adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
DiscoveredCharacteristic.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __DISCOVERED_CHARACTERISTIC_H__ 00018 #define __DISCOVERED_CHARACTERISTIC_H__ 00019 00020 #include "UUID.h" 00021 #include "Gap.h" 00022 #include "GattAttribute.h" 00023 #include "GattClient.h" 00024 #include "CharacteristicDescriptorDiscovery.h" 00025 #include "ble/DiscoveredCharacteristicDescriptor.h" 00026 00027 /** 00028 * @brief Representation of a characteristic discovered during a GattClient 00029 * discovery procedure (see GattClient::launchServiceDiscovery ). 00030 * 00031 * @details Provide detailed informations about a discovered characteristic like: 00032 * - Its UUID (see getUUID()). 00033 * - The most important handles of the characteristic definition 00034 * (see getDeclHandle(), getValueHandle(), getLastHandle()) 00035 * - Its properties (see getProperties()). 00036 * This class also provide functions to operate on the characteristic: 00037 * - Read the characteristic value (see read()) 00038 * - Writing a characteristic value (see write() or writeWoResponse()) 00039 * - Discover descriptors inside the characteristic definition. These descriptors 00040 * extends the characteristic. More information about descriptor usage is 00041 * available in DiscoveredCharacteristicDescriptor class. 00042 */ 00043 class DiscoveredCharacteristic { 00044 public: 00045 /** 00046 * Structure that encapsulates the properties of a discovered 00047 * characteristic. 00048 */ 00049 struct Properties_t { 00050 uint8_t _broadcast :1; /**< Broadcasting the value permitted. */ 00051 uint8_t _read :1; /**< Reading the value permitted. */ 00052 uint8_t _writeWoResp :1; /**< Writing the value with Write Command permitted. */ 00053 uint8_t _write :1; /**< Writing the value with Write Request permitted. */ 00054 uint8_t _notify :1; /**< Notifications of the value permitted. */ 00055 uint8_t _indicate :1; /**< Indications of the value permitted. */ 00056 uint8_t _authSignedWrite :1; /**< Writing the value with Signed Write Command permitted. */ 00057 00058 public: 00059 /** 00060 * @brief Check if broadcasting is permitted. 00061 * 00062 * @return true if broadcasting the value is permitted, and false 00063 * otherwise. 00064 */ 00065 bool broadcast(void) const { 00066 return _broadcast; 00067 } 00068 00069 /** 00070 * @brief Check reading is permitted. 00071 * 00072 * @return true if reading the value is permitted, and false 00073 * otherwise. 00074 */ 00075 bool read(void) const { 00076 return _read; 00077 } 00078 00079 /** 00080 * @brief Check if writing with Write Command is permitted. 00081 * 00082 * @return true if writing the value with Write Command is permitted, 00083 * false otherwise. 00084 */ 00085 bool writeWoResp(void) const { 00086 return _writeWoResp; 00087 } 00088 00089 /** 00090 * @brief Check if writing with Write Request is permitted. 00091 * 00092 * @return true if writing the value with Write Request is permitted, 00093 * false otherwise. 00094 */ 00095 bool write(void) const { 00096 return _write; 00097 } 00098 00099 /** 00100 * @brief Check notifications are permitted. 00101 * 00102 * @return true if notifications of the value are permitted, false 00103 * otherwise. 00104 */ 00105 bool notify(void) const { 00106 return _notify; 00107 } 00108 00109 /** 00110 * @brief Check if indications are permitted. 00111 * 00112 * @return true if indications of the value are permitted, false 00113 * otherwise. 00114 */ 00115 bool indicate(void) const { 00116 return _indicate; 00117 } 00118 00119 /** 00120 * @brief Check if writing with Signed Write Command is permitted. 00121 * 00122 * @return true if writing the value with Signed Write Command is 00123 * permitted, false otherwise. 