adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
DeviceInformationService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __BLE_DEVICE_INFORMATION_SERVICE_H__ 00018 #define __BLE_DEVICE_INFORMATION_SERVICE_H__ 00019 00020 #include "ble/BLE.h" 00021 00022 /** 00023 * @class DeviceInformationService 00024 * @brief BLE Device Information Service 00025 * Service: https://developer.bluetooth.org/gatt/services/Pages/ServiceViewer.aspx?u=org.bluetooth.service.device_information.xml 00026 * Manufacturer Name String Char: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.manufacturer_name_string.xml 00027 */ 00028 class DeviceInformationService { 00029 public: 00030 /** 00031 * @brief Device Information Service Constructor: copies device-specific information 00032 * into the BLE stack. 00033 * 00034 * @param[in] _ble 00035 * A reference to a BLE object for the underlying controller. 00036 * @param[in] manufacturersName 00037 * The name of the manufacturer of the device. 00038 * @param[in] modelNumber 00039 * The model number that is assigned by the device vendor. 00040 * @param[in] serialNumber 00041 * The serial number for a particular instance of the device. 00042 * @param[in] hardwareRevision 00043 * The hardware revision for the hardware within the device. 00044 * @param[in] firmwareRevision 00045 * The device's firmware version. 00046 * @param[in] softwareRevision 00047 * The device's software version. 00048 */ 00049 DeviceInformationService(BLE &_ble, 00050 const char *manufacturersName = NULL, 00051 const char *modelNumber = NULL, 00052 const char *serialNumber = NULL, 00053 const char *hardwareRevision = NULL, 00054 const char *firmwareRevision = NULL, 00055 const char *softwareRevision = NULL) : 00056 ble(_ble), 00057 manufacturersNameStringCharacteristic(GattCharacteristic::UUID_MANUFACTURER_NAME_STRING_CHAR, 00058 (uint8_t *)manufacturersName, 00059 (manufacturersName != NULL) ? strlen(manufacturersName) : 0, /* Min length */ 00060 (manufacturersName != NULL) ? strlen(manufacturersName) : 0, /* Max length */ 00061 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ), 00062 modelNumberStringCharacteristic(GattCharacteristic::UUID_MODEL_NUMBER_STRING_CHAR, 00063 (uint8_t *)modelNumber, 00064 (modelNumber != NULL) ? strlen(modelNumber) : 0, /* Min length */ 00065 (modelNumber != NULL) ? strlen(modelNumber) : 0, /* Max length */ 00066 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ), 00067 serialNumberStringCharacteristic(GattCharacteristic::UUID_SERIAL_NUMBER_STRING_CHAR, 00068 (uint8_t *)serialNumber, 00069 (serialNumber != NULL) ? strlen(serialNumber) : 0, /* Min length */ 00070 (serialNumber != NULL) ? strlen(serialNumber) : 0, /* Max length */ 00071 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ), 00072 hardwareRevisionStringCharacteristic(GattCharacteristic::UUID_HARDWARE_REVISION_STRING_CHAR, 00073 (uint8_t *)hardwareRevision, 00074 (hardwareRevision != NULL) ? strlen(hardwareRevision) : 0, /* Min length */ 00075 (hardwareRevision != NULL) ? strlen(hardwareRevision) : 0, /* Max length */ 00076 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ), 00077 firmwareRevisionStringCharacteristic(GattCharacteristic::UUID_FIRMWARE_REVISION_STRING_CHAR, 00078 (uint8_t *)firmwareRevision, 00079 (firmwareRevision != NULL) ? strlen(firmwareRevision) : 0, /* Min length */ 00080 (firmwareRevision != NULL) ? strlen(firmwareRevision) : 0, /* Max length */ 00081 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ), 00082 softwareRevisionStringCharacteristic(GattCharacteristic::UUID_SOFTWARE_REVISION_STRING_CHAR, 00083 (uint8_t *)softwareRevision, 00084 (softwareRevision != NULL) ? strlen(softwareRevision) : 0, /* Min length */ 00085 (softwareRevision != NULL) ? strlen(softwareRevision) : 0, /* Max length */ 00086 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ) 00087 { 00088 static bool serviceAdded = false; /* We only add the information service once. */ 00089 if (serviceAdded) { 00090 return; 00091 } 00092 00093 GattCharacteristic *charTable[] = {&manufacturersNameStringCharacteristic, 00094 &modelNumberStringCharacteristic, 00095 &serialNumberStringCharacteristic, 00096 &hardwareRevisionStringCharacteristic, 00097 &firmwareRevisionStringCharacteristic, 00098 &softwareRevisionStringCharacteristic}; 00099 GattService deviceInformationService(GattService::UUID_DEVICE_INFORMATION_SERVICE, charTable, 00100 sizeof(charTable) / sizeof(GattCharacteristic *)); 00101 00102 ble.addService(deviceInformationService); 00103 serviceAdded = true; 00104 } 00105 00106 protected: 00107 /** 00108 * A reference to the BLE instance object to which the services and 00109 * characteristics will be added. 00110 */ 00111 BLE &ble; 00112 /** 00113 * BLE characterising to allow BLE peers access to the manufacturer's name. 00114 */ 00115 GattCharacteristic manufacturersNameStringCharacteristic; 00116 /** 00117 * BLE characterising to allow BLE peers access to the model number. 00118 */ 00119 GattCharacteristic modelNumberStringCharacteristic; 00120 /** 00121 * BLE characterising to allow BLE peers access to the serial number. 00122 */ 00123 GattCharacteristic serialNumberStringCharacteristic; 00124 /** 00125 * BLE characterising to allow BLE peers access to the hardware revision string. 00126 */ 00127 GattCharacteristic hardwareRevisionStringCharacteristic; 00128 /** 00129 * BLE characterising to allow BLE peers access to the firmware revision string. 00130 */ 00131 GattCharacteristic firmwareRevisionStringCharacteristic; 00132 /** 00133 * BLE characterising to allow BLE peers access to the software revision string. 00134 */ 00135 GattCharacteristic softwareRevisionStringCharacteristic; 00136 }; 00137 00138 #endif /* #ifndef __BLE_DEVICE_INFORMATION_SERVICE_H__*/
Generated on Wed Jul 13 2022 09:31:10 by
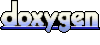