Library for using HC-SR04 with some API Documentation added
Fork of HC_SR04_Ultrasonic_Library by
ultrasonic.h
00001 #ifndef MBED_ULTRASONIC_H 00002 #define MBED_ULTRASONIC_H 00003 00004 #include "mbed.h" 00005 00006 /** ultrasonic class. 00007 * Used for HC-SR04 ultrasonic range sensor 00008 * 00009 * Example: 00010 * @code 00011 * #include "mbed.h" 00012 * #include "ultrasonic.h" 00013 * 00014 * ultrasonic usensor(PA_6, PA_7, 0.1, 0.25, &dist); 00015 * 00016 * int main() { 00017 * //start mesuring the distance 00018 * usensor.startUpdates(); 00019 * while(1){ 00020 * usensor.checkDistance(); 00021 * } 00022 * } 00023 * @endcode 00024 */ 00025 class ultrasonic 00026 { 00027 public: 00028 /**iniates the class with the specified trigger pin, echo pin, update speed and timeout 00029 * @param trigPin The "trigger" pin for the ultrasonic sensor. This is DigitalOut. 00030 * @param echoPin The "echo" pin for the ultrasonic sensor. This is InterruptIn. 00031 * @param updateSpeed How often the ultrasonic sensor pings and listens for a response. 00032 * @param timeout How long to wait before giving up on listening for a ping. 00033 **/ 00034 ultrasonic(PinName trigPin, PinName echoPin, float updateSpeed, float timeout); 00035 /**iniates the class with the specified trigger pin, echo pin, update speed, timeout and method to call when the distance changes 00036 * @param trigPin The "trigger" pin for the ultrasonic sensor. This is DigitalOut. 00037 * @param echoPin The "echo" pin for the ultrasonic sensor. This is InterruptIn. 00038 * @param updateSpeed How often the ultrasonic sensor pings and listens for a response. 00039 * @param timeout How long to wait before giving up on listening for a ping. 00040 * @param onUpdate(int) The function to be called when the distance is detected to have changed. 00041 **/ 00042 ultrasonic(PinName trigPin, PinName echoPin, float updateSpeed, float timeout, void onUpdate(int)); 00043 /** returns the last measured distance**/ 00044 int getCurrentDistance(void); 00045 /** auses measuring the distance**/ 00046 void pauseUpdates(void); 00047 /** tarts mesuring the distance**/ 00048 void startUpdates(void); 00049 /**attachs the method to be called when the distances changes 00050 * @param method(int) The function to be called when the distance is detected to have changed. 00051 */ 00052 void attachOnUpdate(void method(int)); 00053 /** 00054 * Method: 00055 * changes the speed at which updates are made 00056 * @param updateSpeed how often to update the ultrasonic sensor 00057 **/ 00058 void changeUpdateSpeed(float updateSpeed); 00059 /**gets whether the distance has been changed since the last call of isUpdated() or checkDistance()**/ 00060 int isUpdated(void); 00061 /**gets the speed at which updates are made**/ 00062 float getUpdateSpeed(void); 00063 /**call this as often as possible in your code, eg. at the end of a while(1) loop, 00064 and it will check whether the method you have attached needs to be called**/ 00065 void checkDistance(void); 00066 private: 00067 DigitalOut _trig; 00068 InterruptIn _echo; 00069 Timer _t; 00070 Timeout _tout; 00071 int _distance; 00072 float _updateSpeed; 00073 int start; 00074 int end; 00075 volatile int done; 00076 void (*_onUpdateMethod)(int); 00077 void _startT(void); 00078 void _updateDist(void); 00079 void _startTrig(void); 00080 float _timeout; 00081 int d; 00082 }; 00083 #endif
Generated on Wed Jul 13 2022 06:28:11 by
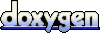