00124 */ 00125 bool authSignedWrite(void) const { 00126 return _authSignedWrite; 00127 } 00128 00129 /** 00130 * @brief "Equal to" operator for DiscoveredCharacteristic::Properties_t 00131 * 00132 * @param[in] lhs The left hand side of the equality expression 00133 * @param[in] rhs The right hand side of the equality expression 00134 * 00135 * @return true if operands are equals, false otherwise. 00136 */ 00137 friend bool operator==(Properties_t lhs, Properties_t rhs) { 00138 return lhs._broadcast == rhs._broadcast && 00139 lhs._read == rhs._read && 00140 lhs._writeWoResp == rhs._writeWoResp && 00141 lhs._write == rhs._write && 00142 lhs._notify == rhs._notify && 00143 lhs._indicate == rhs._indicate && 00144 lhs._authSignedWrite == rhs._authSignedWrite; 00145 } 00146 00147 /** 00148 * @brief "Not equal to" operator for DiscoveredCharacteristic::Properties_t 00149 * 00150 * @param lhs The right hand side of the expression 00151 * @param rhs The left hand side of the expression 00152 * 00153 * @return true if operands are not equals, false otherwise. 00154 */ 00155 friend bool operator!=(Properties_t lhs, Properties_t rhs) { 00156 return !(lhs == rhs); 00157 } 00158 00159 private: 00160 operator uint8_t() const; /* Disallow implicit conversion into an integer. */ 00161 operator unsigned() const; /* Disallow implicit conversion into an integer. */ 00162 }; 00163 00164 /** 00165 * Initiate (or continue) a read for the value attribute, optionally at a 00166 * given offset. If the characteristic or descriptor to be read is longer 00167 * than ATT_MTU - 1, this function must be called multiple times with 00168 * appropriate offset to read the complete value. 00169 * 00170 * @param[in] offset 00171 * The position - in the characteristic value bytes stream - where 00172 * the read operation begin. 00173 * 00174 * @return BLE_ERROR_NONE if a read has been initiated, or 00175 * BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or 00176 * BLE_STACK_BUSY if some client procedure is already in progress, or 00177 * BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties. 00178 */ 00179 ble_error_t read(uint16_t offset = 0) const; 00180 00181 /** 00182 * @brief Same as #read(uint16_t) const but allow the user to register a callback 00183 * which will be fired once the read is done. 00184 * 00185 * @param[in] offset 00186 * The position - in the characteristic value bytes stream - where 00187 * the read operation begin. 00188 * @param[in] onRead 00189 * Continuation of the read operation 00190 */ 00191 ble_error_t read(uint16_t offset, const GattClient::ReadCallback_t & onRead) const; 00192 00193 /** 00194 * Perform a write without response procedure. 00195 * 00196 * @param[in] length 00197 * The amount of data being written. 00198 * @param[in] value 00199 * The bytes being written. 00200 * 00201 * @note It is important to note that a write without response will generate 00202 * an onDataSent() callback when the packet has been transmitted. There 00203 * will be a BLE-stack specific limit to the number of pending 00204 * writeWoResponse operations; the user may want to use the onDataSent() 00205 * callback for flow-control. 00206 * 00207 * @retval BLE_ERROR_NONE Successfully started the Write procedure, or 00208 * BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or 00209 * BLE_STACK_BUSY if some client procedure is already in progress, or 00210 * BLE_ERROR_NO_MEM if there are no available buffers left to process the request, or 00211 * BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties. 00212 */ 00213 ble_error_t writeWoResponse(uint16_t length, const uint8_t *value) const; 00214 00215 /** 00216 * Initiate a GATT Characteristic Descriptor Discovery procedure for descriptors within this characteristic. 00217 * 00218 * @param[in] onDescriptorDiscovered This callback will be called every time a descriptor is discovered 00219 * @param[in] onTermination This callback will be called when the discovery process is over. 00220 * 00221 * @return BLE_ERROR_NONE if descriptor discovery is launched successfully; else an appropriate error. 