
works through pushing box to log data to google spreadsheet
Dependencies: MBed_Adafruit-GPS-Library SDFileSystem mbed
Fork of GPR_Interface by
GPRS.cpp
00001 /* 00002 GPRS.cpp 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-2-24 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #include "mbed.h" 00024 #include "GPRS.h" 00025 00026 GPRS* GPRS::inst; 00027 00028 GPRS::GPRS(PinName tx, PinName rx, int baudRate, const char* apn, const char* userName, const char* passWord) : Modem(tx,rx,baudRate) 00029 { 00030 inst = this; 00031 _apn = apn; 00032 _userName = userName; 00033 _passWord = passWord; 00034 socketID = -1; 00035 _phoneNumber = "12345678910"; 00036 } 00037 00038 bool GPRS::preInit() 00039 { 00040 for(int i = 0; i < 2; i++) { 00041 sendCmd("AT\r\n"); 00042 wait(1); 00043 } 00044 return checkSIMStatus(); 00045 } 00046 // added by Noah Milam 00047 void GPRS::start_server() 00048 { 00049 sendCmdResp("AT+CGATT?\r\n"); 00050 00051 sendCmdResp("AT+CIPSERVER=1,8080\r\n"); 00052 listen_server(); 00053 } 00054 void GPRS::listen_server() 00055 { 00056 gprs_response(); 00057 } 00058 00059 void GPRS::send_SMS(char* IPAdress) 00060 { 00061 printf("sending at command\n"); 00062 sendCmdAndWaitForResp("AT+CMGF=1\r\n","OK",DEFAULT_TIMEOUT,CMD); 00063 wait(2); 00064 //printf("\032\n"); 00065 sendCmdAndWaitForResp("AT+CMGS=\"+17066311506\"\r\n",">",DEFAULT_TIMEOUT,CMD); 00066 printf("sent at cmgf\n"); 00067 wait(2); 00068 //printf("032"); 00069 sendCmd(IPAdress); // sends the address 00070 sendCmd("\x1a"); // this is like pressing control - z to end the send command 00071 wait(10); // giving the send enough time to do its thing 00072 printf("should have been received"); 00073 } 00074 00075 char* GPRS::read_SMS() 00076 { 00077 wait(1); 00078 sendCmd("AT+CMGF=1\r\n"); // sms mode 00079 wait(2); 00080 sendCmd("AT+CMGD=1\r\n"); // delete the frist message so incoming message is spot 1 00081 wait(1); 00082 wait_for_sms(); 00083 wait(10); 00084 sendCmd("AT+CMGR=1\r\n"); 00085 storeResp(); 00086 return get_server_IP(); 00087 } 00088 00089 // end of what Noah Milam added 00090 bool GPRS::checkSIMStatus(void) 00091 { 00092 printf("Checking SIM Status...\r\n"); 00093 char gprsBuffer[32]; 00094 int count = 0; 00095 00096 while(count < 3) { 00097 cleanBuffer(gprsBuffer,32); 00098 sendCmd("AT+CPIN?\r\n"); 00099 readBuffer(gprsBuffer,32,DEFAULT_TIMEOUT); 00100 if((NULL != strstr(gprsBuffer,"+CPIN: READY"))) { 00101 break; 00102 } 00103 printf("SIM Not Ready..Try Again\r\n"); 00104 count++; 00105 wait(1); 00106 } 00107 if(count == 3) { 00108 return false; 00109 } 00110 printf("SIM Status GOOD!\r\n"); 00111 return true; 00112 } 00113 00114 bool GPRS::join() 00115 { 00116 char cmd[64]; 00117 char ipAddr[32]; 00118 char resp[96]; 00119 //get the phone number 00120 printf(">>>>AT+CNUM\r\n"); 00121 sendCmd("AT+CNUM\r\n"); 00122 cleanBuffer(resp,96); 00123 readBuffer(resp,96,DEFAULT_TIMEOUT); 00124 if(NULL != strstr(resp,"+CNUM:")) { 00125 //if(storePhoneNumber(resp)){ 00126 printf("Successfull stored the phone number!\r\n"); 00127 //} 00128 } 00129 else{ 00130 return false; 00131 } 00132 00133 printf(">>>>AT+CREG\r\n"); 00134 sendCmd("AT+CREG?\r\n"); 00135 cleanBuffer(resp,96); 00136 readBuffer(resp,96,DEFAULT_TIMEOUT); 00137 if(NULL != strstr(resp,"+CREG:")) { 00138 } 00139 else{ 00140 return false; 00141 } 00142 //close any existing connections 00143 printf(">>>>AT+CIPSHUT\r\n"); 00144 sendCmd("AT+CIPSHUT\r\n"); 00145 cleanBuffer(resp,96); 00146 readBuffer(resp,96,DEFAULT_TIMEOUT); 00147 if(NULL != strstr(resp,"OK")) { 00148 } 00149 else{ 00150 return false; 00151 } 00152 //Select multiple connection 00153 printf(">>>>AT+CIPMUX=0\r\n"); 00154 sendCmd("AT+CIPMUX=0\r\n"); 00155 cleanBuffer(resp,96); 00156 