Example of using the ATT M2X back end. Connect over Wifi with the ESP8266 chip. This code sends random data to M2X. This code can be used for Any board, just change the RX/TX pins the wifi adapter is connected to.
Dependencies: ESP8266Interface M2XStreamClient jsonlite mbed
Fork of M2X_K64F_Accel_ESP8266-wifi by
main.cpp
00001 #include "mbed.h" 00002 #include "M2XStreamClient.h" 00003 #include "ESP8266Interface.h" 00004 #include "TCPSocketConnection.h" 00005 00006 00007 /* 00008 * ESP8266 Wifi Config for nucleo 411 00009 */ 00010 ESP8266Interface wifi(D8,D2,D3,"wifiName","wifiPassword",115200); // TX,RX,Reset,SSID,Password,Baud 00011 00012 // 00013 // Fill these field in from you ATT M2X Account 00014 // 00015 char deviceId[] = "<deviceID>"; // Device you want to push to 00016 char streamName[] = "<streamID>"; // Stream you want to push to 00017 char m2xKey[] = "<deviceAPIKey>"; // Your M2X API Key or Master API Key 00018 00019 int main() 00020 { 00021 printf("Starting...\r\n"); 00022 00023 // connect to wifi 00024 wifi.init(); //Reset 00025 wifi.connect(); //Use DHCP 00026 printf("IP Address is %s \n\r", wifi.getIPAddress()); 00027 00028 // Initialize the M2X client 00029 Client client; 00030 M2XStreamClient m2xClient(&client, m2xKey,1,"52.22.150.98"); // api-m2x.att.com 00031 00032 int ret; 00033 volatile int randomNumber = 0; 00034 00035 while (true) { 00036 // send a random number to M2X every 5 seconds 00037 randomNumber = rand(); 00038 ret = m2xClient.updateStreamValue(deviceId, streamName, randomNumber); 00039 printf("send() returned %d\r\n", ret); 00040 wait(5.0); 00041 } 00042 }
Generated on Tue Jul 19 2022 02:20:33 by
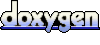