Beacon demo for the BLE API using the nRF51822 native mode drivers
Dependencies: BLE_API mbed nRF51822 X_NUCLEO_IDB0XA1
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "ble/services/iBeacon.h" 00019 00020 BLE ble; 00021 00022 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00023 { 00024 BLE &ble = params->ble; 00025 ble_error_t error = params->error; 00026 00027 if (error != BLE_ERROR_NONE) { 00028 return; 00029 } 00030 00031 /** 00032 * The Beacon payload has the following composition: 00033 * 128-Bit / 16byte UUID = E2 0A 39 F4 73 F5 4B C4 A1 2F 17 D1 AD 07 A9 61 00034 * Major/Minor = 0x1122 / 0x3344 00035 * Tx Power = 0xC8 = 200, 2's compliment is 256-200 = (-56dB) 00036 * 00037 * Note: please remember to calibrate your beacons TX Power for more accurate results. 00038 */ 00039 const uint8_t uuid[] = {0xE2, 0x0A, 0x39, 0xF4, 0x73, 0xF5, 0x4B, 0xC4, 00040 0xA1, 0x2F, 0x17, 0xD1, 0xAD, 0x07, 0xA9, 0x61}; 00041 uint16_t majorNumber = 1122; 00042 uint16_t minorNumber = 3344; 00043 uint16_t txPower = 0xC8; 00044 iBeacon *ibeacon = new iBeacon(ble, uuid, majorNumber, minorNumber, txPower); 00045 00046 ble.gap().setAdvertisingInterval(1000); /* 1000ms. */ 00047 ble.gap().startAdvertising(); 00048 } 00049 00050 int main(void) 00051 { 00052 ble.init(bleInitComplete); 00053 00054 /* SpinWait for initialization to complete. This is necessary because the 00055 * BLE object is used in the main loop below. */ 00056 while (!ble.hasInitialized()) { /* spin loop */ } 00057 00058 while (true) { 00059 ble.waitForEvent(); // allows or low power operation 00060 } 00061 }
Generated on Tue Jul 12 2022 17:14:15 by
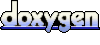