High level Bluetooth Low Energy API and radio abstraction layer
Dependents: BLE_ANCS_SDAPI BLE_temperature BLE_HeartRate BLE_ANCS_SDAPI_IRC ... more
DiscoveredService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __DISCOVERED_SERVICE_H__ 00018 #define __DISCOVERED_SERVICE_H__ 00019 00020 #include "UUID.h" 00021 #include "GattAttribute.h" 00022 00023 /**@brief Type for holding information about the service and the characteristics found during 00024 * the discovery process. 00025 */ 00026 class DiscoveredService { 00027 public: 00028 /** 00029 * Set information about the discovered service. 00030 * 00031 * @param[in] uuidIn 00032 * The UUID of the discovered service. 00033 * @param[in] startHandleIn 00034 * The start handle of the discovered service in the peer's 00035 * ATT table. 00036 * @param[in] endHandleIn 00037 * The end handle of the discovered service in the peer's 00038 * ATT table. 00039 */ 00040 void setup(UUID uuidIn, GattAttribute::Handle_t startHandleIn, GattAttribute::Handle_t endHandleIn) { 00041 uuid = uuidIn; 00042 startHandle = startHandleIn; 00043 endHandle = endHandleIn; 00044 } 00045 00046 /** 00047 * Set the start and end handle of the discovered service. 00048 * @param[in] startHandleIn 00049 * The start handle of the discovered service in the peer's 00050 * ATT table. 00051 * @param[in] endHandleIn 00052 * The end handle of the discovered service in the peer's 00053 * ATT table. 00054 */ 00055 void setup(GattAttribute::Handle_t startHandleIn, GattAttribute::Handle_t endHandleIn) { 00056 startHandle = startHandleIn; 00057 endHandle = endHandleIn; 00058 } 00059 00060 /** 00061 * Set the long UUID of the discovered service. 00062 * 00063 * @param[in] longUUID 00064 * The long UUID of the discovered service. 00065 * @param[in] order 00066 * The byte ordering of @p longUUID. 00067 */ 00068 void setupLongUUID(UUID::LongUUIDBytes_t longUUID, UUID::ByteOrder_t order = UUID::MSB) { 00069 uuid.setupLong(longUUID, order); 00070 } 00071 00072 public: 00073 /** 00074 * Get the UUID of the discovered service. 00075 * 00076 * @return A reference to the UUID of the discovered service. 00077 */ 00078 const UUID &getUUID(void) const { 00079 return uuid; 00080 } 00081 00082 /** 00083 * Get the start handle of the discovered service in the peer's ATT table. 00084 * 00085 * @return A reference to the start handle. 00086 */ 00087 const GattAttribute::Handle_t& getStartHandle(void) const { 00088 return startHandle; 00089 } 00090 00091 /** 00092 * Get the end handle of the discovered service in the peer's ATT table. 00093 * 00094 * @return A reference to the end handle. 00095 */ 00096 const GattAttribute::Handle_t& getEndHandle(void) const { 00097 return endHandle; 00098 } 00099 00100 public: 00101 /** 00102 * Construct a DiscoveredService instance. 00103 */ 00104 DiscoveredService() : uuid(UUID::ShortUUIDBytes_t(0)), 00105 startHandle(GattAttribute::INVALID_HANDLE), 00106 endHandle(GattAttribute::INVALID_HANDLE) { 00107 /* empty */ 00108 } 00109 00110 private: 00111 DiscoveredService(const DiscoveredService &); 00112 00113 private: 00114 UUID uuid; /**< UUID of the service. */ 00115 GattAttribute::Handle_t startHandle; /**< Service Handle Range. */ 00116 GattAttribute::Handle_t endHandle; /**< Service Handle Range. */ 00117 }; 00118 00119 #endif /*__DISCOVERED_SERVICE_H__*/
Generated on Tue Jul 12 2022 12:49:01 by
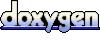