MARMEX-VB : "Mary Camera module" library
Dependents: MARMEX_VB_test MARMEX_VB_Hello
MARMEX_VB.h
00001 /** MARMEX_VB Camera control library 00002 * 00003 * @class MARMEX_VB 00004 * @version 0.3 00005 * @date 16-Jun-2014 00006 * 00007 * Released under the Apache License, Version 2.0 : http://mbed.org/handbook/Apache-Licence 00008 * 00009 * MARMEX_VB Camera control library for mbed 00010 */ 00011 00012 #ifndef MBED_MARMEX_VB 00013 #define MBED_MARMEX_VB 00014 00015 /** MARMEX_VB class 00016 * 00017 * MARMEX_VB camera control library 00018 * This driver provide controls and data transfer interface for MARMEX_VB 00019 * 00020 * Example: 00021 * @code 00022 * 00023 * #include "mbed.h" 00024 * #include "MARMEX_OB_oled.h" 00025 * #include "MARMEX_VB.h" 00026 * 00027 * MARMEX_OB_oled oled1( p5, p7, p20, p16, p15 ); // mosi, sclk, cs, rst, power_control -- maple-mini-type-b-board-slot1 00028 * MARMEX_VB camera( p5, p6, p7, p22, p26, p28, p27 ); // mosi, miso, sclk, cs, reset, I2C_SDA, I2C_SCL -- maple-mini-type-b-board-slot2 00029 * BusOut led( LED3, LED4 ); 00030 * 00031 * #define X_OFFSET ((MARMEX_VB::PIXEL_PER_LINE - MARMEX_OB_oled::WIDTH ) / 2) 00032 * #define Y_OFFSET ((MARMEX_VB::LINE_PER_FRAME - MARMEX_OB_oled::HEIGHT) / 2) 00033 * 00034 * int main() 00035 * { 00036 * led = 0x3; 00037 * 00038 * oled1.cls(); 00039 * 00040 * short buf[ MARMEX_OB_oled::WIDTH ]; 00041 * 00042 * while ( 1 ) { 00043 * 00044 * led = 0x1; 00045 * camera.open_transfer(); 00046 * 00047 * for ( int line = 0; line < 128; line++ ) { 00048 * camera.read_a_line( buf, line + Y_OFFSET, X_OFFSET, 128 ); 00049 * oled1.blit565( 0, line, 128, 1, buf ); 00050 * } 00051 * 00052 * camera.close_transfer(); 00053 * led = 0x2; 00054 * } 00055 * } 00056 * @endcode 00057 */ 00058 00059 #define OPTIMIZATION_ENABLED 00060 00061 #define DEFAULT_PICTURE_SIZE QCIF 00062 //#define DEFAULT_PICTURE_SIZE VGA 00063 //#define DEFAULT_PICTURE_SIZE QVGA 00064 //#define DEFAULT_PICTURE_SIZE QQVGA 00065 00066 class MARMEX_VB 00067 { 00068 public: 00069 typedef enum { 00070 QCIF = 1, /**< QCIF */ 00071 VGA, /**< VGA */ 00072 QVGA, /**< QVGA */ 00073 QQVGA, /**< QQVGA */ 00074 } CameraResolution ; 00075 00076 typedef enum { 00077 OFF = 0, /**< ON */ 00078 ON, /**< OFF */ 00079 } SwitchState ; 00080 00081 /** General parameters for MARMEX_VB */ 00082 enum { 00083 BYTE_PER_PIXEL = 2, /**< bytes per pixel */ 00084 00085 QCIF_PIXEL_PER_LINE = 176, /**< pixels in a line (QCIF horizontal size) */ 00086 QCIF_LINE_PER_FRAME = 144, /**< lines in a frame (QCIF vertical size) */ 00087 VGA_PIXEL_PER_LINE = 640, /**< pixels in a line (VGA horizontal size) */ 00088 VGA_LINE_PER_FRAME = 480, /**< lines in a frame (VGA vertical size) */ 00089 }; 00090 00091 /** General parameters for MARMEX_OB_oled */ 00092 enum { 00093 NO_ERROR = 0 /**< zero */ 00094 }; 00095 00096 /** Create a MARMEX_VB instance connected to specified SPI, DigitalOut and I2C pins 00097 * 00098 * @param SPI_mosi SPI-bus MOSI pin 00099 * @param SPI_miso SPI-bus MISO pin 00100 * @param SPI_sclk SPI-bus SCLK pin 00101 * @param SPI_cs SPI-bus Chip Select pin 00102 * @param cam_reset Camera reset pin 00103 * @param I2C_sda I2C-bus SDA pin 00104 * @param I2C_scl I2C-bus SCL pin 00105 */ 00106 00107 MARMEX_VB( 00108 PinName SPI_mosi, 00109 PinName SPI_miso, 00110 PinName SPI_sck, 00111 PinName SPI_cs, 00112 PinName cam_reset, 00113 PinName I2C_sda, 00114 PinName I2C_scl 00115 ); 00116 00117 /** Initialization 00118 * 00119 * Performs MARMEX_VB reset and initializations 00120 * This function is called from MARMEX_VB constoructor. So user don't have to call in the user code. 00121 * 00122 * This function takes about 2 seconds because there is 99 times I2C access with 20ms interval. 