adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
GattCharacteristic.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_CHARACTERISTIC_H__ 00018 #define __GATT_CHARACTERISTIC_H__ 00019 00020 #include "Gap.h" 00021 #include "SecurityManager.h" 00022 #include "GattAttribute.h" 00023 #include "GattCallbackParamTypes.h" 00024 #include "FunctionPointerWithContext.h" 00025 00026 class GattCharacteristic { 00027 public: 00028 enum { 00029 UUID_BATTERY_LEVEL_STATE_CHAR = 0x2A1B, 00030 UUID_BATTERY_POWER_STATE_CHAR = 0x2A1A, 00031 UUID_REMOVABLE_CHAR = 0x2A3A, 00032 UUID_SERVICE_REQUIRED_CHAR = 0x2A3B, 00033 UUID_ALERT_CATEGORY_ID_CHAR = 0x2A43, 00034 UUID_ALERT_CATEGORY_ID_BIT_MASK_CHAR = 0x2A42, 00035 UUID_ALERT_LEVEL_CHAR = 0x2A06, 00036 UUID_ALERT_NOTIFICATION_CONTROL_POINT_CHAR = 0x2A44, 00037 UUID_ALERT_STATUS_CHAR = 0x2A3F, 00038 UUID_BATTERY_LEVEL_CHAR = 0x2A19, 00039 UUID_BLOOD_PRESSURE_FEATURE_CHAR = 0x2A49, 00040 UUID_BLOOD_PRESSURE_MEASUREMENT_CHAR = 0x2A35, 00041 UUID_BODY_SENSOR_LOCATION_CHAR = 0x2A38, 00042 UUID_BOOT_KEYBOARD_INPUT_REPORT_CHAR = 0x2A22, 00043 UUID_BOOT_KEYBOARD_OUTPUT_REPORT_CHAR = 0x2A32, 00044 UUID_BOOT_MOUSE_INPUT_REPORT_CHAR = 0x2A33, 00045 UUID_CURRENT_TIME_CHAR = 0x2A2B, 00046 UUID_DATE_TIME_CHAR = 0x2A08, 00047 UUID_DAY_DATE_TIME_CHAR = 0x2A0A, 00048 UUID_DAY_OF_WEEK_CHAR = 0x2A09, 00049 UUID_DST_OFFSET_CHAR = 0x2A0D, 00050 UUID_EXACT_TIME_256_CHAR = 0x2A0C, 00051 UUID_FIRMWARE_REVISION_STRING_CHAR = 0x2A26, 00052 UUID_GLUCOSE_FEATURE_CHAR = 0x2A51, 00053 UUID_GLUCOSE_MEASUREMENT_CHAR = 0x2A18, 00054 UUID_GLUCOSE_MEASUREMENT_CONTEXT_CHAR = 0x2A34, 00055 UUID_HARDWARE_REVISION_STRING_CHAR = 0x2A27, 00056 UUID_HEART_RATE_CONTROL_POINT_CHAR = 0x2A39, 00057 UUID_HEART_RATE_MEASUREMENT_CHAR = 0x2A37, 00058 UUID_HID_CONTROL_POINT_CHAR = 0x2A4C, 00059 UUID_HID_INFORMATION_CHAR = 0x2A4A, 00060 UUID_HUMIDITY_CHAR = 0x2A6F, 00061 UUID_IEEE_REGULATORY_CERTIFICATION_DATA_LIST_CHAR = 0x2A2A, 00062 UUID_INTERMEDIATE_CUFF_PRESSURE_CHAR = 0x2A36, 00063 UUID_INTERMEDIATE_TEMPERATURE_CHAR = 0x2A1E, 00064 UUID_LOCAL_TIME_INFORMATION_CHAR = 0x2A0F, 00065 UUID_MANUFACTURER_NAME_STRING_CHAR = 0x2A29, 00066 UUID_MEASUREMENT_INTERVAL_CHAR = 0x2A21, 00067 UUID_MODEL_NUMBER_STRING_CHAR = 0x2A24, 00068 UUID_UNREAD_ALERT_CHAR = 0x2A45, 00069 UUID_NEW_ALERT_CHAR = 0x2A46, 00070 UUID_PNP_ID_CHAR = 0x2A50, 00071 UUID_PRESSURE_CHAR = 0x2A6D, 00072 UUID_PROTOCOL_MODE_CHAR = 0x2A4E, 00073 UUID_RECORD_ACCESS_CONTROL_POINT_CHAR = 0x2A52, 00074 UUID_REFERENCE_TIME_INFORMATION_CHAR = 0x2A14, 00075 UUID_REPORT_CHAR = 0x2A4D, 00076 UUID_REPORT_MAP_CHAR = 0x2A4B, 00077 UUID_RINGER_CONTROL_POINT_CHAR = 0x2A40, 00078 UUID_RINGER_SETTING_CHAR = 0x2A41, 00079 UUID_SCAN_INTERVAL_WINDOW_CHAR = 0x2A4F, 00080 UUID_SCAN_REFRESH_CHAR = 0x2A31, 00081 UUID_SERIAL_NUMBER_STRING_CHAR = 0x2A25, 00082 UUID_SOFTWARE_REVISION_STRING_CHAR = 0x2A28, 00083 UUID_SUPPORTED_NEW_ALERT_CATEGORY_CHAR = 0x2A47, 00084 UUID_SUPPORTED_UNREAD_ALERT_CATEGORY_CHAR = 0x2A48, 00085 UUID_SYSTEM_ID_CHAR = 0x2A23, 00086 UUID_TEMPERATURE_CHAR = 0x2A6E, 00087 UUID_TEMPERATURE_MEASUREMENT_CHAR = 0x2A1C, 00088 UUID_TEMPERATURE_TYPE_CHAR = 0x2A1D, 00089 UUID_TIME_ACCURACY_CHAR = 0x2A12, 00090 UUID_TIME_SOURCE_CHAR = 0x2A13, 00091 UUID_TIME_UPDATE_CONTROL_POINT_CHAR = 0x2A16, 00092 UUID_TIME_UPDATE_STATE_CHAR = 0x2A17, 00093 UUID_TIME_WITH_DST_CHAR = 0x2A11, 00094 UUID_TIME_ZONE_CHAR = 0x2A0E, 00095 UUID_TX_POWER_LEVEL_CHAR = 0x2A07, 00096 UUID_CSC_FEATURE_CHAR = 0x2A5C, 00097 UUID_CSC_MEASUREMENT_CHAR = 0x2A5B, 00098 UUID_RSC_FEATURE_CHAR = 0x2A54, 00099 UUID_RSC_MEASUREMENT_CHAR = 0x2A53 00100 }; 00101 00102 /** 00103 * @brief Standard GATT characteristic presentation format unit types. 00104 * These unit types are used to describe what the raw numeric 00105 * data in a characteristic actually represents. 00106 * 00107 * @note See https://developer.bluetooth.org/gatt/units/Pages/default.aspx 00108 */ 00109 enum { 00110 BLE_GATT_UNIT_NONE = 0x2700, /**< No specified unit type. */ 00111 BLE_GATT_UNIT_LENGTH_METRE = 0x2701, /**< Length, metre. */ 00112 BLE_GATT_UNIT_MASS_KILOGRAM = 0x2702, /**< Mass, kilogram. */ 00113 BLE_GATT_UNIT_TIME_SECOND = 0x2703, /**< Time, second. */ 00114 BLE_GATT_UNIT_ELECTRIC_CURRENT_AMPERE = 0x2704, /**< Electric current, ampere. */ 00115 BLE_GATT_UNIT_THERMODYNAMIC_TEMPERATURE_KELVIN = 0x2705, /**< Thermodynamic temperature, kelvin. */ 00116 BLE_GATT_UNIT_AMOUNT_OF_SUBSTANCE_MOLE = 0x2706, /**< Amount of substance, mole. */ 00117 BLE_GATT_UNIT_LUMINOUS_INTENSITY_CANDELA = 0x2707, /**< Luminous intensity, candela. */ 00118 BLE_GATT_UNIT_AREA_SQUARE_METRES = 0x2710, /**< Area, square metres. */ 00119 BLE_GATT_UNIT_VOLUME_CUBIC_METRES = 0x2711, /**< Volume, cubic