This library controls the WNC. There is a derived class for usage from the K64F board.
Fork of WncControllerLibrary by
The WncControllerLibrary API
Functions | |
WncController (void) | |
Constructor for WncController class, sets up internals. | |
WncState_e | getWncStatus (void) |
Used internally but also make public for a user of the Class to interrogate state as well. | |
bool | setApnName (const char *const apnStr) |
Allows a user to set the WNC modem to use the given Cellular APN. | |
int16_t | getDbmRssi (void) |
Queries the WNC modem for the current RX RSSI in units of coded dBm. | |
int16_t | get3gBer (void) |
Queries the WNC modem for the current Bit Error Rate. | |
bool | powerWncOn (const char *const apn, uint8_t powerUpTimeoutSecs=MAX_POWERUP_TIMEOUT) |
Powers up the WNC modem. | |
bool | getWncNetworkingStats (WncIpStats *s) |
Returns the NAT Self, gateway, masks and dns IP. | |
bool | resolveUrl (uint16_t numSock, const char *url) |
Takes a text URL and converts it internally to an IP address for the socket number given. | |
bool | setIpAddr (uint16_t numSock, const char *ipStr) |
If you know the IP address you can set the socket up to use it rather than using a text URL. | |
bool | openSocket (uint16_t numSock, uint16_t port, bool tcp, uint16_t timeOutSec=30) |
Opens a socket for the given number, port and IP protocol. | |
bool | openSocketUrl (uint16_t numSock, const char *url, uint16_t port, bool tcp, uint16_t timeOutSec=30) |
Opens a socket for the given text URL, number, port and IP protocol. | |
bool | openSocketIpAddr (uint16_t numSock, const char *ipAddr, uint16_t port, bool tcp, uint16_t timeOutSec=30) |
Opens a socket for the given text IP address, number, port and IP protocol. | |
bool | write (uint16_t numSock, const uint8_t *s, uint32_t n) |
Write data bytes to a Socket, the Socket must already be open. | |
size_t | read (uint16_t numSock, uint8_t *readBuf, uint32_t maxReadBufLen) |
Poll to read available data bytes from an already open Socket. | |
size_t | read (uint16_t numSock, const uint8_t **readBuf) |
Poll to read available data bytes from an already open Socket. | |
void | setReadRetries (uint16_t numSock, uint16_t retries) |
Set the number of retries that the read methods will use. | |
void | setReadRetryWait (uint16_t numSock, uint16_t waitMs) |
Set the time between retires that the read methods will use. | |
bool | closeSocket (uint16_t numSock) |
Closes an already open Socket. | |
void | setWncCmdTimeout (uint16_t toMs) |
Sets the amount of time to wait between the raw AT commands that are sent to the WNC modem. | |
bool | getIpAddr (uint16_t numSock, char myIpAddr[MAX_LEN_IP_STR]) |
Gets the IP address of the given socket number. | |
void | enableDebug (bool on, bool moreDebugOn) |
Enables debug output from this class. | |
bool | sendSMSText (const char *const phoneNum, const char *const text) |
Sends an SMS text message to someone. | |
bool | readSMSLog (struct WncSmsList *log) |
Incoming messages are stored in a log in the WNC modem, this will read that log. | |
bool | readUnreadSMSText (struct WncSmsList *w, bool deleteRead=true) |
Incoming messages are stored in a log in the WNC modem, this will read out messages that are unread and also then mark them read. | |
bool | saveSMSText (const char *const phoneNum, const char *const text, char *msgIdx) |
Saves a text message into internal SIM card memory of the WNC modem. | |
bool | sendSMSTextFromMem (char msgIdx) |
Sends a prior stored a text message from internal SIM card memory of the WNC modem. | |
bool | deleteSMSTextFromMem (char msgIdx) |
Deletes a prior stored a text message from internal SIM card memory of the WNC modem. | |
bool | getICCID (string *iccid) |
Retreives the SIM card ICCID number. | |
bool | convertICCIDtoMSISDN (const string &iccid, string *msisdn) |
Converts an ICCID number into a MSISDN number. | |
size_t | getSignalQuality (const char **log) |
Fetches the signal quality log from the WNC modem. | |
bool | getTimeDate (struct WncDateTime *tod) |
Fetches the cell tower's time and date. | |
bool | pingUrl (const char *url) |
ICMP Pings a URL, the results are only output to the debug log for now! | |
bool | pingIp (const char *ip) |
ICMP Pings an IP, the results are only output to the debug log for now! | |
size_t | sendCustomCmd (const char *cmd, char *resp, size_t sizeRespBuf, int ms_timeout) |
Allows a user to send a raw AT command to the WNC modem. |
Function Documentation
bool closeSocket | ( | uint16_t | numSock ) | [inherited] |
Closes an already open Socket.
