Host library for controlling a WiConnect enabled Wi-Fi module.
Dependents: wiconnect-ota_example wiconnect-web_setup_example wiconnect-test-console wiconnect-tcp_server_example ... more
NetworkInterface.h
00001 /** 00002 * ACKme WiConnect Host Library is licensed under the BSD licence: 00003 * 00004 * Copyright (c)2014 ACKme Networks. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 */ 00029 #pragma once 00030 00031 00032 #include "WiconnectTypes.h" 00033 #include "api/types/ScanResultList.h" 00034 00035 00036 namespace wiconnect { 00037 00038 00039 #ifdef WICONNECT_USE_DEFAULT_STRING_BUFFERS 00040 #define ALLOW_NULL_STRING_BUFFER = NULL 00041 #else 00042 #define ALLOW_NULL_STRING_BUFFER 00043 #endif 00044 00045 /** 00046 * @ingroup api_network_types 00047 * 00048 * @brief The provides an interface for joining and/or creating a network. 00049 * It provides other utilities such as scanning for networks, pinging a network, 00050 * resolving a domain name to IP address. 00051 * 00052 * @note This class is an interface to the Wiconnect class. It should never be 00053 * independently instantiated or the parent of another class. 00054 */ 00055 class NetworkInterface 00056 { 00057 public: 00058 /** 00059 * @ingroup api_network_setup 00060 * 00061 * @brief Start the WiConnect WiFi module 'web setup' feature. 00062 * 00063 * This command has an optional background processing feature. 00064 * Background processing is enabled if the completeHandler parameter 00065 * is specified. If enabled, the library will poll the module every second 00066 * for the web setup status (essentially it'll call isWebSetupRunning() every 00067 * second in the background). When the web setup is no longer running the 00068 * callback will be executed. The background processing is disabled when stopWebSetup() 00069 * is called. 00070 * 00071 * @note only the 'result' parameter of the callback handler is valid. 00072 * 00073 * Refer to @ref setting_async_processing for more info. 00074 * 00075 * @param[in] ssid Optional, optionally set the SSID of module's softAp 00076 * @param[in] password Optional, optionally set the WPA2-PSK password for the module'S softap 00077 * Note: make an OPEN softAp, set this parameter to a null string (i.e. "") 00078 * @param[in] completeHandler Optional, callback to be executed when module web setup completes. 00079 * @return Result of method. See @ref WiconnectResult 00080 */ 00081 WiconnectResult startWebSetup(const char *ssid = NULL, const char *password = NULL, const Callback &completeHandler = Callback()); 00082 00083 /** 00084 * @ingroup api_network_setup 00085 * 00086 * @brief Stop the WiConnect WiFi module 'web setup' feature. 00087 * 00088 * This method should be called AFTER startWebSetup() to prematurely terminate 00089 * web setup. Note that this is not needed if web setup completes by itself 00090 * (i.e. if the user exits web setup from the webpage). 00091 * 00092 * @return Result of method. See @ref WiconnectResult 00093 */ 00094 WiconnectResult stopWebSetup(); 00095 00096 /** 00097 * @ingroup api_network_setup 00098 * 00099 * @brief Return status of WiConnect WiFi module 'web setup' feature. 00100 * 00101 * This may be called at any time (whether web setpu has been stared or not). 00102 * 00103 * @param[out] isRunningPtr Pointer to bool to contain TRUE if web setup is running, FALSE else 00104 * @return Result of method. See @ref WiconnectResult 00105 */ 00106 WiconnectResult isWebSetupRunning(bool *isRunningPtr); 00107 00108 00109 // ------------------------------------------------------------------------ 00110 00111 00112 /** 00113 * @ingroup api_network_wlan 00114 * 00115 * @brief Join a WiFi network. 00116 * 00117 * This command has an optional background processing feature. 00118 * Background processing is enabled if the completeHandler parameter 00119 * is specified. If enabled, the library will poll the module every second 00120 * for the join status (essentially it'll call getNetworkStatus() every 00121 * second in the background). When the module join sequence complete the callback will be executed. 00122 * The background processing is disabled when leave() is called. 00123 * 00124 * * If completeHandler parameter is NOT specified: 00125 * This command will BLOCK/return WICONNECT_PROCESSING until the module has 00126 * either successfully joined the network or failed. 00127 * * If the completeHandler parameter IS specified: 00128 * This command will return and use the background processing feature described above. 00129 * 00130 * @note only the 'result' parameter of the callback handler is valid. 00131 * 00132 * Refer to @ref setting_async_processing for more info. 00133 * 00134 * @param[in] ssid Optional, optionally set the SSID of the network to join 00135 * @param[in] password Optional, optionally set the passkey of the network to join 00136 * Note: to join an OPEN network, set this parameter to a null string (i.e. "") 00137 * @param[in] completeHandler Optional, callback to be executed when the join sequence completes. 