
Program files for Example program for EVAL-AD7768-1
Dependencies: platform_drivers
ad77681.c
00001 /***************************************************************************//** 00002 * @file ad77681.c 00003 * @brief Implementation of AD7768-1 Driver. 00004 * @author SPopa (stefan.popa@analog.com) 00005 ******************************************************************************** 00006 * Copyright 2017(c) Analog Devices, Inc. 00007 * 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without 00011 * modification, are permitted provided that the following conditions are met: 00012 * - Redistributions of source code must retain the above copyright 00013 * notice, this list of conditions and the following disclaimer. 00014 * - Redistributions in binary form must reproduce the above copyright 00015 * notice, this list of conditions and the following disclaimer in 00016 * the documentation and/or other materials provided with the 00017 * distribution. 00018 * - Neither the name of Analog Devices, Inc. nor the names of its 00019 * contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * - The use of this software may or may not infringe the patent rights 00022 * of one or more patent holders. This license does not release you 00023 * from the requirement that you obtain separate licenses from these 00024 * patent holders to use this software. 00025 * - Use of the software either in source or binary form, must be run 00026 * on or directly connected to an Analog Devices Inc. component. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00029 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00030 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00031 * IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00033 * LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 *******************************************************************************/ 00039 00040 /******************************************************************************/ 00041 /***************************** Include Files **********************************/ 00042 /******************************************************************************/ 00043 #include "stdio.h" 00044 #include "stdlib.h" 00045 #include "stdbool.h" 00046 #include <string.h> 00047 #include "ad77681.h" 00048 #include "error.h" 00049 #include "delay.h" 00050 00051 /******************************************************************************/ 00052 /************************** Functions Implementation **************************/ 00053 /******************************************************************************/ 00054 /** 00055 * Compute CRC8 checksum. 00056 * @param data - The data buffer. 00057 * @param data_size - The size of the data buffer. 00058 * @param init_val - CRC initial value. 00059 * @return CRC8 checksum. 00060 */ 00061 uint8_t ad77681_compute_crc8(uint8_t *data, 00062 uint8_t data_size, 00063 uint8_t init_val) 00064 { 00065 uint8_t i; 00066 uint8_t crc = init_val; 00067 00068 while (data_size) { 00069 for (i = 0x80; i != 0; i >>= 1) { 00070 if (((crc & 0x80) != 0) != ((*data & i) != 0)) { 00071 crc <<= 1; 00072 crc ^= AD77681_CRC8_POLY; 00073 } else 00074 crc <<= 1; 00075 } 00076 data++; 00077 data_size--; 00078 } 00079 return crc; 00080 } 00081 00082 /** 00083 * Compute XOR checksum. 00084 * @param data - The data buffer. 00085 * @param data_size - The size of the data buffer. 00086 * @param init_val - CRC initial value. 00087 * @return XOR checksum. 00088 */ 00089 uint8_t ad77681_compute_xor(uint8_t *data, 00090 uint8_t data_size, 00091 uint8_t init_val) 00092 { 00093 uint8_t crc = init_val; 00094 uint8_t buf[3]; 00095 uint8_t i; 00096 00097 for (i = 0; i < data_size; i++) { 00098 buf[i] = *data; 00099 crc ^= buf[i]; 00100 data++; 00101 } 00102 return crc; 00103 } 00104 00105 /** 00106 * Read from device. 00107 * @param dev - The device structure. 00108 * @param reg_addr - The register address. 00109 * @param reg_data - The register data. 00110 * @return 0 in case of success, negative error code otherwise. 00111 */ 00112 int32_t ad77681_spi_reg_read(struct ad77681_dev *dev, 00113 uint8_t reg_addr, 00114 uint8_t *reg_data) 00115 { 00116 int32_t ret; 00117 uint8_t crc; 00118 uint8_t buf[3], crc_buf[2]; 00119 uint8_t buf_len = (dev->crc_sel == AD77681_NO_CRC) ? 2 : 3; 00120 00121 buf[0] = AD77681_REG_READ(reg_addr); 00122 buf[1] = 0x00; 00123 00124 ret = spi_write_and_read(dev->spi_desc, buf, buf_len); 00125 if (ret < 0) 00126 return ret; 00127 00128 /* XOR or CRC checksum for read transactions */ 00129 if (dev->crc_sel != AD77681_NO_CRC) { 00130 crc_buf[0] = AD77681_REG_READ(reg_addr); 00131 crc_buf[1] = buf[1]; 00132 00133 if (dev->crc_sel == AD77681_XOR) 00134 /* INITIAL_CRC is 0, when ADC is not in continuous-read mode */ 00135 crc = ad77681_compute_xor(crc_buf, 2, INITIAL_CRC); 00136 else if(dev->crc_sel == AD77681_CRC) 00137 /* INITIAL_CRC is 0, when ADC is not in continuous-read mode */ 00138 crc = ad77681_compute_crc8(crc_buf, 2, INITIAL_CRC); 00139 00140 /* In buf[2] is CRC from the ADC */ 00141 if (crc != buf[2]) 00142 ret = FAILURE; 00143 #ifdef CRC_DEBUG 00144 printf("\n%x\t%x\tCRC/XOR: %s\n", crc, 00145 buf[2], ((crc != buf[2]) ? "FAULT" : "OK")); 00146 #endif /* CRC_DEBUG */ 00147 } 00148 00149 reg_data[0] = AD77681_REG_READ(reg_addr); 00150 memcpy(reg_data + 1, buf + 1, ARRAY_SIZE(buf) - 1); 00151 00152 return ret; 00153 } 00154 00155 /** 00156 * Write to device. 00157 * @param dev - The device structure. 00158 * @param reg_addr - The register address. 00159 * @param reg_data - The register data. 00160 * @return 0 in case of success, negative error code otherwise. 00161 */ 00162 int32_t ad77681_spi_reg_write(struct ad77681_dev *dev, 00163 uint8_t reg_addr, 00164 uint8_t reg_data) 00165 { 00166 uint8_t buf[3]; 00167 /* Buffer length in case of checksum usage */ 00168 uint8_t buf_len = (dev->crc_sel == AD77681_NO_CRC) ? 2 : 3; 00169 00170 buf[0] = AD77681_REG_WRITE(reg_addr); 00171 buf[1] = reg_data; 00172 00173 /* CRC only for read transactions, CRC and XOR for write transactions*/ 00174 if (dev->crc_sel != AD77681_NO_CRC) 00175 buf[2] = ad77681_compute_crc8(buf, 2, INITIAL_CRC); 00176 00177 return spi_write_and_read(dev->spi_desc, buf, buf_len); 00178 } 00179 00180 /** 00181 * SPI read from device using a mask. 00182 * @param dev - The device structure. 00183 * @param reg_addr - The register address. 00184 * @param mask - The mask. 00185 * @param data - The register data. 00186 * @return 0 in case of success, negative error code otherwise. 00187 */ 00188 int32_t ad77681_spi_read_mask(struct ad77681_dev *dev, 00189 uint8_t reg_addr, 00190 uint8_t mask, 00191 uint8_t *data) 00192 { 00193 uint8_t reg_data[3]; 00194 int32_t ret; 00195 00196 ret = ad77681_spi_reg_read(dev, reg_addr, reg_data); 00197 *data = (reg_data[1] & mask); 00198 00199 return ret; 00200 } 00201 00202 /** 00203 * SPI write to device using a mask. 00204 * @param dev - The device structure. 00205 * @param reg_addr - The register address. 00206 * @param mask - The mask. 00207 * @param data - The register data. 00208 * @return 0 in case of success, negative error code otherwise. 00209 */ 00210 int32_t ad77681_spi_write_mask(struct ad77681_dev *dev, 00211 uint8_t reg_addr, 00212 uint8_t mask, 00213 uint8_t data) 00214 { 00215 uint8_t reg_data[3]; 00216 int32_t ret; 00217 00218 ret = ad77681_spi_reg_read(dev, reg_addr, reg_data); 00219 reg_data[1] &= ~mask; 00220 reg_data[1] |= data; 00221 ret |= ad77681_spi_reg_write(dev, reg_addr, reg_data[1]); 00222 00223 return ret; 00224 } 00225 00226 /** 00227 * Helper function to get the number of rx bytes 00228 * @param dev - The device structure. 