- Parameters:
-
numSock - The number of the socket to open.
- Returns:
- true if success else false.
Definition at line 583 of file WncController.cpp.
bool convertICCIDtoMSISDN | ( | const string & | iccid, |
string * | msisdn | ||
) | [inherited] |
Converts an ICCID number into a MSISDN number.
The ATT SMS system for IoT only allows use of the 15-digit MSISDN number.
- Parameters:
-
iccid - the number to convert. msisdn - points to a C++ string that has the converted number.
- Returns:
- true if success else false.
Definition at line 838 of file WncController.cpp.
bool deleteSMSTextFromMem | ( | char | msgIdx ) | [inherited] |
Deletes a prior stored a text message from internal SIM card memory of the WNC modem.
If no messages are stored the behaviour of this method is undefined.
- Parameters:
-
msgIdx - the slot position to save the message: '1', '2', '3' or '*' deletes them all.
- Returns:
- true if success else false.
Definition at line 1295 of file WncController.cpp.
void enableDebug | ( | bool | on, |
bool | moreDebugOn | ||
) | [inherited] |
Enables debug output from this class.
- Parameters:
-
on - true enables debug output, false disables moreDebugOn - true enables verbose debug, false truncates debug output.
- Returns:
- none.
Definition at line 160 of file WncController.cpp.
int16_t get3gBer | ( | void | ) | [inherited] |
Queries the WNC modem for the current Bit Error Rate.
- Returns:
- 0...7 – as RXQUAL values in the table in 3GPP TS 45.008 subclause 8.2.4 99 – not known or not detectable
Definition at line 180 of file WncController.cpp.
int16_t getDbmRssi | ( | void | ) | [inherited] |
Queries the WNC modem for the current RX RSSI in units of coded dBm.
- Returns:
- 0 – -113 dBm or less 1 – -111 dBm 2...30 – -109 dBm to –53 dBm 31 – -51 dBm or greater 99 – not known or not detectable
Definition at line 171 of file WncController.cpp.
bool getICCID | ( | string * | iccid ) | [inherited] |
Retreives the SIM card ICCID number.
- Parameters:
-
iccid - a pointer to C++ string that contains the retrieved number.
- Returns:
- true if success else false.
Definition at line 803 of file WncController.cpp.
bool getIpAddr | ( | uint16_t | numSock, |
char | myIpAddr[MAX_LEN_IP_STR] | ||
) | [inherited] |
Gets the IP address of the given socket number.
- Parameters:
-
numSock - The number of the socket to open. myIpAddr - a c-string that contains the socket's IP address.
- Returns:
- true if success else false.
Definition at line 255 of file WncController.cpp.
size_t getSignalQuality | ( | const char ** | log ) | [inherited] |
Fetches the signal quality log from the WNC modem.
- Parameters:
-
log - a pointer to an internal buffer who's contents contain the signal quality metrics.
- Returns:
- The number of chars in the log.
Definition at line 1027 of file WncController.cpp.
bool getTimeDate | ( | struct WncDateTime * | tod ) | [inherited] |
Fetches the cell tower's time and date.
The time is accurate when read but significant delays exist between the time it is read and returned.
- Parameters:
-
tod - User supplies a pointer to a tod struct and this method fills it in.
- Returns:
- true if success else false.
Definition at line 1106 of file WncController.cpp.
bool getWncNetworkingStats | ( | WncIpStats * | s ) | [inherited] |
Returns the NAT Self, gateway, masks and dns IP.
- Parameters:
-
s - a pointer to a struct that will contain the IP info.
- Returns:
- true if success, else false.
Definition at line 250 of file WncController.cpp.
WncController::WncState_e getWncStatus | ( | void | ) | [inherited] |
Used internally but also make public for a user of the Class to interrogate state as well.
- Returns:
- the current state of the Wnc hardware.
Definition at line 166 of file WncController.cpp.
bool openSocket | ( | uint16_t | numSock, |
uint16_t | port, | ||
bool | tcp, | ||
uint16_t | timeOutSec = 30 |
||
) | [inherited] |
Opens a socket for the given number, port and IP protocol.
Before using open, you must use either resolveUrl() or setIpAddr().
- Parameters:
-
numSock - The number of the socket to open. port - the IP port to open tcp - set true for TCP, false for UDP timeoutSec - the amount of time in seconds to wait for the open to complete
- Returns:
- true if success, else false.
