
works through pushing box to log data to google spreadsheet
Dependencies: MBed_Adafruit-GPS-Library SDFileSystem mbed
Fork of GPR_Interface by
main.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #pragma once 00019 #include "mbed.h" 00020 #include "math.h" 00021 #include "MBed_Adafruit_GPS.h" 00022 #include "SDFileSystem.h" 00023 #include "QAM.h" 00024 #include "GPRSInterface.h" 00025 #include "param.h" 00026 #include "stdlib.h" 00027 00028 #define NUM_SIZE 250 00029 /** On many platforms USBTX/USBRX overlap with serial on D1/D0 pins and enabling the below will interrupt the communication. 00030 * You can use an LCD display to print the values or store them on an SD card etc. 00031 */ 00032 DigitalOut led_red(LED_RED); 00033 Serial pc(USBTX, USBRX); 00034 //Serial gsm(D1,D0); 00035 00036 /************************************************** 00037 ** SD FILE SYSTEM ** 00038 **************************************************/ 00039 //SDFileSystem sdModem(PTE3, PTE1, PTE2, PTE4, "sd"); //MOSI, MISO, SCLK, SSEL. Tested on K64F, correct pins. 00040 FILE *fpData; 00041 FILE *fpLog; 00042 00043 /************************************************** 00044 ** GPS ** 00045 **************************************************/ 00046 Serial * gps_Serial; 00047 00048 /************************************************** 00049 ** GPRS ** 00050 **************************************************/ 00051 /** 00052 * D1 - TX pin (RX on the WiFi side) 00053 * D0 - RX pin (TX on the WiFi side) 00054 * 19200 - Baud rate 00055 * "apn" - APN name 00056 * "username" - APN username 00057 * "password" - APN passowrd 00058 */ 00059 GPRSInterface eth(D1,D0, 19200, "ndo","",""); 00060 /************************************************** 00061 ** SENSOR INPUTS ** 00062 **************************************************/ 00063 AnalogIn An(A0); 00064 AnalogIn AnRef(A2); 00065 Ticker sample_tick; 00066 bool takeSample = false; 00067 void tick(){takeSample = true;} 00068 00069 /************************************************** 00070 ** SIN OUTPUT ** 00071 **************************************************/ 00072 AnalogOut dac0(DAC0_OUT); 00073 int sinRes = (int)1/(CARRIER_FREQ*TIME_CONST); 00074 float sinWave[SIN_LENGTH] = {}; 00075 int sinIndex = 0; 00076 00077 00078 /************************************************** 00079 ** QAM ** 00080 **************************************************/ 00081 float sQ[SAMPLE_LENGTH] = {}; 00082 float sI[SAMPLE_LENGTH] = {}; 00083 float sRefQ[SAMPLE_LENGTH] = {}; 00084 float sRefI[SAMPLE_LENGTH] = {}; 00085 00086 float Iarray[SAMPLE_LENGTH] = {}; 00087 float Qarray[SAMPLE_LENGTH] = {}; 00088 int sampleIndex = 0; 00089 float I = 0; 00090 float Q = 0; 00091 float lon = 0; 00092 float lonRef = 0; 00093 00094 /*Global Variables */ 00095 char c; 00096 int s; 00097 bool run = 0; 00098 bool fil = 0; 00099 float filtered = 0; 00100 float filteredRef = 0; 00101 char gsm_header[250]; //String storing SMS message that will be sent (add 250 length to give leeway) 00102 char gsm_msg[250]; 00103 char num[NUM_SIZE]; //Temporary string storage to help with concatenation of strings 00104 00105 void buildIQ(){ 00106 for(int i = 0; i < SAMPLE_LENGTH; i++){ 00107 Iarray[i] = cos(2*PI*CARRIER_FREQ*i*TIME_CONST); 00108 Qarray[i] = -sin(2*PI*CARRIER_FREQ*i*TIME_CONST); 00109 } 00110 } 00111 00112 void create_sinWave(){ 00113 int i = 0; 00114 for(i = 0; i < SIN_LENGTH; i++){ 00115 sinWave[i] = 0.25 * sin(2.0*PI*i/sinRes) + 0.75; 00116 } 00117 } 00118 void init(Adafruit_GPS myGPS){ 00119 00120 //fprintf(fpLog, "%s\r\n", ":)\r\n"); 00121 00122 //fprintf(fpLog, "%s\r\n", "Initializing the GPS\r\n"); 00123 00124 myGPS.begin(9600); 00125 myGPS.sendCommand(PMTK_SET_NMEA_OUTPUT_RMCGGA); 00126 myGPS.sendCommand(PMTK_SET_NMEA_UPDATE_1HZ); 00127 myGPS.sendCommand(PGCMD_ANTENNA); 00128 //fprintf(fpLog, "%s\r\n", "GPS Initialized\r\n"); 00129 00130 // Initialize filtering structures. 