00229 * @return rx_buf_len - the number of rx bytes 00230 */ 00231 uint8_t ad77681_get_rx_buf_len(struct ad77681_dev *dev) 00232 { 00233 uint8_t rx_buf_len = 0; 00234 uint8_t data_len = 0; 00235 uint8_t crc = 0; 00236 uint8_t status_bit = 0; 00237 00238 data_len = 3; 00239 crc = (dev->crc_sel == AD77681_NO_CRC) ? 0 : 1; // 1 byte for crc 00240 status_bit = dev->status_bit; // one byte for status 00241 00242 rx_buf_len = data_len + crc + status_bit; 00243 00244 return rx_buf_len; 00245 } 00246 00247 /** 00248 * Helper function to get the number of SPI 16bit frames for INTERRUPT ADC DATA READ 00249 * @param dev - The device structure. 00250 * @return frame_16bit - the number of 16 bit SPI frames 00251 */ 00252 uint8_t ad77681_get_frame_byte(struct ad77681_dev *dev) 00253 { 00254 /* number of 8bit frames */ 00255 uint8_t frame_bytes; 00256 if (dev->conv_len == AD77681_CONV_24BIT) 00257 frame_bytes = 3; 00258 else 00259 frame_bytes = 2; 00260 if (dev->crc_sel != AD77681_NO_CRC) 00261 frame_bytes++; 00262 if (dev->status_bit) 00263 frame_bytes++; 00264 00265 dev->data_frame_byte = frame_bytes; 00266 00267 return frame_bytes; 00268 } 00269 00270 /** 00271 * Read conversion result from device. 00272 * @param dev - The device structure. 00273 * @param adc_data - The conversion result data 00274 * @param mode - Data read mode 00275 * Accepted values: AD77681_REGISTER_DATA_READ 00276 * AD77681_CONTINUOUS_DATA_READ 00277 * @return 0 in case of success, negative error code otherwise. 00278 */ 00279 int32_t ad77681_spi_read_adc_data(struct ad77681_dev *dev, 00280 uint8_t *adc_data, 00281 enum ad77681_data_read_mode mode) 00282 { 00283 uint8_t buf[6], crc_xor, add_buff; 00284 int32_t ret; 00285 00286 if (mode == AD77681_REGISTER_DATA_READ) { 00287 buf[0] = AD77681_REG_READ(AD77681_REG_ADC_DATA); 00288 add_buff = 1; 00289 } else { 00290 buf[0] = 0x00; 00291 add_buff = 0; 00292 } 00293 buf[1] = 0x00; /* added 2 more array places for max data length read */ 00294 buf[2] = 0x00; /* For register data read */ 00295 buf[3] = 0x00; /* register address + 3 bytes of data (24bit format) + Status bit + CRC */ 00296 buf[4] = 0x00; /* For continuous data read */ 00297 buf[5] = 0x00; /* 3 bytes of data (24bit format) + Status bit + CRC */ 00298 00299 00300 ret = spi_write_and_read(dev->spi_desc, buf, dev->data_frame_byte + add_buff); 00301 if (ret < 0) 00302 return ret; 00303 00304 if (dev->crc_sel != AD77681_NO_CRC) { 00305 if (dev->crc_sel == AD77681_CRC) 00306 crc_xor = ad77681_compute_crc8(buf + add_buff, dev->data_frame_byte - 1, 00307 INITIAL_CRC_CRC8); 00308 else 00309 crc_xor = ad77681_compute_xor(buf + add_buff, dev->data_frame_byte - 1, 00310 INITIAL_CRC_XOR); 00311 00312 if (crc_xor != buf[dev->data_frame_byte - (1 - add_buff)]) { 00313 printf("%s: CRC Error.\n", __func__); 00314 ret = FAILURE; 00315 } 00316 #ifdef CRC_DEBUG 00317 printf("\n%x\t%x\tCRC/XOR: %s\n", crc_xor, 00318 buf[dev->data_frame_byte - (1 - add_buff)], 00319 ((crc_xor != buf[dev->data_frame_byte - (1 - add_buff)]) ? "FAULT" : "OK")); 00320 #endif /* CRC_DEBUG */ 00321 } 00322 00323 /* Fill the adc_data buffer */ 00324 memcpy(adc_data, buf, ARRAY_SIZE(buf)); 00325 00326 return ret; 00327 } 00328 00329 /** 00330 * CRC and status bit handling after each readout form the ADC 00331 * @param dev - The device structure. 00332 * @param *data_buffer - 16-bit buffer readed from the ADC containing the CRC, 00333 * data and the stattus bit. 00334 * @return 0 in case of success, negative error code otherwise. 00335 */ 00336 int32_t ad77681_CRC_status_handling(struct ad77681_dev *dev, 00337 uint16_t *data_buffer) 00338 { 00339 int32_t ret = 0; 00340 uint8_t status_byte = 0, checksum = 0, checksum_byte = 0, checksum_buf[5], 00341 checksum_length = 0, i; 00342 char print_buf[50]; 00343 00344 /* Status bit handling */ 00345 if (dev->status_bit) { 00346 /* 24bit ADC data + 8bit of status = 2 16bit frames */ 00347 if (dev->conv_len == AD77681_CONV_24BIT) 00348 status_byte = data_buffer[1] & 0xFF; 00349 /* 16bit ADC data + 8bit of status = 2 16bit frames */ 00350 else 00351 status_byte = data_buffer[1] >> 8; 00352 } 00353 00354 /* Checksum bit handling */ 00355 if (dev->crc_sel != AD77681_NO_CRC) { 00356 if ((dev->status_bit == true) & (dev->conv_len == AD77681_CONV_24BIT)) { 00357 /* 24bit ADC data + 8bit of status + 8bit of CRC = 3 16bit frames */ 00358 checksum_byte = data_buffer[2] >> 8; 00359 checksum_length = 4; 00360 } else if ((dev->status_bit == true) & (dev->conv_len == AD77681_CONV_16BIT)) { 00361 /* 16bit ADC data + 8bit of status + 8bit of CRC = 2 16bit frames */ 00362 checksum_byte = data_buffer[1] & 0xFF; 00363 checksum_length = 3; 00364 } else if ((dev->status_bit == false) & (dev->conv_len == AD77681_CONV_24BIT)) { 00365 /* 24bit ADC data + 8bit of CRC = 2 16bit frames */ 00366 checksum_byte = data_buffer[1] & 0xFF; 00367 checksum_length = 3; 00368 } else if ((dev->status_bit == false) & (dev->conv_len == AD77681_CONV_16BIT)) { 00369 /* 16bit ADC data + 8bit of CRC = 2 16bit frames */ 00370 checksum_byte = data_buffer[1] >> 8; 00371 checksum_length = 2; 00372 } 00373 00374 for (i = 0; i < checksum_length; i++) { 00375 if (i % 2) 00376 checksum_buf[i] = data_buffer[i / 2] & 0xFF; 00377 else 00378 checksum_buf[i] = data_buffer[i / 2] >> 8; 00379 } 00380 00381 if (dev->crc_sel == AD77681_CRC) 00382 checksum = ad77681_compute_crc8(checksum_buf, checksum_length, 00383 INITIAL_CRC_CRC8); 00384 else if (dev->crc_sel == AD77681_XOR) 00385 checksum = ad77681_compute_xor(checksum_buf, checksum_length, INITIAL_CRC_XOR); 00386 00387 if (checksum != checksum_byte) 00388 ret = FAILURE; 00389 00390 #ifdef CRC_DEBUG 00391 00392 char ok[3] = { 'O', 'K' }, fault[6] = { 'F', 'A', 'U', 'L', 'T' }; 00393 sprintf(print_buf, "\n%x\t%x\t%x\tCRC %s", checksum_byte, checksum, status_byte, 00394 ((ret == FAILURE) ? (fault) : (ok))); 00395 printf(print_buf); 00396 00397 #endif /* CRC_DEBUG */ 00398 } 00399 00400 return ret; 00401 } 00402 00403 /** 00404 * Conversion from measured data to voltage 00405 * @param dev - The device structure. 00406 * @param raw_code - ADC raw code measurements 00407 * @param voltage - Converted ADC code to voltage 00408 * @return 0 in case of success, negative error code otherwise. 00409 */ 00410 int32_t ad77681_data_to_voltage(struct ad77681_dev *dev, 00411 uint32_t *raw_code, 00412 double *voltage) 00413 { 00414 int32_t converted_data; 00415 00416 if (*raw_code & 0x800000) 00417 converted_data = (int32_t)((0xFF << 24) | *raw_code); 00418 else 00419 converted_data = (int32_t)((0x00 << 24) | *raw_code); 00420 00421 /* ((2*Vref)*code)/2^24 */ 00422 *voltage = (double)(((2.0 * (((double)(dev->vref)) / 1000.0)) / 00423 AD7768_FULL_SCALE) * converted_data); 00424 00425 return SUCCESS; 00426 } 00427 00428 /** 00429 * Update ADCs sample rate depending on MCLK, MCLK_DIV and filter settings 00430 * @param dev - The device structure. 00431 * @return 0 in case of success, negative error code otherwise. 