Definition at line 332 of file WncController.cpp.
bool openSocketIpAddr | ( | uint16_t | numSock, |
const char * | ipAddr, | ||
uint16_t | port, | ||
bool | tcp, | ||
uint16_t | timeOutSec = 30 |
||
) | [inherited] |
Opens a socket for the given text IP address, number, port and IP protocol.
- Parameters:
-
numSock - The number of the socket to open. ipAddr - a c-string text IP address like: "192.168.0.1". port - the IP port to open. tcp - set true for TCP, false for UDP. timeoutSec - the amount of time in seconds to wait for the open to complete.
- Returns:
- true if success, else false.
Definition at line 324 of file WncController.cpp.
bool openSocketUrl | ( | uint16_t | numSock, |
const char * | url, | ||
uint16_t | port, | ||
bool | tcp, | ||
uint16_t | timeOutSec = 30 |
||
) | [inherited] |
Opens a socket for the given text URL, number, port and IP protocol.
- Parameters:
-
numSock - The number of the socket to open. url - a c-string text URL, the one to open a socket for. port - the IP port to open. tcp - set true for TCP, false for UDP. timeoutSec - the amount of time in seconds to wait for the open to complete.
- Returns:
- true if success, else false.
Definition at line 316 of file WncController.cpp.
bool pingIp | ( | const char * | ip ) | [inherited] |
ICMP Pings an IP, the results are only output to the debug log for now!
- Parameters:
-
ip - a c-string whose IP is to be pinged.
- Returns:
- true if success else false.
Definition at line 240 of file WncController.cpp.
bool pingUrl | ( | const char * | url ) | [inherited] |
ICMP Pings a URL, the results are only output to the debug log for now!
- Parameters:
-
url - a c-string whose URL is to be pinged.
- Returns:
- true if success else false.
Definition at line 228 of file WncController.cpp.
bool powerWncOn | ( | const char *const | apn, |
uint8_t | powerUpTimeoutSecs = MAX_POWERUP_TIMEOUT |
||
) | [inherited] |
Powers up the WNC modem.
- Parameters:
-
apn - the apn c-string to set the WNC modem to use powerUpTimeoutSecs - the amount of time to wait for the WNC modem to turn on
- Returns:
- true if powerup was a success, else false.
Definition at line 189 of file WncController.cpp.
size_t read | ( | uint16_t | numSock, |
uint8_t * | readBuf, | ||
uint32_t | maxReadBufLen | ||
) | [inherited] |
Poll to read available data bytes from an already open Socket.
This method will retry reads to what setReadRetries() sets it to and the delay in between retries that is set with setReadRetryWait()
- Parameters:
-
numSock - The number of the socket to open. readBuf - a pointer to where read will put the data. maxReadBufLen - The number of bytes readBuf has room for.
- Returns:
- the number of bytes actually read into readBuf. 0 is a valid value if no data is available.
Definition at line 499 of file WncController.cpp.
size_t read | ( | uint16_t | numSock, |
const uint8_t ** | readBuf | ||
) | [inherited] |
Poll to read available data bytes from an already open Socket.
This method will retry reads to what setReadRetries() sets it to and the delay in between retries that is set with setReadRetryWait()
- Parameters:
-
numSock - The number of the socket to open. readBuf - a pointer to pointer that will be set to point to an internal byte buffer that contains any read data.
- Returns:
- the number of bytes actually read into the pointer that readBuf points to. 0 is a valid value if no data is available.
Definition at line 438 of file WncController.cpp.
bool readSMSLog | ( | struct WncSmsList * | log ) | [inherited] |
Incoming messages are stored in a log in the WNC modem, this will read that log.
- Parameters:
-
log - the log contents if reading it was successful.
- Returns:
- true if success else false.
Definition at line 867 of file WncController.cpp.
bool readUnreadSMSText | ( | struct WncSmsList * | w, |
bool | deleteRead = true |
||
) | [inherited] |
Incoming messages are stored in a log in the WNC modem, this will read out messages that are unread and also then mark them read.
- Parameters:
-
w - a list of SMS messages that unread messages will be put into. deleteRead - if a message is read and this is set true the message will be deleted from the WNC modem log. If it is false the message will remain in the internal log but be marked as read.
- Returns:
- true if success else false.
Definition at line 1005 of file WncController.cpp.
bool resolveUrl | ( | uint16_t | numSock, |
const char * | url | ||
) | [inherited] |
Takes a text URL and converts it internally to an IP address for the socket number given.
- Parameters:
-
numSock - The number of the socket to lookup the IP address for. url - a c-string text URL
- Returns:
- true if success, else false.
