
Sample code for connecting u-blox c027 to AerCloud
Dependencies: C027_Support_AerCloud HTTPClient_AerCloud mbed
Fork of HTTPClient_Cellular_HelloWorld by
main.cpp
00001 // Copyright 2014 Aeris Communications Inc 00002 // 00003 // Licensed under the Apache License, Version 2.0 (the "License"); 00004 // you may not use this file except in compliance with the License. 00005 // You may obtain a copy of the License at 00006 // 00007 // http://www.apache.org/licenses/LICENSE-2.0 00008 // 00009 // Unless required by applicable law or agreed to in writing, software 00010 // distributed under the License is distributed on an "AS IS" BASIS, 00011 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 // See the License for the specific language governing permissions and 00013 // limitations under the License. 00014 00015 // 00016 // AerCloud sample code for the u-blox C027 board 00017 // Details on the C027: http://developer.mbed.org/platforms/u-blox-C027/ 00018 // Details on AerCloud: http://www.aeris.com/technology/aercloud/ 00019 // AerCloud Developer Forum: https://developer.aeris.com/ 00020 // 00021 // Sample writes latitude, longitude, altitude, speed & GPS time to an AerCloud container 00022 // 00023 // Dependencies: 00024 // mbed HTTP Client Libary 00025 // C027_Support Library 00026 // mbed Library 00027 // 00028 // To get the sample running, you'll need to fill in the following parameters below 00029 // Your cellular provider's APN: APN 00030 // AerCloud API Key: AC_APIKEY 00031 // AerCloud Account ID: acId 00032 // AerCloud Container: AC_CONTAINER 00033 // 00034 // and you'll also need to create an AerCloud contianer with the schema 00035 // described below 00036 // 00037 00038 #include "mbed.h" 00039 #include "HTTPClient.h" 00040 #include "GPS.h" 00041 00042 #include "MDM.h" 00043 00044 //------------------------------------------------------------------------------------ 00045 // Connectivity Parameters 00046 //------------------------------------------------------------------------------------ 00047 00048 //! Aeris SIM does not use PIN 00049 #define SIMPIN NULL 00050 00051 //! The APN of your Aeris SIM 00052 // You can find the APN for your SIM at https://aerport.aeris.com 00053 #define APN "_INSERT_YOUR_APN_HERE_" 00054 00055 //! User name and password are not required to use Aeris APN 00056 #define USERNAME NULL 00057 00058 //! User name and password are not required to use Aeris APN 00059 #define PASSWORD NULL 00060 00061 00062 // ------------------------------------------------------------------------------------ 00063 // AerCloud Paramers 00064 // ------------------------------------------------------------------------------------ 00065 00066 // AerCloud BASE URL 00067 #define AC_BASE "http://api.aercloud.aeris.com/v1" 00068 00069 //! AerCloud API Key 00070 // You can find your api key at https://aerport.aeris.com" 00071 #define AC_APIKEY "_INSERT_YOUR_AERCLOUD_API_KEY_HERE_" 00072 00073 //! Aercloud Account Id 00074 // You can find your account id at https://aerport.aeris.com 00075 int acId = 1; 00076 00077 // AerCloud Container 00078 // The name of the AerCloud Container to write data into 00079 #define AC_CONTAINER "C027_Sample" // Example Container 00080 00081 //------------------------------------------------------------------------------------ 00082 // AerCloud SCL (Device) Data Model 00083 // ------------------------------------------------------------------------------------ 00084 // 00085 // Code assumes an AerCloud Data Model with the following fields 00086 // 00087 // Altitude DOUBLE GPS altitude 00088 // Speed DOUBLE GPS speed 00089 // VSLat STRING GPS latitude 00090 // VSLong STRING GPS longitude 00091 // GPSTime DOUBLE Raw GPS time 00092 // GPSDate DOUBLE Raw GPS Date 00093 // SCLID INT AerCloud Device Identifier (i.e. IMEI) 00094 // 00095 00096 //------------------------------------------------------------------------------------ 00097 00098 char str[512]; 00099 char simImei[16]; 00100 00101 const char* HttpResultToString(HTTPResult r) { 00102 switch(r) { 00103 case HTTP_PROCESSING: 00104 return "HTTP_PROCESSING"; 00105 case HTTP_PARSE: 00106 return "HTTP_PARSE"; 00107 case HTTP_DNS: 00108 return "HTTP_DNS"; 00109 case HTTP_PRTCL: 00110 return "HTTP_PRTCL"; 00111 case HTTP_NOTFOUND: 00112 return "HTTP_NOTFOUND - 404"; 00113 case HTTP_REFUSED: 00114 return "HTTP_REFUSED - 403"; 00115 case HTTP_ERROR: 00116 return "HTTP_ERROR"; 00117 case HTTP_TIMEOUT: 00118 return "HTTP_TIMEOUT"; 00119 case HTTP_CONN: 00120 return "HTTP_CONN"; 00121 case HTTP_CLOSED: 00122 return "HTTP_CLOSED"; 00123 // case HTTP_OK: 00124 // return "HTTP_OK"; 00125 } 00126 00127 return "UNKNOWN ERROR CODE"; 00128 } 00129 00130 int main() 00131 { 00132 bool simProvisioned = false; 00133 int ret; 00134 char buf[512] = ""; 00135 int itn = 580; 00136 const int wait = 100; 00137 double lastLat = 0, lastLong = 0, lastAlt = 0, lastSpeed = 0, lastTime = 0, lastDate = 0; 00138 bool abort = false; 00139 00140 GPSI2C gps; 00141 00142 printf("GPS object created\r\n"); 00143 // turn on the supplies of the Modem 00144 MDMSerial mdm; 00145 printf("Modem object created\r\n"); 00146 //mdm.setDebug(4); // enable this for debugging issues 00147 if (!mdm.connect(SIMPIN, APN,USERNAME,PASSWORD)) { 00148 printf("Unabled to connect to the network.\r\n"); 00149 return -1; 00150 } 00151 HTTPClient http; 00152 00153 //get SIM IMEI 00154 mdm.getIMEI(simImei); 00155 printf("Requesting provision info for IMEI %s\r\n",simImei); 00156 00157 // Check if SIM is provisioned in AerCloud 00158 printf("\r\nIs the SIM provisioned? "); 00159 char url[512]; 00160 snprintf(url, sizeof(url), "%s/%d/scls/%s?apiKey=%s", AC_BASE, acId, simImei, AC_APIKEY); 00161 ret = http.get(url, str, 128); 00162 if (ret == HTTP_OK) 00163 { 00164 // We're already provisioned 00165 printf("Yes\r\n"); 00166 printf("Page fetched successfully - read %d characters\r\n", strlen(str)); 00167 printf("Result: %s\r\n", str); 00168 simProvisioned = true; 00169 } 00170 else 00171 { 00172 printf("No\r\n"); 00173 printf("Error - ret = %d - HTTP return code = %d\r\n", ret, http.getHTTPResponseCode()); 00174 //SIM is not provisioned. trying to provision it... 00175 char url[512]; 00176 snprintf(url, sizeof(url), "%s/%d/scls?apiKey=%s", AC_BASE, acId, AC_APIKEY); 00177 00178 snprintf(str, sizeof(str), "{\"sclId\":\"%s\"}\0",simImei); 00179 HTTPText