adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
HeartRateService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __BLE_HEART_RATE_SERVICE_H__ 00018 #define __BLE_HEART_RATE_SERVICE_H__ 00019 00020 #include "ble/BLE.h" 00021 00022 /** 00023 * @class HeartRateService 00024 * @brief BLE Service for HeartRate. This BLE Service contains the location of the sensor and the heart rate in beats per minute. 00025 * Service: https://developer.bluetooth.org/gatt/services/Pages/ServiceViewer.aspx?u=org.bluetooth.service.heart_rate.xml 00026 * HRM Char: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.heart_rate_measurement.xml 00027 * Location: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.body_sensor_location.xml 00028 */ 00029 class HeartRateService { 00030 public: 00031 /** 00032 * @enum SensorLocation 00033 * @brief Location of the heart rate sensor on body. 00034 */ 00035 enum { 00036 LOCATION_OTHER = 0, /*!< Other location. */ 00037 LOCATION_CHEST , /*!< Chest. */ 00038 LOCATION_WRIST , /*!< Wrist. */ 00039 LOCATION_FINGER , /*!< Finger. */ 00040 LOCATION_HAND , /*!< Hand. */ 00041 LOCATION_EAR_LOBE , /*!< Earlobe. */ 00042 LOCATION_FOOT , /*!< Foot. */ 00043 }; 00044 00045 public: 00046 /** 00047 * @brief Constructor with 8-bit HRM Counter value. 00048 * 00049 * @param[ref] _ble 00050 * Reference to the underlying BLE. 00051 * @param[in] hrmCounter (8-bit) 00052 * Initial value for the HRM counter. 00053 * @param[in] location 00054 * Sensor's location. 00055 */ 00056 HeartRateService(BLE &_ble, uint8_t hrmCounter, uint8_t location) : 00057 ble(_ble), 00058 valueBytes(hrmCounter), 00059 hrmRate(GattCharacteristic::UUID_HEART_RATE_MEASUREMENT_CHAR, valueBytes.getPointer(), 00060 valueBytes.getNumValueBytes(), HeartRateValueBytes::MAX_VALUE_BYTES, 00061 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00062 hrmLocation(GattCharacteristic::UUID_BODY_SENSOR_LOCATION_CHAR, &location), 00063 controlPoint(GattCharacteristic::UUID_HEART_RATE_CONTROL_POINT_CHAR, &controlPointValue) { 00064 setupService(); 00065 } 00066 00067 /** 00068 * @brief Constructor with a 16-bit HRM Counter value. 00069 * 00070 * @param[in] _ble 00071 * Reference to the underlying BLE. 00072 * @param[in] hrmCounter (8-bit) 00073 * Initial value for the HRM counter. 00074 * @param[in] location 00075 * Sensor's location. 00076 */ 00077 HeartRateService(BLE &_ble, uint16_t hrmCounter, uint8_t location) : 00078 ble(_ble), 00079 valueBytes(hrmCounter), 00080 hrmRate(GattCharacteristic::UUID_HEART_RATE_MEASUREMENT_CHAR, valueBytes.getPointer(), 00081 valueBytes.getNumValueBytes(), HeartRateValueBytes::MAX_VALUE_BYTES, 00082 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00083 hrmLocation(GattCharacteristic::UUID_BODY_SENSOR_LOCATION_CHAR, &location), 00084 controlPoint(GattCharacteristic::UUID_HEART_RATE_CONTROL_POINT_CHAR, &controlPointValue) { 00085 setupService(); 00086 } 00087 00088 /** 00089 * @brief Set a new 8-bit value for the heart rate. 00090 * 00091 * @param[in] hrmCounter 00092 * Heart rate in BPM. 00093 */ 00094 void updateHeartRate(uint8_t hrmCounter) { 00095 valueBytes.updateHeartRate(hrmCounter); 00096 ble.gattServer().write(hrmRate.getValueHandle(), valueBytes.getPointer(), valueBytes.getNumValueBytes()); 00097 } 00098 00099 /** 00100 * Set a new 16-bit value for the heart rate. 00101 * 00102 * @param[in] hrmCounter 00103 * Heart rate in BPM. 00104 */ 00105 void updateHeartRate(uint16_t hrmCounter) { 00106 valueBytes.updateHeartRate(hrmCounter); 00107 ble.gattServer().write(hrmRate.getValueHandle(), valueBytes.getPointer(), valueBytes.getNumValueBytes()); 00108 } 00109 00110 /** 00111 * This callback allows the heart rate service to receive updates to the 00112 * controlPoint characteristic. 