00131 buildIQ(); 00132 create_sinWave(); 00133 00134 00135 00136 //Intialize the dhcp (or die trying) 00137 s = eth.init(); 00138 if (s != NULL) { 00139 printf(">>> Could not initialise. Halting!\n"); 00140 exit(0); 00141 } 00142 00143 //Check the SIM card 00144 s= eth.preInit(); 00145 if(s == true) { 00146 //fprintf(fpLog, "%s\r\n", "SIM Card is good!\r\n"); 00147 } else { 00148 //fprintf(fpLog, "%s\r\n", "check your baud rate\r\n"); 00149 } 00150 } 00151 void gatherData(Adafruit_GPS myGPS){ 00152 //sample 00153 if(takeSample){ 00154 00155 dac0 = sinWave[sinIndex]; 00156 00157 lon = An.read(); 00158 lonRef = AnRef.read(); 00159 00160 I = Iarray[sampleIndex]; 00161 Q = Qarray[sampleIndex]; 00162 sI[sampleIndex] = lon*I; 00163 sQ[sampleIndex] = lon*Q; 00164 sRefI[sampleIndex] = lonRef*I; 00165 sRefQ[sampleIndex] = lonRef*Q; 00166 00167 takeSample = false; 00168 sinIndex++; 00169 if((sinIndex+1) > SIN_LENGTH){ 00170 sinIndex = 0; 00171 } 00172 00173 sampleIndex++; 00174 if(sampleIndex+1 > SAMPLE_LENGTH){ 00175 fil = 1; 00176 } 00177 } 00178 //Filter 00179 if(fil==1){ 00180 00181 fil = 0; 00182 run = 1; 00183 sampleIndex = 0; 00184 filtered = 15*QAM(sI, sQ); 00185 filteredRef = QAM(sRefI, sRefQ); 00186 } 00187 00188 c = myGPS.read(); 00189 if ( myGPS.newNMEAreceived() ) { 00190 if ( !myGPS.parse(myGPS.lastNMEA()) ) { 00191 //fprintf(fpLog, "%s\r\n", "Couldn't parse the GPS Data\r\n"); 00192 } 00193 } 00194 if(run){ 00195 run = 0; 00196 printf("Logged Data\r\n"); 00197 /*fprintf(fpLog, "15CM: %f, ", filtered); 00198 fprintf(fpLog, "15CM Reference: %f\r\n", filteredRef); 00199 fprintf(fpLog, "15CM / 15CM Refernce: %f\r\n", (filteredRef ? (filtered/filteredRef) : 0)); 00200 fprintf(fpLog, "GPS TIME: %d:%d:%d \r\n", myGPS.hour-6, myGPS.minute, myGPS.seconds);*/ 00201 00202 if (myGPS.fix){ 00203 printf("Lat: %5.2f%c, Long: %5.2f%c\r\n", myGPS.latitude, myGPS.lat, myGPS.longitude, myGPS.lon); 00204 //fprintf(fpLog, "Lat: %5.2f%c, Long: %5.2f%c\r\n", myGPS.latitude, myGPS.lat, myGPS.longitude, myGPS.lon); 00205 } 00206 else{ 00207 printf("No GPS FIX\r\n"); 00208 /*fprintf(fpLog, "%s\r\n", "No GPS fix\r\n"); 00209 fprintf(fpLog, "%s\r\n", "--------------------------------\r\n");*/ 00210 } 00211 00212 /*fpData = fopen("/sd/data.txt", "a"); 00213 if (fpData != NULL){ 00214 00215 fprintf(fpData, "%f, ", filtered); 00216 fprintf(fpData, "%f, ", filteredRef); 00217 fprintf(fpData, "%d:%d:%d\r\n", myGPS.hour-6, myGPS.minute, myGPS.seconds); 00218 if (myGPS.fix) fprintf(fpData, "%5.2f%c, %5.2f%c\r\n", myGPS.latitude, myGPS.lat, myGPS.longitude, myGPS.lon); 00219 else fprintf(fpData, "No_GPS_fix\r\n"); 00220 00221 fclose(fpData); 00222 }*/ 00223 00224 } 00225 00226 } 00227 int main() 00228 { 00229 //set the baud rates 00230 //gsm.baud(19200); 00231 00232 gps_Serial = new Serial(PTC4,PTC3); ////Serial gsm(D1,D0); 00233 Adafruit_GPS myGPS(gps_Serial); 00234 00235 //Initialize SD Card with log and data 00236 /*fpLog = fopen("/sd/log.txt", "a"); 00237 if (fpLog != NULL){ 00238 fprintf(fpLog, "%s\r\n", "--------------- DCS LOG ------------------"); 00239 } 00240 fpData = fopen("/sd/data.txt", "a"); 00241 if (fpData != NULL){ 00242 fprintf(fpData, "%s", "--------------- DCS DATA ------------------"); 00243 } 00244 00245 //Successful Connection Message 00246 fprintf(fpLog, "%s\r\n", "---DPG Connection Successful!---\r\n");*/ 00247 00248 //initialize gps, gprs, sd card etc... 00249 init(myGPS); 00250 00251 //fprintf(fpLog, "%s\r\n", ">>> Get IP address...\r\n"); 00252 while (1) { 00253 s = eth.connect(); // Connect to network 00254 if (s == false || s < 0) { 00255 printf(">>> Could not connect to network. Retrying!