readBuffer(resp,96,DEFAULT_TIMEOUT); 00157 if(NULL != strstr(resp,"CIPMUX")) { 00158 } 00159 else{ 00160 return false; 00161 } 00162 //get signal strength 00163 printf(">>>>AT+CSQ\r\n"); 00164 sendCmd("AT+CSQ\r\n"); 00165 cleanBuffer(resp,96); 00166 readBuffer(resp,96,DEFAULT_TIMEOUT); 00167 if(NULL != strstr(resp,"+CSQ:")) { 00168 } 00169 else{ 00170 return false; 00171 } 00172 00173 //attach the device 00174 printf(">>>>AT+CGATT=1\r\n"); 00175 sendCmd("AT+CGATT=1\r\n"); 00176 cleanBuffer(resp,96); 00177 readBuffer(resp,96,DEFAULT_TIMEOUT); 00178 if(NULL != strstr(resp,"OK")) { 00179 } 00180 else{ 00181 return false; 00182 } 00183 00184 //set APN 00185 snprintf(cmd,sizeof(cmd),"AT+CSTT=\"%s\",\"%s\",\"%s\"\r\n",_apn,_userName,_passWord); 00186 printf(">>>>%s",cmd); 00187 //sendCmdAndWaitForRes p(cmd, "OK", DEFAULT_TIMEOUT,CMD); 00188 sendCmd(cmd); 00189 cleanBuffer(resp,96); 00190 readBuffer(resp,96,DEFAULT_TIMEOUT); 00191 if(NULL != strstr(resp,"OK")) { 00192 } 00193 else{ 00194 return false; 00195 } 00196 //Brings up wireless connection 00197 printf(">>>>AT+CIICR\r\n"); 00198 sendCmd("AT+CIICR\r\n"); 00199 cleanBuffer(resp,96); 00200 readBuffer(resp,96,DEFAULT_TIMEOUT); 00201 if(NULL != strstr(resp,"OK")) { 00202 } 00203 else{ 00204 return false; 00205 } 00206 00207 //Get local IP address 00208 printf(">>>>AT+CIFSR\r\n"); 00209 sendCmd("AT+CIFSR\r\n"); 00210 readBuffer(ipAddr,32,2); 00211 printf(">>>>AT+CIFSR returns: %s\r\n", ipAddr); 00212 if(NULL != strstr(ipAddr,"AT+CIFSR")) { 00213 _ip = str_to_ip(ipAddr+12); 00214 if(_ip != 0) { 00215 //set to quick sending mode 00216 printf(">>>>AT+CIPQSEND=1\r\n"); 00217 sendCmd("AT+CIPQSEND=1\r\n"); 00218 cleanBuffer(resp,96); 00219 readBuffer(resp,96,DEFAULT_TIMEOUT); 00220 if(NULL != strstr(resp,"OK")) { 00221 return true; 00222 } 00223 } 00224 } 00225 00226 return false; 00227 } 00228 00229 bool GPRS::setProtocol(int socket, Protocol p) 00230 { 00231 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00232 return false; 00233 } 00234 //ToDo: setProtocol 00235 return true; 00236 } 00237 00238 bool GPRS::connect(int socket, Protocol ptl,const char * host, int port, int timeout) 00239 { 00240 char cmd[64]; 00241 char resp[96]; 00242 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00243 return false; 00244 } 00245 if(ptl == TCP) { 00246 sprintf(cmd, "AT+CIPSTART=\"TCP\",\"%s\",\"%d\"\r\n", host, port); 00247 } else if(ptl == UDP) { 00248 sprintf(cmd, "AT+CIPSTART=%d,\"UDP\",\"%s\",%d\r\n",socket, host, port); 00249 } else { 00250 return false; 00251 } 00252 00253 sendCmd(cmd); 00254 readBuffer(resp,96,DEFAULT_TIMEOUT); 00255 if(NULL != strstr(resp,"CONNECT")) { //ALREADY CONNECT or CONNECT OK 00256 return true; 00257 } 00258 return false;//ERROR 00259 } 00260 00261 bool GPRS::gethostbyname(const char* host, uint32_t* ip) 00262 { 00263 uint32_t addr = str_to_ip(host); 00264 char buf[17]; 00265 snprintf(buf, sizeof(buf), "%d.%d.%d.%d", (addr>>24)&0xff, (addr>>16)&0xff, (addr>>8)&0xff, addr&0xff); 00266 if (strcmp(buf, host) == 0) { 00267 *ip = addr; 00268 return true; 00269 } 00270 return false; 00271 } 00272 00273 bool GPRS::disconnect() 00274 { 00275 sendCmd("AT+CIPSHUT\r\n"); 00276 return true; 00277 } 00278 00279 bool GPRS::is_connected(int socket) 00280 { 00281 00282 char cmd[16]; 00283 char resp[96]; 00284 while(1){ 00285 snprintf(cmd,16,"AT+CIPSTATUS\r\n"); 00286 sendCmd(cmd); 00287 readBuffer(resp,sizeof(resp),DEFAULT_TIMEOUT); 00288 if(NULL != strstr(resp,"CONNECTED")) { 00289 return true; 00290 } else { 00291 return false; 00292 } 00293 } 00294 } 00295 00296 void GPRS::reset() 00297 { 00298 } 00299 00300 bool GPRS::close(int socket) 00301 { 00302 char cmd[16]; 00303 char resp[16]; 00304 00305 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00306 