Demo application for using the AT&T IoT Starter Kit Powered by AWS.
Dependencies: SDFileSystem
Fork of ATT_AWS_IoT_demo by
timer.cpp
00001 /** 00002 * @file timer.c 00003 * @brief mbed-os implementation of the timer interface needed for AWS. 00004 */ 00005 00006 #include <stddef.h> 00007 #include "timer_interface.h" 00008 00009 TimerExt::TimerExt() { 00010 unsigned int timeout_ms = 0; 00011 }; 00012 00013 char expired(Timer* timer) { 00014 if (timer->read_ms() > timer->timeout_ms) 00015 return 1; 00016 00017 return 0; 00018 } 00019 00020 void countdown_ms(Timer* timer, unsigned int timeout) { 00021 timer->timeout_ms = timeout; 00022 timer->reset(); 00023 timer->start(); 00024 } 00025 00026 void countdown(Timer* timer, unsigned int timeout) { 00027 timer->timeout_ms = (timeout * 1000); 00028 timer->reset(); 00029 timer->start(); 00030 } 00031 00032 uint32_t left_ms(Timer* timer) { 00033 if (timer->read_ms() < timer->timeout_ms) 00034 return (timer->timeout_ms - timer->read_ms()); 00035 00036 return 0; 00037 } 00038 00039 void InitTimer(Timer* timer) { 00040 timer->stop(); 00041 timer->reset(); 00042 timer->timeout_ms = 0; 00043 } 00044 00045
Generated on Tue Jul 12 2022 22:13:21 by
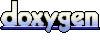