metres. */ 00120 BLE_GATT_UNIT_VELOCITY_METRES_PER_SECOND = 0x2712, /**< Velocity, metres per second. */ 00121 BLE_GATT_UNIT_ACCELERATION_METRES_PER_SECOND_SQUARED = 0x2713, /**< Acceleration, metres per second squared. */ 00122 BLE_GATT_UNIT_WAVENUMBER_RECIPROCAL_METRE = 0x2714, /**< Wave number reciprocal, metre. */ 00123 BLE_GATT_UNIT_DENSITY_KILOGRAM_PER_CUBIC_METRE = 0x2715, /**< Density, kilogram per cubic metre. */ 00124 BLE_GATT_UNIT_SURFACE_DENSITY_KILOGRAM_PER_SQUARE_METRE = 0x2716, /**< */ 00125 BLE_GATT_UNIT_SPECIFIC_VOLUME_CUBIC_METRE_PER_KILOGRAM = 0x2717, /**< */ 00126 BLE_GATT_UNIT_CURRENT_DENSITY_AMPERE_PER_SQUARE_METRE = 0x2718, /**< */ 00127 BLE_GATT_UNIT_MAGNETIC_FIELD_STRENGTH_AMPERE_PER_METRE = 0x2719, /**< Magnetic field strength, ampere per metre. */ 00128 BLE_GATT_UNIT_AMOUNT_CONCENTRATION_MOLE_PER_CUBIC_METRE = 0x271A, /**< */ 00129 BLE_GATT_UNIT_MASS_CONCENTRATION_KILOGRAM_PER_CUBIC_METRE = 0x271B, /**< */ 00130 BLE_GATT_UNIT_LUMINANCE_CANDELA_PER_SQUARE_METRE = 0x271C, /**< */ 00131 BLE_GATT_UNIT_REFRACTIVE_INDEX = 0x271D, /**< */ 00132 BLE_GATT_UNIT_RELATIVE_PERMEABILITY = 0x271E, /**< */ 00133 BLE_GATT_UNIT_PLANE_ANGLE_RADIAN = 0x2720, /**< */ 00134 BLE_GATT_UNIT_SOLID_ANGLE_STERADIAN = 0x2721, /**< */ 00135 BLE_GATT_UNIT_FREQUENCY_HERTZ = 0x2722, /**< Frequency, hertz. */ 00136 BLE_GATT_UNIT_FORCE_NEWTON = 0x2723, /**< Force, newton. */ 00137 BLE_GATT_UNIT_PRESSURE_PASCAL = 0x2724, /**< Pressure, pascal. */ 00138 BLE_GATT_UNIT_ENERGY_JOULE = 0x2725, /**< Energy, joule. */ 00139 BLE_GATT_UNIT_POWER_WATT = 0x2726, /**< Power, watt. */ 00140 BLE_GATT_UNIT_ELECTRIC_CHARGE_COULOMB = 0x2727, /**< Electrical charge, coulomb. */ 00141 BLE_GATT_UNIT_ELECTRIC_POTENTIAL_DIFFERENCE_VOLT = 0x2728, /**< Electrical potential difference, voltage. */ 00142 BLE_GATT_UNIT_CAPACITANCE_FARAD = 0x2729, /**< */ 00143 BLE_GATT_UNIT_ELECTRIC_RESISTANCE_OHM = 0x272A, /**< */ 00144 BLE_GATT_UNIT_ELECTRIC_CONDUCTANCE_SIEMENS = 0x272B, /**< */ 00145 BLE_GATT_UNIT_MAGNETIC_FLEX_WEBER = 0x272C, /**< */ 00146 BLE_GATT_UNIT_MAGNETIC_FLEX_DENSITY_TESLA = 0x272D, /**< */ 00147 BLE_GATT_UNIT_INDUCTANCE_HENRY = 0x272E, /**< */ 00148 BLE_GATT_UNIT_THERMODYNAMIC_TEMPERATURE_DEGREE_CELSIUS = 0x272F, /**< */ 00149 BLE_GATT_UNIT_LUMINOUS_FLUX_LUMEN = 0x2730, /**< */ 00150 BLE_GATT_UNIT_ILLUMINANCE_LUX = 0x2731, /**< */ 00151 BLE_GATT_UNIT_ACTIVITY_REFERRED_TO_A_RADIONUCLIDE_BECQUEREL = 0x2732, /**< */ 00152 BLE_GATT_UNIT_ABSORBED_DOSE_GRAY = 0x2733, /**< */ 00153 BLE_GATT_UNIT_DOSE_EQUIVALENT_SIEVERT = 0x2734, /**< */ 00154 BLE_GATT_UNIT_CATALYTIC_ACTIVITY_KATAL = 0x2735, /**< */ 00155 BLE_GATT_UNIT_DYNAMIC_VISCOSITY_PASCAL_SECOND = 0x2740, /**< */ 00156 BLE_GATT_UNIT_MOMENT_OF_FORCE_NEWTON_METRE = 0x2741, /**< */ 00157 BLE_GATT_UNIT_SURFACE_TENSION_NEWTON_PER_METRE = 0x2742, /**< */ 00158 BLE_GATT_UNIT_ANGULAR_VELOCITY_RADIAN_PER_SECOND = 0x2743, /**< */ 00159 BLE_GATT_UNIT_ANGULAR_ACCELERATION_RADIAN_PER_SECOND_SQUARED = 0x2744, /**< */ 00160 BLE_GATT_UNIT_HEAT_FLUX_DENSITY_WATT_PER_SQUARE_METRE = 0x2745, /**< */ 00161 BLE_GATT_UNIT_HEAT_CAPACITY_JOULE_PER_KELVIN = 0x2746, /**< */ 00162 BLE_GATT_UNIT_SPECIFIC_HEAT_CAPACITY_JOULE_PER_KILOGRAM_KELVIN = 0x2747, /**< */ 00163 BLE_GATT_UNIT_SPECIFIC_ENERGY_JOULE_PER_KILOGRAM = 0x2748, /**< */ 00164 BLE_GATT_UNIT_THERMAL_CONDUCTIVITY_WATT_PER_METRE_KELVIN = 0x2749, /**< */ 00165 BLE_GATT_UNIT_ENERGY_DENSITY_JOULE_PER_CUBIC_METRE = 0x274A, /**< */ 00166 BLE_GATT_UNIT_ELECTRIC_FIELD_STRENGTH_VOLT_PER_METRE = 0x274B, /**< */ 00167 BLE_GATT_UNIT_ELECTRIC_CHARGE_DENSITY_COULOMB_PER_CUBIC_METRE = 0x274C, /**< */ 00168 BLE_GATT_UNIT_SURFACE_CHARGE_DENSITY_COULOMB_PER_SQUARE_METRE = 0x274D, /**< */ 00169 BLE_GATT_UNIT_ELECTRIC_FLUX_DENSITY_COULOMB_PER_SQUARE_METRE = 0x274E, /**< */ 00170 BLE_GATT_UNIT_PERMITTIVITY_FARAD_PER_METRE = 0x274F, /**< */ 00171 BLE_GATT_UNIT_PERMEABILITY_HENRY_PER_METRE = 0x2750, /**< */ 00172 BLE_GATT_UNIT_MOLAR_ENERGY_JOULE_PER_MOLE = 0x2751, /**< */ 00173 BLE_GATT_UNIT_MOLAR_ENTROPY_JOULE_PER_MOLE_KELVIN = 0x2752, /**< */ 00174 BLE_GATT_UNIT_EXPOSURE_COULOMB_PER_KILOGRAM = 0x2753, /**< */ 00175 BLE_GATT_UNIT_ABSORBED_DOSE_RATE_GRAY_PER_SECOND = 0x2754, /**< */ 00176 BLE_GATT_UNIT_RADIANT_INTENSITY_WATT_PER_STERADIAN = 0x2755, /**< */ 00177 BLE_GATT_UNIT_RADIANCE_WATT_PER_SQUARE_METRE_STERADIAN = 0x2756, /**< */ 00178 BLE_GATT_UNIT_CATALYTIC_ACTIVITY_CONCENTRATION_KATAL_PER_CUBIC_METRE = 0x2757, /**< */ 00179 BLE_GATT_UNIT_TIME_MINUTE = 0x2760, /**< Time, minute. */ 00180 BLE_GATT_UNIT_TIME_HOUR = 0x2761, /**< Time, hour. */ 00181 BLE_GATT_UNIT_TIME_DAY = 0x2762, /**< Time, day. */ 00182 BLE_GATT_UNIT_PLANE_ANGLE_DEGREE = 0x2763, /**< */ 00183 BLE_GATT_UNIT_PLANE_ANGLE_MINUTE = 0x2764, /**< */ 00184 BLE_GATT_UNIT_PLANE_ANGLE_SECOND = 0x2765, /**< */ 00185 BLE_GATT_UNIT_AREA_HECTARE = 0x2766, /**< */ 00186 BLE_GATT_UNIT_VOLUME_LITRE = 0x2767, /**< */ 00187 BLE_GATT_UNIT_MASS_TONNE = 0x2768, /**< */ 00188 BLE_GATT_UNIT_PRESSURE_BAR = 0x2780, /**< Pressure, bar. */ 00189 BLE_GATT_UNIT_PRESSURE_MILLIMETRE_OF_MERCURY = 0x2781, /**< Pressure, millimetre of mercury. */ 00190 BLE_GATT_UNIT_LENGTH_ANGSTROM = 0x2782, /**< */ 00191 BLE_GATT_UNIT_LENGTH_NAUTICAL_MILE = 0x2783, /**< */ 00192 BLE_GATT_UNIT_AREA_BARN = 0x2784, /**< */ 00193 BLE_GATT_UNIT_VELOCITY_KNOT = 0x2785, /**< */ 00194 BLE_GATT_UNIT_LOGARITHMIC_RADIO_QUANTITY_NEPER = 0x2786, /**< */ 00195 BLE_GATT_UNIT_LOGARITHMIC_RADIO_QUANTITY_BEL = 0x2787, /**< */ 00196 BLE_GATT_UNIT_LENGTH_YARD = 0x27A0, /**< Length, yard. */ 00197 BLE_GATT_UNIT_LENGTH_PARSEC = 0x27A1, /**< Length, parsec. */ 00198 BLE_GATT_UNIT_LENGTH_INCH = 0x27A2, /**< Length, inch. */ 00199 BLE_GATT_UNIT_LENGTH_FOOT = 0x27A3, /**< Length, foot. */ 00200 BLE_GATT_UNIT_LENGTH_MILE = 0x27A4, /**< Length, mile. */ 00201 BLE_GATT_UNIT_PRESSURE_POUND_FORCE_PER_SQUARE_INCH = 0x27A5, /**< */ 00202 BLE_GATT_UNIT_VELOCITY_KILOMETRE_PER_HOUR = 0x27A6, /**< Velocity, kilometre per hour. */ 00203 BLE_GATT_UNIT_VELOCITY_MILE_PER_HOUR = 0x27A7, /**< Velocity, mile per hour. */ 00204 BLE_GATT_UNIT_ANGULAR_VELOCITY_REVOLUTION_PER_MINUTE = 0x27A8, /**< Angular Velocity, revolution per minute. */ 00205 BLE_GATT_UNIT_ENERGY_GRAM_CALORIE = 0x27A9, /**< Energy, gram calorie. */ 00206 BLE_GATT_UNIT_ENERGY_KILOGRAM_CALORIE = 0x27AA, /**< Energy, kilogram calorie. */ 00207 BLE_GATT_UNIT_ENERGY_KILOWATT_HOUR = 0x27AB, /**< Energy, killowatt hour. */ 00208 BLE_GATT_UNIT_THERMODYNAMIC_TEMPERATURE_DEGREE_FAHRENHEIT = 0x27AC, /**< */ 00209 BLE_GATT_UNIT_PERCENTAGE = 0x27AD, /**< Percentage. */ 00210 BLE_GATT_UNIT_PER_MILLE = 0x27AE, /**< */ 00211 BLE_GATT_UNIT_PERIOD_BEATS_PER_MINUTE = 0x27AF, /**< */ 00212 BLE_GATT_UNIT_ELECTRIC_CHARGE_AMPERE_HOURS = 0x27B0, /**< */ 00213 BLE_GATT_UNIT_MASS_DENSITY_MILLIGRAM_PER_DECILITRE = 0x27B1, /**< */ 00214 BLE_GATT_UNIT_MASS_DENSITY_MILLIMOLE_PER_LITRE = 0x27B2, /**< */ 00215 BLE_GATT_UNIT_TIME_YEAR = 0x27B3, /**< Time, year. */ 00216 BLE_GATT_UNIT_TIME_MONTH = 0x27B4, /**< Time, month. */ 00217 BLE_GATT_UNIT_CONCENTRATION_COUNT_PER_CUBIC_METRE = 0x27B5, /**< */ 00218 BLE_GATT_UNIT_IRRADIANCE_WATT_PER_SQUARE_METRE = 0x27B6 /**< */ 00219 }; 00220 00221 /** 00222 * @brief Standard GATT number types. 00223 * 00224 * @note See Bluetooth Specification 4.0 (Vol. 3), Part G, Section 3.3.3.5.2. 00225 * 00226 * @note See http://developer.bluetooth.org/gatt/descriptors/Pages/DescriptorViewer.aspx?u=org.bluetooth.descriptor.gatt.characteristic_presentation_format.xml 00227 */ 00228 enum { 00229 BLE_GATT_FORMAT_RFU = 0x00, /**< Reserved for future use. */ 00230 BLE_GATT_FORMAT_BOOLEAN = 0x01, /**< Boolean. */ 00231 BLE_GATT_FORMAT_2BIT = 0x02, /**< Unsigned 2-bit integer. */ 00232 BLE_GATT_FORMAT_NIBBLE = 0x03, /**< Unsigned 4-bit integer. */ 00233 BLE_GATT_FORMAT_UINT8 = 0x04, /**< Unsigned 8-bit integer. */ 00234 BLE_GATT_FORMAT_UINT12 = 0x05, /**< Unsigned 12-bit integer. */ 00235 BLE_GATT_FORMAT_UINT16 = 0x06, /**< Unsigned 16-bit integer. */ 00236 BLE_GATT_FORMAT_UINT24 = 0x07, /**< Unsigned 24-bit integer. */ 00237 BLE_GATT_FORMAT_UINT32 = 0x08, /**< Unsigned 32-bit integer. */ 00238 BLE_GATT_FORMAT_UINT48 = 0x09, /**< Unsigned 48-bit integer. */ 00239 BLE_GATT_FORMAT_UINT64 = 0x0A, /**< Unsigned 64-bit integer. */ 00240 BLE_GATT_FORMAT_UINT128 = 0x0B, /**< Unsigned 128-bit integer. */ 00241 BLE_GATT_FORMAT_SINT8 = 0x0C, /**< Signed 2-bit integer. */ 00242 BLE_GATT_FORMAT_SINT12 = 0x0D, /**< Signed 12-bit integer. */ 00243 BLE_GATT_FORMAT_SINT16 = 0x0E, /**< Signed 16-bit integer. */ 00244 BLE_GATT_FORMAT_SINT24 = 0x0F, /**< Signed 24-bit integer. */ 00245 BLE_GATT_FORMAT_SINT32 = 0x10, /**< Signed 32-bit integer. */ 00246 BLE_GATT_FORMAT_SINT48 = 0x11, /**< Signed 48-bit integer. */ 00247 BLE_GATT_FORMAT_SINT64 = 0x12, /**< Signed 64-bit integer. */ 00248 BLE_GATT_FORMAT_SINT128 = 0x13, /**< Signed 128-bit integer. */ 00249 BLE_GATT_FORMAT_FLOAT32 = 0x14, /**< IEEE-754 32-bit floating point. */ 00250 BLE_GATT_FORMAT_FLOAT64 = 0x15, /**< IEEE-754 64-bit floating point. */ 00251 BLE_GATT_FORMAT_SFLOAT = 0x16, /**< IEEE-11073 16-bit SFLOAT. */ 00252 BLE_GATT_FORMAT_FLOAT = 0x17, /**< IEEE-11073 32-bit FLOAT. */ 00253 BLE_GATT_FORMAT_DUINT16 = 0x18, /**< IEEE-20601 format. */ 00254 BLE_GATT_FORMAT_UTF8S = 0x19, /**< UTF-8 string. */ 00255 BLE_GATT_FORMAT_UTF16S = 0x1A, /**< UTF-16 string. */ 00256 BLE_GATT_FORMAT_STRUCT = 0x1B /**< Opaque Structure. */ 00257 }; 00258 00259 /*! 