adaptation for book and plug demo
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
BatteryService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __BLE_BATTERY_SERVICE_H__ 00018 #define __BLE_BATTERY_SERVICE_H__ 00019 00020 #include "ble/BLE.h" 00021 00022 /** 00023 * @class BatteryService 00024 * @brief BLE Battery Service. This service displays the battery level from 0% to 100%, represented as an 8bit number. 00025 * Service: https://developer.bluetooth.org/gatt/services/Pages/ServiceViewer.aspx?u=org.bluetooth.service.battery_service.xml 00026 * Battery Level Char: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.battery_level.xml 00027 */ 00028 class BatteryService { 00029 public: 00030 /** 00031 * @param[in] _ble 00032 * BLE object for the underlying controller. 00033 * @param[in] level 00034 * 8bit batterly level. Usually used to represent percentage of batterly charge remaining. 00035 */ 00036 BatteryService (BLE &_ble, uint8_t level = 100) : 00037 ble(_ble), 00038 batteryLevel(level), 00039 batteryLevelCharacteristic(GattCharacteristic::UUID_BATTERY_LEVEL_CHAR, &batteryLevel, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY) { 00040 00041 GattCharacteristic *charTable[] = {&batteryLevelCharacteristic}; 00042 GattService batteryService(GattService::UUID_BATTERY_SERVICE, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00043 00044 ble.addService(batteryService); 00045 } 00046 00047 /** 00048 * @brief Update the battery level with a new value. Valid values lie between 0 and 100, 00049 * anything outside this range will be ignored. 00050 * 00051 * @param newLevel 00052 * Update to battery level. 00053 */ 00054 void updateBatteryLevel(uint8_t newLevel) { 00055 batteryLevel = newLevel; 00056 ble.gattServer().write(batteryLevelCharacteristic.getValueHandle(), &batteryLevel, 1); 00057 } 00058 00059 protected: 00060 /** 00061 * A reference to the underlying BLE instance that this object is attached to. 00062 * The services and characteristics will be registered in this BLE instance. 00063 */ 00064 BLE &ble; 00065 00066 /** 00067 * The current battery level represented as an integer from 0% to 100%. 00068 */ 00069 uint8_t batteryLevel; 00070 /** 00071 * A ReadOnlyGattCharacteristic that allows access to the peer device to the 00072 * batteryLevel value through BLE. 00073 */ 00074 ReadOnlyGattCharacteristic<uint8_t> batteryLevelCharacteristic; 00075 }; 00076 00077 #endif /* #ifndef __BLE_BATTERY_SERVICE_H__*/
Generated on Wed Jul 13 2022 09:31:10 by
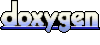