'ptr' argument given to 'free'. caller the caller of the memory operation.
Definition at line 72 of file mbed_mem_trace.c.
void* mbed_mem_trace_malloc | ( | void * | res, |
size_t | size, | ||
void * | caller | ||
) |
Trace a call to 'malloc'.
- Parameters:
-
res the result of running 'malloc'. size the 'size' argument given to 'malloc'. caller the caller of the memory operation.
- Returns:
- 'res' (the first argument).
Definition at line 42 of file mbed_mem_trace.c.
void* mbed_mem_trace_realloc | ( | void * | res, |
void * | ptr, | ||
size_t | size, | ||
void * | caller | ||
) |
Trace a call to 'realloc'.
- Parameters:
-
res the result of running 'realloc'. ptr the 'ptr' argument given to 'realloc'. size the 'size' argument given to 'realloc'.
- Returns:
- 'res' (the first argument).
Definition at line 52 of file mbed_mem_trace.c.
void mbed_mem_trace_set_callback | ( | mbed_mem_trace_cb_t | cb ) |
Set the callback used by the memory tracer (use NULL for disable tracing).
- Parameters:
-
cb the callback to call on each memory operation.
Definition at line 38 of file mbed_mem_trace.c.
void mbed_start_application | ( | uintptr_t | address ) |
Start the application at the given address.
This function does not return. It is the applications responsibility for flushing to or powering down external components such as filesystems or socket connections before calling this function. For Cortex-M devices this function powers down generic system components such as the NVIC and set the vector table to that of the new image followed by jumping to the reset handler of the new image.
- Parameters:
-
address Starting address of next application to run
Definition at line 28 of file mbed_application.c.
void mbed_stats_heap_get | ( | mbed_stats_heap_t * | stats ) |
Fill the passed in heap stat structure with heap stats.
- Parameters:
-
stats A pointer to the mbed_stats_heap_t structure to fill
Definition at line 57 of file mbed_alloc_wrappers.cpp.
void mbed_stats_stack_get | ( | mbed_stats_stack_t * | stats ) |
Fill the passed in structure with stack stats.
- Parameters:
-
stats A pointer to the mbed_stats_stack_t structure to fill
Definition at line 10 of file mbed_stats.c.
size_t mbed_stats_stack_get_each | ( | mbed_stats_stack_t * | stats, |
size_t | count | ||
) |
Fill the passed array of stat structures with the stack stats for each available stack.
- Parameters:
-
stats A pointer to an array of mbed_stats_stack_t structures to fill count The number of mbed_stats_stack_t structures in the provided array
- Returns:
- The number of mbed_stats_stack_t structures that have been filled, this is equal to the number of stacks on the system.
Definition at line 36 of file mbed_stats.c.
operator bool | ( | ) | const [inherited] |
Test if function has been attached.
Definition at line 1150 of file Callback.h.
operator bool | ( | ) | const [inherited] |
Test if function has been attached.
Definition at line 1745 of file Callback.h.
operator bool | ( | ) | const [inherited] |
Test if function has been attached.
Definition at line 3530 of file Callback.h.
operator bool | ( | ) | const [inherited] |
Test if function has been attached.
Definition at line 2935 of file Callback.h.
operator bool | ( | ) | const [inherited] |
Test if function has been attached.
Definition at line 555 of file Callback.h.
operator bool | ( | ) | const [inherited] |
Test if function has been attached.
Definition at line 2340 of file Callback.h.
R operator() | ( | A0 | a0, |
A1 | a1, | ||
A2 | a2, | ||
A3 | a3, | ||
A4 | a4 | ||
) | const [inherited] |
Call the attached function.
Definition at line 3524 of file Callback.h.
R operator() | ( | A0 | a0, |
A1 | a1, | ||
A2 | a2, | ||
A3 | a3 | ||
) | const [inherited] |
Call the attached function.
Definition at line 2929 of file Callback.h.
R operator() | ( | void | ) | const [inherited] |
Call the attached function.
Definition at line 549 of file Callback.h.
R operator() | ( | A0 | a0, |
A1 | a1, | ||
A2 | a2 | ||
) | const [inherited] |
Call the attached function.
Definition at line 2334 of file Callback.h.
R operator() | ( | A0 | a0, |
A1 | a1 | ||
) | const [inherited] |
Call the attached function.
Definition at line 1739 of file Callback.h.
R operator() | ( | A0 | a0 ) | const [inherited] |
Call the attached function.
Definition at line 1144 of file Callback.h.
Callback& operator= | ( | const Callback< R()> & | that ) | [inherited] |
Assign a callback.
Definition at line 531 of file Callback.h.
Callback& operator= | ( | const Callback< R(A0, A1, A2, A3, A4)> & | that ) | [inherited] |
Assign a callback.
Definition at line 3506 of file Callback.h.
Callback& operator= | ( | const Callback< R(A0, A1)> & | that ) | [inherited] |
Assign a callback.