00432 */ 00433 int32_t ad77681_update_sample_rate(struct ad77681_dev *dev) 00434 { 00435 uint8_t mclk_div; 00436 uint16_t osr; 00437 00438 /* Finding out MCLK divider */ 00439 switch (dev->mclk_div) { 00440 case AD77681_MCLK_DIV_16: 00441 mclk_div = 16; 00442 break; 00443 case AD77681_MCLK_DIV_8: 00444 mclk_div = 8; 00445 break; 00446 case AD77681_MCLK_DIV_4: 00447 mclk_div = 4; 00448 break; 00449 case AD77681_MCLK_DIV_2: 00450 mclk_div = 2; 00451 break; 00452 default: 00453 return FAILURE; 00454 break; 00455 } 00456 00457 /* Finding out decimation ratio */ 00458 switch (dev->filter) { 00459 case (AD77681_SINC5 | AD77681_FIR): 00460 /* Decimation ratio of FIR or SINC5 (x32 to x1024) */ 00461 switch (dev->decimate) { 00462 case AD77681_SINC5_FIR_DECx32: 00463 osr = 32; 00464 break; 00465 case AD77681_SINC5_FIR_DECx64: 00466 osr = 64; 00467 break; 00468 case AD77681_SINC5_FIR_DECx128: 00469 osr = 128; 00470 break; 00471 case AD77681_SINC5_FIR_DECx256: 00472 osr = 256; 00473 break; 00474 case AD77681_SINC5_FIR_DECx512: 00475 osr = 512; 00476 break; 00477 case AD77681_SINC5_FIR_DECx1024: 00478 osr = 1024; 00479 break; 00480 default: 00481 return FAILURE; 00482 break; 00483 } 00484 break; 00485 /* Decimation ratio of SINC5 x8 */ 00486 case AD77681_SINC5_DECx8: 00487 osr = 8; 00488 break; 00489 /* Decimation ratio of SINC5 x16 */ 00490 case AD77681_SINC5_DECx16: 00491 osr = 16; 00492 break; 00493 /* Decimation ratio of SINC3 */ 00494 case AD77681_SINC3: 00495 osr = (dev->sinc3_osr + 1) * 32; 00496 break; 00497 default: 00498 return FAILURE; 00499 break; 00500 } 00501 00502 /* Sample rate to Hz */ 00503 dev->sample_rate = (dev->mclk / (osr*mclk_div)) * 1000; 00504 00505 return SUCCESS; 00506 } 00507 00508 /** 00509 * Get SINC3 filter oversampling ratio register value based on user's inserted 00510 * output data rate ODR 00511 * @param dev - The device structure. 00512 * @param sinc3_dec_reg - Returned closest value of SINC3 register 00513 * @param sinc3_odr - Desired output data rage 00514 * @return 0 in case of success, negative error code otherwise. 00515 */ 00516 int32_t ad77681_SINC3_ODR(struct ad77681_dev *dev, 00517 uint16_t *sinc3_dec_reg, 00518 float sinc3_odr) 00519 { 00520 uint8_t mclk_div; 00521 float odr; 00522 00523 if (sinc3_odr < 0) 00524 return FAILURE; 00525 00526 switch (dev->mclk_div) { 00527 case AD77681_MCLK_DIV_16: 00528 mclk_div = 16; 00529 break; 00530 case AD77681_MCLK_DIV_8: 00531 mclk_div = 8; 00532 break; 00533 case AD77681_MCLK_DIV_4: 00534 mclk_div = 4; 00535 break; 00536 case AD77681_MCLK_DIV_2: 00537 mclk_div = 2; 00538 break; 00539 default: 00540 return FAILURE; 00541 break; 00542 } 00543 00544 odr = ((float)(dev->mclk * 1000.0) / (sinc3_odr * (float)(32 * mclk_div))) - 1; 00545 00546 /* Sinc3 oversamplig register has 13 bits, biggest value = 8192 */ 00547 if (odr < 8193) 00548 *sinc3_dec_reg = (uint16_t)(odr); 00549 else 00550 return FAILURE; 00551 00552 return SUCCESS; 00553 } 00554 00555 /** 00556 * Set the power consumption mode of the ADC core. 00557 * @param dev - The device structure. 00558 * @param mode - The power mode. 00559 * Accepted values: AD77681_ECO 00560 * AD77681_MEDIAN 00561 * AD77681_FAST 00562 * @return 0 in case of success, negative error code otherwise. 00563 */ 00564 int32_t ad77681_set_power_mode(struct ad77681_dev *dev, 00565 enum ad77681_power_mode mode) 00566 { 00567 int32_t ret; 00568 00569 ret = ad77681_spi_write_mask(dev, 00570 AD77681_REG_POWER_CLOCK, 00571 AD77681_POWER_CLK_PWRMODE_MSK, 00572 AD77681_POWER_CLK_PWRMODE(mode)); 00573 00574 if (ret == SUCCESS) 00575 dev->power_mode = mode; 00576 00577 return ret; 00578 } 00579 00580 /** 00581 * Set the MCLK divider. 00582 * @param dev - The device structure. 00583 * @param clk_div - The MCLK divider. 00584 * Accepted values: AD77681_MCLK_DIV_16 00585 * AD77681_MCLK_DIV_8 00586 * AD77681_MCLK_DIV_4 00587 * AD77681_MCLK_DIV_2 00588 * @return 0 in case of success, negative error code otherwise. 00589 */ 00590 int32_t ad77681_set_mclk_div(struct ad77681_dev *dev, 00591 enum ad77681_mclk_div clk_div) 00592 { 00593 int32_t ret; 00594 00595 ret = ad77681_spi_write_mask(dev, 00596 AD77681_REG_POWER_CLOCK, 00597 AD77681_POWER_CLK_MCLK_DIV_MSK, 00598 AD77681_POWER_CLK_MCLK_DIV(clk_div)); 00599 00600 if (ret == SUCCESS) 00601 dev->mclk_div = clk_div; 00602 00603 return ret; 00604 } 00605 00606 /** 00607 * Set the VCM output. 00608 * @param dev - The device structure. 00609 * @param VCM_out - The VCM output voltage. 00610 * Accepted values: AD77681_VCM_HALF_VCC 00611 * AD77681_VCM_2_5V 00612 * AD77681_VCM_2_05V 00613 * AD77681_VCM_1_9V 00614 * AD77681_VCM_1_65V 00615 * AD77681_VCM_1_1V 00616 * AD77681_VCM_0_9V 00617 * AD77681_VCM_OFF 00618 * @return 0 in case of success, negative error code otherwise. 00619 */ 00620 int32_t ad77681_set_VCM_output(struct ad77681_dev *dev, 00621 enum ad77681_VCM_out VCM_out) 00622 { 00623 int32_t ret; 00624 00625 ret = ad77681_spi_write_mask(dev, 00626 AD77681_REG_ANALOG2, 00627 AD77681_ANALOG2_VCM_MSK, 00628 AD77681_ANALOG2_VCM(VCM_out)); 00629 00630 if (ret == SUCCESS) 00631 dev->VCM_out = VCM_out; 00632 00633 return ret; 00634 } 00635 00636 /** 00637 * Set the AIN- precharge buffer. 00638 * @param dev - The device structure. 00639 * @param AINn - The negative analog input precharge buffer selector 00640 * Accepted values: AD77681_AINn_ENABLED 00641 * AD77681_AINn_DISABLED 00642 * @return 0 in case of success, negative error code otherwise. 00643 */ 00644 int32_t ad77681_set_AINn_buffer(struct ad77681_dev *dev, 00645 enum ad77681_AINn_precharge AINn) 00646 { 00647 int32_t ret; 00648 00649 ret = ad77681_spi_write_mask(dev, 00650 AD77681_REG_ANALOG, 00651 AD77681_ANALOG_AIN_BUF_NEG_OFF_MSK, 00652 AD77681_ANALOG_AIN_BUF_NEG_OFF(AINn)); 00653 00654 if (ret == SUCCESS) 00655 dev->AINn = AINn; 00656 00657 return ret; 00658 } 00659 00660 /** 00661 * Set the AIN+ precharge buffer. 00662 * @param dev - The device structure. 00663 * @param AINp - The positive analog input precharge buffer selector 00664 * Accepted values: AD77681_AINp_ENABLED 00665 * AD77681_AINp_DISABLED 00666 * @return 0 in case of success, negative error code otherwise. 00667 */ 00668 int32_t ad77681_set_AINp_buffer(struct ad77681_dev *dev, 00669 enum ad77681_AINp_precharge AINp) 00670 { 00671 int32_t ret; 00672 00673 ret = ad77681_spi_write_mask(dev, 00674 AD77681_REG_ANALOG, 00675 AD77681_ANALOG_AIN_BUF_POS_OFF_MSK, 00676 AD77681_ANALOG_AIN_BUF_POS_OFF(AINp)); 00677 00678 if (ret == SUCCESS) 00679 dev->AINp = AINp; 00680 00681 return ret; 00682 } 00683 00684 /** 00685 * Set the REF- reference buffer 00686 * @param dev - The device structure. 00687 * @param REFn - The negative reference buffer selector 00688 * Accepted values: AD77681_BUFn_DISABLED 00689 * AD77681_BUFn_ENABLED 00690 * AD77681_BUFn_FULL_BUFFER_ON 00691 * @return 0 in case of success, negative error code otherwise. 00692 */ 00693 int32_t ad77681_set_REFn_buffer(struct ad77681_dev *dev, 00694 enum ad77681_REFn_buffer REFn) 00695 { 00696 int32_t ret; 00697 00698 ret = ad77681_spi_write_mask(dev, 00699 AD77681_REG_ANALOG, 00700 AD77681_ANALOG_REF_BUF_NEG_MSK, 00701 AD77681_ANALOG_REF_BUF_NEG(REFn)); 00702 00703 if (ret == SUCCESS) 00704 dev->REFn = REFn; 00705 00706 return ret; 00707 } 00708 00709 /** 00710 * Set the REF+ reference buffer 00711 * @param dev - The device structure. 00712 * @param REFp - The positive reference buffer selector 00713 * Accepted values: AD77681_BUFp_DISABLED 00714 * AD77681_BUFp_ENABLED 00715 * AD77681_BUFp_FULL_BUFFER_ON 00716 * @return 0 in case of success, negative error code otherwise. 