
Program files for Example program for EVAL-AD7768-1
Dependencies: platform_drivers
ad77681_data_capture.c
00001 /***************************************************************************//** 00002 * @file ad77681_data_capture.c 00003 * @brief Data capture interface for AD7768-1 IIO application 00004 * @details This module handles the AD7768-1 data capturing 00005 ******************************************************************************** 00006 * Copyright (c) 2021 Analog Devices, Inc. 00007 * 00008 * All rights reserved. 00009 * 00010 * This software is proprietary to Analog Devices, Inc. and its licensors. 00011 * By using this software you agree to the terms of the associated 00012 * Analog Devices Software License Agreement. 00013 *******************************************************************************/ 00014 00015 /******************************************************************************/ 00016 /***************************** Include Files **********************************/ 00017 /******************************************************************************/ 00018 00019 #include <string.h> 00020 #include <stdlib.h> 00021 00022 #include "app_config.h" 00023 #include "ad77681_iio.h" 00024 #include "ad77681_data_capture.h" 00025 #include "adc_data_capture.h" 00026 #include "error.h" 00027 00028 /******************************************************************************/ 00029 /********************** Macros and Constants Definition ***********************/ 00030 /******************************************************************************/ 00031 00032 /* AD77681 ADC Resolution */ 00033 #define AD77681_ADC_RESOLUTION 24 00034 /* AD77681 Buffer Length */ 00035 #define AD77681_SAMPLE_DATA_BUFF_LEN 6 00036 /* AD77681 24 Bits Sign Extension Value */ 00037 #define AD77681_24_BITS_SIGN_EXTENSION 0xFFFFFF 00038 /* AD77681 2 Bytes Shift Value */ 00039 #define AD77681_2_BYTES_SHIFT 16 00040 /* AD77681 1 Byte Shift Value */ 00041 #define AD77681_1_BYTE_SHIFT 8 00042 00043 /******************************************************************************/ 00044 /********************** Variables and User Defined Data Types *****************/ 00045 /******************************************************************************/ 00046 00047 /******************************************************************************/ 00048 /************************ Functions Declarations ******************************/ 00049 /******************************************************************************/ 00050 00051 /* Perform single sample read pre-operations */ 00052 static int32_t ad77681_single_sample_read_start_ops(uint8_t input_chn); 00053 /* Perform continuous sample read pre-operations */ 00054 static int32_t ad77681_continuous_sample_read_start_ops(uint32_t chn_mask); 00055 /* Perform conversion on input channel and read single conversion sample */ 00056 static int32_t ad77681_perform_conv_and_read_sample(uint32_t *read_adc_data, 00057 uint8_t chn); 00058 /* Read ADC sampled/conversion data */ 00059 static int32_t ad77681_read_converted_sample(uint32_t *adc_raw, uint8_t chn_indx); 00060 00061 /* Define the variable for data_capture_ops structure */ 00062 struct data_capture_ops data_capture_ops = { 00063 /* Point AD77681 data capture functions to generic ADC data capture functions */ 00064 .single_sample_read_start_ops = ad77681_single_sample_read_start_ops, 00065 .perform_conv_and_read_sample = ad77681_perform_conv_and_read_sample, 00066 .single_sample_read_stop_ops = NULL, 00067 .continuous_sample_read_start_ops = ad77681_continuous_sample_read_start_ops, 00068 .read_converted_sample = ad77681_read_converted_sample, 00069 .continuous_sample_read_stop_ops = NULL, 00070 .trigger_next_conversion = NULL, 00071 }; 00072 00073 /******************************************************************************/ 00074 /************************ Functions Definitions *******************************/ 00075 /******************************************************************************/ 00076 00077 /*! 00078 * @brief Perform the operations required before starting continuous sample read 00079 * @param input_chn[out] - ADC input channel 00080 * @return SUCCESS in case of success, FAILURE otherwise 00081 */ 00082 int32_t ad77681_single_sample_read_start_ops(uint8_t input_chn) 00083 { 00084 00085 int32_t single_read_sts = FAILURE; 00086 00087 single_read_sts = ad77681_set_conv_mode 00088 ( 00089 p_ad77681_dev_inst, 00090 AD77681_CONV_SINGLE, 00091 AD77681_AIN_SHORT, 00092 false 00093 ); 00094 00095 return single_read_sts; 00096 } 00097 00098 /*! 00099 * @brief Perform the operations required before starting continuous sample read 00100 * @param chn_mask[in] - holds the list of all active channels set from IIO client for data capturing 00101 * @return SUCCESS in case of success, FAILURE otherwise 00102 */ 00103 int32_t ad77681_continuous_sample_read_start_ops(uint32_t chn_mask) 00104 { 00105 int32_t cont_read_sts = FAILURE; 00106 00107 cont_read_sts = ad77681_set_conv_mode 00108 ( 00109 p_ad77681_dev_inst, 00110 AD77681_CONV_CONTINUOUS, 00111 AD77681_AIN_SHORT, 00112 false 00113 ); 00114 00115 return cont_read_sts; 00116 } 00117 00118 /*! 00119 * @brief Read ADC raw data for recently sampled channel 00120 * @param adc_raw[out] - Pointer to adc data read variable 00121 * @param chn_indx[in] - Channel for which data is to read 00122 * @return SUCCESS in case of success, FAILURE otherwise 00123 * @note This function is intended to call from the conversion end trigger 00124 * event. Therefore, this function should just read raw ADC data 00125 * without further monitoring conversion end event 00126 */ 00127 int32_t ad77681_read_converted_sample(uint32_t *adc_raw, uint8_t chn_indx) 00128 { 00129 uint32_t ad77681_sample_data = 0; 00130 uint8_t ad77681_sample_data_buff[AD77681_SAMPLE_DATA_BUFF_LEN] = { 0, 0, 0, 0, 0, 0 }; 00131 00132 if (!adc_raw) { 00133 return FAILURE; 00134 } 00135 00136 if (ad77681_spi_read_adc_data(p_ad77681_dev_inst, ad77681_sample_data_buff, 00137 AD77681_REGISTER_DATA_READ) != SUCCESS) { 00138 return FAILURE; 00139 } 00140 00141 ad77681_sample_data = (uint32_t) 00142 ( 00143 (ad77681_sample_data_buff[1] << AD77681_2_BYTES_SHIFT) | 00144 (ad77681_sample_data_buff[2] << AD77681_1_BYTE_SHIFT ) | 00145 (ad77681_sample_data_buff[3]) 00146 ); 00147 *adc_raw = ad77681_sample_data & AD77681_24_BITS_SIGN_EXTENSION; 00148 00149 return SUCCESS; 00150 } 00151 00152 /*! 00153 * @brief Read ADC single sample data 00154 * @param read_adc_data[out] - Pointer to adc data read variable 00155 * @param chn[in] - Channel for which data is to read 00156 * @return SUCCESS in case of success, FAILURE otherwise 00157 * @details This function performs the sampling on previously active channel 00158 * and then read conversion result 00159 */ 00160 int32_t ad77681_perform_conv_and_read_sample(uint32_t *read_adc_data, 00161 uint8_t chn) 00162 { 00163 uint32_t ad77681_sample_data = 0; 00164 00165 if (ad77681_read_converted_sample(&ad77681_sample_data, 0) != SUCCESS) { 00166 return FAILURE; 00167 } 00168 *read_adc_data = ad77681_sample_data; 00169 00170 return SUCCESS; 00171 }
Generated on Tue Jul 12 2022 19:15:23 by
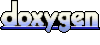