
uses pushing box to write to google spreadsheets
Dependencies: GSM_PUSHING_BOX_STATE_MACHINE MBed_Adafruit-GPS-Library SDFileSystem mbed
Fork of DCS by
Sensor.cpp
00001 #pragma once 00002 #include "mbed.h" 00003 #include "math.h" 00004 #include "MBed_Adafruit_GPS.h" 00005 #include "SDFileSystem.h" 00006 #include "QAM.h" 00007 #include "param.h" 00008 #include "GSMLibrary.h" 00009 #include "stdlib.h" 00010 #include "GPRSInterface.h" 00011 00012 DigitalOut led_red(LED_RED); 00013 Serial pc(USBTX, USBRX); 00014 Timer t; 00015 bool run = 0; 00016 bool fil = 0; 00017 00018 /************************************************** 00019 ** SD FILE SYSTEM ** 00020 **************************************************/ 00021 SDFileSystem sd(PTE3, PTE1, PTE2, PTE4, "sd"); 00022 FILE *fpLog; 00023 FILE *fpData; 00024 /************************************************** 00025 ** GPS ** 00026 **************************************************/ 00027 Serial * gps_Serial; 00028 00029 /************************************************** 00030 ** SENSOR INPUTS ** 00031 **************************************************/ 00032 AnalogIn AnLong(A0); 00033 AnalogIn AnRefLong(A2); 00034 Ticker sample_tick; 00035 bool takeSample = false; 00036 void tick() 00037 { 00038 takeSample = true; 00039 } 00040 00041 /************************************************** 00042 ** SIN OUTPUT ** 00043 **************************************************/ 00044 AnalogOut dac0(DAC0_OUT); 00045 int sinRes = (int)1/(CARRIER_FREQ*TIME_CONST); 00046 float sinWave[SIN_LENGTH] = {}; 00047 int sinIndex = 0; 00048 00049 00050 /************************************************** 00051 ** QAM ** 00052 **************************************************/ 00053 float sLQ[SAMPLE_LENGTH] = {}; 00054 float sLI[SAMPLE_LENGTH] = {}; 00055 float sRefLQ[SAMPLE_LENGTH] = {}; 00056 float sRefLI[SAMPLE_LENGTH] = {}; 00057 00058 float Iarray[SAMPLE_LENGTH] = {}; 00059 float Qarray[SAMPLE_LENGTH] = {}; 00060 int sampleIndex = 0; 00061 float I = 0; 00062 float Q = 0; 00063 float lon = 0; 00064 float lonRef = 0; 00065 00066 /*Global Variables*/ 00067 extern GPRSInterface eth; 00068 00069 /*End Global Variables*/ 00070 void buildIQ() 00071 { 00072 for(int i = 0; i < SAMPLE_LENGTH; i++) { 00073 Iarray[i] = cos(2*PI*CARRIER_FREQ*i*TIME_CONST); 00074 Qarray[i] = -sin(2*PI*CARRIER_FREQ*i*TIME_CONST); 00075 } 00076 } 00077 00078 void create_sinWave() 00079 { 00080 int i = 0; 00081 for(i = 0; i < SIN_LENGTH; i++) { 00082 sinWave[i] = 0.25 * sin(2.0*PI*i/sinRes) + 0.75; 00083 } 00084 } 00085 00086 int main () 00087 { 00088 pc.printf("hello\r\n"); 00089 //Initilialize the GSM 00090 //gsm_initialize(); 00091 // GPS INITIALIZATION ////////////////////////////// 00092 gps_Serial = new Serial(PTC4,PTC3); ////Serial gsm(D1,D0); 00093 Adafruit_GPS myGPS(gps_Serial); 00094 char c; 00095 myGPS.begin(9600); 00096 