Definition at line 279 of file WncController.cpp.
bool saveSMSText | ( | const char *const | phoneNum, |
const char *const | text, | ||
char * | msgIdx | ||
) | [inherited] |
Saves a text message into internal SIM card memory of the WNC modem.
There are only 3 slots available this is for unread, read and saved.
- Parameters:
-
phoneNum - c-string 15 digit MSISDN number or ATT Jasper number (standard phone number not supported because ATT IoT SMS does not support it). text - the c-string text to send to someone. msgIdx - the slot position to save the message: '1', '2', '3'
- Returns:
- true if success else false.
Definition at line 1416 of file WncController.cpp.
size_t sendCustomCmd | ( | const char * | cmd, |
char * | resp, | ||
size_t | sizeRespBuf, | ||
int | ms_timeout | ||
) | [inherited] |
Allows a user to send a raw AT command to the WNC modem.
- Parameters:
-
cmd - the c-string cmd to send like: "AT" resp - a pointer to the c-string cmd's response. sizeRespBuf - how large the command response buffer is, sets the max response length. ms_timeout - how long to wait for the WNC to respond to your command.
- Returns:
- the number of characters in the response from the WNC modem.
Definition at line 210 of file WncController.cpp.
bool sendSMSText | ( | const char *const | phoneNum, |
const char *const | text | ||
) | [inherited] |
Sends an SMS text message to someone.
- Parameters:
-
phoneNum - c-string 15 digit MSISDN number or ATT Jasper number (standard phone number not supported because ATT IoT SMS does not support it). text - the c-string text to send to someone.
- Returns:
- true if success else false.
Definition at line 857 of file WncController.cpp.
bool sendSMSTextFromMem | ( | char | msgIdx ) | [inherited] |
Sends a prior stored a text message from internal SIM card memory of the WNC modem.
If no messages are stored the behaviour of this method is undefined.
- Parameters:
-
msgIdx - the slot position to save the message: '1', '2', '3'
- Returns:
- true if success else false.
Definition at line 1323 of file WncController.cpp.
bool setApnName | ( | const char *const | apnStr ) | [inherited] |
Allows a user to set the WNC modem to use the given Cellular APN.
- Parameters:
-
apnStr - a null terminated c-string
- Returns:
- true if the APN set was succesful, else false
Definition at line 268 of file WncController.cpp.
bool setIpAddr | ( | uint16_t | numSock, |
const char * | ipStr | ||
) | [inherited] |
If you know the IP address you can set the socket up to use it rather than using a text URL.
- Parameters:
-
numSock - The number of the socket to use the IP address for. ipStr - a c-string text IP addrese like: 192.168.0.1
- Returns:
- true if success, else false.
Definition at line 299 of file WncController.cpp.
void setReadRetries | ( | uint16_t | numSock, |
uint16_t | retries | ||
) | [inherited] |
Set the number of retries that the read methods will use.
If a read returns 0 data this setting will have the read re-read to see if new data is available.
- Parameters:
-
numSock - The number of the socket to open. retries - the number of retries to perform.
- Returns:
- none.
Definition at line 567 of file WncController.cpp.
void setReadRetryWait | ( | uint16_t | numSock, |
uint16_t | waitMs | ||
) | [inherited] |
Set the time between retires that the read methods will use.
If a read returns 0 data this setting will have the read re-read and use this amount of delay in between the re-reads.
- Parameters:
-
numSock - The number of the socket to open. waitMs - the amount of time in mS to wait between retries.
- Returns:
- none.
Definition at line 575 of file WncController.cpp.
void setWncCmdTimeout | ( | uint16_t | toMs ) | [inherited] |
Sets the amount of time to wait between the raw AT commands that are sent to the WNC modem.
Generally you don't want to use this but it is here just in case.
- Parameters:
-
toMs - num mS to wait between the AT cmds.
- Returns:
- none.
Definition at line 311 of file WncController.cpp.
WncController | ( | void | ) | [inherited] |
Constructor for WncController class, sets up internals.
- Returns:
- none.
Definition at line 154 of file WncController.cpp.
bool write | ( | uint16_t | numSock, |
const uint8_t * | s, | ||
uint32_t | n | ||
) | [inherited] |
Write data bytes to a Socket, the Socket must already be open.
- Parameters:
-
numSock - The number of the socket to open. s - an array of bytes to write to the socket. n - the number of bytes to write.
- Returns:
- true if success, else false.
Definition at line 399 of file WncController.cpp.
Generated on Tue Jul 12 2022 14:17:29 by
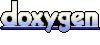