Implemented first Hangar-Service
Dependencies: CalibrateMagneto QuaternionMath
Fork of SML2 by
PIDController Class Reference
Implements a PID controller. More...
#include <PIDController.h>
Public Member Functions | |
PIDController (float const _Kp=1.0f, float const _Ki=0.0f, float const _Kd=0.0f, float const _setpoint=0.0f, bool const _clippingEnabled=false, float const _maximum=0.0) | |
Constructor. | |
void | reset () |
Reset PID controller (integral, differential) to zero. | |
float | output (float const input) |
Performs one iteration of the PID control loop. | |
Data Fields | |
float | Kp |
The proportional term of the PID controller. | |
float | Ki |
The integral term of the PID controller. | |
float | Kd |
The differential term of the PID controller. | |
float | setPoint |
The set point of the PID controller (the output value that it will try to maintain) | |
bool | clippingEnabled |
Whether to clip the integral term so that it never exceeds the maximum. | |
float | maximum |
The maximum value the integral term is allowed to reach, if clipping is enabled. | |
float | e |
error signal (setpoint minus input) | |
float | int_e |
integral of error signal | |
float | diff_e |
derivative of error signal | |
float | prev_e |
error at t_-1 |
Detailed Description
Implements a PID controller.
A PID controller can be used to control some variable parameter (the 'output') so that the input reaches a particular 'set point.' This is done by changing the output a little and checking the error still remaining. The parameters Kp, Ki and Kd control the time behaviour.
Definition at line 10 of file PIDController.h.
Constructor & Destructor Documentation
PIDController | ( | float const | _Kp = 1.0f , |
float const | _Ki = 0.0f , |
||
float const | _Kd = 0.0f , |
||
float const | _setpoint = 0.0f , |
||
bool const | _clippingEnabled = false , |
||
float const | _maximum = 0.0 |
||
) |
Constructor.
- Parameters:
-
_Kp initial Kp, default = 1.0 _Ki initial Ki, default = 0.0 _Kd initial Kd, default = 0.0 _setpoint initial setpoint, default = 0.0 _clippingEnabled whether the PID controller integral term will be clipped _maximum if clipping is enabled, the maximum value of the integral term
Definition at line 20 of file PIDController.h.
Member Function Documentation
float output | ( | float const | input ) |
Performs one iteration of the PID control loop.
If clipping
- Parameters:
-
input The input to the PID controller
- Returns:
- The PID controller's output
Definition at line 51 of file PIDController.h.
void reset | ( | ) |
Reset PID controller (integral, differential) to zero.
Definition at line 43 of file PIDController.h.
Field Documentation
bool clippingEnabled |
Whether to clip the integral term so that it never exceeds the maximum.
Definition at line 35 of file PIDController.h.
float diff_e |
derivative of error signal
Definition at line 39 of file PIDController.h.
float e |
error signal (setpoint minus input)
Definition at line 37 of file PIDController.h.
float int_e |
integral of error signal
Definition at line 38 of file PIDController.h.
float Kd |
The differential term of the PID controller.
Definition at line 33 of file PIDController.h.
float Ki |
The integral term of the PID controller.
Definition at line 32 of file PIDController.h.
float Kp |
The proportional term of the PID controller.
Definition at line 31 of file PIDController.h.
float maximum |
The maximum value the integral term is allowed to reach, if clipping is enabled.
Definition at line 36 of file PIDController.h.
float prev_e |
error at t_-1
Definition at line 40 of file PIDController.h.
float setPoint |
The set point of the PID controller (the output value that it will try to maintain)
Definition at line 34 of file PIDController.h.
Generated on Wed Jul 13 2022 08:50:41 by
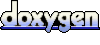