myGPS.sendCommand(PMTK_SET_NMEA_OUTPUT_RMCGGA); 00097 myGPS.sendCommand(PMTK_SET_NMEA_UPDATE_1HZ); 00098 myGPS.sendCommand(PGCMD_ANTENNA); 00099 //////////////////////////////////////////////////// 00100 00101 buildIQ(); 00102 create_sinWave(); 00103 00104 float filteredLong = 0; 00105 float filteredLongRef = 0; 00106 00107 fpData = fopen("/sd/data.txt", "a"); 00108 if (fpData != NULL) { 00109 fprintf(fpData, "--------------- DCS ------------------"); 00110 } 00111 00112 00113 void gsm_initialize(); 00114 00115 sample_tick.attach(&tick, 0.0001); 00116 00117 t.start(); 00118 00119 while(1) { 00120 00121 if(takeSample) { 00122 00123 dac0 = sinWave[sinIndex]; 00124 00125 lon = AnLong.read(); 00126 lonRef = AnRefLong.read(); 00127 00128 I = Iarray[sampleIndex]; 00129 Q = Qarray[sampleIndex]; 00130 sLI[sampleIndex] = lon*I; 00131 sLQ[sampleIndex] = lon*Q; 00132 sRefLI[sampleIndex] = lonRef*I; 00133 sRefLQ[sampleIndex] = lonRef*Q; 00134 00135 takeSample = false; 00136 00137 sinIndex++; 00138 if((sinIndex+1) > SIN_LENGTH) { 00139 sinIndex = 0; 00140 } 00141 00142 00143 sampleIndex++; 00144 if(sampleIndex+1 > SAMPLE_LENGTH) { 00145 fil = 1; 00146 } 00147 00148 00149 } 00150 00151 if(fil==1) { 00152 00153 fil = 0; 00154 run = 1; 00155 00156 sampleIndex = 0; 00157 00158 gsm_tick(); 00159 00160 filteredLong = 15*QAM(sLI, sLQ); 00161 filteredLongRef = QAM(sRefLI, sRefLQ); 00162 00163 gsm_tick(); 00164 00165 } 00166 c = myGPS.read(); 00167 if ( myGPS.newNMEAreceived() ) { 00168 if ( !myGPS.parse(myGPS.lastNMEA()) ) { 00169 //continue; 00170 } 00171 } 00172 00173 if(run) { 00174 led_red = !led_red; 00175 run = 0; 00176 00177 pc.printf("%f, ", filteredLong); 00178 pc.printf("%f, ", filteredLongRef); 00179 pc.printf("%f\r\n", filteredLong/filteredLongRef); 00180 pc.printf("%02d:%02d:%02d \r\n", myGPS.hour-6, myGPS.minute, myGPS.seconds); 00181 00182 if (myGPS.fix) pc.printf("%5.2f%c, %5.2f%c\r\n", myGPS.latitude, myGPS.lat, myGPS.longitude, myGPS.lon); 00183 else pc.printf("No GPS fix\r\n"); 00184 pc.printf("--------------------------------\r\n"); 00185 00186 fpData = fopen("/sd/data.txt", "a"); 00187 if (fpData != NULL) { 00188 fprintf(fpData, "%f, ", filteredLong); 00189 fprintf(fpData, "%f\r\n", filteredLongRef); 00190 fprintf(fpData, "%02d:%02d:%02d\r\n", myGPS.hour-6, myGPS.minute, myGPS.seconds); 00191 if (myGPS.fix) fprintf(fpData, "%5.2f%c, %5.2f%c\r\n", myGPS.latitude, myGPS.lat, myGPS.longitude, myGPS.lon); 00192 fclose(fpData); 00193 } 00194 if(myGPS.fix) 00195 gsm_send_data(filteredLong, filteredLongRef, myGPS.hour-6, myGPS.minute, myGPS.seconds, myGPS.latitude, myGPS.lat, myGPS.longitude, myGPS.lon); 00196 else 00197 gsm_send_data(filteredLong, filteredLongRef,myGPS.hour-6, myGPS.minute, myGPS.seconds, 0, 0, 0, 0); 00198 00199 } 00200 00201 } 00202 }
Generated on Thu Jul 14 2022 02:13:22 by
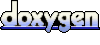