adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
EnvironmentalService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __BLE_ENVIRONMENTAL_SERVICE_H__ 00018 #define __BLE_ENVIRONMENTAL_SERVICE_H__ 00019 00020 #include "ble/BLE.h" 00021 00022 /** 00023 * @class EnvironmentalService 00024 * @brief BLE Environmental Service. This service provides temperature, humidity and pressure measurement. 00025 * Service: https://developer.bluetooth.org/gatt/services/Pages/ServiceViewer.aspx?u=org.bluetooth.service.environmental_sensing.xml 00026 * Temperature: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.temperature.xml 00027 * Humidity: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.humidity.xml 00028 * Pressure: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.pressure.xml 00029 */ 00030 class EnvironmentalService { 00031 public: 00032 typedef int16_t TemperatureType_t; 00033 typedef uint16_t HumidityType_t; 00034 typedef uint32_t PressureType_t; 00035 00036 /** 00037 * @brief EnvironmentalService constructor. 00038 * @param ble Reference to BLE device. 00039 * @param temperature_en Enable this characteristic. 00040 * @param humidity_en Enable this characteristic. 00041 * @param pressure_en Enable this characteristic. 00042 */ 00043 EnvironmentalService(BLE& _ble) : 00044 ble(_ble), 00045 temperatureCharacteristic(GattCharacteristic::UUID_TEMPERATURE_CHAR, &temperature), 00046 humidityCharacteristic(GattCharacteristic::UUID_HUMIDITY_CHAR, &humidity), 00047 pressureCharacteristic(GattCharacteristic::UUID_PRESSURE_CHAR, &pressure) 00048 { 00049 static bool serviceAdded = false; /* We should only ever need to add the information service once. */ 00050 if (serviceAdded) { 00051 return; 00052 } 00053 00054 GattCharacteristic *charTable[] = { &humidityCharacteristic, 00055 &pressureCharacteristic, 00056 &temperatureCharacteristic }; 00057 00058 GattService environmentalService(GattService::UUID_ENVIRONMENTAL_SERVICE, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00059 00060 ble.gattServer().addService(environmentalService); 00061 serviceAdded = true; 00062 } 00063 00064 /** 00065 * @brief Update humidity characteristic. 00066 * @param newHumidityVal New humidity measurement. 00067 */ 00068 void updateHumidity(HumidityType_t newHumidityVal) 00069 { 00070 humidity = (HumidityType_t) (newHumidityVal * 100); 00071 ble.gattServer().write(humidityCharacteristic.getValueHandle(), (uint8_t *) &humidity, sizeof(HumidityType_t)); 00072 } 00073 00074 /** 00075 * @brief Update pressure characteristic. 00076 * @param newPressureVal New pressure measurement. 00077 */ 00078 void updatePressure(PressureType_t newPressureVal) 00079 { 00080 pressure = (PressureType_t) (newPressureVal * 10); 00081 ble.gattServer().write(pressureCharacteristic.getValueHandle(), (uint8_t *) &pressure, sizeof(PressureType_t)); 00082 } 00083 00084 /** 00085 * @brief Update temperature characteristic. 00086 * @param newTemperatureVal New temperature measurement. 00087 */ 00088 void updateTemperature(float newTemperatureVal) 00089 { 00090 temperature = (TemperatureType_t) (newTemperatureVal * 100); 00091 ble.gattServer().write(temperatureCharacteristic.getValueHandle(), (uint8_t *) &temperature, sizeof(TemperatureType_t)); 00092 } 00093 00094 private: 00095 BLE& ble; 00096 00097 TemperatureType_t temperature; 00098 HumidityType_t humidity; 00099 PressureType_t pressure; 00100 00101 ReadOnlyGattCharacteristic<TemperatureType_t> temperatureCharacteristic; 00102 ReadOnlyGattCharacteristic<HumidityType_t> humidityCharacteristic; 00103 ReadOnlyGattCharacteristic<PressureType_t> pressureCharacteristic; 00104 }; 00105 00106 #endif /* #ifndef __BLE_ENVIRONMENTAL_SERVICE_H__*/
Generated on Wed Jul 13 2022 09:31:10 by
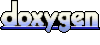