00717 */ 00718 int32_t ad77681_set_REFp_buffer(struct ad77681_dev *dev, 00719 enum ad77681_REFp_buffer REFp) 00720 { 00721 int32_t ret; 00722 00723 ret = ad77681_spi_write_mask(dev, 00724 AD77681_REG_ANALOG, 00725 AD77681_ANALOG_REF_BUF_POS_MSK, 00726 AD77681_ANALOG_REF_BUF_POS(REFp)); 00727 00728 if (ret == SUCCESS) 00729 dev->REFp = REFp; 00730 else 00731 return FAILURE; 00732 00733 return ret; 00734 } 00735 00736 /** 00737 * Set filter type and decimation ratio 00738 * @param dev - The device structure. 00739 * @param decimate - Decimation ratio of filter 00740 * Accepted values: AD77681_SINC5_FIR_DECx32 00741 * AD77681_SINC5_FIR_DECx64 00742 * AD77681_SINC5_FIR_DECx128 00743 * AD77681_SINC5_FIR_DECx256 00744 * AD77681_SINC5_FIR_DECx512 00745 * AD77681_SINC5_FIR_DECx1024 00746 * @param filter - Select filter type 00747 * Accepted values: AD77681_SINC5 00748 * AD77681_SINC5_DECx8 00749 * AD77681_SINC5_DECx16 00750 * AD77681_SINC3 00751 * AD77681_FIR 00752 * @param sinc3_osr - Select decimation ratio for SINC3 filter separately as 00753 * integer from 0 to 8192. 00754 * See the AD7768-1 datasheet for more info 00755 * @return 0 in case of success, negative error code otherwise. 00756 */ 00757 int32_t ad77681_set_filter_type(struct ad77681_dev *dev, 00758 enum ad77681_sinc5_fir_decimate decimate, 00759 enum ad77681_filter_type filter, 00760 uint16_t sinc3_osr) 00761 { 00762 int32_t ret; 00763 00764 ret = ad77681_spi_reg_write(dev, AD77681_REG_DIGITAL_FILTER, 0x00); 00765 00766 /* SINC5 for OSR 8x and 16x*/ 00767 if ((filter == AD77681_SINC5_DECx8) || (filter == AD77681_SINC5_DECx16)) { 00768 ret |= ad77681_spi_write_mask(dev, 00769 AD77681_REG_DIGITAL_FILTER, 00770 AD77681_DIGI_FILTER_FILTER_MSK, 00771 AD77681_DIGI_FILTER_FILTER(filter)); 00772 /* SINC5 and FIR for osr 32x to 1024x */ 00773 } else if ((filter == AD77681_SINC5) || (filter == AD77681_FIR)) { 00774 ret |= ad77681_spi_write_mask(dev, 00775 AD77681_REG_DIGITAL_FILTER, 00776 AD77681_DIGI_FILTER_FILTER_MSK, 00777 AD77681_DIGI_FILTER_FILTER(filter)); 00778 00779 ret |= ad77681_spi_write_mask(dev, 00780 AD77681_REG_DIGITAL_FILTER, 00781 AD77681_DIGI_FILTER_DEC_RATE_MSK, 00782 AD77681_DIGI_FILTER_DEC_RATE(decimate)); 00783 /* SINC3*/ 00784 } else { 00785 uint8_t sinc3_LSB = 0, sinc3_MSB = 0; 00786 00787 sinc3_MSB = sinc3_osr >> 8; 00788 sinc3_LSB = sinc3_osr & 0x00FF; 00789 00790 ret |= ad77681_spi_write_mask(dev, 00791 AD77681_REG_DIGITAL_FILTER, 00792 AD77681_DIGI_FILTER_FILTER_MSK, 00793 AD77681_DIGI_FILTER_FILTER(filter)); 00794 00795 ret |= ad77681_spi_write_mask(dev, 00796 AD77681_REG_SINC3_DEC_RATE_MSB, 00797 AD77681_SINC3_DEC_RATE_MSB_MSK, 00798 AD77681_SINC3_DEC_RATE_MSB(sinc3_MSB)); 00799 00800 ret |= ad77681_spi_write_mask(dev, 00801 AD77681_REG_SINC3_DEC_RATE_LSB, 00802 AD77681_SINC3_DEC_RATE_LSB_MSK, 00803 AD77681_SINC3_DEC_RATE_LSB(sinc3_LSB)); 00804 } 00805 00806 if ( ret == SUCCESS) { 00807 dev->decimate = decimate; 00808 dev->filter = filter; 00809 /* Sync pulse after each filter change */ 00810 ret |= ad77681_initiate_sync(dev); 00811 } 00812 00813 return ret; 00814 } 00815 00816 /** 00817 * Enable 50/60 Hz rejection 00818 * @param dev - The device structure. 00819 * @param enable - The positive reference buffer selector 00820 * Accepted values: true 00821 * false 00822 * @return 0 in case of success, negative error code otherwise. 00823 */ 00824 int32_t ad77681_set_50HZ_rejection(struct ad77681_dev *dev, 00825 uint8_t enable) 00826 { 00827 int32_t ret; 00828 00829 ret = ad77681_spi_write_mask(dev, 00830 AD77681_REG_DIGITAL_FILTER, 00831 AD77681_DIGI_FILTER_60HZ_REJ_EN_MSK, 00832 AD77681_DIGI_FILTER_60HZ_REJ_EN(enable)); 00833 00834 return ret; 00835 } 00836 00837 /** 00838 * Set the REF- reference buffer 00839 * @param dev - The device structure. 00840 * @param continuous_enable - Continous read enable 00841 * Accepted values: AD77681_CONTINUOUS_READ_ENABLE 00842 * AD77681_CONTINUOUS_READ_DISABLE 00843 * @return 0 in case of success, negative error code otherwise. 00844 */ 00845 int32_t ad77681_set_continuos_read(struct ad77681_dev *dev, 00846 enum ad77681_continuous_read continuous_enable) 00847 { 00848 int32_t ret; 00849 00850 if (continuous_enable) { 00851 ret = ad77681_spi_write_mask(dev, 00852 AD77681_REG_INTERFACE_FORMAT, 00853 AD77681_INTERFACE_CONT_READ_MSK, 00854 AD77681_INTERFACE_CONT_READ_EN(continuous_enable)); 00855 } else { 00856 /* To exit the continuous read mode, a key 0x6C must be 00857 written into the device over the SPI*/ 00858 uint8_t end_key = EXIT_CONT_READ; 00859 ret = spi_write_and_read(dev->spi_desc, &end_key, 1); 00860 } 00861 00862 return ret; 00863 } 00864 00865 /** 00866 * Power down / power up the device 00867 * @param dev - The device structure. 00868 * @param sleep_wake - Power down, or power up the ADC 00869 * Accepted values: AD77681_SLEEP 00870 * AD77681_WAKE 00871 * @return 0 in case of success, negative error code otherwise. 00872 */ 00873 int32_t ad77681_power_down(struct ad77681_dev *dev, 00874 enum ad77681_sleep_wake sleep_wake) 00875 { 00876 int32_t ret; 00877 00878 if (sleep_wake == AD77681_SLEEP) { 00879 ret = ad77681_spi_reg_write(dev, AD77681_REG_POWER_CLOCK, 00880 AD77681_POWER_CLK_POWER_DOWN); 00881 } else { 00882 /* Wake up the ADC over SPI, by sending a wake-up sequence: 00883 1 followed by 63 zeroes and CS hold low*/ 00884 uint8_t wake_sequence[8] = { 0 }; 00885 /* Insert '1' to the beginning of the wake_sequence*/ 00886 wake_sequence[0] = 0x80; 00887 ret = spi_write_and_read(dev->spi_desc, wake_sequence, 00888 ARRAY_SIZE(wake_sequence)); 00889 } 00890 00891 return ret; 00892 } 00893 00894 /** 00895 * Conversion mode and source select 00896 * @param dev - The device structure. 00897 * @param conv_mode - Sets the conversion mode of the ADC 00898 * Accepted values: AD77681_CONV_CONTINUOUS 00899 * AD77681_CONV_ONE_SHOT 00900 * AD77681_CONV_SINGLE 00901 * AD77681_CONV_PERIODIC 00902 * @param diag_mux_sel - Selects which signal to route through diagnostic mux 00903 * Accepted values: AD77681_TEMP_SENSOR 00904 * AD77681_AIN_SHORT 00905 * AD77681_POSITIVE_FS 00906 * AD77681_NEGATIVE_FS 00907 * @param conv_diag_sel - Select the input for conversion as AIN or diagnostic mux 00908 * Accepted values: true 00909 * false 00910 * @return 0 in case of success, negative error code otherwise. 00911 */ 00912 int32_t ad77681_set_conv_mode(struct ad77681_dev *dev, 00913 enum ad77681_conv_mode conv_mode, 00914 enum ad77681_conv_diag_mux diag_mux_sel, 00915 bool conv_diag_sel) 00916 { 00917 int32_t ret; 00918 00919 ret = ad77681_spi_write_mask(dev, 00920 AD77681_REG_CONVERSION, 00921 AD77681_CONVERSION_MODE_MSK, 00922 AD77681_CONVERSION_MODE(conv_mode)); 00923 00924 ret |= ad77681_spi_write_mask(dev, 00925 AD77681_REG_CONVERSION, 00926 AD77681_CONVERSION_DIAG_MUX_MSK, 00927 AD77681_CONVERSION_DIAG_MUX_SEL(diag_mux_sel)); 00928 00929 ret |= ad77681_spi_write_mask(dev, 00930 AD77681_REG_CONVERSION, 00931 AD77681_CONVERSION_DIAG_SEL_MSK, 00932 AD77681_CONVERSION_DIAG_SEL(conv_diag_sel)); 00933 00934 if (ret == SUCCESS) { 00935 dev->conv_mode = conv_mode; 00936 dev->diag_mux_sel = diag_mux_sel; 00937 dev->conv_diag_sel = conv_diag_sel; 00938 } 00939 00940 return ret; 00941 } 00942 00943 /** 00944 * Set the Conversion Result Output Length. 00945 * @param dev - The device structure. 00946 * @param conv_len - The MCLK divider. 00947 * Accepted values: AD77681_CONV_24BIT 00948 * AD77681_CONV_16BIT 00949 * @return 0 in case of success, negative error code otherwise. 