00113 * 00114 * @param[in] params 00115 * Information about the characterisitc being updated. 00116 */ 00117 virtual void onDataWritten(const GattWriteCallbackParams *params) { 00118 if (params->handle == controlPoint.getValueAttribute().getHandle()) { 00119 /* Do something here if the new value is 1; else you can override this method by 00120 * extending this class. 00121 * @NOTE: If you are extending this class, be sure to also call 00122 * ble.onDataWritten(this, &ExtendedHRService::onDataWritten); in 00123 * your constructor. 00124 */ 00125 } 00126 } 00127 00128 protected: 00129 void setupService(void) { 00130 GattCharacteristic *charTable[] = {&hrmRate, &hrmLocation, &controlPoint}; 00131 GattService hrmService(GattService::UUID_HEART_RATE_SERVICE, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00132 00133 ble.addService(hrmService); 00134 ble.onDataWritten(this, &HeartRateService::onDataWritten); 00135 } 00136 00137 protected: 00138 /* Private internal representation for the bytes used to work with the value of the heart rate characteristic. */ 00139 struct HeartRateValueBytes { 00140 static const unsigned MAX_VALUE_BYTES = 3; /* Flags, and up to two bytes for heart rate. */ 00141 static const unsigned FLAGS_BYTE_INDEX = 0; 00142 00143 static const unsigned VALUE_FORMAT_BITNUM = 0; 00144 static const uint8_t VALUE_FORMAT_FLAG = (1 << VALUE_FORMAT_BITNUM); 00145 00146 HeartRateValueBytes(uint8_t hrmCounter) : valueBytes() { 00147 updateHeartRate(hrmCounter); 00148 } 00149 00150 HeartRateValueBytes(uint16_t hrmCounter) : valueBytes() { 00151 updateHeartRate(hrmCounter); 00152 } 00153 00154 void updateHeartRate(uint8_t hrmCounter) { 00155 valueBytes[FLAGS_BYTE_INDEX] &= ~VALUE_FORMAT_FLAG; 00156 valueBytes[FLAGS_BYTE_INDEX + 1] = hrmCounter; 00157 } 00158 00159 void updateHeartRate(uint16_t hrmCounter) { 00160 valueBytes[FLAGS_BYTE_INDEX] |= VALUE_FORMAT_FLAG; 00161 valueBytes[FLAGS_BYTE_INDEX + 1] = (uint8_t)(hrmCounter & 0xFF); 00162 valueBytes[FLAGS_BYTE_INDEX + 2] = (uint8_t)(hrmCounter >> 8); 00163 } 00164 00165 uint8_t *getPointer(void) { 00166 return valueBytes; 00167 } 00168 00169 const uint8_t *getPointer(void) const { 00170 return valueBytes; 00171 } 00172 00173 unsigned getNumValueBytes(void) const { 00174 return 1 + ((valueBytes[FLAGS_BYTE_INDEX] & VALUE_FORMAT_FLAG) ? sizeof(uint16_t) : sizeof(uint8_t)); 00175 } 00176 00177 private: 00178 /* First byte: 8-bit values, no extra info. Second byte: uint8_t HRM value */ 00179 /* See https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.heart_rate_measurement.xml */ 00180 uint8_t valueBytes[MAX_VALUE_BYTES]; 00181 }; 00182 00183 protected: 00184 BLE &ble; 00185 00186 HeartRateValueBytes valueBytes; 00187 uint8_t controlPointValue; 00188 00189 GattCharacteristic hrmRate; 00190 ReadOnlyGattCharacteristic<uint8_t> hrmLocation; 00191 WriteOnlyGattCharacteristic<uint8_t> controlPoint; 00192 }; 00193 00194 #endif /* #ifndef __BLE_HEART_RATE_SERVICE_H__*/
Generated on Wed Jul 13 2022 09:31:10 by
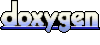