00260 * @brief Standard GATT characteristic properties. 00261 * 00262 * @note See Bluetooth Specification 4.0 (Vol. 3), Part G, Section 3.3.1.1 00263 * and Section 3.3.3.1 for Extended Properties. 00264 */ 00265 enum Properties_t { 00266 BLE_GATT_CHAR_PROPERTIES_NONE = 0x00, 00267 BLE_GATT_CHAR_PROPERTIES_BROADCAST = 0x01, /**< Permits broadcasts of the characteristic value using the Server Characteristic Configuration descriptor. */ 00268 BLE_GATT_CHAR_PROPERTIES_READ = 0x02, /**< Permits reads of the characteristic value. */ 00269 BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE = 0x04, /**< Permits writes of the characteristic value without response. */ 00270 BLE_GATT_CHAR_PROPERTIES_WRITE = 0x08, /**< Permits writes of the characteristic value with response. */ 00271 BLE_GATT_CHAR_PROPERTIES_NOTIFY = 0x10, /**< Permits notifications of a characteristic value without acknowledgment. */ 00272 BLE_GATT_CHAR_PROPERTIES_INDICATE = 0x20, /**< Permits indications of a characteristic value with acknowledgment. */ 00273 BLE_GATT_CHAR_PROPERTIES_AUTHENTICATED_SIGNED_WRITES = 0x40, /**< Permits signed writes to the characteristic value. */ 00274 BLE_GATT_CHAR_PROPERTIES_EXTENDED_PROPERTIES = 0x80 /**< Additional characteristic properties are defined in the Characteristic Extended Properties descriptor */ 00275 }; 00276 00277 /** 00278 * @brief GATT presentation format wrapper. 00279 * 00280 * @note See Bluetooth Specification 4.0 (Vol. 3), Part G, Section 3.3.3.5. 00281 * 00282 * @note See https://developer.bluetooth.org/gatt/descriptors/Pages/DescriptorViewer.aspx?u=org.bluetooth.descriptor.gatt.characteristic_presentation_format.xml 00283 */ 00284 struct PresentationFormat_t { 00285 uint8_t gatt_format; /**< Format of the value. */ 00286 int8_t exponent; /**< Exponent for integer data types. Example: if Exponent = -3 and the char value is 3892, the actual value is 3.892 */ 00287 uint16_t gatt_unit; /**< UUID from Bluetooth Assigned Numbers. */ 00288 uint8_t gatt_namespace; /**< Namespace from Bluetooth Assigned Numbers, normally '1'. */ 00289 uint16_t gatt_nsdesc; /**< Namespace description from Bluetooth Assigned Numbers, normally '0'. */ 00290 }; 00291 00292 /** 00293 * @brief Creates a new GattCharacteristic using the specified 16-bit 00294 * UUID, value length, and properties. 00295 * 00296 * @param[in] uuid 00297 * The UUID to use for this characteristic. 00298 * @param[in] valuePtr 00299 * The memory holding the initial value. The value is copied 00300 * into the stack when the enclosing service is added, and 00301 * is thereafter maintained internally by the stack. 00302 * @param[in] len 00303 * The length in bytes of this characteristic's value. 00304 * @param[in] maxLen 00305 * The max length in bytes of this characteristic's value. 00306 * @param[in] props 00307 * The 8-bit field containing the characteristic's properties. 00308 * @param[in] descriptors 00309 * A pointer to an array of descriptors to be included within 00310 * this characteristic. The memory for the descriptor array is 00311 * owned by the caller, and should remain valid at least until 00312 * the enclosing service is added to the GATT table. 00313 * @param[in] numDescriptors 00314 * The number of descriptors in the previous array. 00315 * @param[in] hasVariableLen 00316 * Whether the attribute's value length changes over time. 00317 * 00318 * @note The UUID value must be unique in the service and is normally >1. 00319 * 00320 * @note If valuePtr == NULL, length == 0, and properties == READ 00321 * for the value attribute of a characteristic, then that particular 00322 * characteristic may be considered optional and dropped while 00323 * instantiating the service with the underlying BLE stack. 00324 */ 00325 GattCharacteristic(const UUID &uuid, 00326 uint8_t *valuePtr = NULL, 00327 uint16_t len = 0, 00328 uint16_t maxLen = 0, 00329 uint8_t props = BLE_GATT_CHAR_PROPERTIES_NONE, 00330 GattAttribute *descriptors[] = NULL, 00331 unsigned numDescriptors = 0, 00332 bool hasVariableLen = true) : 00333 _valueAttribute(uuid, valuePtr, len, maxLen, hasVariableLen), 00334 _properties(props), 00335 _requiredSecurity(SecurityManager::SECURITY_MODE_ENCRYPTION_OPEN_LINK), 00336 _descriptors(descriptors), 00337 _descriptorCount(numDescriptors), 00338 enabledReadAuthorization(false), 00339 enabledWriteAuthorization(false), 00340 readAuthorizationCallback(), 00341 writeAuthorizationCallback() { 00342 /* empty */ 00343 } 00344 00345 public: 00346 /** 00347 * Set up the minimum security (mode and level) requirements for access to 00348 * the characteristic's value attribute. 00349 * 00350 * @param[in] securityMode 00351 * Can be one of encryption or signing, with or without 00352 * protection for man in the middle attacks (MITM). 