00950 */ 00951 int32_t ad77681_set_convlen(struct ad77681_dev *dev, 00952 enum ad77681_conv_len conv_len) 00953 { 00954 int32_t ret; 00955 00956 ret = ad77681_spi_write_mask(dev, 00957 AD77681_REG_INTERFACE_FORMAT, 00958 AD77681_INTERFACE_CONVLEN_MSK, 00959 AD77681_INTERFACE_CONVLEN(conv_len)); 00960 00961 if (ret == SUCCESS) { 00962 dev->conv_len = conv_len; 00963 ad77681_get_frame_byte(dev); 00964 } 00965 00966 return ret; 00967 } 00968 00969 /** 00970 * Activates CRC on all SPI transactions and 00971 * Selects CRC method as XOR or 8-bit polynomial 00972 * @param dev - The device structure. 00973 * @param crc_sel - The CRC type. 00974 * Accepted values: AD77681_CRC 00975 * AD77681_XOR 00976 * AD77681_NO_CRC 00977 * @return 0 in case of success, negative error code otherwise. 00978 */ 00979 int32_t ad77681_set_crc_sel(struct ad77681_dev *dev, 00980 enum ad77681_crc_sel crc_sel) 00981 { 00982 int32_t ret; 00983 00984 if (crc_sel == AD77681_NO_CRC) { 00985 ret = ad77681_spi_write_mask(dev, 00986 AD77681_REG_INTERFACE_FORMAT, 00987 AD77681_INTERFACE_CRC_EN_MSK, 00988 AD77681_INTERFACE_CRC_EN(0)); 00989 } else { 00990 ret = ad77681_spi_write_mask(dev, 00991 AD77681_REG_INTERFACE_FORMAT, 00992 AD77681_INTERFACE_CRC_EN_MSK, 00993 AD77681_INTERFACE_CRC_EN(1)); 00994 00995 ret |= ad77681_spi_write_mask(dev, 00996 AD77681_REG_INTERFACE_FORMAT, 00997 AD77681_INTERFACE_CRC_TYPE_MSK, 00998 AD77681_INTERFACE_CRC_TYPE(crc_sel)); 00999 } 01000 01001 if (ret == SUCCESS) { 01002 dev->crc_sel = crc_sel; 01003 ad77681_get_frame_byte(dev); 01004 } 01005 01006 return ret; 01007 } 01008 01009 /** 01010 * Enables Status bits output 01011 * @param dev - The device structure. 01012 * @param status_bit - enable or disable status bit 01013 * Accepted values: true 01014 * false 01015 * @return 0 in case of success, negative error code otherwise. 01016 */ 01017 int32_t ad77681_set_status_bit(struct ad77681_dev *dev, 01018 bool status_bit) 01019 { 01020 int32_t ret; 01021 01022 // Set status bit 01023 ret = ad77681_spi_write_mask(dev, 01024 AD77681_REG_INTERFACE_FORMAT, 01025 AD77681_INTERFACE_STATUS_EN_MSK, 01026 AD77681_INTERFACE_STATUS_EN(status_bit)); 01027 01028 if (ret == SUCCESS) { 01029 dev->status_bit = status_bit; 01030 ad77681_get_frame_byte(dev); 01031 } 01032 01033 return ret; 01034 } 01035 01036 /** 01037 * Device reset over SPI. 01038 * @param dev - The device structure. 01039 * @return 0 in case of success, negative error code otherwise. 01040 */ 01041 int32_t ad77681_soft_reset(struct ad77681_dev *dev) 01042 { 01043 int32_t ret = 0; 01044 01045 // Two writes are required to initialize the reset 01046 ret |= ad77681_spi_write_mask(dev, 01047 AD77681_REG_SYNC_RESET, 01048 AD77681_SYNC_RST_SPI_RESET_MSK, 01049 AD77681_SYNC_RST_SPI_RESET(0x3)); 01050 01051 ret |= ad77681_spi_write_mask(dev, 01052 AD77681_REG_SYNC_RESET, 01053 AD77681_SYNC_RST_SPI_RESET_MSK, 01054 AD77681_SYNC_RST_SPI_RESET(0x2)); 01055 01056 return ret; 01057 } 01058 01059 /** 01060 * Initiate a SYNC_OUT pulse over spi 01061 * @param dev - The device structure. 01062 * @return 0 in case of success, negative error code otherwise. 01063 */ 01064 int32_t ad77681_initiate_sync(struct ad77681_dev *dev) 01065 { 01066 return ad77681_spi_write_mask(dev, 01067 AD77681_REG_SYNC_RESET, 01068 AD77681_SYNC_RST_SPI_STARTB_MSK, 01069 AD77681_SYNC_RST_SPI_STARTB(0)); 01070 } 01071 01072 /** 01073 * Write to offset registers 01074 * @param dev The device structure. 01075 * @param value The desired value of the whole 24-bit offset register 01076 * @return 0 in case of success, negative error code otherwise. 01077 */ 01078 int32_t ad77681_apply_offset(struct ad77681_dev *dev, 01079 uint32_t value) 01080 { 01081 int32_t ret; 01082 uint8_t offset_HI = 0, offset_MID = 0, offset_LO = 0; 01083 01084 offset_HI = (value & 0x00FF0000) >> 16; 01085 offset_MID = (value & 0x0000FF00) >> 8; 01086 offset_LO = (value & 0x000000FF); 01087 01088 ret = ad77681_spi_write_mask(dev, 01089 AD77681_REG_OFFSET_HI, 01090 AD77681_OFFSET_HI_MSK, 01091 AD77681_OFFSET_HI(offset_HI)); 01092 01093 ret |= ad77681_spi_write_mask(dev, 01094 AD77681_REG_OFFSET_MID, 01095 AD77681_OFFSET_MID_MSK, 01096 AD77681_OFFSET_MID(offset_MID)); 01097 01098 ret |= ad77681_spi_write_mask(dev, 01099 AD77681_REG_OFFSET_LO, 01100 AD77681_OFFSET_LO_MSK, 01101 AD77681_OFFSET_LO(offset_LO)); 01102 01103 return ret; 01104 } 01105 01106 /** 01107 * Write to gain registers 01108 * @param dev - The device structure. 01109 * @param value - The desired value of the whole 24-bit gain register 01110 * @return 0 in case of success, negative error code otherwise. 01111 */ 01112 int32_t ad77681_apply_gain(struct ad77681_dev *dev, 01113 uint32_t value) 01114 { 01115 int32_t ret; 01116 uint8_t gain_HI = 0, gain_MID = 0, gain_LO = 0; 01117 01118 gain_HI = (value & 0x00FF0000) >> 16; 01119 gain_MID = (value & 0x0000FF00) >> 8; 01120 gain_LO = (value & 0x000000FF); 01121 01122 ret = ad77681_spi_write_mask(dev, 01123 AD77681_REG_GAIN_HI, 01124 AD77681_GAIN_HI_MSK, 01125 AD77681_GAIN_HI(gain_HI)); 01126 01127 ret |= ad77681_spi_write_mask(dev, 01128 AD77681_REG_GAIN_MID, 01129 AD77681_GAIN_MID_MSK, 01130 AD77681_GAIN_MID(gain_MID)); 01131 01132 ret |= ad77681_spi_write_mask(dev, 01133 AD77681_REG_GAIN_LO, 01134 AD77681_GAIN_LOW_MSK, 01135 AD77681_GAIN_LOW(gain_LO)); 01136 01137 return ret; 01138 } 01139 01140 /** 01141 * Upload sequence for Programmamble FIR filter 01142 * @param dev - The device structure. 01143 * @param coeffs - Pointer to the desired filter coefficients array to be written 01144 * @param num_coeffs - Count of active filter coeffs 01145 * @return 0 in case of success, negative error code otherwise. 01146 */ 01147 int32_t ad77681_programmable_filter(struct ad77681_dev *dev, 01148 const float *coeffs, 01149 uint8_t num_coeffs) 01150 { 01151 uint8_t coeffs_buf[4], coeffs_index, check_back = 0, i, address; 01152 uint32_t twait; 01153 int32_t twos_complement, ret; 01154 const uint8_t coeff_reg_length = 56; 01155 01156 /* Specific keys in the upload sequence */ 01157 const uint8_t key1 = 0xAC, key2 = 0x45, key3 = 0x55; 01158 /* Scaling factor for all coefficients 2^22 */ 01159 const float coeff_scale_factor = (1 << 22); 01160 /* Wait time in uS necessary to access the COEFF_CONTROL and */ 01161 /* COEFF_DATA registers. Twait = 512/MCLK */ 01162 twait = (uint32_t)(((512.0) / ((float)(dev->mclk))) * 1000.0) + 1; 01163 01164 /* Set Filter to FIR */ 01165 ret = ad77681_spi_write_mask(dev, 01166 AD77681_REG_DIGITAL_FILTER, 01167 AD77681_DIGI_FILTER_FILTER_MSK, 01168 AD77681_DIGI_FILTER_FILTER(AD77681_FIR)); 01169 01170 /* Check return value before proceeding */ 01171 if (ret < 0) 01172 return ret; 01173 01174 /* Write the first access key to the ACCESS_KEY register */ 01175 ret = ad77681_spi_write_mask(dev, 01176 AD77681_REG_ACCESS_KEY, 01177 AD77681_ACCESS_KEY_MSK, 01178 AD77681_ACCESS_KEY(key1)); 01179 01180 /* Check return value before proceeding */ 01181 if (ret < 0) 01182 return ret; 01183 01184 /* Write the second access key to the ACCESS_KEY register */ 01185 ret = ad77681_spi_write_mask(dev, 01186 AD77681_REG_ACCESS_KEY, 01187 AD77681_ACCESS_KEY_MSK, 01188 AD77681_ACCESS_KEY(key2)); 01189 01190 /* Check return value before proceeding */ 01191 if (ret < 0) 01192 return ret; 01193 01194 /* Read the the ACCESS_KEY register bit 0, the key bit */ 01195 ret = ad77681_spi_read_mask(dev, 01196 AD77681_REG_ACCESS_KEY, 01197 AD77681_ACCESS_KEY_CHECK_MSK, 01198 &check_back); 01199 01200 /* Checks ret and key bit, return FAILURE in case key bit not equal to 1 */ 01201 if ((ret < 0) || (check_back != 1)) 01202 return FAILURE; 01203 01204 /* Set the initial adress to 0 and enable the write and coefficient access bits */ 01205 address = AD77681_COEF_CONTROL_COEFFACCESSEN_MSK 01206 | AD77681_COEF_CONTROL_COEFFWRITEEN_MSK; 01207 01208 /* The COEFF_DATA register has to be filled with 56 coeffs.