
AltBeacon program for embedded BLE. This program demonstrates how to set up a BLE device to broadcast AltBLE compatible data. Please see the official website for more details. https://github.com/AltBeacon/spec and http://altbeacon.org/
Dependencies: BLE_API mbed nRF51822
Fork of BLE_AltBeacon by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "AltBeaconService.h" 00019 #include "ble/BLE.h" 00020 00021 /** 00022 * For this demo application, populate the beacon advertisement payload 00023 * with 2 AD structures: FLAG and MSD (manufacturer specific data). 00024 * 00025 * Reference: 00026 * Bluetooth Core Specification 4.0 (Vol. 3), Part C, Section 11, 18 00027 */ 00028 00029 /** 00030 * The AltBeacon requires a manufacturer ID, and a Beacon ID 00031 * the first 16 bytes of the BeaconID should be a UUID and the remaining 00032 * 4 bytes can be used as you see fit. 00033 * 00034 * Note: please remember to calibrate your beacon 00035 * RSSI for more accurate results. 00036 */ 00037 uint8_t beaconID[] = {0x00,0x01,0x02,0x03,0x04,0x05,0x06,0x07,0x08,0x09, 00038 0x10,0x11,0x12,0x13,0x14,0x15,0x00,0x01,0x00,0x02}; 00039 uint16_t manufacturerID = 0x5900; /* Nordic SIG ID */ 00040 int8_t rssi = -122; 00041 00042 AltBeaconService *altBeaconServicePtr; 00043 00044 /** 00045 * This function is called when the ble initialization process has failed 00046 */ 00047 void onBleInitError(BLE &ble, ble_error_t error) 00048 { 00049 /* Initialization error handling should go here */ 00050 } 00051 00052 /** 00053 * Callback triggered when the ble initialization process has finished 00054 */ 00055 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00056 { 00057 BLE& ble = params->ble; 00058 ble_error_t error = params->error; 00059 00060 if (error != BLE_ERROR_NONE) { 00061 /* In case of error, forward the error handling to onBleInitError */ 00062 onBleInitError(ble, error); 00063 return; 00064 } 00065 00066 /* Ensure that it is the default instance of BLE */ 00067 if(ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00068 return; 00069 } 00070 00071 /* Initialize AltBeacon */ 00072 altBeaconServicePtr =new AltBeaconService(ble, manufacturerID, beaconID, rssi); 00073 00074 /* Set advertising time */ 00075 ble.setAdvertisingInterval(160); /* 100ms; in multiples of 0.625ms. */ 00076 00077 /* Start advertising */ 00078 ble.startAdvertising(); 00079 } 00080 00081 int main(void) 00082 { 00083 BLE& ble = BLE::Instance(); 00084 00085 /* Initialize BLE baselayer */ 00086 ble.init(bleInitComplete); 00087 00088 while(true) { 00089 ble.waitForEvent(); /* Allow low power operation */ 00090 } 00091 }
Generated on Sat Jul 16 2022 23:03:38 by
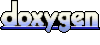