00353 */ 00354 void requireSecurity(SecurityManager::SecurityMode_t securityMode) { 00355 _requiredSecurity = securityMode; 00356 } 00357 00358 public: 00359 /** 00360 * Set up callback that will be triggered before the GATT Client is allowed 00361 * to write this characteristic. The handler will determine the 00362 * authorization reply for the write. 00363 * 00364 * @param[in] callback 00365 * Event handler being registered. 00366 */ 00367 void setWriteAuthorizationCallback(void (*callback)(GattWriteAuthCallbackParams *)) { 00368 writeAuthorizationCallback.attach(callback); 00369 enabledWriteAuthorization = true; 00370 } 00371 00372 /** 00373 * Same as GattCharacrteristic::setWriteAuthorizationCallback(), but allows 00374 * the possibility to add an object reference and member function as 00375 * handler for connection event callbacks. 00376 * 00377 * @param[in] object 00378 * Pointer to the object of a class defining the member callback 00379 * function (@p member). 00380 * @param[in] member 00381 * The member callback (within the context of an object) to be 00382 * invoked. 00383 */ 00384 template <typename T> 00385 void setWriteAuthorizationCallback(T *object, void (T::*member)(GattWriteAuthCallbackParams *)) { 00386 writeAuthorizationCallback.attach(object, member); 00387 enabledWriteAuthorization = true; 00388 } 00389 00390 /** 00391 * Set up callback that will be triggered before the GATT Client is allowed 00392 * to read this characteristic. The handler will determine the 00393 * authorizaion reply for the read. 00394 * 00395 * @param[in] callback 00396 * Event handler being registered. 00397 */ 00398 void setReadAuthorizationCallback(void (*callback)(GattReadAuthCallbackParams *)) { 00399 readAuthorizationCallback.attach(callback); 00400 enabledReadAuthorization = true; 00401 } 00402 00403 /** 00404 * Same as GattCharacrteristic::setReadAuthorizationCallback(), but allows 00405 * the possibility to add an object reference and member function as 00406 * handler for connection event callbacks. 00407 * 00408 * @param[in] object 00409 * Pointer to the object of a class defining the member callback 00410 * function (@p member). 00411 * @param[in] member 00412 * The member callback (within the context of an object) to be 00413 * invoked. 00414 */ 00415 template <typename T> 00416 void setReadAuthorizationCallback(T *object, void (T::*member)(GattReadAuthCallbackParams *)) { 00417 readAuthorizationCallback.attach(object, member); 00418 enabledReadAuthorization = true; 00419 } 00420 00421 /** 00422 * Helper that calls the registered handler to determine the authorization 00423 * reply for a write request. This function is meant to be called from the 00424 * BLE stack specific implementation. 00425 * 00426 * @param[in] params 00427 * To capture the context of the write-auth request. Also 00428 * contains an out-parameter for reply. 00429 * 00430 * @return A GattAuthCallbackReply_t value indicating whether authorization 00431 * is granted. 00432 */ 00433 GattAuthCallbackReply_t authorizeWrite(GattWriteAuthCallbackParams *params) { 00434 if (!isWriteAuthorizationEnabled()) { 00435 return AUTH_CALLBACK_REPLY_SUCCESS; 00436 } 00437 00438 params->authorizationReply = AUTH_CALLBACK_REPLY_SUCCESS; /* Initialized to no-error by default. */ 00439 writeAuthorizationCallback.call(params); 00440 return params->authorizationReply; 00441 } 00442 00443 /** 00444 * Helper that calls the registered handler to determine the authorization 00445 * reply for a read request. This function is meant to be called from the 00446 * BLE stack specific implementation. 00447 * 00448 * @param[in] params 00449 * To capture the context of the read-auth request. 00450 * 00451 * @return A GattAuthCallbackReply_t value indicating whether authorization 00452 * is granted. 00453 * 00454 * @note To authorize or deny the read the params->authorizationReply field 00455 * should be set to true (authorize) or false (deny). 00456 * 00457 * @note If the read is approved and params->data is unchanged (NULL), 00458 * the current characteristic value will be used. 00459 * 00460 * @note If the read is approved, a new value can be provided by setting 00461 * the params->data pointer and params->len fields. 00462 */ 00463 GattAuthCallbackReply_t authorizeRead(GattReadAuthCallbackParams *params) { 00464 if (!isReadAuthorizationEnabled()) { 00465 return AUTH_CALLBACK_REPLY_SUCCESS; 00466 } 00467 00468 params->authorizationReply = AUTH_CALLBACK_REPLY_SUCCESS; /* Initialized to no-error by default. */ 00469 readAuthorizationCallback.call(params); 00470 return params->authorizationReply; 00471 } 00472 00473 public: 00474 /** 00475 * Get the characteristic's value attribute. 