
uses pushing box to write to google spreadsheets
Dependencies: GSM_PUSHING_BOX_STATE_MACHINE MBed_Adafruit-GPS-Library SDFileSystem mbed
Fork of DCS by
GPRS.cpp
00001 /* 00002 GPRS.cpp 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-2-24 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #include "mbed.h" 00024 #include "GPRS.h" 00025 00026 GPRS* GPRS::inst; 00027 char* numbers[100]; 00028 FILE *fpNum; 00029 int number_index = 0; 00030 char phone_number[12]; 00031 00032 GPRS::GPRS(PinName tx, PinName rx, int baudRate, const char* apn, const char* userName, const char* passWord) : Modem(tx,rx,baudRate) 00033 { 00034 inst = this; 00035 _apn = apn; 00036 _userName = userName; 00037 _passWord = passWord; 00038 socketID = -1; 00039 } 00040 00041 bool GPRS::preInit() 00042 { 00043 //send AT command a couple of times 00044 for(int i = 0; i < 2; i++) { 00045 sendCmd("AT\r\n"); 00046 } 00047 return checkSIMStatus(); 00048 } 00049 // added by Noah Milam 00050 void GPRS::start_server() 00051 { 00052 sendCmdResp("AT+CGATT?\r\n"); 00053 00054 sendCmdResp("AT+CIPSERVER=1,1234\r\n"); 00055 listen_server(); 00056 } 00057 void GPRS::listen_server() 00058 { 00059 gprs_response(); 00060 } 00061 00062 void GPRS::send_SMS(char* number, char* data) 00063 { 00064 printf("sending text message to: %s\r\n", number); 00065 sendCmdAndWaitForResp("AT+CMGF=1\r\n","OK",DEFAULT_TIMEOUT,CMD); 00066 wait(2); 00067 //printf("\032\n"); 00068 char cmd[64]; 00069 snprintf(cmd,sizeof(cmd),"AT+CMGS=\"+%s\"\r\n",number); 00070 printf(">>>>%s",cmd); 00071 sendCmdAndWaitForResp(cmd,">",DEFAULT_TIMEOUT,CMD); 00072 printf("sent at cmgf\n"); 00073 wait(2); 00074 //printf("032"); 00075 sendCmd(data); // sends the address 00076 sendCmd("\x1a"); // this is like pressing control - z to end the send command 00077 wait(10); // giving the send enough time to do its thing 00078 printf("should have been received"); 00079 } 00080 00081 char* GPRS::read_SMS() 00082 { 00083 wait(1); 00084 sendCmd("AT+CMGF=1\r\n"); // sms mode 00085 wait(2); 00086 sendCmd("AT+CMGD=1\r\n"); // delete the frist message so incoming message is spot 1 00087 wait(1); 00088 wait_for_sms(); 00089 wait(10); 00090 sendCmd("AT+CMGR=1\r\n"); 00091 storeResp(); 00092 return get_server_IP(); 00093 } 00094 00095 // end of what Noah Milam added 00096 bool GPRS::checkSIMStatus(void) 00097 { 00098 printf("Checking SIM Status...\r\n"); 00099 char gprsBuffer[32]; 00100 int count = 0; 00101 00102 while(count < 3) { 00103 cleanBuffer(gprsBuffer,32); 00104 sendCmd("AT+CPIN?\r\n"); 00105 readBuffer(gprsBuffer,32,DEFAULT_TIMEOUT); 00106 if((NULL != strstr(gprsBuffer,"+CPIN: READY"))) { 00107 break; 00108 } 00109 printf("SIM Not Ready..Try Again\r\n--%s--\r\n", gprsBuffer); 00110 count++; 00111 wait(1); 00112 } 00113 if(count == 3) { 00114 return false; 00115 } 00116 printf("SIM Status GOOD!\r\n"); 00117 return true; 00118 } 00119 00120 bool GPRS::join() 00121 { 00122 char cmd[64]; 00123 char ipAddr[32]; 00124 char resp[96]; 00125 00126 printf(">>>>AT+CREG\r\n"); 00127 sendCmd("AT+CREG?