Definition at line 1721 of file Callback.h.
Callback& operator= | ( | const Callback< R(A0, A1, A2)> & | that ) | [inherited] |
Assign a callback.
Definition at line 2316 of file Callback.h.
Callback& operator= | ( | const Callback< R(A0)> & | that ) | [inherited] |
Assign a callback.
Definition at line 1126 of file Callback.h.
Callback& operator= | ( | const Callback< R(A0, A1, A2, A3)> & | that ) | [inherited] |
Assign a callback.
Definition at line 2911 of file Callback.h.
bool pop | ( | T & | data ) | [inherited] |
Pop the transaction from the buffer.
- Parameters:
-
data Data to be pushed to the buffer
- Returns:
- True if the buffer is not empty and data contains a transaction, false otherwise
Definition at line 62 of file CircularBuffer.h.
void push | ( | const T & | data ) | [inherited] |
Push the transaction to the buffer.
This overwrites the buffer if it's full
- Parameters:
-
data Data to be pushed to the buffer
Definition at line 43 of file CircularBuffer.h.
bool remove | ( | pFunctionPointer_t | f ) | [inherited] |
Remove a function object from the chain.
- f the function object to remove
- Returns:
- true if the function object was found and removed, false otherwise.
Definition at line 96 of file CallChain.cpp.
void reset | ( | ) | [inherited] |
Reset the buffer.
Definition at line 100 of file CircularBuffer.h.
void set_time | ( | time_t | t ) |
Implementation of the C time.h functions.
Provides mechanisms to set and read the current time, based on the microcontroller Real-Time Clock (RTC), plus some standard C manipulation and formating functions.
Example:
#include "mbed.h" int main() { set_time(1256729737); // Set RTC time to Wed, 28 Oct 2009 11:35:37 while(1) { time_t seconds = time(NULL); printf("Time as seconds since January 1, 1970 = %d\n", seconds); printf("Time as a basic string = %s", ctime(&seconds)); char buffer[32]; strftime(buffer, 32, "%I:%M %p\n", localtime(&seconds)); printf("Time as a custom formatted string = %s", buffer); wait(1); } }
Set the current time
Initialises and sets the time of the microcontroller Real-Time Clock (RTC) to the time represented by the number of seconds since January 1, 1970 (the UNIX timestamp).
- Parameters:
-
t Number of seconds since January 1, 1970 (the UNIX timestamp)
Synchronization level: Thread safe
Example:
#include "mbed.h" int main() { set_time(1256729737); // Set time to Wed, 28 Oct 2009 11:35:37 }
Definition at line 68 of file mbed_rtc_time.cpp.
static void singleton_lock | ( | void | ) | [static] |
Lock the singleton mutex.
This function is typically used to provide exclusive access when initializing a global object.
Definition at line 39 of file SingletonPtr.h.
static void singleton_unlock | ( | void | ) | [static] |
Unlock the singleton mutex.
This function is typically used to provide exclusive access when initializing a global object.
Definition at line 52 of file SingletonPtr.h.
static __INLINE void sleep | ( | void | ) | [static] |
Send the microcontroller to sleep.
- Note:
- This function can be a noop if not implemented by the platform.
- This function will only put device to sleep in release mode (small profile or when NDEBUG is defined).
The processor is setup ready for sleep, and sent to sleep using __WFI(). In this mode, the system clock to the core is stopped until a reset or an interrupt occurs. This eliminates dynamic power used by the processor, memory systems and buses. The processor, peripheral and memory state are maintained, and the peripherals continue to work and can generate interrupts.
The processor can be woken up by any internal peripheral interrupt or external pin interrupt.
- Note:
- The mbed interface semihosting is disconnected as part of going to sleep, and can not be restored. Flash re-programming and the USB serial port will remain active, but the mbed program will no longer be able to access the LocalFileSystem
Definition at line 45 of file mbed_sleep.h.
static R thunk | ( | void * | func, |
A0 | a0, | ||
A1 | a1, | ||
A2 | a2, | ||
A3 | a3, | ||
A4 | a4 | ||
) | [static, inherited] |
Static thunk for passing as C-style function.
- Parameters:
-
func Callback to call passed as void pointer
Definition at line 3549 of file Callback.h.
static R thunk | ( | void * | func, |
A0 | a0 | ||
) | [static, inherited] |
Static thunk for passing as C-style function.
- Parameters:
-
func Callback to call passed as void pointer
Definition at line 1169 of file Callback.h.
static R thunk | ( | void * | func, |
A0 | a0, | ||
A1 | a1, | ||
A2 | a2, | ||
A3 | a3 | ||
) | [static, inherited] |
Static thunk for passing as C-style function.
- Parameters:
-
func Callback to call passed as void pointer
Definition at line 2954 of file Callback.h.
static R thunk | ( | void * | func ) | [static, inherited] |
Static thunk for passing as C-style function.
