Demo application for using the AT&T IoT Starter Kit Powered by AWS.
Dependencies: SDFileSystem
Fork of ATT_AWS_IoT_demo by
aws_iot_shadow.cpp
00001 /* 00002 * Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 #include "aws_iot_error.h" 00017 #include "aws_iot_log.h" 00018 #include "aws_iot_shadow_actions.h" 00019 #include "aws_iot_shadow_json.h" 00020 #include "aws_iot_shadow_key.h" 00021 #include "aws_iot_shadow_records.h" 00022 00023 const ShadowParameters_t ShadowParametersDefault = { 00024 .pMyThingName = AWS_IOT_MY_THING_NAME, 00025 .pMqttClientId = AWS_IOT_MQTT_CLIENT_ID, 00026 .pHost = AWS_IOT_MQTT_HOST, 00027 .port = AWS_IOT_MQTT_PORT, 00028 .pRootCA = NULL, 00029 .pClientCRT = NULL, 00030 .pClientKey = NULL 00031 }; 00032 00033 void aws_iot_shadow_reset_last_received_version(void) { 00034 shadowJsonVersionNum = 0; 00035 } 00036 00037 uint32_t aws_iot_shadow_get_last_received_version(void) { 00038 return shadowJsonVersionNum; 00039 } 00040 00041 void aws_iot_shadow_enable_discard_old_delta_msgs(void) { 00042 shadowDiscardOldDeltaFlag = true; 00043 } 00044 00045 void aws_iot_shadow_disable_discard_old_delta_msgs(void) { 00046 shadowDiscardOldDeltaFlag = false; 00047 } 00048 00049 IoT_Error_t aws_iot_shadow_init(MQTTClient_t *pClient) { 00050 00051 IoT_Error_t rc = NONE_ERROR; 00052 00053 if (pClient == NULL) { 00054 return NULL_VALUE_ERROR; 00055 } 00056 00057 resetClientTokenSequenceNum(); 00058 aws_iot_shadow_reset_last_received_version(); 00059 initDeltaTokens(); 00060 return NONE_ERROR; 00061 } 00062 00063 IoT_Error_t aws_iot_shadow_connect(MQTTClient_t *pClient, ShadowParameters_t *pParams) { 00064 IoT_Error_t rc = NONE_ERROR; 00065 00066 MQTTConnectParams ConnectParams = MQTTConnectParamsDefault; 00067 if (pClient == NULL) { 00068 return NULL_VALUE_ERROR; 00069 } 00070 00071 if (pClient->connect == NULL) { 00072 return NULL_VALUE_ERROR; 00073 } 00074 00075 snprintf(myThingName, MAX_SIZE_OF_THING_NAME, "%s", pParams->pMyThingName ); 00076 snprintf(mqttClientID, MAX_SIZE_OF_UNIQUE_CLIENT_ID_BYTES, "%s", pParams->pMqttClientId ); 00077 00078 INFO("...Thing Name %s", myThingName); 00079 INFO("...MQTT Client ID %s", mqttClientID); 00080 00081 ConnectParams.KeepAliveInterval_sec = 10; 00082 ConnectParams.MQTTVersion = MQTT_3_1_1; 00083 ConnectParams.mqttCommandTimeout_ms = 10000;//2000; 00084 ConnectParams.tlsHandshakeTimeout_ms = 10000; 00085 ConnectParams.isCleansession = true; 00086 ConnectParams.isSSLHostnameVerify = true; 00087 ConnectParams.isWillMsgPresent = false; 00088 ConnectParams.pClientID = pParams->pMqttClientId; 00089 ConnectParams.pDeviceCertLocation = pParams->pClientCRT; 00090 ConnectParams.pDevicePrivateKeyLocation = pParams->pClientKey; 00091 ConnectParams.pRootCALocation = pParams->pRootCA; 00092 ConnectParams.pPassword = NULL; 00093 ConnectParams.pUserName = NULL; 00094 ConnectParams.pHostURL = pParams->pHost; 00095 ConnectParams.port = pParams->port; 00096 ConnectParams.disconnectHandler = NULL; 00097 00098 rc = pClient->connect(&ConnectParams); 00099 00100 if(rc == NONE_ERROR){ 00101 initializeRecords(pClient); 00102 } 00103 00104 return rc; 00105 } 00106 00107 IoT_Error_t aws_iot_shadow_register_delta(MQTTClient_t *pClient, jsonStruct_t *pStruct) { 00108 IoT_Error_t rc = NONE_ERROR; 00109 00110 if (!(pClient->isConnected())) { 00111 return CONNECTION_ERROR; 00112 } 00113 00114 rc = registerJsonTokenOnDelta(pStruct); 00115 00116 return rc; 00117 } 00118 00119 IoT_Error_t aws_iot_shadow_yield(MQTTClient_t *pClient, int timeout) { 00120 HandleExpiredResponseCallbacks(); 00121 return pClient->yield(timeout); 00122 } 00123 00124 IoT_Error_t aws_iot_shadow_disconnect(MQTTClient_t *pClient) { 00125 return pClient->disconnect(); 00126 } 00127 00128 IoT_Error_t aws_iot_shadow_update(MQTTClient_t *pClient, const char *pThingName, char *pJsonString, 00129 fpActionCallback_t callback, void *pContextData, uint8_t timeout_seconds, bool isPersistentSubscribe) { 00130 00131 IoT_Error_t ret_val = NONE_ERROR; 00132 00133 if (!(pClient->isConnected())) { 00134 return CONNECTION_ERROR; 00135 } 00136 00137 ret_val = iot_shadow_action(pClient, pThingName, SHADOW_UPDATE, pJsonString, callback, pContextData, 00138 timeout_seconds, isPersistentSubscribe); 00139 00140 return ret_val; 00141 } 00142 00143 IoT_Error_t aws_iot_shadow_delete(MQTTClient_t *pClient, const char *pThingName, fpActionCallback_t callback, 00144 void *pContextData, uint8_t timeout_seconds, bool isPersistentSubscribe) { 00145 IoT_Error_t ret_val = NONE_ERROR; 00146 00147 if (!(pClient->isConnected())) { 00148 return CONNECTION_ERROR; 00149 } 00150 00151 char deleteRequestJsonBuf[MAX_SIZE_CLIENT_TOKEN_CLIENT_SEQUENCE]; 00152 iot_shadow_delete_request_json(deleteRequestJsonBuf); 00153 ret_val = iot_shadow_action(pClient, pThingName, SHADOW_DELETE, deleteRequestJsonBuf, callback, pContextData, 00154 timeout_seconds, isPersistentSubscribe); 00155 00156 return ret_val; 00157 } 00158 00159 IoT_Error_t aws_iot_shadow_get(MQTTClient_t *pClient, const char *pThingName, fpActionCallback_t callback, 00160 void *pContextData, uint8_t timeout_seconds, bool isPersistentSubscribe) { 00161 00162 IoT_Error_t ret_val = NONE_ERROR; 00163 00164 if (!(pClient->isConnected())) { 00165 return CONNECTION_ERROR; 00166 } 00167 00168 char getRequestJsonBuf[MAX_SIZE_CLIENT_TOKEN_CLIENT_SEQUENCE]; 00169 00170 iot_shadow_get_request_json(getRequestJsonBuf); 00171 00172 ret_val = iot_shadow_action(pClient, pThingName, SHADOW_GET, getRequestJsonBuf, callback, pContextData, 00173 timeout_seconds, isPersistentSubscribe); 00174 00175 return ret_val; 00176 } 00177
Generated on Tue Jul 12 2022 22:13:20 by
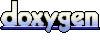