
Program files for Example program for EVAL-AD7768-1
Dependencies: platform_drivers
app_config_mbed.h
00001 /***************************************************************************//* 00002 * @file app_config_mbed.h 00003 * @brief Header file for Mbed platform configurations 00004 ****************************************************************************** 00005 * Copyright (c) 2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 ******************************************************************************/ 00012 00013 #ifndef _APP_CONFIG_MBED_H_ 00014 #define _APP_CONFIG_MBED_H_ 00015 00016 /******************************************************************************/ 00017 /***************************** Include Files **********************************/ 00018 /******************************************************************************/ 00019 00020 #include <stdint.h> 00021 #include <PinNames.h> 00022 00023 #include "uart_extra.h" 00024 #include "irq_extra.h" 00025 #include "spi_extra.h" 00026 00027 /******************************************************************************/ 00028 /********************** Macros and Constants Definition ***********************/ 00029 /******************************************************************************/ 00030 00031 // Pin mapping of AD7768-1 with arduino 00032 #define SPI_SS D10 // SPI_CS 00033 #define SPI_MOSI D11 // SPI_MOSI 00034 #define SPI_MISO D12 // SPI_MISO 00035 #define SPI_SCK D13 // SPI_SCK 00036 #define DRDY D2 // Conversion ready interrupt 00037 00038 /* Common pin mapping */ 00039 #define UART_TX USBTX 00040 #define UART_RX USBRX 00041 #define LED_GREEN LED3 00042 00043 /* Define the max possible sampling frequency (or output data) rate for AD77681 (in SPS). 00044 * This is also used to find the time period to trigger a periodic conversion event. 00045 * Note: Max possible ODR is 64KSPS for continuous data capture on IIO Client. 00046 * This is derived by capturing data from the firmware using the SDP-K1 controller board 00047 * @22.5Mhz SPI clock. The max possible ODR can vary from board to board and 00048 * data continuity is not guaranteed above this ODR on IIO oscilloscope */ 00049 00050 /* AD77681 default internal clock frequency (MCLK = 16.384 Mhz) */ 00051 #define AD77681_MCLK (16384) 00052 00053 /* AD77681 decimation rate */ 00054 #define AD77681_DECIMATION_RATE (32U) 00055 00056 /* AD77681 default mclk_div value */ 00057 #define AD77681_DEFAULT_MCLK_DIV (8) 00058 00059 /* AD77681 ODR conversion */ 00060 #define AD77681_ODR_CONV_SCALER (AD77681_DECIMATION_RATE * AD77681_DEFAULT_MCLK_DIV) 00061 00062 /* AD77681 default sampling frequency */ 00063 #define AD77681_DEFAULT_SAMPLING_FREQ ((AD77681_MCLK * 1000) / AD77681_ODR_CONV_SCALER) 00064 00065 /******************************************************************************/ 00066 /********************* Public/Extern Declarations *****************************/ 00067 /******************************************************************************/ 00068 extern mbed_irq_init_param mbed_ext_int_init_param; 00069 extern mbed_uart_init_param mbed_uart_extra_init_param; 00070 extern mbed_spi_init_param mbed_spi_init_extra_params; 00071 00072 #endif /* _APP_CONFIG_H_ */
Generated on Tue Jul 12 2022 19:15:23 by
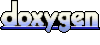