
AltBeacon program for embedded BLE. This program demonstrates how to set up a BLE device to broadcast AltBLE compatible data. Please see the official website for more details. https://github.com/AltBeacon/spec and http://altbeacon.org/
Dependencies: BLE_API mbed nRF51822
Fork of BLE_AltBeacon by
AltBeaconService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef __BLE_ALTBEACON_SERVICE_H__ 00017 #define __BLE_ALTBEACON_SERVICE_H__ 00018 00019 #include "ble/BLE.h" 00020 00021 /** 00022 * @class AltBeaconService 00023 * @brief AltBeacon Service. This service sets up a device to broadcast advertising packets to mimic an AltBeacon<br> 00024 */ 00025 00026 class AltBeaconService 00027 { 00028 public: 00029 /** 00030 * @param[in] _ble 00031 * BLE object for the underlying controller. 00032 * @param[in] mfgID 00033 * The beacon device manufacturer's company identifier code. 00034 * Usually this will coorespond to the companies BLE SIG assigned number. 00035 * @param[in] beaconID 00036 * A 20-byte value uniquely identifying the beacon. 00037 * The big endian representation of the beacon identifier. 00038 * For interoperability purposes, the first 16+ bytes of the beacon 00039 * identifier should be unique to the advertiser's organizational unit. 00040 * Any remaining bytes of the beacon identifier may be subdivided as needed for the use case. 00041 * @param[in] refRSSI 00042 * The RSSI of the beacon (as signed value from 0 to -127) as measured 1 meter from the device. Used for micro-location. 00043 * @param[in] mfgReserved 00044 * Used for special manufacturer data. Defaults to 0x00 if not specified. 00045 */ 00046 AltBeaconService (BLE &_ble, uint16_t mfgID, uint8_t beaconID[20], int8_t refRSSI, uint8_t mfgReserved = 0x00): 00047 ble(_ble) 00048 { 00049 /* refRSSI can only be 0 to -127, smash everything above 0 to zero */ 00050 if (refRSSI > 0) { 00051 refRSSI = 0; 00052 } 00053 data.mfgID = ((mfgID<<8) | (mfgID >>8)); 00054 data.refRSSI = refRSSI; 00055 data.beaconCode = 0xACBE; 00056 data.mfgReserved = mfgReserved; 00057 00058 /* copy across beacon ID */ 00059 for(int x = 0; x < sizeof(data.beaconID); x++) { 00060 data.beaconID[x] = beaconID[x]; 00061 } 00062 00063 /* Set up alt beacon */ 00064 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00065 /* Generate the 0x1BFF part of the Alt Prefix */ 00066 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, data.raw, sizeof(data.raw)); 00067 00068 /* Set advertising type */ 00069 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_NON_CONNECTABLE_UNDIRECTED); 00070 } 00071 00072 public: 00073 union { 00074 uint8_t raw[26]; // AltBeacon advertisment data 00075 struct { 00076 uint16_t mfgID; // little endian representation of manufacturer ID 00077 uint16_t beaconCode; // Big Endian representation of 0xBEAC 00078 uint8_t beaconID[20]; // 20byte beacon ID, usually 16byte UUID w/ remainder used as necessary 00079 int8_t refRSSI; // 1 byte signed data, 0 to -127 00080 uint8_t mfgReserved; // reserved for use by manufacturer to implement special features 00081 }; 00082 } data; 00083 00084 private: 00085 BLE &ble; 00086 00087 }; 00088 00089 #endif /* __BLE_ALTBEACON_SERVICE_H__ */
Generated on Sat Jul 16 2022 23:03:38 by
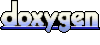