\r\n"); 00128 cleanBuffer(resp,96); 00129 readBuffer(resp,96,DEFAULT_TIMEOUT); 00130 if(NULL != strstr(resp,"+CREG:")) { 00131 } 00132 else{ 00133 return false; 00134 } 00135 //close any existing connections 00136 printf(">>>>AT+CIPSHUT\r\n"); 00137 sendCmd("AT+CIPSHUT\r\n"); 00138 cleanBuffer(resp,96); 00139 readBuffer(resp,96,DEFAULT_TIMEOUT); 00140 if(NULL != strstr(resp,"OK")) { 00141 } 00142 else{ 00143 return false; 00144 } 00145 //Select multi connection (it can be a server or client) 00146 printf(">>>>AT+CIPMUX=0\r\n"); 00147 sendCmd("AT+CIPMUX=0 \r\n"); 00148 cleanBuffer(resp,96); 00149 readBuffer(resp,96,DEFAULT_TIMEOUT); 00150 if(NULL != strstr(resp,"CIPMUX")) { 00151 } 00152 else{ 00153 return false; 00154 } 00155 //get signal strength 00156 printf(">>>>AT+CSQ\r\n"); 00157 sendCmd("AT+CSQ\r\n"); 00158 cleanBuffer(resp,96); 00159 readBuffer(resp,96,DEFAULT_TIMEOUT); 00160 if(NULL != strstr(resp,"+CSQ:")) { 00161 } 00162 else{ 00163 return false; 00164 } 00165 //check if device is attached 00166 printf(">>>>AT+CGATT?\r\n"); 00167 sendCmd("AT+CGATT?\r\n"); 00168 cleanBuffer(resp,96); 00169 readBuffer(resp,96,DEFAULT_TIMEOUT); 00170 if(NULL != strstr(resp,"+CGATT:")) { 00171 } 00172 else{ 00173 return false; 00174 } 00175 //attach the device 00176 printf(">>>>AT+CGATT=1\r\n"); 00177 sendCmd("AT+CGATT=1\r\n"); 00178 cleanBuffer(resp,96); 00179 readBuffer(resp,96,DEFAULT_TIMEOUT); 00180 if(NULL != strstr(resp,"OK")) { 00181 } 00182 else{ 00183 printf("Response: %s\r\n", resp); 00184 return false; 00185 } 00186 00187 //set APN 00188 snprintf(cmd,sizeof(cmd),"AT+CSTT=\"%s\",\"%s\",\"%s\"\r\n",_apn,_userName,_passWord); 00189 printf(">>>>%s",cmd); 00190 //sendCmdAndWaitForRes p(cmd, "OK", DEFAULT_TIMEOUT,CMD); 00191 sendCmd(cmd); 00192 cleanBuffer(resp,96); 00193 readBuffer(resp,96,DEFAULT_TIMEOUT); 00194 if(NULL != strstr(resp,"OK")) { 00195 } 00196 else{ 00197 return false; 00198 } 00199 //Brings up wireless connection 00200 printf(">>>>AT+CIICR\r\n"); 00201 sendCmd("AT+CIICR\r\n"); 00202 cleanBuffer(resp,96); 00203 readBuffer(resp,96,DEFAULT_TIMEOUT); 00204 if(NULL != strstr(resp,"OK")) { 00205 } 00206 else{ 00207 return false; 00208 } 00209 printf(">>>>AT+CIPHEAD\r\n"); 00210 sendCmd("AT+CIPHEAD=1\r\n"); 00211 cleanBuffer(resp,96); 00212 readBuffer(resp,96,DEFAULT_TIMEOUT); 00213 if(NULL != strstr(resp,"OK")) { 00214 } 00215 else{ 00216 return false; 00217 } 00218 //Get local IP address 00219 printf(">>>>AT+CIFSR\r\n"); 00220 sendCmd("AT+CIFSR\r\n"); 00221 readBuffer(ipAddr,32,2); 00222 printf(">>>>AT+CIFSR returns: %s\r\n", ipAddr); 00223 if(NULL != strstr(ipAddr,"AT+CIFSR")) { 00224 _ip = str_to_ip(ipAddr+12); 00225 if(_ip != 0) { 00226 //possibly send ip address to server for future TCP connections 00227 return true; 00228 } 00229 } 00230 return false; 00231 } 00232 00233 bool GPRS::setProtocol(int socket, Protocol p) 00234 { 00235 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00236 return false; 00237 } 00238 //ToDo: setProtocol 00239 return true; 00240 } 00241 00242 bool GPRS::connect(int socket, Protocol ptl,const char * host, int port, int timeout) 00243 { 00244 char cmd[64]; 00245 char resp[96]; 00246 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00247 return false; 00248 } 00249 if(ptl == TCP) { 00250 sprintf(cmd, "AT+CIPSTART=\"TCP\",\"%s\",\"%d\"\r\n", host, port); 00251 } else if(ptl == UDP) { 00252 sprintf(cmd, "AT+CIPSTART=%d,\"UDP\",\"%s\",%d\r\n",socket, host, port); 00253 } else { 00254 return false; 00255 } 00256 00257 sendCmd(cmd); 00258 readBuffer(resp,96,DEFAULT_TIMEOUT); 00259 if(NULL != strstr(resp,"CONNECT")) { //ALREADY CONNECT or CONNECT OK 00260 printf("Response: %s\r\n", resp); 00261 return true; 00262 } 00263 return false;//ERROR 00264 } 00265 00266 bool GPRS::gethostbyname(const char* host, uint32_t* ip) 00267 { 00268 uint32_t addr = str_to_ip(host); 00269 char buf[17]; 00270 snprintf(buf, sizeof(buf), "%d.%d.%d.