adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
ServiceDiscovery.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __SERVICE_DISOVERY_H__ 00018 #define __SERVICE_DISOVERY_H__ 00019 00020 #include "UUID.h" 00021 #include "Gap.h" 00022 #include "GattAttribute.h" 00023 00024 class DiscoveredService; 00025 class DiscoveredCharacteristic; 00026 00027 class ServiceDiscovery { 00028 public: 00029 /* 00030 * Exposed application callback types. 00031 */ 00032 00033 /** 00034 * Callback type for when a matching service is found during service- 00035 * discovery. The receiving function is passed in a pointer to a 00036 * DiscoveredService object, which will remain valid for the lifetime of the 00037 * callback. Memory for this object is owned by the BLE_API eventing 00038 * framework. The application can safely make a persistent shallow-copy of 00039 * this object to work with the service beyond the callback. 00040 */ 00041 typedef FunctionPointerWithContext<const DiscoveredService *> ServiceCallback_t; 00042 00043 /** 00044 * Callback type for when a matching characteristic is found during service- 00045 * discovery. The receiving function is passed in a pointer to a 00046 * DiscoveredCharacteristic object, which will remain valid for the lifetime 00047 * of the callback. Memory for this object is owned by the BLE_API eventing 00048 * framework. The application can safely make a persistent shallow-copy of 00049 * this object to work with the characteristic beyond the callback. 00050 */ 00051 typedef FunctionPointerWithContext<const DiscoveredCharacteristic *> CharacteristicCallback_t; 00052 00053 /** 00054 * Callback type for when serviceDiscovery terminates. 00055 */ 00056 typedef FunctionPointerWithContext<Gap::Handle_t> TerminationCallback_t; 00057 00058 public: 00059 /** 00060 * Launch service discovery. Once launched, service discovery will remain 00061 * active with callbacks being issued back into the application for matching 00062 * services or characteristics. isActive() can be used to determine status, and 00063 * a termination callback (if set up) will be invoked at the end. Service 00064 * discovery can be terminated prematurely, if needed, using terminate(). 00065 * 00066 * @param connectionHandle 00067 * Handle for the connection with the peer. 00068 * @param sc 00069 * This is the application callback for a matching service. Taken as 00070 * NULL by default. Note: service discovery may still be active 00071 * when this callback is issued; calling asynchronous BLE-stack 00072 * APIs from within this application callback might cause the 00073 * stack to abort service discovery. If this becomes an issue, it 00074 * may be better to make a local copy of the discoveredService and 00075 * wait for service discovery to terminate before operating on the 00076 * service. 00077 * @param cc 00078 * This is the application callback for a matching characteristic. 00079 * Taken as NULL by default. Note: service discovery may still be 00080 * active when this callback is issued; calling asynchronous 00081 * BLE-stack APIs from within this application callback might cause 00082 * the stack to abort service discovery. If this becomes an issue, 00083 * it may be better to make a local copy of the discoveredCharacteristic 00084 * and wait for service discovery to terminate before operating on the 00085 * characteristic. 00086 * @param matchingServiceUUID 00087 * UUID-based filter for specifying a service in which the application is 00088 * interested. By default it is set as the wildcard UUID_UNKNOWN, 00089 * in which case it matches all services. If characteristic-UUID 00090 * filter (below) is set to the wildcard value, then a service 00091 * callback will be invoked for the matching service (or for every 00092 * service if the service filter is a wildcard). 00093 * @param matchingCharacteristicUUIDIn 00094 * UUID-based filter for specifying a characteristic in which the application 00095 * is interested. By default it is set as the wildcard UUID_UKNOWN 00096 * to match against any characteristic. If both service-UUID 00097 * filter and characteristic-UUID filter are used with non-wildcard 00098 * values, then only a single characteristic callback is 00099 * invoked for the matching characteristic. 00100 * 00101 * @note Using wildcard values for both service-UUID and characteristic- 00102 * UUID will result in complete service discovery: callbacks being 00103 * called for every service and characteristic. 00104 * 00105 * @note Providing NULL for the characteristic callback will result in 00106 * characteristic discovery being skipped for each matching 00107 * service. This allows for an inexpensive method to discover only 00108 * services. 00109 * 00110 * @return 00111 * BLE_ERROR_NONE if service discovery is launched successfully; else an appropriate error. 00112 */ 00113 virtual ble_error_t launch(Gap::Handle_t connectionHandle, 00114 ServiceCallback_t sc = NULL, 00115 CharacteristicCallback_t cc = NULL, 00116 const UUID &matchingServiceUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN), 00117 const UUID &matchingCharacteristicUUIDIn = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN)) = 0; 00118 00119 /** 00120 * Check whether service-discovery is currently active. 00121 */ 00122 virtual bool isActive(void) const = 0; 00123 00124 /** 00125 * Terminate an ongoing service discovery. This should result in an 00126 * invocation of the TerminationCallback if service discovery is active. 00127 */ 00128 virtual void terminate(void) = 0; 00129 00130 /** 00131 * Set up a callback to be invoked when service discovery is terminated. 00132 */ 00133 virtual void onTermination(TerminationCallback_t callback) = 0; 00134 00135 /** 00136 * Clear all ServiceDiscovery state of the associated object. 00137 * 00138 * This function is meant to be overridden in the platform-specific 00139 * sub-class. Nevertheless, the sub-class is only expected to reset its 00140 * state and not the data held in ServiceDiscovery members. This shall be 00141 * achieved by a call to ServiceDiscovery::reset() from the sub-class' 00142 * reset() implementation. 00143 * 00144 * @return BLE_ERROR_NONE on success. 00145 */ 00146 virtual ble_error_t reset(void) { 00147 connHandle = 0; 00148 matchingServiceUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN); 00149 serviceCallback = NULL; 00150 matchingCharacteristicUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN); 00151 characteristicCallback = NULL; 00152 00153 return BLE_ERROR_NONE; 00154 } 00155 00156 protected: 00157 /** 00158 * Connection handle as provided by the SoftDevice. 00159 */ 00160 Gap::Handle_t connHandle; 00161 /** 00162 * UUID-based filter that specifies the service that the application is 00163 * interested in. 00164 */ 00165 UUID matchingServiceUUID; 00166 /** 00167 * The registered callback handle for when a matching service is found 00168 * during service-discovery. 00169 */ 00170 ServiceCallback_t serviceCallback; 00171 /** 00172 * UUID-based filter that specifies the characteristic that the 00173 * application is interested in. 00174 */ 00175 UUID matchingCharacteristicUUID; 00176 /** 00177 * The registered callback handler for when a matching characteristic is 00178 * found during service-discovery. 00179 */ 00180 CharacteristicCallback_t characteristicCallback; 00181 }; 00182 00183 #endif /* ifndef __SERVICE_DISOVERY_H__ */
Generated on Wed Jul 13 2022 09:31:10 by
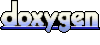