*/ 01209 /* In case the number of active filter coefficient is less */ 01210 /* than 56, zeros will be padded before the desired coeff. */ 01211 for (i = 0; i < coeff_reg_length; i++) { 01212 /* Set the coeff address */ 01213 ret = ad77681_spi_reg_write(dev, 01214 AD77681_REG_COEFF_CONTROL, 01215 address); 01216 01217 /* Check return value before proceeding */ 01218 if (ret < 0) 01219 return ret; 01220 01221 /* Wait for Twait uSeconds*/ 01222 udelay(twait); 01223 01224 /* Padding of zeros before the desired coef in case the coef count in less than 56 */ 01225 if((num_coeffs + i) < coeff_reg_length) { 01226 /* wirte zeroes to COEFF_DATA, in case of less coeffs than 56*/ 01227 coeffs_buf[0] = AD77681_REG_WRITE(AD77681_REG_COEFF_DATA); 01228 coeffs_buf[1] = 0; 01229 coeffs_buf[2] = 0; 01230 coeffs_buf[3] = 0; 01231 } else {/* Writting of desired filter coefficients */ 01232 /* Computes the index of coefficients to be uploaded */ 01233 coeffs_index = (num_coeffs + i) - coeff_reg_length; 01234 /* Scaling the coefficient value and converting it to 2's complement */ 01235 twos_complement = (int32_t)(coeffs[coeffs_index] * coeff_scale_factor); 01236 01237 /* Write coefficients to COEFF_DATA */ 01238 coeffs_buf[0] = AD77681_REG_WRITE(AD77681_REG_COEFF_DATA); 01239 coeffs_buf[1] = (twos_complement & 0xFF0000) >> 16; 01240 coeffs_buf[2] = (twos_complement & 0x00FF00) >> 8; 01241 coeffs_buf[3] = (twos_complement & 0x0000FF); 01242 } 01243 01244 ret = spi_write_and_read(dev->spi_desc, coeffs_buf, 4); 01245 01246 /* Check return value before proceeding */ 01247 if (ret < 0) 01248 return ret; 01249 01250 /* Increment the address*/ 01251 address++; 01252 /* Wait for Twait uSeconds*/ 01253 udelay(twait); 01254 } 01255 01256 /* Disable coefficient write */ 01257 ret = ad77681_spi_write_mask(dev, 01258 AD77681_REG_COEFF_CONTROL, 01259 AD77681_COEF_CONTROL_COEFFWRITEEN_MSK, 01260 AD77681_COEF_CONTROL_COEFFWRITEEN(0x00)); 01261 01262 /* Check return value before proceeding */ 01263 if (ret < 0) 01264 return ret; 01265 01266 udelay(twait); 01267 01268 /* Disable coefficient access */ 01269 ret = ad77681_spi_write_mask(dev, 01270 AD77681_REG_COEFF_CONTROL, 01271 AD77681_COEF_CONTROL_COEFFACCESSEN_MSK, 01272 AD77681_COEF_CONTROL_COEFFACCESSEN(0x00)); 01273 01274 /* Check return value before proceeding */ 01275 if (ret < 0) 01276 return ret; 01277 01278 /* Toggle the synchronization pulse and to begin reading data */ 01279 /* Write 0x800000 to COEFF_DATA */ 01280 coeffs_buf[0] = AD77681_REG_WRITE(AD77681_REG_COEFF_DATA); 01281 coeffs_buf[1] = 0x80; 01282 coeffs_buf[2] = 0x00; 01283 coeffs_buf[3] = 0x00; 01284 01285 ret = spi_write_and_read(dev->spi_desc, coeffs_buf, 4); 01286 01287 /* Check return value before proceeding */ 01288 if (ret < 0) 01289 return ret; 01290 01291 /* Exit filter upload by wirting specific access key 0x55*/ 01292 ret = ad77681_spi_write_mask(dev, 01293 AD77681_REG_ACCESS_KEY, 01294 AD77681_ACCESS_KEY_MSK, 01295 AD77681_ACCESS_KEY(key3)); 01296 01297 /* Check return value before proceeding */ 01298 if (ret < 0) 01299 return ret; 01300 01301 /* Send synchronization pulse */ 01302 ad77681_initiate_sync(dev); 01303 01304 return ret; 01305 } 01306 01307 /** 01308 * Read value from GPIOs present in AD7768-1 separately, or all GPIOS at once. 01309 * @param dev - The device structure. 01310 * @param value - Readed value. 01311 * @param gpio_number - Number of GPIO, the value will be written into 01312 * Accepted values: AD77681_GPIO0 01313 * AD77681_GPIO1 01314 * AD77681_GPIO2 01315 * AD77681_GPIO3 01316 * AD77681_ALL_GPIOS 01317 * @return 0 in case of success, negative error code otherwise. 01318 */ 01319 int32_t ad77681_gpio_read(struct ad77681_dev *dev, 01320 uint8_t *value, 01321 enum ad77681_gpios gpio_number) 01322 { 01323 int32_t ret; 01324 01325 switch (gpio_number) { 01326 case AD77681_GPIO0: /* Read to GPIO0 */ 01327 ret = ad77681_spi_read_mask(dev, 01328 AD77681_REG_GPIO_READ, 01329 AD77681_GPIO_READ_0_MSK, 01330 value); 01331 break; 01332 case AD77681_GPIO1: /* Read to GPIO1 */ 01333 ret = ad77681_spi_read_mask(dev, 01334 AD77681_REG_GPIO_READ, 01335 AD77681_GPIO_READ_1_MSK, 01336 value); 01337 break; 01338 case AD77681_GPIO2: /* Read to GPIO2 */ 01339 ret = ad77681_spi_read_mask(dev, 01340 AD77681_REG_GPIO_READ, 01341 AD77681_GPIO_READ_2_MSK, 01342 value); 01343 break; 01344 case AD77681_GPIO3: /* Read to GPIO3 */ 01345 ret = ad77681_spi_read_mask(dev, 01346 AD77681_REG_GPIO_READ, 01347 AD77681_GPIO_READ_3_MSK, 01348 value); 01349 break; 01350 case AD77681_ALL_GPIOS: /* Read to all GPIOs */ 01351 ret = ad77681_spi_read_mask(dev, 01352 AD77681_REG_GPIO_READ, 01353 AD77681_GPIO_READ_ALL_MSK, 01354 value); 01355 break; 01356 default: 01357 return FAILURE; 01358 break; 01359 } 01360 01361 return ret; 01362 } 01363 01364 /** 01365 * Write value to GPIOs present in AD7768-1 separately, or all GPIOS at once. 01366 * @param dev - The device structure. 01367 * @param value - Value to be written into GPIO 01368 * Accepted values: GPIO_HIGH 01369 * GPIO_LOW 01370 * 4-bit value for all gpios 01371 * @param gpio_number - Number of GPIO, the value will be written into 01372 * Accepted values: AD77681_GPIO0 01373 * AD77681_GPIO1 01374 * AD77681_GPIO2 01375 * AD77681_GPIO3 01376 * AD77681_ALL_GPIOS 01377 * @return 0 in case of success, negative error code otherwise. 01378 */ 01379 int32_t ad77681_gpio_write(struct ad77681_dev *dev, 01380 uint8_t value, 01381 enum ad77681_gpios gpio_number) 01382 { 01383 int32_t ret; 01384 01385 switch (gpio_number) { 01386 case AD77681_GPIO0: /* Write to GPIO0 */ 01387 ret = ad77681_spi_write_mask(dev, 01388 AD77681_REG_GPIO_WRITE, 01389 AD77681_GPIO_WRITE_0_MSK, 01390 AD77681_GPIO_WRITE_0(value)); 01391 break; 01392 case AD77681_GPIO1: /* Write to GPIO1 */ 01393 ret = ad77681_spi_write_mask(dev, 01394 AD77681_REG_GPIO_WRITE, 01395 AD77681_GPIO_WRITE_1_MSK, 01396 AD77681_GPIO_WRITE_1(value)); 01397 break; 01398 case AD77681_GPIO2: /* Write to GPIO2 */ 01399 ret = ad77681_spi_write_mask(dev, 01400 AD77681_REG_GPIO_WRITE, 01401 AD77681_GPIO_WRITE_2_MSK, 01402 AD77681_GPIO_WRITE_2(value)); 01403 break; 01404 case AD77681_GPIO3: /* Write to GPIO3 */ 01405 ret = ad77681_spi_write_mask(dev, 01406 AD77681_REG_GPIO_WRITE, 01407 AD77681_GPIO_WRITE_3_MSK, 01408 AD77681_GPIO_WRITE_3(value)); 01409 break; 01410 case AD77681_ALL_GPIOS: /* Write to all GPIOs */ 01411 ret = ad77681_spi_write_mask(dev, 01412 AD77681_REG_GPIO_WRITE, 01413 AD77681_GPIO_WRITE_ALL_MSK, 01414 AD77681_GPIO_WRITE_ALL(value)); 01415 break; 01416 default: 01417 return FAILURE; 01418 break; 01419 } 01420 01421 return ret; 01422 } 01423 01424 /** 01425 * Set AD7768-1s GPIO as input or output. 01426 * @param dev - The device structure. 01427 * @param direction - Direction of the GPIO 01428 * Accepted values: GPIO_INPUT 01429 * GPIO_OUTPUT 01430 * 4-bit value for all gpios 01431 * @param gpio_number - Number of GPIO, which will be affected 01432 * Accepted values: AD77681_GPIO0 01433 * AD77681_GPIO1 01434 * AD77681_GPIO2 01435 * AD77681_GPIO3 01436 * AD77681_ALL_GPIOS 01437 * @return 0 in case of success, negative error code otherwise. 