Demo application for using the AT&T IoT Starter Kit Powered by AWS.
Dependencies: SDFileSystem
Fork of ATT_AWS_IoT_demo by
network.cpp
00001 /** 00002 * @file timer.c 00003 * @brief mbed-os implementation of the network interface needed for AWS. 00004 */ 00005 #include <stdlib.h> /* atoi */ 00006 #include "network_interface.h" 00007 #include "EthernetInterface.h" 00008 00009 #include "aws_iot_config.h" 00010 #include "aws_iot_log.h" 00011 00012 #include "mbedtls/net_sockets.h" 00013 00014 //===================================================================================================================== 00015 // 00016 // Uses Avnet Sheild (AT&T wireless LTE) 00017 // 00018 //===================================================================================================================== 00019 #ifdef USING_AVNET_SHIELD 00020 #include "WNCInterface.h" 00021 #include "WNCTCPSocketConnection.h" 00022 00023 // Expose serial for WNC boot 00024 extern MODSERIAL pc; 00025 extern char iccidName[21]; 00026 00027 // Network socket 00028 WNCTCPSocketConnection* _tcpsocket; 00029 00030 int net_modem_boot() 00031 { 00032 INFO("Booting WNC modem..."); 00033 int rc = -1; 00034 string str; 00035 WNCInterface eth_iface; 00036 00037 INFO("...Using Avnet Shield and AT&T wireless LTE"); 00038 rc = eth_iface.init(NULL, &pc); 00039 INFO("WNC Module %s initialized (%02X).", rc?"IS":"IS NOT", rc); 00040 if( !rc ) { 00041 ERROR("DHCP failed."); 00042 return rc; 00043 } 00044 00045 eth_iface.connect(); 00046 INFO("...IP Address: %s ", eth_iface.getIPAddress()); 00047 00048 // Get SIM card number (ICCID) 00049 eth_iface.getICCID(&str); 00050 strcpy(iccidName, str.c_str()); 00051 INFO("...ICCID: %s", iccidName); 00052 return rc; 00053 } 00054 00055 void mbedtls_net_init( mbedtls_net_context *ctx ) 00056 { 00057 DEBUG("...mbedtls_net_init()"); 00058 00059 _tcpsocket = new WNCTCPSocketConnection; 00060 00061 return; 00062 } 00063 00064 int mbedtls_net_connect( mbedtls_net_context *ctx, const char *host, const char *port, int proto ) 00065 { 00066 DEBUG("...mbedtls_net_connect"); 00067 int ret = -1; 00068 00069 /* Connect to the server */ 00070 INFO("Connecting with %s\r\n", host); 00071 ret = _tcpsocket->connect(host, atoi(port)); 00072 00073 return ret; 00074 } 00075 00076 int mbedtls_net_recv_timeout( void *ctx, unsigned char *buf, size_t len, uint32_t timeout ) 00077 { 00078 DEBUG("...mbedtls_net_recv_timeout len: %d, timeout: %d", len, timeout); 00079 return (int)_tcpsocket->receive((char*)buf, len); 00080 } 00081 00082 int mbedtls_net_send( void *ctx, const unsigned char *buf, size_t len ) 00083 { 00084 DEBUG("...mbedtls_net_send"); 00085 return _tcpsocket->send((char*)buf, len); 00086 } 00087 00088 int mbedtls_net_set_block( mbedtls_net_context *ctx ) 00089 { 00090 DEBUG("...mbedtls_net_set_block"); 00091 _tcpsocket->set_blocking (false,1500); 00092 return 0; 00093 } 00094 00095 void mbedtls_net_free( mbedtls_net_context *ctx ) 00096 { 00097 DEBUG("...mbedtls_net_free"); 00098 return; 00099 } 00100 #endif 00101 00102 //===================================================================================================================== 00103 // 00104 // Uses FRDM-K64F wired ethernet 00105 // 00106 //===================================================================================================================== 00107 #ifdef USING_FRDM_K64F_LWIP 00108 // Network Socket 00109 TCPSocket* _tcpsocket; 00110 00111 int net_modem_boot() 00112 { 00113 // Do nothing 00114 return 0; 00115 } 00116 00117 void mbedtls_net_init( mbedtls_net_context *ctx ) 00118 { 00119 DEBUG("...mbedtls_net_init()"); 00120 EthernetInterface eth_iface; 00121 00122 eth_iface.connect(); 00123 INFO("...Using FRDM-K64F Ethernet LWIP"); 00124 const char *ip_addr = eth_iface.get_ip_address(); 00125 if (ip_addr) { 00126 INFO("...Client IP Address is %s", ip_addr); 00127 } else { 00128 INFO("...No Client IP Address"); 00129 } 00130 _tcpsocket = new TCPSocket(ð_iface); 00131 00132 return; 00133 } 00134 00135 int mbedtls_net_connect( mbedtls_net_context *ctx, const char *host, const char *port, int proto ) 00136 { 00137 DEBUG("...mbedtls_net_connect"); 00138 int ret; 00139 00140 INFO("...Connecting with %s", host); 00141 ret = _tcpsocket->connect(host, atoi(port)); 00142 if (ret != NSAPI_ERROR_OK) { 00143 ERROR("Failed to connect"); 00144 return ret; 00145 } 00146 00147 INFO("...Connected to Amazon!"); 00148 return ret; 00149 } 00150 00151 int mbedtls_net_recv_timeout( void *ctx, unsigned char *buf, size_t len, uint32_t timeout ) 00152 { 00153 DEBUG("...mbedtls_net_recv_timeout len: %d, timeout: %d", len, timeout); 00154 int recv = -1; 00155 00156 _tcpsocket->set_timeout(timeout); 00157 recv = _tcpsocket->recv(buf, len); 00158 00159 if(NSAPI_ERROR_WOULD_BLOCK == recv || 00160 recv == 0){ 00161 DEBUG("...NSAPI_ERROR_WOULD_BLOCK"); 00162 return 0; 00163 }else if(recv < 0){ 00164 ERROR("...RECV FAIL"); 00165 return -1; 00166 }else{ 00167 DEBUG("...RECV OK: %d, %d", len, recv); 00168 return recv; 00169 } 00170 } 00171 00172 int mbedtls_net_send( void *ctx, const unsigned char *buf, size_t len ) 00173 { 00174 DEBUG("...mbedtls_net_send"); 00175 int size = -1; 00176 00177 size = _tcpsocket->send(buf, len); 00178 00179 if(NSAPI_ERROR_WOULD_BLOCK == size){ 00180 DEBUG("...SEND OK, len = %d", len); 00181 return len; 00182 }else if(size < 0){ 00183 ERROR("...SEND FAIL"); 00184 return -1; 00185 }else{ 00186 DEBUG("...SEND OK, size = %d", size); 00187 return size; 00188 } 00189 } 00190 00191 int mbedtls_net_set_block( mbedtls_net_context *ctx ) 00192 { 00193 DEBUG("...mbedtls_net_set_block"); 00194 _tcpsocket->set_blocking(false); 00195 return 0; 00196 } 00197 00198 void mbedtls_net_free( mbedtls_net_context *ctx ) 00199 { 00200 DEBUG("...mbedtls_net_free"); 00201 return; 00202 } 00203 #endif
Generated on Tue Jul 12 2022 22:13:21 by
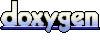