return false; 00307 } 00308 // if not connected, return 00309 if (is_connected(socket) == false) { 00310 return true; 00311 } 00312 snprintf(cmd, sizeof(cmd),"AT+CIPCLOSE=%d\r\n",socket); 00313 snprintf(resp,sizeof(resp),"%d, CLOSE OK",socket); 00314 if(0 != sendCmdAndWaitForResp(cmd, resp, DEFAULT_TIMEOUT,CMD)) { 00315 return false; 00316 } 00317 return true; 00318 } 00319 00320 bool GPRS::readable(void) 00321 { 00322 return readable(); 00323 } 00324 00325 int GPRS::wait_readable(int socket, int wait_time) 00326 { 00327 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00328 return -1; 00329 } 00330 char resp[16]; 00331 snprintf(resp,sizeof(resp),"\r\n\r\n+RECEIVE,%d",socket);//"+RECEIVE:<socketID>,<length>" 00332 int len = strlen(resp); 00333 00334 if(false == respCmp(resp,len,wait_time)) { 00335 return -1; 00336 } 00337 char c = readByte();//',' 00338 char dataLen[4]; 00339 int i = 0; 00340 c = readByte(); 00341 while((c >= '0') && (c <= '9')) { 00342 dataLen[i++] = c; 00343 c = readByte(); 00344 } 00345 c = readByte();//'\n' 00346 len = atoi(dataLen); 00347 return len; 00348 } 00349 00350 int GPRS::wait_writeable(int socket, int req_size) 00351 { 00352 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00353 return -1; 00354 } 00355 return req_size>256?256:req_size+1; 00356 } 00357 00358 int GPRS::send(int socket, const char * str, int len) 00359 { 00360 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00361 return -1; 00362 } 00363 char cmd[32]; 00364 wait(1); 00365 if(len > 0){ 00366 snprintf(cmd,sizeof(cmd),"AT+CIPSEND\r\n"); 00367 if(0 != sendCmdAndWaitForResp(cmd,">",1,CMD)) { 00368 00369 return false; 00370 } 00371 sendCmd(str); 00372 printf("Sending: %s\r\n", str); 00373 serialModem.putc((char)0x1a); 00374 00375 } 00376 return len; 00377 } 00378 00379 int GPRS::recv(int socket, char* buf, int len) 00380 { 00381 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00382 return -1; 00383 } 00384 cleanBuffer(buf,len); 00385 readBuffer(buf,len,DEFAULT_TIMEOUT/2); 00386 return len; 00387 //return strlen(buf); 00388 } 00389 00390 int GPRS::new_socket() 00391 { 00392 socketID = 0; //we only support one socket. 00393 return socketID; 00394 } 00395 00396 uint16_t GPRS::new_port() 00397 { 00398 uint16_t port = rand(); 00399 port |= 49152; 00400 return port; 00401 } 00402 00403 uint32_t GPRS::str_to_ip(const char* str) 00404 { 00405 uint32_t ip = 0; 00406 char* p = (char*)str; 00407 for(int i = 0; i < 4; i++) { 00408 ip |= atoi(p); 00409 p = strchr(p, '.'); 00410 if (p == NULL) { 00411 break; 00412 } 00413 ip <<= 8; 00414 p++; 00415 } 00416 return ip; 00417 } 00418 bool GPRS::storePhoneNumber(char* atCNumValue){ 00419 char* leftDelimiter = "+"; 00420 char* rightDelimiter = "\""; 00421 // find the left delimiter and use it as the beginning of the substring 00422 const char* beginning = strstr(atCNumValue, leftDelimiter); 00423 if(beginning == NULL) 00424 return 1; // left delimiter not found 00425 00426 // find the right delimiter 00427 const char* end = strstr(atCNumValue, rightDelimiter); 00428 if(end == NULL) 00429 return 2; // right delimiter not found 00430 00431 // offset the beginning by the length of the left delimiter, so beginning points _after_ the left delimiter 00432 beginning += strlen(leftDelimiter); 00433 00434 // get the length of the substring 00435 ptrdiff_t segmentLength = end - beginning; 00436 00437 // allocate memory and copy the substring there 00438 //*_phoneNumber = (char*)malloc(segmentLength + 1); 00439 strncpy(_phoneNumber, beginning, segmentLength); 00440 (_phoneNumber)[segmentLength] = 0; 00441 00442 printf("Phone Number: %s\r\n", _phoneNumber); 00443 return 0; // success! 00444 }
Generated on Wed Jul 13 2022 11:50:29 by
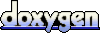