01438 */ 01439 int32_t ad77681_gpio_inout(struct ad77681_dev *dev, 01440 uint8_t direction, 01441 enum ad77681_gpios gpio_number) 01442 { 01443 int32_t ret; 01444 01445 switch (gpio_number) { 01446 case AD77681_GPIO0: /* Set direction of GPIO0 */ 01447 ret = ad77681_spi_write_mask(dev, 01448 AD77681_REG_GPIO_CONTROL, 01449 AD77681_GPIO_CNTRL_GPIO0_OP_EN_MSK, 01450 AD77681_GPIO_CNTRL_GPIO0_OP_EN(direction)); 01451 break; 01452 case AD77681_GPIO1: /* Set direction of GPIO1 */ 01453 ret = ad77681_spi_write_mask(dev, 01454 AD77681_REG_GPIO_CONTROL, 01455 AD77681_GPIO_CNTRL_GPIO1_OP_EN_MSK, 01456 AD77681_GPIO_CNTRL_GPIO1_OP_EN(direction)); 01457 break; 01458 case AD77681_GPIO2: /* Set direction of GPIO2 */ 01459 ret = ad77681_spi_write_mask(dev, 01460 AD77681_REG_GPIO_CONTROL, 01461 AD77681_GPIO_CNTRL_GPIO2_OP_EN_MSK, 01462 AD77681_GPIO_CNTRL_GPIO2_OP_EN(direction)); 01463 break; 01464 case AD77681_GPIO3: /* Set direction of GPIO3 */ 01465 ret = ad77681_spi_write_mask(dev, 01466 AD77681_REG_GPIO_CONTROL, 01467 AD77681_GPIO_CNTRL_GPIO3_OP_EN_MSK, 01468 AD77681_GPIO_CNTRL_GPIO3_OP_EN(direction)); 01469 break; 01470 case AD77681_ALL_GPIOS: /* Set direction of all GPIOs */ 01471 ret = ad77681_spi_write_mask(dev, 01472 AD77681_REG_GPIO_CONTROL, 01473 AD77681_GPIO_CNTRL_ALL_GPIOS_OP_EN_MSK, 01474 AD77681_GPIO_CNTRL_ALL_GPIOS_OP_EN(direction)); 01475 break; 01476 default: 01477 return FAILURE; 01478 break; 01479 } 01480 01481 return ret; 01482 } 01483 01484 /** 01485 * Enable global GPIO bit. 01486 * This bit must be set high to change GPIO settings. 01487 * @param dev - The device structure. 01488 * @param gpio_enable - Enable or diable the global GPIO pin 01489 * Accepted values: AD77681_GLOBAL_GPIO_ENABLE 01490 * AD77681_GLOBAL_GPIO_DISABLE 01491 * @return 0 in case of success, negative error code otherwise. 01492 */ 01493 int32_t ad77681_global_gpio(struct ad77681_dev *dev, 01494 enum ad77681_gobal_gpio_enable gpio_enable) 01495 { 01496 return ad77681_spi_write_mask(dev, 01497 AD77681_REG_GPIO_CONTROL, 01498 AD77681_GPIO_CNTRL_UGPIO_EN_MSK, 01499 AD77681_GPIO_CNTRL_UGPIO_EN(gpio_enable)); 01500 } 01501 01502 /** 01503 * Read and write from ADC scratchpad register to check SPI Communication in 01504 * the very beginning, during inicialization. 01505 * @param dev - The device structure. 01506 * @param sequence - The sequence which will be written into scratchpad and the 01507 * readed sequence will be returned 01508 * @return 0 in case of success, negative error code otherwise. 01509 */ 01510 int32_t ad77681_scratchpad(struct ad77681_dev *dev, 01511 uint8_t *sequence) 01512 { 01513 int32_t ret; 01514 const uint8_t check = *sequence;/* Save the original sequence */ 01515 uint8_t ret_sequence = 0;/* Return sequence */ 01516 01517 ret = ad77681_spi_write_mask(dev, 01518 AD77681_REG_SCRATCH_PAD, 01519 AD77681_SCRATCHPAD_MSK, 01520 AD77681_SCRATCHPAD(check)); 01521 01522 ret |= ad77681_spi_read_mask(dev, 01523 AD77681_REG_SCRATCH_PAD, 01524 AD77681_SCRATCHPAD_MSK, 01525 &ret_sequence); 01526 01527 if (check != ret_sequence)/* Compare original an returned sequence */ 01528 return FAILURE; 01529 01530 return ret; 01531 } 01532 01533 /** 01534 * Set AD7768-1s GPIO output type between strong driver and open drain. 01535 * GPIO3 can not be accessed! 01536 * @param dev - The device structure. 01537 * @param gpio_number - AD7768-1s GPIO to be affected (Only GPIO0, GPIO1 and GPIO2) 01538 * Accepted values: AD77681_GPIO0 01539 * AD77681_GPIO1 01540 * AD77681_GPIO2 01541 * AD77681_ALL_GPIOS 01542 * 01543 * @param output_type - Output type of the GPIO 01544 * Accepted values: AD77681_GPIO_STRONG_DRIVER 01545 * AD77681_GPIO_OPEN_DRAIN 01546 * @return 0 in case of success, negative error code otherwise. 01547 */ 01548 int32_t ad77681_gpio_open_drain(struct ad77681_dev *dev, 01549 enum ad77681_gpios gpio_number, 01550 enum ad77681_gpio_output_type output_type) 01551 { 01552 int32_t ret; 01553 01554 switch (gpio_number) { 01555 case AD77681_GPIO0: /* Set ouptut type of GPIO0 */ 01556 ret = ad77681_spi_write_mask(dev, 01557 AD77681_REG_GPIO_CONTROL, 01558 AD77681_GPIO_CNTRL_GPIO0_OD_EN_MSK, 01559 AD77681_GPIO_CNTRL_GPIO0_OD_EN(output_type)); 01560 break; 01561 case AD77681_GPIO1: /* Set ouptut type of GPIO1 */ 01562 ret = ad77681_spi_write_mask(dev, 01563 AD77681_REG_GPIO_CONTROL, 01564 AD77681_GPIO_CNTRL_GPIO1_OD_EN_MSK, 01565 AD77681_GPIO_CNTRL_GPIO1_OD_EN(output_type)); 01566 break; 01567 case AD77681_GPIO2: /* Set ouptut type of GPIO2 */ 01568 ret = ad77681_spi_write_mask(dev, 01569 AD77681_REG_GPIO_CONTROL, 01570 AD77681_GPIO_CNTRL_GPIO2_OD_EN_MSK, 01571 AD77681_GPIO_CNTRL_GPIO2_OD_EN(output_type)); 01572 break; 01573 case AD77681_ALL_GPIOS: /* Set ouptut type of all GPIOs */ 01574 ret = ad77681_spi_write_mask(dev, 01575 AD77681_REG_GPIO_CONTROL, 01576 AD77681_GPIO_CNTRL_ALL_GPIOS_OD_EN_MSK, 01577 AD77681_GPIO_CNTRL_ALL_GPIOS_OD_EN(output_type)); 01578 break; 01579 default: 01580 return FAILURE; 01581 break; 01582 } 01583 01584 return ret; 01585 } 01586 01587 /** 01588 * Clear all error flags at once 01589 * @param dev - The device structure. 01590 * @return 0 in case of success, negative error code otherwise. 01591 */ 01592 int32_t ad77681_clear_error_flags(struct ad77681_dev *dev) 01593 { 01594 int32_t ret; 01595 01596 /* SPI ignore error CLEAR */ 01597 ret = ad77681_spi_write_mask(dev, 01598 AD77681_REG_SPI_DIAG_STATUS, 01599 AD77681_SPI_IGNORE_ERROR_MSK, 01600 AD77681_SPI_IGNORE_ERROR_CLR(ENABLE)); 01601 /* SPI read error CLEAR */ 01602 ret |= ad77681_spi_write_mask(dev, 01603 AD77681_REG_SPI_DIAG_STATUS, 01604 AD77681_SPI_READ_ERROR_MSK, 01605 AD77681_SPI_READ_ERROR_CLR(ENABLE)); 01606 /* SPI write error CLEAR */ 01607 ret |= ad77681_spi_write_mask(dev, 01608 AD77681_REG_SPI_DIAG_STATUS, 01609 AD77681_SPI_WRITE_ERROR_MSK, 01610 AD77681_SPI_WRITE_ERROR_CLR(ENABLE)); 01611 /* SPI CRC error CLEAR */ 01612 ret |= ad77681_spi_write_mask(dev, 01613 AD77681_REG_SPI_DIAG_STATUS, 01614 AD77681_SPI_CRC_ERROR_MSK, 01615 AD77681_SPI_CRC_ERROR_CLR(ENABLE)); 01616 01617 return ret; 01618 } 01619 01620 /** 01621 * Enabling error flags. All error flags enabled by default 01622 * @param dev - The device structure. 01623 * @return 0 in case of success, negative error code otherwise. 01624 */ 01625 int32_t ad77681_error_flags_enabe(struct ad77681_dev *dev) 01626 { 01627 int32_t ret; 01628 01629 /* SPI ERRORS ENABLE */ 01630 /* SPI ignore error enable */ 01631 ret = ad77681_spi_write_mask(dev, 01632 AD77681_REG_SPI_DIAG_ENABLE, 01633 AD77681_SPI_DIAG_ERR_SPI_IGNORE_MSK, 01634 AD77681_SPI_DIAG_ERR_SPI_IGNORE(ENABLE)); 01635 /* SPI Clock count error enable */ 01636 ret |= ad77681_spi_write_mask(dev, 01637 AD77681_REG_SPI_DIAG_ENABLE, 01638 AD77681_SPI_DIAG_ERR_SPI_CLK_CNT_MSK, 01639 AD77681_SPI_DIAG_ERR_SPI_CLK_CNT(ENABLE)); 01640 /* SPI Read error enable */ 01641 ret |= ad77681_spi_write_mask(dev, 01642 AD77681_REG_SPI_DIAG_ENABLE, 01643 AD77681_SPI_DIAG_ERR_SPI_RD_MSK, 01644 AD77681_SPI_DIAG_ERR_SPI_RD(ENABLE)); 01645 /* SPI Write error enable */ 01646 ret |= ad77681_spi_write_mask(dev, 01647 AD77681_REG_SPI_DIAG_ENABLE, 01648 AD77681_SPI_DIAG_ERR_SPI_WR_MSK, 01649 AD77681_SPI_DIAG_ERR_SPI_WR(ENABLE)); 01650 01651 /* ADC DIAG ERRORS ENABLE */ 01652 /* DLDO PSM error enable */ 01653 ret |= ad77681_spi_write_mask(dev, 01654 AD77681_REG_ADC_DIAG_ENABLE, 01655 AD77681_ADC_DIAG_ERR_DLDO_PSM_MSK, 01656 AD77681_ADC_DIAG_ERR_DLDO_PSM(ENABLE)); 01657 /* ALDO PSM error enable */ 01658 ret |= ad77681_spi_write_mask(dev, 01659 AD77681_REG_ADC_DIAG_ENABLE, 01660 