adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
DiscoveredCharacteristic.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "ble/DiscoveredCharacteristic.h" 00018 #include "ble/GattClient.h" 00019 00020 ble_error_t 00021 DiscoveredCharacteristic::read(uint16_t offset) const 00022 { 00023 if (!props.read()) { 00024 return BLE_ERROR_OPERATION_NOT_PERMITTED; 00025 } 00026 00027 if (!gattc) { 00028 return BLE_ERROR_INVALID_STATE; 00029 } 00030 00031 return gattc->read(connHandle, valueHandle, offset); 00032 } 00033 00034 struct OneShotReadCallback { 00035 static void launch(GattClient* client, Gap::Handle_t connHandle, 00036 GattAttribute::Handle_t handle, const GattClient::ReadCallback_t & cb) { 00037 OneShotReadCallback* oneShot = new OneShotReadCallback(client, connHandle, handle, cb); 00038 oneShot->attach(); 00039 // delete will be made when this callback is called 00040 } 00041 00042 private: 00043 OneShotReadCallback(GattClient* client, Gap::Handle_t connHandle, 00044 GattAttribute::Handle_t handle, const GattClient::ReadCallback_t & cb) : 00045 _client(client), 00046 _connHandle(connHandle), 00047 _handle(handle), 00048 _callback(cb) { } 00049 00050 void attach() { 00051 _client->onDataRead(makeFunctionPointer(this, &OneShotReadCallback::call)); 00052 } 00053 00054 void call(const GattReadCallbackParams* params) { 00055 // verifiy that it is the right characteristic on the right connection 00056 if (params->connHandle == _connHandle && params->handle == _handle) { 00057 _callback(params); 00058 _client->onDataRead().detach(makeFunctionPointer(this, &OneShotReadCallback::call)); 00059 delete this; 00060 } 00061 } 00062 00063 GattClient* _client; 00064 Gap::Handle_t _connHandle; 00065 GattAttribute::Handle_t _handle; 00066 GattClient::ReadCallback_t _callback; 00067 }; 00068 00069 ble_error_t DiscoveredCharacteristic::read(uint16_t offset, const GattClient::ReadCallback_t & onRead) const { 00070 ble_error_t error = read(offset); 00071 if (error) { 00072 return error; 00073 } 00074 00075 OneShotReadCallback::launch(gattc, connHandle, valueHandle, onRead); 00076 00077 return error; 00078 } 00079 00080 ble_error_t 00081 DiscoveredCharacteristic::write(uint16_t length, const uint8_t *value) const 00082 { 00083 if (!props.write()) { 00084 return BLE_ERROR_OPERATION_NOT_PERMITTED; 00085 } 00086 00087 if (!gattc) { 00088 return BLE_ERROR_INVALID_STATE; 00089 } 00090 00091 return gattc->write(GattClient::GATT_OP_WRITE_REQ, connHandle, valueHandle, length, value); 00092 } 00093 00094 ble_error_t 00095 DiscoveredCharacteristic::writeWoResponse(uint16_t length, const uint8_t *value) const 00096 { 00097 if (!props.writeWoResp()) { 00098 return BLE_ERROR_OPERATION_NOT_PERMITTED; 00099 } 00100 00101 if (!gattc) { 00102 return BLE_ERROR_INVALID_STATE; 00103 } 00104 00105 return gattc->write(GattClient::GATT_OP_WRITE_CMD, connHandle, valueHandle, length, value); 00106 } 00107 00108 struct OneShotWriteCallback { 00109 static void launch(GattClient* client, Gap::Handle_t connHandle, 00110 GattAttribute::Handle_t handle, const GattClient::WriteCallback_t & cb) { 00111 OneShotWriteCallback* oneShot = new OneShotWriteCallback(client, connHandle, handle, cb); 00112 oneShot->attach(); 00113 // delete will be made when this callback is called 00114 } 00115 00116 private: 00117 OneShotWriteCallback(GattClient* client, Gap::Handle_t connHandle, 00118 GattAttribute::Handle_t handle, const GattClient::WriteCallback_t & cb) : 00119 _client(client), 00120 _connHandle(connHandle), 00121 _handle(handle), 00122 _callback(cb) { } 00123 00124 void attach() { 00125 _client->onDataWritten(makeFunctionPointer(this, &OneShotWriteCallback::call)); 00126 } 00127 00128 void call(const GattWriteCallbackParams* params) { 00129 // verifiy that it is the right characteristic on the right connection 00130 if (params->connHandle == _connHandle && params->handle == _handle) { 00131 _callback(params); 00132 _client->onDataWritten().detach(makeFunctionPointer(this, &OneShotWriteCallback::call)); 00133 delete this; 00134 } 00135 } 00136 00137 GattClient* _client; 00138 Gap::Handle_t _connHandle; 00139 GattAttribute::Handle_t _handle; 00140 GattClient::WriteCallback_t _callback; 00141 }; 00142 00143 ble_error_t DiscoveredCharacteristic::write(uint16_t length, const uint8_t *value, const GattClient::WriteCallback_t & onRead) const { 00144 ble_error_t error = write(length, value); 00145 if (error) { 00146 return error; 00147 } 00148 00149 OneShotWriteCallback::launch(gattc, connHandle, valueHandle, onRead); 00150 00151 return error; 00152 } 00153 00154 ble_error_t DiscoveredCharacteristic::discoverDescriptors( 00155 const CharacteristicDescriptorDiscovery::DiscoveryCallback_t& onCharacteristicDiscovered, 00156 const CharacteristicDescriptorDiscovery::TerminationCallback_t& onTermination) const { 00157 00158 if(!gattc) { 00159 return BLE_ERROR_INVALID_STATE; 00160 } 00161 00162 ble_error_t err = gattc->discoverCharacteristicDescriptors( 00163 *this, onCharacteristicDiscovered, onTermination 00164 ); 00165 00166 return err; 00167 }
Generated on Wed Jul 13 2022 09:31:10 by
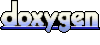