00476 * 00477 * @return A reference to the characteristic's value attribute. 00478 */ 00479 GattAttribute& getValueAttribute() { 00480 return _valueAttribute; 00481 } 00482 00483 /** 00484 * A const alternative to GattCharacteristic::getValueAttribute(). 00485 * 00486 * @return A const reference to the characteristic's value attribute. 00487 */ 00488 const GattAttribute& getValueAttribute() const { 00489 return _valueAttribute; 00490 } 00491 00492 /** 00493 * Get the characteristic's value attribute handle in the ATT table. 00494 * 00495 * @return The value attribute handle. 00496 * 00497 * @note The attribute handle is typically assigned by the underlying BLE 00498 * stack. 00499 */ 00500 GattAttribute::Handle_t getValueHandle(void) const { 00501 return getValueAttribute().getHandle(); 00502 } 00503 00504 /** 00505 * Get the characteristic's propertied. Refer to 00506 * GattCharacteristic::Properties_t. 00507 * 00508 * @return The characteristic's properties. 00509 */ 00510 uint8_t getProperties(void) const { 00511 return _properties; 00512 } 00513 00514 /** 00515 * Get the characteristic's required security. 00516 * 00517 * @return The characteristic's required security. 00518 */ 00519 SecurityManager::SecurityMode_t getRequiredSecurity() const { 00520 return _requiredSecurity; 00521 } 00522 00523 /** 00524 * Get the total number of descriptors within this characteristic. 00525 * 00526 * @return The total number of descriptors. 00527 */ 00528 uint8_t getDescriptorCount(void) const { 00529 return _descriptorCount; 00530 } 00531 00532 /** 00533 * Check whether read authorization is enabled i.e. check whether a 00534 * read authorization callback was previously registered. Refer to 00535 * GattCharacteristic::setReadAuthorizationCallback(). 00536 * 00537 * @return true if read authorization is enabled, false otherwise. 00538 */ 00539 bool isReadAuthorizationEnabled() const { 00540 return enabledReadAuthorization; 00541 } 00542 00543 /** 00544 * Check whether write authorization is enabled i.e. check whether a 00545 * write authorization callback was previously registered. Refer to 00546 * GattCharacteristic::setReadAuthorizationCallback(). 00547 * 00548 * @return true if write authorization is enabled, false otherwise. 00549 */ 00550 bool isWriteAuthorizationEnabled() const { 00551 return enabledWriteAuthorization; 00552 } 00553 00554 /** 00555 * Get this characteristic's descriptor at a specific index. 00556 * 00557 * @param[in] index 00558 * The descriptor's index. 00559 * 00560 * @return A pointer the requested descriptor if @p index contains a valid 00561 * descriptor, or NULL otherwise. 00562 */ 00563 GattAttribute *getDescriptor(uint8_t index) { 00564 if (index >= _descriptorCount) { 00565 return NULL; 00566 } 00567 00568 return _descriptors[index]; 00569 } 00570 00571 private: 00572 /** 00573 * Attribute that contains the actual value of this characteristic. 00574 */ 00575 GattAttribute _valueAttribute; 00576 /** 00577 * The characteristic's properties. Refer to 00578 * GattCharacteristic::Properties_t. 00579 */ 00580 uint8_t _properties; 00581 /** 00582 * The characteristic's required security. 00583 */ 00584 SecurityManager::SecurityMode_t _requiredSecurity; 00585 /** 00586 * The characteristic's descriptor attributes. 00587 */ 00588 GattAttribute **_descriptors; 00589 /** 00590 * The number of descriptors in this characteristic. 00591 */ 00592 uint8_t _descriptorCount; 00593 00594 /** 00595 * Whether read authorization is enabled i.e. whether there is a registered 00596 * callback to determine read authorization reply. 00597 */ 00598 bool enabledReadAuthorization; 00599 /** 00600 * Whether write authorization is enabled i.e. whether there is a registered 00601 * callback to determine write authorization reply. 00602 */ 00603 bool enabledWriteAuthorization; 00604 /** 00605 * The registered callback handler for read authorization reply. 00606 */ 00607 FunctionPointerWithContext<GattReadAuthCallbackParams *> readAuthorizationCallback; 00608 /** 00609 * The registered callback handler for write authorization reply. 00610 */ 00611 FunctionPointerWithContext<GattWriteAuthCallbackParams *> writeAuthorizationCallback; 00612 00613 private: 00614 /* Disallow copy and assignment. */ 00615 GattCharacteristic(const GattCharacteristic &); 00616 GattCharacteristic& operator=(const GattCharacteristic &); 00617 }; 00618 00619 /** 00620 * Helper class to construct a read-only GattCharacteristic. 00621 */ 00622 template <typename T> 00623 class ReadOnlyGattCharacteristic : public GattCharacteristic { 00624 public: 00625 /** 00626 * Construct a ReadOnlyGattCharacteristic. 