%d", (addr>>24)&0xff, (addr>>16)&0xff, (addr>>8)&0xff, addr&0xff); 00271 if (strcmp(buf, host) == 0) { 00272 *ip = addr; 00273 return true; 00274 } 00275 return false; 00276 } 00277 00278 bool GPRS::disconnect() 00279 { 00280 sendCmd("AT+CIPSHUT\r\n"); 00281 return true; 00282 } 00283 00284 bool GPRS::is_connected(int socket) 00285 { 00286 00287 char cmd[16]; 00288 char resp[96]; 00289 while(1){ 00290 snprintf(cmd,16,"AT+CIPSTATUS=%d\r\n",socket); 00291 sendCmd(cmd); 00292 readBuffer(resp,sizeof(resp),DEFAULT_TIMEOUT); 00293 if(NULL != strstr(resp,"CONNECTED")) { 00294 return true; 00295 } else { 00296 return false; 00297 } 00298 } 00299 } 00300 00301 void GPRS::reset() 00302 { 00303 } 00304 00305 bool GPRS::close(int socket) 00306 { 00307 char cmd[16]; 00308 char resp[16]; 00309 00310 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00311 return false; 00312 } 00313 // if not connected, return 00314 if (is_connected(socket) == false) { 00315 return true; 00316 } 00317 snprintf(cmd, sizeof(cmd),"AT+CIPCLOSE=%d\r\n",socket); 00318 snprintf(resp,sizeof(resp),"%d, CLOSE OK",socket); 00319 if(0 != sendCmdAndWaitForResp(cmd, resp, DEFAULT_TIMEOUT,CMD)) { 00320 return false; 00321 } 00322 return true; 00323 } 00324 00325 bool GPRS::readable(void) 00326 { 00327 return readable(); 00328 } 00329 00330 int GPRS::wait_readable(int socket, int wait_time) 00331 { 00332 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00333 return -1; 00334 } 00335 char resp[16]; 00336 snprintf(resp,sizeof(resp),"\r\n\r\n+RECEIVE,%d",socket);//"+RECEIVE:<socketID>,<length>" 00337 int len = strlen(resp); 00338 00339 if(false == respCmp(resp,len,wait_time)) { 00340 return -1; 00341 } 00342 char c = readByte();//',' 00343 char dataLen[4]; 00344 int i = 0; 00345 c = readByte(); 00346 while((c >= '0') && (c <= '9')) { 00347 dataLen[i++] = c; 00348 c = readByte(); 00349 } 00350 c = readByte();//'\n' 00351 len = atoi(dataLen); 00352 return len; 00353 } 00354 00355 int GPRS::wait_writeable(int socket, int req_size) 00356 { 00357 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00358 return -1; 00359 } 00360 return req_size>256?256:req_size+1; 00361 } 00362 00363 int GPRS::send(int socket, const char * str, int len) 00364 { 00365 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00366 return -1; 00367 } 00368 char cmd[32]; 00369 wait(1); 00370 if(len > 0){ 00371 printf("Sending data: %s\r\n", str); 00372 snprintf(cmd,sizeof(cmd),"AT+CIPSEND\r\n"); 00373 if(0 != sendCmdAndWaitForResp(cmd,">",2,CMD)) { 00374 printf("Couldn't send data!!\r\n"); 00375 return false; 00376 } 00377 sendCmd(str); 00378 serialModem.putc((char)0x1a); 00379 } 00380 return len; 00381 } 00382 00383 int GPRS::recv(int socket, char* buf, int len) 00384 { 00385 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00386 return -1; 00387 } 00388 cleanBuffer(buf,len); 00389 readBuffer(buf,len,DEFAULT_TIMEOUT/2); 00390 return len; 00391 //return strlen(buf); 00392 } 00393 00394 int GPRS::new_socket() 00395 { 00396 socketID = 0; //we only support one socket. 00397 return socketID; 00398 } 00399 00400 uint16_t GPRS::new_port() 00401 { 00402 uint16_t port = rand(); 00403 port |= 49152; 00404 return port; 00405 } 00406 00407 uint32_t GPRS::str_to_ip(const char* str) 00408 { 00409 uint32_t ip = 0; 00410 char* p = (char*)str; 00411 for(int i = 0; i < 4; i++) { 00412 ip |= atoi(p); 00413 p = strchr(p, '.'); 00414 if (p == NULL) { 00415 break; 00416 } 00417 ip <<= 8; 00418 p++; 00419 } 00420 return ip; 00421 }
Generated on Thu Jul 14 2022 02:13:22 by
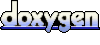