adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
GattAttribute.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_ATTRIBUTE_H__ 00018 #define __GATT_ATTRIBUTE_H__ 00019 00020 #include "UUID.h" 00021 00022 /** 00023 * Instances of this class encapsulate the data that belongs to a Bluetooth Low 00024 * Energy attribute. 00025 */ 00026 class GattAttribute { 00027 public: 00028 /** 00029 * Type for the handle or ID of the attribute in the ATT table. These are 00030 * unique and are usually generated by the underlying BLE stack. 00031 */ 00032 typedef uint16_t Handle_t; 00033 /** 00034 * Define the value of an invalid attribute handle. 00035 */ 00036 static const Handle_t INVALID_HANDLE = 0x0000; 00037 00038 public: 00039 /** 00040 * @brief Creates a new GattAttribute using the specified 00041 * UUID, value length, and inital value. 00042 * 00043 * @param[in] uuid 00044 * The UUID to use for this attribute. 00045 * @param[in] valuePtr 00046 * The memory holding the initial value. 00047 * @param[in] len 00048 * The length in bytes of this attribute's value. 00049 * @param[in] maxLen 00050 * The max length in bytes of this attribute's value. 00051 * @param[in] hasVariableLen 00052 * Whether the attribute's value length changes overtime. 00053 * 00054 * @section EXAMPLE 00055 * 00056 * @code 00057 * 00058 * // UUID = 0x2A19, Min length 2, Max len = 2 00059 * GattAttribute attr = GattAttribute(0x2A19, &someValue, 2, 2); 00060 * 00061 * @endcode 00062 */ 00063 GattAttribute(const UUID &uuid, uint8_t *valuePtr = NULL, uint16_t len = 0, uint16_t maxLen = 0, bool hasVariableLen = true) : 00064 _uuid(uuid), _valuePtr(valuePtr), _lenMax(maxLen), _len(len), _hasVariableLen(hasVariableLen), _handle() { 00065 /* Empty */ 00066 } 00067 00068 public: 00069 /** 00070 * Get the attribute's handle in the ATT table. 00071 * 00072 * @return The attribute's handle. 00073 */ 00074 Handle_t getHandle(void) const { 00075 return _handle; 00076 } 00077 00078 /** 00079 * The UUID of the characteristic that this attribute belongs to. 00080 * 00081 * @return The characteristic's UUID. 00082 */ 00083 const UUID &getUUID(void) const { 00084 return _uuid; 00085 } 00086 00087 /** 00088 * Get the current length of the attribute value. 00089 * 00090 * @return The current length of the attribute value. 00091 */ 00092 uint16_t getLength(void) const { 00093 return _len; 00094 } 00095 00096 /** 00097 * Get the maximum length of the attribute value. 00098 * 00099 * The maximum length of the attribute value. 00100 */ 00101 uint16_t getMaxLength(void) const { 00102 return _lenMax; 00103 } 00104 00105 /** 00106 * Get a pointer to the current length of the attribute value. 00107 * 00108 * @return A pointer to the current length of the attribute value. 00109 */ 00110 uint16_t *getLengthPtr(void) { 00111 return &_len; 00112 } 00113 00114 /** 00115 * Set the attribute handle. 00116 * 00117 * @param[in] id 00118 * The new attribute handle. 00119 */ 00120 void setHandle(Handle_t id) { 00121 _handle = id; 00122 } 00123 00124 /** 00125 * Get a pointer to the attribute value. 00126 * 00127 * @return A pointer to the attribute value. 00128 */ 00129 uint8_t *getValuePtr(void) { 00130 return _valuePtr; 00131 } 00132 00133 /** 00134 * Check whether the length of the attribute's value can change over time. 00135 * 00136 * @return true if the attribute has variable length, false otherwise. 00137 */ 00138 bool hasVariableLength(void) const { 00139 return _hasVariableLen; 00140 } 00141 00142 private: 00143 /** 00144 * Characteristic's UUID. 00145 */ 00146 UUID _uuid; 00147 /** 00148 * Pointer to the attribute's value. 00149 */ 00150 uint8_t *_valuePtr; 00151 /** 00152 * Maximum length of the value pointed to by GattAttribute::_valuePtr. 00153 */ 00154 uint16_t _lenMax; 00155 /** 00156 * Current length of the value pointed to by GattAttribute::_valuePtr. 00157 */ 00158 uint16_t _len; 00159 /** 00160 * Whether the length of the value can change over time. 00161 */ 00162 bool _hasVariableLen; 00163 /** 00164 * The attribute's handle in the ATT table. 00165 */ 00166 Handle_t _handle; 00167 00168 private: 00169 /* Disallow copy and assignment. */ 00170 GattAttribute(const GattAttribute &); 00171 GattAttribute& operator=(const GattAttribute &); 00172 }; 00173 00174 #endif /* ifndef __GATT_ATTRIBUTE_H__ */
Generated on Wed Jul 13 2022 09:31:10 by
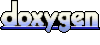