Demo application for using the AT&T IoT Starter Kit Powered by AWS.
Dependencies: SDFileSystem
Fork of ATT_AWS_IoT_demo by
WncControllerK64F.cpp
00001 /* 00002 Copyright (c) 2016 Fred Kellerman 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 00022 @file WncController.cpp 00023 @purpose Controls WNC Cellular Modem 00024 @version 1.0 00025 @date July 2016 00026 @author Fred Kellerman 00027 */ 00028 00029 #include "WncControllerK64F.h" 00030 00031 using namespace WncControllerK64F_fk; 00032 00033 WncControllerK64F::WncControllerK64F(struct WncGpioPinListK64F * pPins, MODSERIAL * wnc_uart, MODSERIAL * debug_uart) 00034 { 00035 m_logTimer.start(); // Start the log timer now! 00036 m_pDbgUart = debug_uart; 00037 m_pWncUart = wnc_uart; 00038 m_gpioPinList = *pPins; 00039 } 00040 00041 bool WncControllerK64F::enterWncTerminalMode(MODSERIAL * pUart, bool echoOn) 00042 { 00043 if (pUart == NULL) 00044 return (false); // Need a uart! 00045 00046 string * resp; 00047 AtCmdErr_e r = sendWncCmd("AT", &resp, 500); 00048 if (r == WNC_AT_CMD_TIMEOUT) 00049 return (false); 00050 00051 pUart->puts("\r\nEntering WNC Terminal Mode - press <CTRL>-Q to exit!\r\n"); 00052 00053 while (1) { 00054 if (pUart->readable()) { 00055 char c = pUart->getc(); 00056 if (c == '\x11') { 00057 pUart->puts("\r\nExiting WNC Terminal Mode!\r\n"); 00058 // Cleanup in case user doesn't finish command: 00059 sendWncCmd("AT", &resp, 300); 00060 // Above AT may fail but should get WNC back in sync 00061 return (sendWncCmd("AT", &resp, 500) == WNC_AT_CMD_OK); 00062 } 00063 if (echoOn == true) { 00064 pUart->putc(c); 00065 } 00066 m_pWncUart->putc(c); 00067 } 00068 if (m_pWncUart->readable()) 00069 pUart->putc(m_pWncUart->getc()); 00070 } 00071 } 00072 00073 00074 int WncControllerK64F::putc(char c) 00075 { 00076 return (m_pWncUart->putc(c)); 00077 } 00078 00079 int WncControllerK64F::puts(const char * s) 00080 { 00081 return (m_pWncUart->puts(s)); 00082 } 00083 00084 char WncControllerK64F::getc(void) 00085 { 00086 return (m_pWncUart->getc()); 00087 } 00088 00089 int WncControllerK64F::charReady(void) 00090 { 00091 return (m_pWncUart->readable()); 00092 } 00093 00094 int WncControllerK64F::dbgWriteChar(char b) 00095 { 00096 if (m_pDbgUart != NULL) 00097 return (m_pDbgUart->putc(b)); 00098 else 00099 return (0); 00100 } 00101 00102 int WncControllerK64F::dbgWriteChars(const char * b) 00103 { 00104 if (m_pDbgUart != NULL) 00105 return (m_pDbgUart->puts(b)); 00106 else 00107 return (0); 00108 } 00109 00110 bool WncControllerK64F::initWncModem(uint8_t powerUpTimeoutSecs) 00111 { 00112 // Hard reset the modem (doesn't go through 00113 // the signal level translator) 00114 *m_gpioPinList.mdm_reset = 0; 00115 00116 // disable signal level translator (necessary 00117 // for the modem to boot properly). All signals 00118 // except mdm_reset go through the level translator 00119 // and have internal pull-up/down in the module. While 00120 // the level translator is disabled, these pins will 00121 // be in the correct state. 00122 *m_gpioPinList.shield_3v3_1v8_sig_trans_ena = 0; 00123 00124 // While the level translator is disabled and ouptut pins 00125 // are tristated, make sure the inputs are in the same state 00126 // as the WNC Module pins so that when the level translator is 00127 // enabled, there are no differences. 00128 *m_gpioPinList.mdm_uart2_rx_boot_mode_sel = 1; // UART2_RX should be high 00129 *m_gpioPinList.mdm_power_on = 0; // powr_on should be low 00130 *m_gpioPinList.mdm_wakeup_in = 1; // wake-up should be high 00131 *m_gpioPinList.mdm_uart1_cts = 0; // indicate that it is ok to send 00132 00133 // Now, wait for the WNC Module to perform its initial boot correctly 00134 waitMs(1000); 00135 00136 // The WNC module initializes comms at 115200 8N1 so set it up 00137 m_pWncUart->baud(115200); 00138 00139 //Now, enable the level translator, the input pins should now be the 00140 //same as how the M14A module is driving them with internal pull ups/downs. 00141 //When enabled, there will be no changes in these 4 pins... 00142 *m_gpioPinList.shield_3v3_1v8_sig_trans_ena = 1; 00143 00144 bool res = waitForPowerOnModemToRespond(powerUpTimeoutSecs); 00145 00146 // Toggle wakeup to prevent future dropped 'A' of "AT", this was 00147 // suggested by ATT. 00148 if (res == true) { 00149 m_pDbgUart->puts("\r\nToggling Wakeup...\r\n"); 00150 waitMs(20); 00151 *m_gpioPinList.mdm_wakeup_in = 0; 00152 waitMs(2000); 00153 *m_gpioPinList.mdm_wakeup_in = 1; 00154 waitMs(20); 00155 m_pDbgUart->puts("Toggling complete.\r\n"); 00156 } 00157 00158 return (res); 00159 } 00160 00161 void WncControllerK64F::waitMs(int t) 00162 { 00163 wait_ms(t); 00164 } 00165 00166 void WncControllerK64F::waitUs(int t) 00167 { 00168 wait_ms(t); 00169 } 00170 00171 int WncControllerK64F::getLogTimerTicks(void) 00172 { 00173 return (m_logTimer.read_us()); 00174 } 00175 00176 void WncControllerK64F::startTimerA(void) 00177 { 00178 m_timerA.start(); 00179 m_timerA.reset(); 00180 } 00181 00182 void WncControllerK64F::stopTimerA(void) 00183 { 00184 m_timerA.stop(); 00185 } 00186 00187 int WncControllerK64F::getTimerTicksA_mS(void) 00188 { 00189 return (m_timerA.read_ms()); 00190 } 00191 00192 void WncControllerK64F::startTimerB(void) 00193 { 00194 m_timerB.start(); 00195 m_timerB.reset(); 00196 } 00197 00198 void WncControllerK64F::stopTimerB(void) 00199 { 00200 m_timerB.stop(); 00201 } 00202 00203 int WncControllerK64F::getTimerTicksB_mS(void) 00204 { 00205 return (m_timerB.read_ms()); 00206 } 00207
Generated on Tue Jul 12 2022 22:13:21 by
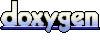