
works through pushing box to log data to google spreadsheet
Dependencies: MBed_Adafruit-GPS-Library SDFileSystem mbed
Fork of GPR_Interface by
modem.cpp
00001 /* 00002 modem.cpp 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-2-24 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #include "modem.h" 00024 //Serial pc(USBTX,USBRX); 00025 SDFileSystem sd(PTE3, PTE1, PTE2, PTE4, "sd"); //MOSI, MISO, SCLK, SSEL. Tested on K64F, correct pins. 00026 AnalogIn LM35(PTB2); 00027 // added by Noah Milam 00028 void Modem::gprs_response() 00029 { 00030 printf("\nlistening for client\n"); 00031 char buffer[100]; 00032 int count = 0; 00033 00034 mkdir("/sd/mydir", 0777); // makes directory if needed 00035 FILE *fp = fopen("/sd/mydir/chemData.csv", "w"); // creats new file to write 00036 fprintf(fp,"phone Number,chem data, latitude,longitude\n"); // writes in a header for the table 00037 fclose(fp); // closes file 00038 00039 while(1) 00040 { if(serialModem.readable()) { 00041 00042 while(serialModem.readable()) { 00043 char c = serialModem.getc(); 00044 buffer[count++] = c; 00045 //pc.puts(&c); 00046 if(count == 64) break; 00047 } 00048 00049 if(buffer[0] != '\0'){ 00050 buffer[count] = '\0'; 00051 FILE *fp = fopen("/sd/mydir/chemicalData.csv", "a"); // opens file to append it 00052 fprintf(fp,"%s\n",buffer);//writes to file 00053 fclose(fp); // closes file 00054 00055 printf("%s \n",buffer); 00056 for(int i = 0; i < count+2; i++) { 00057 buffer[i] = NULL; 00058 } 00059 } 00060 count = 0; 00061 } 00062 } 00063 } 00064 00065 void Modem::wait_for_sms(){ 00066 printf("waiting for message\n"); 00067 while(1){ 00068 if(serialModem.readable()){ 00069 return; 00070 } 00071 } 00072 } 00073 void Modem::get_message(){ 00074 char tempStr[30]; 00075 int count= 0; 00076 int line_count = 0; 00077 for(int i= 0;i < strlen(IPAdd);i++){ 00078 if(line_count == 3){ 00079 tempStr[count++] = IPAdd[i]; 00080 } 00081 if(IPAdd[i] == '\n'){ 00082 line_count++; 00083 } 00084 } 00085 tempStr[count - 2] = '\0'; 00086 count++; 00087 strncpy(IPAdd, tempStr, count); 00088 printf("IP Addr > %s \n", IPAdd); 00089 printf("text size > %d\n",strlen(IPAdd)); 00090 } 00091 void Modem::storeResp(){ 00092 00093 int line_count = 0; 00094 int read = -1; 00095 char buffer[100]; 00096 int count = 0; 00097 timeCnt.start(); 00098 while(timeCnt.read() < 5) 00099 { 00100 while(serialModem.readable()) { 00101 char c = serialModem.getc(); 00102 buffer[count++] = c; 00103 } 00104 } 00105 timeCnt.stop(); 00106 timeCnt.reset(); 00107 buffer[count] = '\0'; 00108 00109 strncpy(IPAdd, buffer, count); 00110 printf("original>> %s",IPAdd); 00111 printf("size of text > %d",strlen(IPAdd)); 00112 count = 0; 00113 get_message(); 00114 } 00115 00116 char* Modem::get_server_IP(){ 00117 return IPAdd; 00118 } 00119 //end added by Noah Milam 00120 char Modem::readByte(void) 00121 { 00122 return serialModem.getc(); 00123 } 00124 00125 bool Modem::readable() 00126 { 00127 return serialModem.readable(); 00128 } 00129 00130 int Modem::readBuffer(char *buffer,int count, unsigned int timeOut) 00131 { 00132 int i = 0; 00133 timeCnt.start(); 00134 while(1) { 00135 while (serialModem.readable()) { 00136 char c = serialModem.getc(); 00137 buffer[i++] = c; 00138 if(i >= count)break; 00139 } 00140 if(i >= count)break; 00141 if(timeCnt.read() > timeOut) { 00142 timeCnt.stop(); 00143 timeCnt.reset(); 00144 break; 00145 } 00146 } 00147 return 0; 00148 } 00149 00150 void Modem::cleanBuffer(char *buffer, int count) 00151 { 00152 for(int i=0; i < count; i++) { 00153 buffer[i] = '\0'; 00154 } 00155 } 00156 00157 void Modem::sendCmd(const char* cmd) 00158 { 00159 serialModem.puts(cmd); 00160 } 00161 void Modem::sendCmdResp(const char* cmd) 00162 { 00163 serialModem.puts(cmd); 00164 } 00165 void Modem::getResp() 00166 { 00167 char buffer[1000]; 00168 int count = 0; 00169 timeCnt.start(); 00170 while(timeCnt.read() < 5) 00171 { while(serialModem.readable()) { 00172 char c = serialModem.getc(); 00173 buffer[count++] = c; 00174 } 00175 } 00176 timeCnt.stop(); 00177 timeCnt.reset(); 00178 buffer[count] = '\0'; 00179 printf("Response:--%s--\r\n",buffer); 00180 for(int i = 0; i < count+2; i++) { 00181 buffer[i] = NULL; 00182 } 00183 count = 0; 00184 } 00185 00186 00187 void Modem::sendATTest(void) 00188 { 00189 sendCmdAndWaitForResp("AT\r\n","OK",DEFAULT_TIMEOUT,CMD); 00190 } 00191 00192 bool Modem::respCmp(const char *resp, unsigned int len, unsigned int timeout) 00193 { 00194 int sum=0; 00195 timeCnt.start(); 00196 00197 while(1) { 00198 if(serialModem.readable()) { 00199 char c = serialModem.getc(); 00200 sum = (c==resp[sum]) ? sum+1 : 0; 00201 if(sum == len)break; 00202 } 00203 if(timeCnt.read() > timeout) { 00204 timeCnt.stop(); 00205 timeCnt.reset(); 00206 return false; 00207 } 00208 } 00209 timeCnt.stop(); 00210 timeCnt.reset(); 00211 00212 return true; 00213 } 00214 00215 int Modem::waitForResp(const char *resp, unsigned int timeout,DataType type) 00216 { 00217 int len = strlen(resp); 00218 int sum=0; 00219 timeCnt.start(); 00220 00221 while(1) { 00222 if(serialModem.readable()) { 00223 char c = serialModem.getc(); 00224 sum = (c==resp[sum]) ? sum+1 : 0; 00225 if(sum == len)break; 00226 } 00227 if(timeCnt.read() > timeout) { 00228 timeCnt.stop(); 00229 timeCnt.reset(); 00230 return -1; 00231 } 00232 } 00233 00234 timeCnt.stop(); 00235 timeCnt.reset(); 00236 00237 if(type == CMD) { 00238 while(serialModem.readable()) { 00239 char c = serialModem.getc(); 00240 } 00241 00242 } 00243 return 0; 00244 } 00245 00246 int Modem::sendCmdAndWaitForResp(const char* data, const char *resp, unsigned timeout,DataType type) 00247 { 00248 sendCmd(data); 00249 return waitForResp(resp,timeout,type); 00250 }
Generated on Wed Jul 13 2022 11:50:29 by
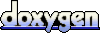