\r\n"); 00256 wait(3); 00257 } else { 00258 break; 00259 } 00260 } 00261 //fprintf(fpLog, ">>> Got IP address: %s\r\n", eth.getIPAddress()); 00262 00263 //fprintf(fpLog, "%s\r\n", ">>> Create a TCP Socket Connection\r\n"); 00264 TCPSocketConnection sock; 00265 sock.set_blocking(true,5000); 00266 00267 sock.connect("api.pushingbox.com",80); 00268 00269 //fprintf(fpLog, "%s\r\n", "Gathering Data...\r\n"); 00270 while(1){ 00271 00272 //take a sample 00273 tick(); 00274 gatherData(myGPS); 00275 //if(!myGPS.fix){ 00276 printf("Done Gathering Data\r\n"); 00277 00278 //fprintf(fpLog, "%s\r\n", "Begin Sending data...\r\n"); 00279 //Concatenate data 00280 gsm_msg[0] = NULL; 00281 gsm_header[0] = NULL; 00282 int contentLength = 0; 00283 snprintf(num, NUM_SIZE, "&phone=%s", "3852368101"); 00284 contentLength += strlen(num); 00285 strcat(gsm_msg, num); 00286 snprintf(num, NUM_SIZE, "&data=%f", filtered); 00287 contentLength += strlen(num); 00288 strcat(gsm_msg, num); 00289 snprintf(num, NUM_SIZE, "&dataRef=%f", filteredRef); 00290 contentLength += strlen(num); 00291 strcat(gsm_msg, num); 00292 snprintf(num, NUM_SIZE, "&dataRatio=%f", (filteredRef ? (filtered/filteredRef) : 0)); 00293 contentLength += strlen(num); 00294 strcat(gsm_msg, num); 00295 snprintf(num, NUM_SIZE, "&time=%02d:%02d:%02d", myGPS.hour, myGPS.minute, myGPS.seconds); //If there is no data from GPS, the time will just be "00:00:00" (that is okay) 00296 contentLength += strlen(num); 00297 strcat(gsm_msg, num); 00298 if (myGPS.lat != NULL) //If there is a gps fix (i.e. the gps has data on our location), ns will be set 00299 { 00300 snprintf(num, NUM_SIZE, "&latitude=%.4f&longitude=%.4f", (myGPS.lat == 'N') ? myGPS.latitude : -myGPS.latitude, (myGPS.lon == 'E') ? myGPS.longitude : -myGPS.longitude); //Use + or - rather than N/S, E/W 00301 contentLength += strlen(num); 00302 strcat(gsm_msg, num); 00303 } 00304 else { 00305 snprintf(num, NUM_SIZE,"&latitude=0&longitude=0"); 00306 strcat(gsm_msg, num); //Otherwise just send 0's for latitude and longitude 00307 contentLength += strlen(num); 00308 } 00309 00310 00311 //header information 00312 snprintf(num, NUM_SIZE, "%s", "POST /pushingbox?devid=v941C443DE0C7B14"); 00313 strcat(gsm_header, num); 00314 strcat(gsm_header, gsm_msg); 00315 snprintf(num, NUM_SIZE, "%s\r\n"," HTTP/1.1"); 00316 strcat(gsm_header, num); 00317 snprintf(num, NUM_SIZE, "%s\r\n","Host: api.pushingbox.com"); 00318 strcat(gsm_header, num); 00319 snprintf(num, NUM_SIZE, "%s\r\n","Connection: Keep-Alive"); 00320 strcat(gsm_header, num); 00321 snprintf(num, NUM_SIZE, "%s\r\n","User-Agent: FRDM-KD64"); 00322 strcat(gsm_header, num); 00323 //must have two blank lines after so the server knows that this is the end of headers 00324 snprintf(num, NUM_SIZE, "Content-Length: %d\r\n\r\n\r\n", contentLength); 00325 strcat(gsm_header, num); 00326 00327 //fprintf(fpLog, "%s\r\n", ">>> Sending data to the server: %s\r\n",gsm_header); 00328 int result = sock.send_all(gsm_header, contentLength); 00329 //sock.set_blocking(false); 00330 if (result > 0) { 00331 //fprintf(fpLog, "%s\r\n", ">>> Successfully Sent Data to Server\r\n"); 00332 printf("Successfully send data\r\n"); 00333 } else { 00334 //fprintf(fpLog, "%s\r\n", ">>> Failed to Send Data, Error Code: %d \r\n",result); 00335 printf("Data Not Sent!! Content-Length:%d\r\n", contentLength); 00336 } 00337 00338 //check that we are still connected (if not reconnect) 00339 //sock.close(); 00340 //printf("end of program\n"); 00341 // Disconnect from network 00342 eth.disconnect(); 00343 //return 0; 00344 /*} 00345 else{ 00346 fprintf(fpLog, "%s\r\n", ">>>No GPS Fix\r\n"); 00347 }*/ 00348 //wait(4); 00349 } 00350 00351 } 00352
Generated on Wed Jul 13 2022 11:50:29 by
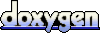