00123 * 00124 * @param res select camera resolution : QCIF(default), VGA, QVGA or QQVGA 00125 */ 00126 int init( CameraResolution res = QCIF ); 00127 00128 /** Color bar ON/OFF 00129 * 00130 * Set colorbar ON/OFF 00131 * 00132 * @param sw turn-ON or -OFF colorbar : ON or OFF 00133 */ 00134 void colorbar( SwitchState sw ); 00135 00136 /** Get holizontal size 00137 * 00138 * Returns horizontal image size (pixels) 00139 * 00140 * @return holizontal size 00141 */ 00142 int get_horizontal_size( void ); 00143 00144 /** Get vertical size 00145 * 00146 * Returns vertical image size (pixels) 00147 * 00148 * @return vertical size 00149 */ 00150 int get_vertical_size( void ); 00151 00152 /** Check camera availability 00153 * 00154 * Returns last I2C access result 00155 * This returns non-zero value if the camera initialization failed 00156 * 00157 * @return error code in init function (I2C API return value) 00158 */ 00159 int ready( void ); 00160 00161 /** Open transfer 00162 * 00163 * Let the MARMEX_VB get ready to transfer the data. 00164 * When this function is called, the camera will stop updating buffer (on camera board) at end of frame. 00165 * 00166 * @return error code in init function (I2C API return value) 00167 */ 00168 void open_transfer( void ); 00169 00170 /** Close transfer 00171 * 00172 * Letting the MARMEX_VB to know the data transfer done. 00173 * This function should be called when the data transfer done to resume the buffer update by camera 00174 */ 00175 void close_transfer( void ); 00176 00177 /** Read one line data 00178 * 00179 * Reads 1 line data from MARMEX_VB 00180 * This function should be called when the data transfer done to resume the buffer update by camera 00181 * 00182 * @param *p pointer to array of short 00183 * @param line_number to select which line want to read 00184 * @param x_offset holizontal offset (from left) to start the read 00185 * @param n_of_pixels pixels to be read 00186 */ 00187 void read_a_line( short *p, int line_number, int x_offset, int n_of_pixels ); 00188 00189 /** Read order change 00190 * 00191 * Toggles flag for read order of 2 byte data. 00192 * 00193 * @return new status of the read order flag (false = normal / true = swapped) 00194 */ 00195 int read_order_change( void ); 00196 00197 private: 00198 int send_spi( char data ); 00199 void write_register( char reg, char value ); 00200 int read_register( char reg ); 00201 void set_address( int address ); 00202 00203 SPI _spi; 00204 DigitalOut _cs; 00205 DigitalOut _reset; 00206 I2C _i2c; 00207 int _error_state; 00208 int _horizontal_size; 00209 int _vertical_size; 00210 int _read_order_change; 00211 00212 }; 00213 00214 #endif // MBED_MARMEX_VB
Generated on Wed Jul 13 2022 17:47:09 by
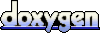