00627 * 00628 * @param[in] uuid 00629 * The characteristic's UUID. 00630 * @param[in] valuePtr 00631 * Pointer to the characterisitic's initial value. 00632 * @param[in] additionalProperties 00633 * Additional characterisitic properties. By default, the 00634 * properties are set to 00635 * Properties_t::BLE_GATT_CHAR_PROPERTIES_READ. 00636 * @param[in] descriptors 00637 * An array of pointers to descriptors to be added to the new 00638 * characteristic. 00639 * @param[in] numDescriptors 00640 * The total number of descriptors in @p descriptors. 00641 * 00642 * @note Instances of ReadOnlyGattCharacteristic have a fixed length 00643 * attribute value that equals sizeof(T). For a variable length 00644 * alternative use GattCharacteristic directly. 00645 */ 00646 ReadOnlyGattCharacteristic<T>(const UUID &uuid, 00647 T *valuePtr, 00648 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 00649 GattAttribute *descriptors[] = NULL, 00650 unsigned numDescriptors = 0) : 00651 GattCharacteristic(uuid, reinterpret_cast<uint8_t *>(valuePtr), sizeof(T), sizeof(T), 00652 BLE_GATT_CHAR_PROPERTIES_READ | additionalProperties, descriptors, numDescriptors, false) { 00653 /* empty */ 00654 } 00655 }; 00656 00657 /** 00658 * Helper class to construct a write-only GattCharacteristic. 00659 */ 00660 template <typename T> 00661 class WriteOnlyGattCharacteristic : public GattCharacteristic { 00662 public: 00663 /** 00664 * Construct a WriteOnlyGattCharacteristic. 00665 * 00666 * @param[in] uuid 00667 * The characteristic's UUID. 00668 * @param[in] valuePtr 00669 * Pointer to the characterisitic's initial value. 00670 * @param[in] additionalProperties 00671 * Additional characterisitic properties. By default, the 00672 * properties are set to 00673 * Properties_t::BLE_GATT_CHAR_PROPERTIES_WRITE. 00674 * @param[in] descriptors 00675 * An array of pointers to descriptors to be added to the new 00676 * characteristic. 00677 * @param[in] numDescriptors 00678 * The total number of descriptors in @p descriptors. 00679 * 00680 * @note Instances of WriteOnlyGattCharacteristic have variable length 00681 * attribute value with maximum size equal to sizeof(T). For a fixed length 00682 * alternative use GattCharacteristic directly. 00683 */ 00684 WriteOnlyGattCharacteristic<T>(const UUID &uuid, 00685 T *valuePtr, 00686 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 00687 GattAttribute *descriptors[] = NULL, 00688 unsigned numDescriptors = 0) : 00689 GattCharacteristic(uuid, reinterpret_cast<uint8_t *>(valuePtr), sizeof(T), sizeof(T), 00690 BLE_GATT_CHAR_PROPERTIES_WRITE | additionalProperties, descriptors, numDescriptors) { 00691 /* empty */ 00692 } 00693 }; 00694 00695 /** 00696 * Helper class to construct a readable and writable GattCharacteristic. 00697 */ 00698 template <typename T> 00699 class ReadWriteGattCharacteristic : public GattCharacteristic { 00700 public: 00701 /** 00702 * Construct a ReadWriteGattCharacteristic. 00703 * 00704 * @param[in] uuid 00705 * The characteristic's UUID. 00706 * @param[in] valuePtr 00707 * Pointer to the characterisitic's initial value. 00708 * @param[in] additionalProperties 00709 * Additional characterisitic properties. By default, the 00710 * properties are set to 00711 * Properties_t::BLE_GATT_CHAR_PROPERTIES_WRITE | 00712 * Properties_t::BLE_GATT_CHAR_PROPERTIES_READ. 00713 * @param[in] descriptors 00714 * An array of pointers to descriptors to be added to the new 00715 * characteristic. 00716 * @param[in] numDescriptors 00717 * The total number of descriptors in @p descriptors. 00718 * 00719 * @note Instances of ReadWriteGattCharacteristic have variable length 00720 * attribute value with maximum size equal to sizeof(T). For a fixed length 00721 * alternative use GattCharacteristic directly. 00722 */ 00723 ReadWriteGattCharacteristic<T>(const UUID &uuid, 00724 T *valuePtr, 00725 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 00726 GattAttribute *descriptors[] = NULL, 00727 unsigned numDescriptors = 0) : 00728 GattCharacteristic(uuid, reinterpret_cast<uint8_t *>(valuePtr), sizeof(T), sizeof(T), 00729 BLE_GATT_CHAR_PROPERTIES_READ | BLE_GATT_CHAR_PROPERTIES_WRITE | additionalProperties, descriptors, numDescriptors) { 00730 /* empty */ 00731 } 00732 }; 00733 00734 /** 00735 * Helper class to construct a write-only GattCharacteristic with an array 00736 * value. 00737 */ 00738 template <typename T, unsigned NUM_ELEMENTS> 00739 class WriteOnlyArrayGattCharacteristic : public GattCharacteristic { 00740 public: 00741 /** 00742 * Construct a WriteOnlyGattCharacteristic. 00743 * 00744 * @param[in] uuid 00745 * The characteristic's UUID. 00746 * @param[in] valuePtr 00747 * Pointer to an array of length NUM_ELEMENTS containing the 00748 * characteristic's intitial value. 