00222 */ 00223 ble_error_t discoverDescriptors(const CharacteristicDescriptorDiscovery::DiscoveryCallback_t& onDescriptorDiscovered, 00224 const CharacteristicDescriptorDiscovery::TerminationCallback_t& onTermination) const; 00225 00226 /** 00227 * Perform a write procedure. 00228 * 00229 * @param[in] length 00230 * The amount of data being written. 00231 * @param[in] value 00232 * The bytes being written. 00233 * 00234 * @note It is important to note that a write will generate 00235 * an onDataWritten() callback when the peer acknowledges the request. 00236 * 00237 * @retval BLE_ERROR_NONE Successfully started the Write procedure, or 00238 * BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or 00239 * BLE_STACK_BUSY if some client procedure is already in progress, or 00240 * BLE_ERROR_NO_MEM if there are no available buffers left to process the request, or 00241 * BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties. 00242 */ 00243 ble_error_t write(uint16_t length, const uint8_t *value) const; 00244 00245 /** 00246 * Same as write(uint16_t, const uint8_t *) const but register a callback 00247 * which will be called once the data has been written. 00248 * 00249 * @param[in] length 00250 * The amount of bytes to write. 00251 * @param[in] value 00252 * The bytes to write. 00253 * @param[in] onWrite 00254 * Continuation callback for the write operation 00255 * 00256 * @retval BLE_ERROR_NONE Successfully started the Write procedure, or 00257 * BLE_ERROR_INVALID_STATE if some internal state about the connection is invalid, or 00258 * BLE_STACK_BUSY if some client procedure is already in progress, or 00259 * BLE_ERROR_NO_MEM if there are no available buffers left to process the request, or 00260 * BLE_ERROR_OPERATION_NOT_PERMITTED due to the characteristic's properties. 00261 */ 00262 ble_error_t write(uint16_t length, const uint8_t *value, const GattClient::WriteCallback_t & onWrite) const; 00263 00264 void setupLongUUID(UUID::LongUUIDBytes_t longUUID, UUID::ByteOrder_t order = UUID::MSB) { 00265 uuid.setupLong(longUUID, order); 00266 } 00267 00268 public: 00269 /** 00270 * @brief Get the UUID of the discovered characteristic 00271 * @return the UUID of this characteristic 00272 */ 00273 const UUID& getUUID(void) const { 00274 return uuid; 00275 } 00276 00277 /** 00278 * @brief Get the properties of this characteristic 00279 * @return the set of properties of this characteristic 00280 */ 00281 const Properties_t& getProperties(void) const { 00282 return props; 00283 } 00284 00285 /** 00286 * @brief Get the declaration handle of this characteristic. 00287 * @details The declaration handle is the first handle of a characteristic 00288 * definition. The value accessible at this handle contains the following 00289 * informations: 00290 * - The characteristics properties (see Properties_t). This value can 00291 * be accessed by using #getProperties . 00292 * - The characteristic value attribute handle. This field can be accessed 00293 * by using #getValueHandle . 00294 * - The characteristic UUID, this value can be accessed by using the 00295 * function #getUUID . 00296 * @return the declaration handle of this characteristic. 00297 */ 00298 GattAttribute::Handle_t getDeclHandle(void) const { 00299 return declHandle; 00300 } 00301 00302 /** 00303 * @brief Return the handle used to access the value of this characteristic. 00304 * @details This handle is the one provided in the characteristic declaration 00305 * value. Usually, it is equal to #getDeclHandle() + 1. But it is not always 00306 * the case. Anyway, users are allowed to use #getDeclHandle() + 1 to access 00307 * the value of a characteristic. 00308 * @return The handle to access the value of this characteristic. 00309 */ 00310 GattAttribute::Handle_t getValueHandle(void) const { 00311 return valueHandle; 00312 } 00313 00314 /** 00315 * @brief Return the last handle of the characteristic definition. 