adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
DFUService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifdef TARGET_NRF51822 /* DFU only supported on nrf51 platforms */ 00018 00019 #ifndef __BLE_DFU_SERVICE_H__ 00020 #define __BLE_DFU_SERVICE_H__ 00021 00022 #include "ble/BLE.h" 00023 #include "ble/UUID.h" 00024 00025 extern "C" { 00026 #include "dfu_app_handler.h" 00027 } 00028 00029 extern const uint8_t DFUServiceBaseUUID[]; 00030 extern const uint16_t DFUServiceShortUUID; 00031 extern const uint16_t DFUServiceControlCharacteristicShortUUID; 00032 00033 extern const uint8_t DFUServiceUUID[]; 00034 extern const uint8_t DFUServiceControlCharacteristicUUID[]; 00035 extern const uint8_t DFUServicePacketCharacteristicUUID[]; 00036 00037 /** 00038 * @class DFUService 00039 * @brief Device Firmware Update Service. 00040 */ 00041 class DFUService { 00042 public: 00043 /** 00044 * @brief Signature for the handover callback. The application may provide this 00045 * callback when setting up the DFU service. The callback is then 00046 * invoked before handing control over to the bootloader. 00047 */ 00048 typedef void (*ResetPrepare_t)(void); 00049 00050 public: 00051 /** 00052 * @brief Adds Device Firmware Update Service to an existing BLE object. 00053 * 00054 * @param[ref] _ble 00055 * BLE object for the underlying controller. 00056 * @param[in] _handoverCallback 00057 * Application-specific handover callback. 00058 */ 00059 DFUService(BLE &_ble, ResetPrepare_t _handoverCallback = NULL) : 00060 ble(_ble), 00061 controlPoint(DFUServiceControlCharacteristicUUID, controlBytes, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00062 packet(DFUServicePacketCharacteristicUUID, packetBytes, SIZEOF_PACKET_BYTES, SIZEOF_PACKET_BYTES, 00063 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE), 00064 controlBytes(), 00065 packetBytes() { 00066 static bool serviceAdded = false; /* We only add the DFU service once. */ 00067 if (serviceAdded) { 00068 return; 00069 } 00070 00071 /* Set an initial value for control bytes, so that the application's DFU service can 00072 * be distinguished from the real DFU service provided by the bootloader. */ 00073 controlBytes[0] = 0xFF; 00074 controlBytes[1] = 0xFF; 00075 00076 GattCharacteristic *dfuChars[] = {&controlPoint, &packet}; 00077 GattService dfuService(DFUServiceUUID, dfuChars, sizeof(dfuChars) / sizeof(GattCharacteristic *)); 00078 00079 ble.addService(dfuService); 00080 handoverCallback = _handoverCallback; 00081 serviceAdded = true; 00082 00083 ble.onDataWritten(this, &DFUService::onDataWritten); 00084 } 00085 00086 /** 00087 * @brief Get the handle for the value attribute of the control characteristic. 00088 */ 00089 uint16_t getControlHandle(void) const { 00090 return controlPoint.getValueHandle(); 00091 } 00092 00093 /** 00094 * @brief This callback allows the DFU service to receive the initial trigger to 00095 * hand control over to the bootloader. First, the application is given a 00096 * chance to clean up. 00097 * 00098 * @param[in] params 00099 * Information about the characterisitc being updated. 00100 */ 00101 virtual void onDataWritten(const GattWriteCallbackParams *params) { 00102 if (params->handle == controlPoint.getValueHandle()) { 00103 /* At present, writing anything will do the trick - this needs to be improved. */ 00104 if (handoverCallback) { 00105 handoverCallback(); 00106 } 00107 00108 // Call bootloader_start implicitly trough a event handler call 00109 // it is a work around for bootloader_start not being public in sdk 8.1 00110 ble_dfu_t p_dfu; 00111 ble_dfu_evt_t p_evt; 00112 00113 p_dfu.conn_handle = params->connHandle; 00114 p_evt.ble_dfu_evt_type = BLE_DFU_START; 00115 00116 dfu_app_on_dfu_evt(&p_dfu, &p_evt); 00117 } 00118 } 00119 00120 protected: 00121 static const unsigned SIZEOF_CONTROL_BYTES = 2; 00122 static const unsigned SIZEOF_PACKET_BYTES = 20; 00123 00124 protected: 00125 BLE &ble; 00126 00127 /**< Writing to the control characteristic triggers the handover to DFU 00128 * bootloader. At present, writing anything will do the trick - this needs 00129 * to be improved. */ 00130 WriteOnlyArrayGattCharacteristic<uint8_t, SIZEOF_CONTROL_BYTES> controlPoint; 00131 00132 /**< The packet characteristic in this service doesn't do anything meaningful; 00133 * it is only a placeholder to mimic the corresponding characteristic in the 00134 * actual DFU service implemented by the bootloader. Without this, some 00135 * FOTA clients might get confused, because service definitions change after 00136 * handing control over to the bootloader. */ 00137 GattCharacteristic packet; 00138 00139 uint8_t controlBytes[SIZEOF_CONTROL_BYTES]; 00140 uint8_t packetBytes[SIZEOF_PACKET_BYTES]; 00141 00142 static ResetPrepare_t handoverCallback; /**< Application-specific handover callback. */ 00143 }; 00144 00145 #endif /* #ifndef __BLE_DFU_SERVICE_H__*/ 00146 #endif /* #ifdef TARGET_NRF51822 */
Generated on Wed Jul 13 2022 09:31:10 by
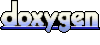