outText(str); 00180 HTTPText inText(str, 512); 00181 ret = http.post(url, outText, &inText); 00182 if (!ret) 00183 { 00184 printf("Executed POST successfully - read %d characters\r\n", strlen(str)); 00185 printf("Result: %s\r\n", str); 00186 simProvisioned = true; 00187 } 00188 else 00189 { 00190 if(http.getHTTPResponseCode() == 200) 00191 simProvisioned = true; 00192 printf("Error - ret = %d - HTTP return code = %d\r\n", ret, http.getHTTPResponseCode()); 00193 } 00194 } 00195 00196 //POST data to containers if SIM has been successfully provisioned 00197 if(simProvisioned) 00198 { 00199 //Read GPS 00200 while (!abort) 00201 { 00202 while ((ret = gps.getMessage(buf, sizeof(buf))) > 0) 00203 { 00204 int len = LENGTH(ret); 00205 if ((PROTOCOL(ret) == GPSParser::NMEA) && (len > 6)) 00206 { 00207 if (!strncmp("$GPGLL", buf, 6)) 00208 { 00209 //Get Lat/Long 00210 double la = 0, lo = 0; 00211 char ch; 00212 if (gps.getNmeaAngle(1,buf,len,la) && 00213 gps.getNmeaAngle(3,buf,len,lo) && 00214 gps.getNmeaItem(6,buf,len,ch) && ch == 'A') 00215 { 00216 lastLat = la; 00217 lastLong = lo; 00218 } 00219 } 00220 else if (!strncmp("$GPGGA", buf, 6)) 00221 { 00222 //Get Altitude 00223 double a = 0; 00224 if (gps.getNmeaItem(9,buf,len,a)) // altitude msl [m] 00225 { 00226 lastAlt = a; 00227 } 00228 } 00229 else if (!strncmp("$GPVTG", buf, 6)) 00230 { 00231 //Get Speed 00232 double s = 0; 00233 if (gps.getNmeaItem(7,buf,len,s)) // speed [km/h] 00234 { 00235 lastSpeed = s; 00236 } 00237 } 00238 else if (!strncmp("$GPRMC", buf, 6)) 00239 { 00240 //Get Timestamp 00241 double fixTime = 0; 00242 double fixDate = 0; 00243 00244 if (gps.getNmeaItem(1,buf,len,fixTime)) // speed [km/h] 00245 { 00246 lastTime = fixTime; 00247 } 00248 if (gps.getNmeaItem(9,buf,len,fixDate)) // speed [km/h] 00249 { 00250 lastDate = fixDate; 00251 } 00252 // printf("time: %f\n Date:%f\n", lastTime, lastDate); 00253 } 00254 } 00255 } 00256 wait_ms(wait); 00257 itn++; 00258 //post every 60 seconds 00259 if(itn == 590) 00260 { 00261 // POST data to AerCLoud 00262 char url[512]; 00263 snprintf(url, sizeof(url), "%s/%d/scls/%s/containers/%s/contentInstances?apiKey=%s", AC_BASE, acId, simImei, AC_CONTAINER, AC_APIKEY); 00264 00265 sprintf(str,"{\"VSLat\": %.5f, \"VSLong\": %.5f, \"Altitude\": %.5f, \"Speed\":%.5f, \"GPSTime\":%.5f, \"GPSDate\":%.5f, \"SCLID\":\"%s\"}", lastLat, lastLong, lastAlt, lastSpeed, lastTime, lastDate, simImei); 00266 HTTPText outText(str); 00267 HTTPText inText(str, 512); 00268 printf("\r\nPost acquired data...\r\n"); 00269 ret = http.post(url, outText, &inText); 00270 if (ret == HTTP_OK) 00271 { 00272 printf("Executed POST successfully - read %d characters\r\n", strlen(str)); 00273 printf("Result: %s\r\n", str); 00274 } 00275 else 00276 { 00277 printf("Error - ret = %d (%s), - HTTP return code = %d\r\n", ret, HttpResultToString((HTTPResult)ret), http.getHTTPResponseCode()); 00278 } 00279 itn = 0; 00280 } 00281 } 00282 gps.powerOff(); 00283 } else { 00284 printf("SIM is not provisioned.\r\n"); 00285 } 00286 00287 00288 mdm.disconnect(); 00289 mdm.powerOff(); 00290 00291 return 0; 00292 } 00293
Generated on Thu Jul 28 2022 04:18:10 by
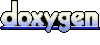