adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
LinkLossService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __BLE_LINK_LOSS_SERVICE_H__ 00018 #define __BLE_LINK_LOSS_SERVICE_H__ 00019 00020 #include "ble/Gap.h" 00021 00022 /** 00023 * @class LinkLossService 00024 * @brief This service defines behavior when a link is lost between two devices. 00025 * Service: https://developer.bluetooth.org/gatt/services/Pages/ServiceViewer.aspx?u=org.bluetooth.service.link_loss.xml 00026 * Alertness Level Char: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.alert_level.xml 00027 */ 00028 class LinkLossService { 00029 public: 00030 enum AlertLevel_t { 00031 NO_ALERT = 0, 00032 MILD_ALERT = 1, 00033 HIGH_ALERT = 2 00034 }; 00035 00036 typedef void (* callback_t)(AlertLevel_t level); 00037 00038 /** 00039 * @param[ref] ble 00040 * BLE object for the underlying controller. 00041 */ 00042 LinkLossService (BLE &bleIn, callback_t callbackIn, AlertLevel_t levelIn = NO_ALERT) : 00043 ble(bleIn), 00044 alertLevel(levelIn), 00045 callback(callbackIn), 00046 alertLevelChar(GattCharacteristic::UUID_ALERT_LEVEL_CHAR, reinterpret_cast<uint8_t *>(&alertLevel)) { 00047 static bool serviceAdded = false; /* We should only ever add one LinkLoss service. */ 00048 if (serviceAdded) { 00049 return; 00050 } 00051 00052 GattCharacteristic *charTable[] = {&alertLevelChar}; 00053 GattService linkLossService(GattService::UUID_LINK_LOSS_SERVICE, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00054 00055 ble.gattServer().addService(linkLossService); 00056 serviceAdded = true; 00057 00058 ble.gap().onDisconnection(this, &LinkLossService::onDisconnectionFilter); 00059 ble.gattServer().onDataWritten(this, &LinkLossService::onDataWritten); 00060 } 00061 00062 /** 00063 * Update the callback. 00064 */ 00065 void setCallback(callback_t newCallback) { 00066 callback = newCallback; 00067 } 00068 00069 /** 00070 * Update alertness level. 00071 */ 00072 void setAlertLevel(AlertLevel_t newLevel) { 00073 alertLevel = newLevel; 00074 } 00075 00076 protected: 00077 /** 00078 * This callback allows receiving updates to the AlertLevel characteristic. 00079 * 00080 * @param[in] params 00081 * Information about the characterisitc being updated. 00082 */ 00083 virtual void onDataWritten(const GattWriteCallbackParams *params) { 00084 if (params->handle == alertLevelChar.getValueHandle()) { 00085 alertLevel = *reinterpret_cast<const AlertLevel_t *>(params->data); 00086 } 00087 } 00088 00089 void onDisconnectionFilter(const Gap::DisconnectionCallbackParams_t *params) { 00090 if (alertLevel != NO_ALERT) { 00091 callback(alertLevel); 00092 } 00093 } 00094 00095 protected: 00096 BLE &ble; 00097 AlertLevel_t alertLevel; 00098 callback_t callback; 00099 00100 ReadWriteGattCharacteristic<uint8_t> alertLevelChar; 00101 }; 00102 00103 #endif /* __BLE_LINK_LOSS_SERVICE_H__ */
Generated on Wed Jul 13 2022 09:31:10 by
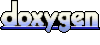