Demo application for using the AT&T IoT Starter Kit Powered by AWS.
Dependencies: SDFileSystem
Fork of ATT_AWS_IoT_demo by
MQTTPacket.h
00001 /******************************************************************************* 00002 * Copyright (c) 2014 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 * Xiang Rong - 442039 Add makefile to Embedded C client 00016 *******************************************************************************/ 00017 00018 #ifndef MQTTPACKET_H_ 00019 #define MQTTPACKET_H_ 00020 00021 #if defined(__cplusplus) /* If this is a C++ compiler, use C linkage */ 00022 extern "C" { 00023 #endif 00024 00025 #if defined(WIN32_DLL) || defined(WIN64_DLL) 00026 #define DLLImport __declspec(dllimport) 00027 #define DLLExport __declspec(dllexport) 00028 #elif defined(LINUX_SO) 00029 #define DLLImport extern 00030 #define DLLExport __attribute__ ((visibility ("default"))) 00031 #else 00032 #define DLLImport 00033 #define DLLExport 00034 #endif 00035 00036 #include "stdint.h" 00037 #include "stddef.h" 00038 #include "MQTTReturnCodes.h" 00039 #include "MQTTMessage.h" 00040 00041 /** 00042 * Bitfields for the MQTT header byte. 00043 */ 00044 typedef union { 00045 unsigned char byte; /**< the whole byte */ 00046 #if defined(REVERSED) 00047 struct { 00048 unsigned int type : 4; /**< message type nibble */ 00049 unsigned int dup : 1; /**< DUP flag bit */ 00050 unsigned int qos : 2; /**< QoS value, 0, 1 or 2 */ 00051 unsigned int retain : 1; /**< retained flag bit */ 00052 } bits; 00053 #else 00054 struct { 00055 unsigned int retain : 1; /**< retained flag bit */ 00056 unsigned int qos : 2; /**< QoS value, 0, 1 or 2 */ 00057 unsigned int dup : 1; /**< DUP flag bit */ 00058 unsigned int type : 4; /**< message type nibble */ 00059 } bits; 00060 #endif 00061 } MQTTHeader; 00062 00063 typedef struct { 00064 size_t len; 00065 char *data; 00066 } MQTTLenString; 00067 00068 typedef struct { 00069 char *cstring; 00070 MQTTLenString lenstring; 00071 } MQTTString; 00072 00073 #define MQTTString_initializer {NULL, {0, NULL}} 00074 00075 MQTTReturnCode MQTTPacket_InitHeader(MQTTHeader *header, MessageTypes message_type, 00076 QoS qos, uint8_t dup, uint8_t retained); 00077 size_t MQTTstrlen(MQTTString mqttstring); 00078 00079 #include "MQTTConnect.h" 00080 #include "MQTTPublish.h" 00081 #include "MQTTSubscribe.h" 00082 #include "MQTTUnsubscribe.h" 00083 00084 MQTTReturnCode MQTTSerialize_ack(unsigned char *buf, size_t buflen, 00085 unsigned char type, unsigned char dup, uint16_t packetid, 00086 uint32_t *serialized_len); 00087 MQTTReturnCode MQTTDeserialize_ack(unsigned char *packettype, unsigned char *dup, 00088 uint16_t *packetid, unsigned char *buf, 00089 size_t buflen); 00090 00091 size_t MQTTPacket_len(size_t rem_len); 00092 uint8_t MQTTPacket_equals(MQTTString *a, char *bptr); 00093 00094 uint32_t MQTTPacket_encode(unsigned char *buf, size_t length); 00095 MQTTReturnCode MQTTPacket_decode(uint32_t (*getcharfn)(unsigned char *, uint32_t), uint32_t *value, uint32_t *readBytesLen); 00096 MQTTReturnCode MQTTPacket_decodeBuf(unsigned char *buf, uint32_t *value, uint32_t *readBytesLen); 00097 00098 uint16_t readPacketId(unsigned char **pptr); 00099 void writePacketId(unsigned char** pptr, uint16_t anInt); 00100 00101 int32_t readInt(unsigned char **pptr); 00102 size_t readSizeT(unsigned char **pptr); 00103 unsigned char readChar(unsigned char **pptr); 00104 void writeChar(unsigned char **pptr, unsigned char c); 00105 void writeInt(unsigned char **pptr, int32_t anInt); 00106 void writeSizeT(unsigned char **pptr, size_t size); 00107 MQTTReturnCode readMQTTLenString(MQTTString *mqttstring, unsigned char **pptr, unsigned char *enddata); 00108 void writeCString(unsigned char **pptr, const char *string); 00109 void writeMQTTString(unsigned char **pptr, MQTTString mqttstring); 00110 00111 #ifdef __cplusplus /* If this is a C++ compiler, use C linkage */ 00112 } 00113 #endif 00114 00115 00116 #endif /* MQTTPACKET_H_ */ 00117 00118
Generated on Tue Jul 12 2022 22:13:21 by
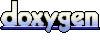