- Parameters:
-
func Callback to call passed as void pointer
Definition at line 574 of file Callback.h.
static R thunk | ( | void * | func, |
A0 | a0, | ||
A1 | a1, | ||
A2 | a2 | ||
) | [static, inherited] |
Static thunk for passing as C-style function.
- Parameters:
-
func Callback to call passed as void pointer
Definition at line 2359 of file Callback.h.
static R thunk | ( | void * | func, |
A0 | a0, | ||
A1 | a1 | ||
) | [static, inherited] |
Static thunk for passing as C-style function.
- Parameters:
-
func Callback to call passed as void pointer
Definition at line 1764 of file Callback.h.
~Callback | ( | ) | [inherited] |
Destroy a callback.
Definition at line 1453 of file Callback.h.
~Callback | ( | ) | [inherited] |
Destroy a callback.
Definition at line 2643 of file Callback.h.
~Callback | ( | ) | [inherited] |
Destroy a callback.
Definition at line 858 of file Callback.h.
~Callback | ( | ) | [inherited] |
Destroy a callback.
Definition at line 263 of file Callback.h.
~Callback | ( | ) | [inherited] |
Destroy a callback.
Definition at line 2048 of file Callback.h.
~Callback | ( | ) | [inherited] |
Destroy a callback.
Definition at line 3238 of file Callback.h.
Variable Documentation
event_callback_t callback [inherited] |
User's callback.
Definition at line 34 of file Transaction.h.
uint32_t event [inherited] |
Event for a transaction.
Definition at line 33 of file Transaction.h.
void* rx_buffer [inherited] |
Rx buffer.
Definition at line 31 of file Transaction.h.
size_t rx_length [inherited] |
Length of Rx buffer.
Definition at line 32 of file Transaction.h.
size_t tx_length [inherited] |
Length of Tx buffer.
Definition at line 30 of file Transaction.h.
uint8_t width [inherited] |
Buffer's word width (8, 16, 32, 64)
Definition at line 35 of file Transaction.h.
Friends
bool operator!= | ( | const Callback< R()> & | l, |
const Callback< R()> & | r | ||
) | [friend, inherited] |
Test for inequality.
Definition at line 567 of file Callback.h.
bool operator!= | ( | const Callback< R(A0)> & | l, |
const Callback< R(A0)> & | r | ||
) | [friend, inherited] |
Test for inequality.
Definition at line 1162 of file Callback.h.
bool operator!= | ( | const Callback< R(A0, A1, A2)> & | l, |
const Callback< R(A0, A1, A2)> & | r | ||
) | [friend, inherited] |
Test for inequality.
Definition at line 2352 of file Callback.h.
bool operator!= | ( | const Callback< R(A0, A1, A2, A3)> & | l, |
const Callback< R(A0, A1, A2, A3)> & | r | ||
) | [friend, inherited] |
Test for inequality.
Definition at line 2947 of file Callback.h.
bool operator!= | ( | const Callback< R(A0, A1)> & | l, |
const Callback< R(A0, A1)> & | r | ||
) | [friend, inherited] |
Test for inequality.
Definition at line 1757 of file Callback.h.
bool operator!= | ( | const Callback< R(A0, A1, A2, A3, A4)> & | l, |
const Callback< R(A0, A1, A2, A3, A4)> & | r | ||
) | [friend, inherited] |
Test for inequality.
Definition at line 3542 of file Callback.h.
bool operator== | ( | const Callback< R(A0, A1, A2)> & | l, |
const Callback< R(A0, A1, A2)> & | r | ||
) | [friend, inherited] |
Test for equality.
Definition at line 2346 of file Callback.h.
bool operator== | ( | const Callback< R(A0, A1, A2, A3)> & | l, |
const Callback< R(A0, A1, A2, A3)> & | r | ||
) | [friend, inherited] |
Test for equality.
Definition at line 2941 of file Callback.h.
bool operator== | ( | const Callback< R(A0, A1, A2, A3, A4)> & | l, |
const Callback< R(A0, A1, A2, A3, A4)> & | r | ||
) | [friend, inherited] |
Test for equality.
Definition at line 3536 of file Callback.h.
bool operator== | ( | const Callback< R(A0)> & | l, |
const Callback< R(A0)> & | r | ||
) | [friend, inherited] |
Test for equality.
Definition at line 1156 of file Callback.h.
bool operator== | ( | const Callback< R()> & | l, |
const Callback< R()> & | r | ||
) | [friend, inherited] |
Test for equality.
Definition at line 561 of file Callback.h.
bool operator== | ( | const Callback< R(A0, A1)> & | l, |
const Callback< R(A0, A1)> & | r | ||
) | [friend, inherited] |
Test for equality.
Definition at line 1751 of file Callback.h.
Generated on Tue Jul 12 2022 17:41:26 by
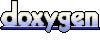