00316 * @details A Characteristic definition can contain a lot of handles: 00317 * - one for the declaration (see #getDeclHandle) 00318 * - one for the value (see #getValueHandle) 00319 * - zero of more for the characteristic descriptors. 00320 * This handle is the last handle of the characteristic definition. 00321 * @return The last handle of this characteristic definition. 00322 */ 00323 GattAttribute::Handle_t getLastHandle(void) const { 00324 return lastHandle; 00325 } 00326 00327 /** 00328 * @brief Return the GattClient which can operate on this characteristic. 00329 * @return The GattClient which can operate on this characteristic. 00330 */ 00331 GattClient* getGattClient() { 00332 return gattc; 00333 } 00334 00335 /** 00336 * @brief Return the GattClient which can operate on this characteristic. 00337 * @return The GattClient which can operate on this characteristic. 00338 */ 00339 const GattClient* getGattClient() const { 00340 return gattc; 00341 } 00342 00343 /** 00344 * @brief Return the connection handle to the GattServer which contain 00345 * this characteristic. 00346 * @return the connection handle to the GattServer which contain 00347 * this characteristic. 00348 */ 00349 Gap::Handle_t getConnectionHandle() const { 00350 return connHandle; 00351 } 00352 00353 /** 00354 * @brief "Equal to" operator for DiscoveredCharacteristic 00355 * 00356 * @param[in] lhs 00357 * The left hand side of the equality expression 00358 * @param[in] rhs 00359 * The right hand side of the equality expression 00360 * 00361 * @return true if operands are equals, false otherwise. 00362 */ 00363 friend bool operator==(const DiscoveredCharacteristic& lhs, const DiscoveredCharacteristic& rhs) { 00364 return lhs.gattc == rhs.gattc && 00365 lhs.uuid == rhs.uuid && 00366 lhs.props == rhs.props && 00367 lhs.declHandle == rhs.declHandle && 00368 lhs.valueHandle == rhs.valueHandle && 00369 lhs.lastHandle == rhs.lastHandle && 00370 lhs.connHandle == rhs.connHandle; 00371 } 00372 00373 /** 00374 * @brief "Not equal to" operator for DiscoveredCharacteristic 00375 * 00376 * @param[in] lhs 00377 * The right hand side of the expression 00378 * @param[in] rhs 00379 * The left hand side of the expression 00380 * 00381 * @return true if operands are not equal, false otherwise. 00382 */ 00383 friend bool operator !=(const DiscoveredCharacteristic& lhs, const DiscoveredCharacteristic& rhs) { 00384 return !(lhs == rhs); 00385 } 00386 00387 public: 00388 DiscoveredCharacteristic() : gattc(NULL), 00389 uuid(UUID::ShortUUIDBytes_t(0)), 00390 props(), 00391 declHandle(GattAttribute::INVALID_HANDLE), 00392 valueHandle(GattAttribute::INVALID_HANDLE), 00393 lastHandle(GattAttribute::INVALID_HANDLE), 00394 connHandle() { 00395 /* empty */ 00396 } 00397 00398 protected: 00399 /** 00400 * Pointer to the underlying GattClient for this DiscoveredCharacteristic object. 00401 */ 00402 GattClient *gattc; 00403 00404 protected: 00405 /** 00406 * Discovered characteristic's UUID. 00407 */ 00408 UUID uuid; 00409 /** 00410 * Hold the configured properties of the discovered characteristic. 00411 * For more information refer to Properties_t. 00412 */ 00413 Properties_t props; 00414 /** 00415 * Value handle of the discovered characteristic's declaration attribute. 00416 */ 00417 GattAttribute::Handle_t declHandle; 00418 /** 00419 * Value handle of the discovered characteristic's value attribute. 00420 */ 00421 GattAttribute::Handle_t valueHandle; 00422 /** 00423 * Value handle of the discovered characteristic's last attribute. 00424 */ 00425 GattAttribute::Handle_t lastHandle; 00426 00427 /** 00428 * Handle for the connection where the characteristic was discovered. 00429 */ 00430 Gap::Handle_t connHandle; 00431 }; 00432 00433 #endif /*__DISCOVERED_CHARACTERISTIC_H__*/
Generated on Wed Jul 13 2022 09:31:10 by
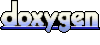