AD77681_ADC_DIAG_ERR_ALDO_PSM_MSK, 01661 AD77681_ADC_DIAG_ERR_ALDO_PSM(ENABLE)); 01662 /* Filter saturated error enable */ 01663 ret |= ad77681_spi_write_mask(dev, 01664 AD77681_REG_ADC_DIAG_ENABLE, 01665 AD77681_ADC_DIAG_ERR_FILT_SAT_MSK, 01666 AD77681_ADC_DIAG_ERR_FILT_SAT(ENABLE)); 01667 /* Filter not settled error enable */ 01668 ret |= ad77681_spi_write_mask(dev, 01669 AD77681_REG_ADC_DIAG_ENABLE, 01670 AD77681_ADC_DIAG_ERR_FILT_NOT_SET_MSK, 01671 AD77681_ADC_DIAG_ERR_FILT_NOT_SET(ENABLE)); 01672 /* External clock check error enable */ 01673 ret |= ad77681_spi_write_mask(dev, 01674 AD77681_REG_ADC_DIAG_ENABLE, 01675 AD77681_ADC_DIAG_ERR_EXT_CLK_QUAL_MSK, 01676 AD77681_ADC_DIAG_ERR_EXT_CLK_QUAL(ENABLE)); 01677 01678 /* DIG DIAG ENABLE */ 01679 /* Memory map CRC error enabled */ 01680 ret |= ad77681_spi_write_mask(dev, 01681 AD77681_REG_DIG_DIAG_ENABLE, 01682 AD77681_DIG_DIAG_ERR_MEMMAP_CRC_MSK, 01683 AD77681_DIG_DIAG_ERR_MEMMAP_CRC(ENABLE)); 01684 /* RAM CRC error enabled */ 01685 ret |= ad77681_spi_write_mask(dev, 01686 AD77681_REG_DIG_DIAG_ENABLE, 01687 AD77681_DIG_DIAG_ERR_RAM_CRC_MSK, 01688 AD77681_DIG_DIAG_ERR_RAM_CRC(ENABLE)); 01689 /* FUSE CRC error enabled */ 01690 ret |= ad77681_spi_write_mask(dev, 01691 AD77681_REG_DIG_DIAG_ENABLE, 01692 AD77681_DIG_DIAG_ERR_FUSE_CRC_MSK, 01693 AD77681_DIG_DIAG_ERR_FUSE_CRC(ENABLE)); 01694 /* Enable MCLK Counter */ 01695 ret |= ad77681_spi_write_mask(dev, 01696 AD77681_REG_DIG_DIAG_ENABLE, 01697 AD77681_DIG_DIAG_FREQ_COUNT_EN_MSK, 01698 AD77681_DIG_DIAG_FREQ_COUNT_EN(ENABLE)); 01699 01700 return ret; 01701 } 01702 01703 /** 01704 * Read from all ADC status registers 01705 * @param dev - The device structure. 01706 * @param status - Structure with all satuts bits 01707 * @return 0 in case of success, negative error code otherwise. 01708 */ 01709 int32_t ad77681_status(struct ad77681_dev *dev, 01710 struct ad77681_status_registers *status) 01711 { 01712 int32_t ret; 01713 uint8_t buf[3]; 01714 01715 /* Master status register */ 01716 ret = ad77681_spi_reg_read(dev, AD77681_REG_MASTER_STATUS,buf); 01717 status->master_error = buf[1] & AD77681_MASTER_ERROR_MSK; 01718 status->adc_error = buf[1] & AD77681_MASTER_ADC_ERROR_MSK; 01719 status->dig_error = buf[1] & AD77681_MASTER_DIG_ERROR_MSK; 01720 status->adc_err_ext_clk_qual = buf[1] & AD77681_MASTER_DIG_ERR_EXT_CLK_MSK; 01721 status->adc_filt_saturated = buf[1] & AD77681_MASTER_FILT_SAT_MSK; 01722 status->adc_filt_not_settled = buf[1] & AD77681_MASTER_FILT_NOT_SET_MSK; 01723 status->spi_error = buf[1] & AD77681_MASTER_SPI_ERROR_MSK; 01724 status->por_flag = buf[1] & AD77681_MASTER_POR_FLAG_MSK; 01725 /* SPI diag status register */ 01726 ret |= ad77681_spi_reg_read(dev, AD77681_REG_SPI_DIAG_STATUS, buf); 01727 status->spi_ignore = buf[1] & AD77681_SPI_IGNORE_ERROR_MSK; 01728 status->spi_clock_count = buf[1] & AD77681_SPI_CLK_CNT_ERROR_MSK; 01729 status->spi_read_error = buf[1] & AD77681_SPI_READ_ERROR_MSK; 01730 status->spi_write_error = buf[1] & AD77681_SPI_WRITE_ERROR_MSK; 01731 status->spi_crc_error = buf[1] & AD77681_SPI_CRC_ERROR_MSK; 01732 /* ADC diag status register */ 01733 ret |= ad77681_spi_reg_read(dev, AD77681_REG_ADC_DIAG_STATUS,buf); 01734 status->dldo_psm_error = buf[1] & AD77681_ADC_DLDO_PSM_ERROR_MSK; 01735 status->aldo_psm_error = buf[1] & AD77681_ADC_ALDO_PSM_ERROR_MSK; 01736 status->ref_det_error = buf[1] & AD77681_ADC_REF_DET_ERROR_MSK; 01737 status->filt_sat_error = buf[1] & AD77681_ADC_FILT_SAT_MSK; 01738 status->filt_not_set_error = buf[1] & AD77681_ADC_FILT_NOT_SET_MSK; 01739 status->ext_clk_qual_error = buf[1] & AD77681_ADC_DIG_ERR_EXT_CLK_MSK; 01740 /* DIG diag status register */ 01741 ret |= ad77681_spi_reg_read(dev, AD77681_REG_DIG_DIAG_STATUS,buf); 01742 status->memoy_map_crc_error = buf[1] & AD77681_DIG_MEMMAP_CRC_ERROR_MSK; 01743 status->ram_crc_error = buf[1] & AD77681_DIG_RAM_CRC_ERROR_MSK; 01744 status->fuse_crc_error = buf[1] & AD77681_DIG_FUS_CRC_ERROR_MSK; 01745 01746 return ret; 01747 } 01748 01749 /** 01750 * Initialize the device. 01751 * @param device - The device structure. 01752 * @param init_param - The structure that contains the device initial 01753 * parameters. 01754 * @param status - The structure that will contains the ADC status 01755 * @return 0 in case of success, negative error code otherwise. 01756 */ 01757 int32_t ad77681_setup(struct ad77681_dev **device, 01758 struct ad77681_init_param init_param, 01759 struct ad77681_status_registers **status) 01760 { 01761 struct ad77681_dev *dev; 01762 struct ad77681_status_registers *stat; 01763 int32_t ret; 01764 uint8_t scratchpad_check = 0xAD; 01765 01766 dev = (struct ad77681_dev *)malloc(sizeof(*dev)); 01767 if (!dev) { 01768 return -1; 01769 } 01770 01771 stat = (struct ad77681_status_registers *)malloc(sizeof(*stat)); 01772 if (!stat) { 01773 free(dev); 01774 return -1; 01775 } 01776 01777 dev->power_mode = init_param.power_mode; 01778 dev->mclk_div = init_param.mclk_div; 01779 dev->conv_diag_sel = init_param.conv_diag_sel; 01780 dev->conv_mode = init_param.conv_mode; 01781 dev->diag_mux_sel = init_param.diag_mux_sel; 01782 dev->conv_len = init_param.conv_len; 01783 dev->crc_sel = AD77681_NO_CRC; 01784 dev->status_bit = init_param.status_bit; 01785 dev->VCM_out = init_param.VCM_out; 01786 dev->AINn = init_param.AINn; 01787 dev->AINp = init_param.AINp; 01788 dev->REFn = init_param.REFn; 01789 dev->REFp = init_param.REFp; 01790 dev->filter = init_param.filter; 01791 dev->decimate = init_param.decimate; 01792 dev->sinc3_osr = init_param.sinc3_osr; 01793 dev->vref = init_param.vref; 01794 dev->mclk = init_param.mclk; 01795 dev->sample_rate = init_param.sample_rate; 01796 dev->data_frame_byte = init_param.data_frame_byte; 01797 01798 ret = spi_init(&dev->spi_desc, &init_param.spi_eng_dev_init); 01799 if (ret < 0) { 01800 free(dev); 01801 free(stat); 01802 return ret; 01803 } 01804 01805 ret |= ad77681_soft_reset(dev); 01806 01807 udelay(200); 01808 01809 /* Check physical connection using scratchpad*/ 01810 if (ad77681_scratchpad(dev, &scratchpad_check) == FAILURE) { 01811 scratchpad_check = 0xAD;/* If failure, second try */ 01812 ret |= (ad77681_scratchpad(dev, &scratchpad_check)); 01813 if(ret == FAILURE) { 01814 free(dev); 01815 free(stat); 01816 return ret; 01817 } 01818 } 01819 ret |= ad77681_set_power_mode(dev, dev->power_mode); 01820 ret |= ad77681_set_mclk_div(dev, dev->mclk_div); 01821 ret |= ad77681_set_conv_mode(dev, 01822 dev->conv_mode, 01823 dev->diag_mux_sel, 01824 dev->conv_diag_sel); 01825 ret |= ad77681_set_convlen(dev, dev->conv_len); 01826 ret |= ad77681_set_status_bit(dev, dev->status_bit); 01827 ret |= ad77681_set_crc_sel(dev, init_param.crc_sel); 01828 ret |= ad77681_set_VCM_output(dev, dev->VCM_out); 01829 ret |= ad77681_set_AINn_buffer(dev, dev->AINn); 01830 ret |= ad77681_set_AINp_buffer(dev, dev->AINp); 01831 ret |= ad77681_set_REFn_buffer(dev, dev->REFn); 01832 ret |= ad77681_set_REFp_buffer(dev, dev->REFp); 01833 ret |= ad77681_set_filter_type(dev, dev->decimate, dev->filter, dev->sinc3_osr); 01834 ret |= ad77681_error_flags_enabe(dev); 01835 ret |= ad77681_clear_error_flags(dev); 01836 ret |= ad77681_status(dev, stat); 01837 ad77681_get_frame_byte(dev); 01838 ad77681_update_sample_rate(dev); 01839 *status = stat; 01840 *device = dev; 01841 01842 if (!ret) 01843 printf("ad77681 successfully initialized\n"); 01844 01845 return ret; 01846 } 01847
Generated on Tue Jul 12 2022 19:15:23 by
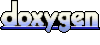