00749 * @param[in] additionalProperties 00750 * Additional characterisitic properties. By default, the 00751 * properties are set to 00752 * Properties_t::BLE_GATT_CHAR_PROPERTIES_WRITE. 00753 * @param[in] descriptors 00754 * An array of pointers to descriptors to be added to the new 00755 * characteristic. 00756 * @param[in] numDescriptors 00757 * The total number of descriptors in @p descriptors. 00758 * 00759 * @note Instances of WriteOnlyGattCharacteristic have variable length 00760 * attribute value with maximum size equal to sizeof(T) * NUM_ELEMENTS. 00761 * For a fixed length alternative use GattCharacteristic directly. 00762 */ 00763 WriteOnlyArrayGattCharacteristic<T, NUM_ELEMENTS>(const UUID &uuid, 00764 T valuePtr[NUM_ELEMENTS], 00765 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 00766 GattAttribute *descriptors[] = NULL, 00767 unsigned numDescriptors = 0) : 00768 GattCharacteristic(uuid, reinterpret_cast<uint8_t *>(valuePtr), sizeof(T) * NUM_ELEMENTS, sizeof(T) * NUM_ELEMENTS, 00769 BLE_GATT_CHAR_PROPERTIES_WRITE | additionalProperties, descriptors, numDescriptors) { 00770 /* empty */ 00771 } 00772 }; 00773 00774 /** 00775 * Helper class to construct a read-only GattCharacteristic with an array 00776 * value. 00777 */ 00778 template <typename T, unsigned NUM_ELEMENTS> 00779 class ReadOnlyArrayGattCharacteristic : public GattCharacteristic { 00780 public: 00781 /** 00782 * Construct a ReadOnlyGattCharacteristic. 00783 * 00784 * @param[in] uuid 00785 * The characteristic's UUID. 00786 * @param[in] valuePtr 00787 * Pointer to an array of length NUM_ELEMENTS containing the 00788 * characteristic's intitial value. 00789 * @param[in] additionalProperties 00790 * Additional characterisitic properties. By default, the 00791 * properties are set to 00792 * Properties_t::BLE_GATT_CHAR_PROPERTIES_READ. 00793 * @param[in] descriptors 00794 * An array of pointers to descriptors to be added to the new 00795 * characteristic. 00796 * @param[in] numDescriptors 00797 * The total number of descriptors in @p descriptors. 00798 * 00799 * @note Instances of ReadOnlyGattCharacteristic have fixed length 00800 * attribute value that equals sizeof(T) * NUM_ELEMENTS. 00801 * For a variable length alternative use GattCharacteristic directly. 00802 */ 00803 ReadOnlyArrayGattCharacteristic<T, NUM_ELEMENTS>(const UUID &uuid, 00804 T valuePtr[NUM_ELEMENTS], 00805 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 00806 GattAttribute *descriptors[] = NULL, 00807 unsigned numDescriptors = 0) : 00808 GattCharacteristic(uuid, reinterpret_cast<uint8_t *>(valuePtr), sizeof(T) * NUM_ELEMENTS, sizeof(T) * NUM_ELEMENTS, 00809 BLE_GATT_CHAR_PROPERTIES_READ | additionalProperties, descriptors, numDescriptors, false) { 00810 /* empty */ 00811 } 00812 }; 00813 00814 /** 00815 * Helper class to construct a readable and writable GattCharacteristic with an array 00816 * value. 00817 */ 00818 template <typename T, unsigned NUM_ELEMENTS> 00819 class ReadWriteArrayGattCharacteristic : public GattCharacteristic { 00820 public: 00821 /** 00822 * Construct a ReadWriteGattCharacteristic. 00823 * 00824 * @param[in] uuid 00825 * The characteristic's UUID. 00826 * @param[in] valuePtr 00827 * Pointer to an array of length NUM_ELEMENTS containing the 00828 * characteristic's intitial value. 00829 * @param[in] additionalProperties 00830 * Additional characterisitic properties. By default, the 00831 * properties are set to 00832 * Properties_t::BLE_GATT_CHAR_PROPERTIES_WRITE | 00833 * Properties_t::BLE_GATT_CHAR_PROPERTIES_READ. 00834 * @param[in] descriptors 00835 * An array of pointers to descriptors to be added to the new 00836 * characteristic. 00837 * @param[in] numDescriptors 00838 * The total number of descriptors in @p descriptors. 00839 * 00840 * @note Instances of ReadWriteGattCharacteristic have variable length 00841 * attribute value with maximum size equal to sizeof(T) * NUM_ELEMENTS. 00842 * For a fixed length alternative use GattCharacteristic directly. 00843 */ 00844 ReadWriteArrayGattCharacteristic<T, NUM_ELEMENTS>(const UUID &uuid, 00845 T valuePtr[NUM_ELEMENTS], 00846 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 00847 GattAttribute *descriptors[] = NULL, 00848 unsigned numDescriptors = 0) : 00849 GattCharacteristic(uuid, reinterpret_cast<uint8_t *>(valuePtr), sizeof(T) * NUM_ELEMENTS, sizeof(T) * NUM_ELEMENTS, 00850 BLE_GATT_CHAR_PROPERTIES_READ | BLE_GATT_CHAR_PROPERTIES_WRITE | additionalProperties, descriptors, numDescriptors) { 00851 /* empty */ 00852 } 00853 }; 00854 00855 #endif /* ifndef __GATT_CHARACTERISTIC_H__ */
Generated on Wed Jul 13 2022 09:31:10 by
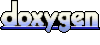