
works through pushing box to log data to google spreadsheet
Dependencies: MBed_Adafruit-GPS-Library SDFileSystem mbed
Fork of GPR_Interface by
GPRS.h
00001 /* 00002 GPRS.h 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-2-24 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #ifndef __GPRS_H__ 00024 #define __GPRS_H__ 00025 00026 #include "mbed.h" 00027 #include "modem.h" 00028 00029 #define DEFAULT_WAIT_RESP_TIMEOUT 500 00030 #define MAX_SOCK_NUM 7 //(0~6) 00031 00032 enum Protocol { 00033 CLOSED = 0, 00034 TCP = 1, 00035 UDP = 2, 00036 }; 00037 00038 /** The GPRS class 00039 * 00040 */ 00041 class GPRS: public Modem 00042 { 00043 00044 public: 00045 /** Constructor 00046 * @param tx mbed pin to use for tx line of Serial interface 00047 * @param rx mbed pin to use for rx line of Serial interface 00048 * @param baudRate serial communicate baud rate 00049 * @param apn name of the gateway for GPRS to connect to the network 00050 * @param userName apn's username, usually is NULL 00051 * @param passWord apn's password, usually is NULL 00052 */ 00053 GPRS(PinName tx, PinName rx, int baudRate, const char* apn, const char* userName = NULL, const char *passWord = NULL); 00054 00055 /** Get instance of GPRS class 00056 */ 00057 static GPRS* getInstance() { 00058 return inst; 00059 }; 00060 00061 /** Connect the GPRS module to the network. 00062 * @return true if connected, false otherwise 00063 */ 00064 bool join(void); 00065 // added by Noah Milam 00066 void start_server(); 00067 void send_SMS(char*); 00068 char* read_SMS(); 00069 void listen_server(); 00070 00071 // end of what Noah Milam added 00072 /** Disconnect the GPRS module from the network 00073 * @returns true if successful 00074 */ 00075 bool disconnect(void); 00076 00077 /** Close a tcp connection 00078 * @returns true if successful 00079 */ 00080 bool close(int socket); 00081 00082 /** Open a tcp/udp connection with the specified host on the specified port 00083 * @param socket an endpoint of an inter-process communication flow of GPRS module,for SIM900 module, it is in [0,6] 00084 * @param ptl protocol for socket, TCP/UDP can be choosen 00085 * @param host host (can be either an ip address or a name. If a name is provided, a dns request will be established) 00086 * @param port port 00087 * @param timeout wait seconds till connected 00088 * @returns true if successful 00089 */ 00090 bool connect(int socket, Protocol ptl, const char * host, int port, int timeout = DEFAULT_TIMEOUT); 00091 00092 /** Set the protocol (UDP or TCP) 00093 * @param socket socket 00094 * @param p protocol 00095 * @returns true if successful 00096 */ 00097 bool setProtocol(int socket, Protocol p); 00098 00099 /** Reset the GPRS module 00100 */ 00101 void reset(); 00102 00103 /** Check if GPRS module is readable or not 00104 * @returns true if readable 00105 */ 00106 bool readable(void); 00107 00108 /** Wait a few time to check if GPRS module is readable or not 00109 * @param socket socket 00110 * @param wait_time time of waiting 00111 */ 00112 int wait_readable(int socket, int wait_time); 00113 00114 /** Wait a few time to check if GPRS module is writeable or not 00115 * @param socket socket 00116 * @param wait_time time of waiting 00117 */ 00118 int wait_writeable(int socket, int req_size); 00119 00120 /** Check if a tcp link is active 00121 * @returns true if successful 00122 */ 00123 bool is_connected(int socket); 00124 00125 /** Send data to socket 00126 * @param socket socket 00127 * @param str string to be sent 00128 * @param len string length 00129 * @returns return bytes that actually been send 00130 */ 00131 int send(int socket, const char * str, int len); 00132 00133 /** Read data from socket 00134 * @param socket socket 00135 * @param buf buffer that will store the data read from socket 00136 * @param len string length need to read from socket 00137 * @returns bytes that actually read 00138 */ 00139 int recv(int socket, char* buf, int len); 00140 00141 /** Convert the host to ip 00142 * @param host host ip string, ex. 10.11.12.13 00143 * @param ip long int ip address, ex. 0x11223344 00144 * @returns true if successful 00145 */ 00146 bool gethostbyname(const char* host, uint32_t* ip); 00147 00148 /** Parse the phone number from the AT command return value and store it 00149 * @param atCNumValue the return value from the AT+CNUM AT Command 00150 * @return true if successful 00151 */ 00152 bool storePhoneNumber(char* atCNumValue); 00153 00154 int new_socket(); 00155 uint16_t new_port(); 00156 uint32_t _ip; 00157 bool preInit(); 00158 protected: 00159 00160 00161 bool checkSIMStatus(void); 00162 uint32_t str_to_ip(const char* str); 00163 static GPRS* inst; 00164 int socketID; 00165 const char* _apn; 00166 const char* _userName; 00167 const char* _passWord; 00168 char* _phoneNumber; 00169 }; 00170 00171 #endif
Generated on Wed Jul 13 2022 11:50:29 by
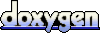