00138 * The 'result' callback parameter contains the WiconnectResult of joining. 00139 * The 'arg1' parameter is a @ref NetworkJoinResult of joining. 00140 * @return Result of method. See @ref WiconnectResult 00141 */ 00142 WiconnectResult join(const char* ssid = NULL, const char *password = NULL, const Callback &completeHandler = Callback()); 00143 00144 /** 00145 * @ingroup api_network_wlan 00146 * 00147 * @brief Leave a WiFi network. 00148 * 00149 * This method may be called to either terminate a join sequence or 00150 * leave a previously connected networked. 00151 * 00152 * @return Result of method. See @ref WiconnectResult 00153 */ 00154 WiconnectResult leave(); 00155 00156 /** 00157 * @ingroup api_network_wlan 00158 * 00159 * @brief Get connection status to WiFi network. 00160 * 00161 * Refer to @ref NetworkStatus for more info. 00162 * 00163 * @param[out] statusPtr Point to a @ref NetworkStatus which will hold current network status of module 00164 * @return Result of method. See @ref WiconnectResult 00165 */ 00166 WiconnectResult getNetworkStatus(NetworkStatus *statusPtr); 00167 00168 /** 00169 * @ingroup api_network_wlan 00170 * 00171 * @brief Get the result of joining the network 00172 * 00173 * Refer to @ref NetworkJoinResult for more info. 00174 * 00175 * @param[out] joinResultPtr Point to a @ref NetworkJoinResult which will hold the result of joining the network 00176 * @return Result of method. See @ref WiconnectResult 00177 */ 00178 WiconnectResult getNetworkJoinResult(NetworkJoinResult *joinResultPtr); 00179 00180 /** 00181 * @ingroup api_network_wlan 00182 * 00183 * @brief Get @ref NetworkSignalStrength of WiFi network module is connected 00184 */ 00185 WiconnectResult getSignalStrength(NetworkSignalStrength *signalStrengthPtr); 00186 00187 /** 00188 * @ingroup api_network_wlan 00189 * 00190 * @brief Get the RSSI in dBm of WiFi network 00191 */ 00192 WiconnectResult getRssi(int32_t *rssiPtr); 00193 00194 /** 00195 * @ingroup api_network_wlan 00196 * 00197 * @brief Get MAC address of the WiFi module 00198 */ 00199 WiconnectResult getMacAddress(MacAddress *macAddress); 00200 00201 00202 // ------------------------------------------------------------------------ 00203 00204 00205 // WiconnectResult startSoftAp(const char* ssid = NULL, const char *password = NULL, const Callback &clientConnectedCallback = Callback()); 00206 // WiconnectResult stopSoftAp(); 00207 // WiconnectResult getSoftApClientList(); 00208 00209 00210 // ------------------------------------------------------------------------ 00211 00212 00213 /** 00214 * @ingroup api_network_util 00215 * 00216 * @brief Scan for available WiFi networks. 00217 * 00218 * The populate the supplied @ref ScanResultList with @ref ScanResult of each found network. 00219 * 00220 * Optionally only scan of specific channels by supplying a null terminated list of channels. 00221 * Example: 00222 * @code 00223 * const uint8_t channelsToScan[] = {1, 6, 11, 0}; 00224 * @endcode 00225 * 00226 * @param[out] resultList List to populate with scan results. 00227 * @param[in] channelList Optional, null terminated list of channels to scan. 00228 * @param[in] ssid Optional, specific network name to scan for. 00229 * @return Result of method. See @ref WiconnectResult 00230 */ 00231 WiconnectResult scan(ScanResultList &resultList, const uint8_t *channelList = NULL, const char* ssid = NULL); 00232 00233 /** 00234 * @ingroup api_network_util 00235 * 00236 * @brief Ping a WiFi network. 00237 * 00238 * Optionally ping a specific server and return the time in milliseconds it took 00239 * for the network to response. If no domain is supplied, the module pings to gateway 00240 * (i.e router it's connected to). 00241 * 00242 * @param[in] domain Optional, the domain name to ping 00243 * @param[out] timeMsPtr Optional, pointer to uint32 to hold time in milliseconds the ping took 00244 * @return Result of method. See @ref WiconnectResult 00245 */ 00246 WiconnectResult ping(const char *domain = NULL, uint32_t *timeMsPtr = NULL); 00247 00248 /** 00249 * @ingroup api_network_util 00250 * 00251 * @brief Resolve domain name into IP address. 00252 * 00253 * @param[in] domain The domain name to resolve 00254 * @param[out] ipAddressPtr pointer to uint32 to hold resolved IP address. Note, the IP address is in network-byte-order. 00255 * @return Result of method. See @ref WiconnectResult 00256 */ 00257 WiconnectResult lookup(const char *domain, uint32_t *ipAddressPtr); 00258 00259 00260 // ------------------------------------------------------------------------ 00261 00262 00263 /** 00264 * @ingroup api_network_settings 00265 * 00266 * @brief Set DHCP enabled. 00267 * 00268 * @return Result of method. See @ref WiconnectResult 00269 */ 00270 WiconnectResult setDhcpEnabled(bool enabled); 00271 00272 /** 00273 * @ingroup api_network_settings 00274 * 00275 * @brief Get if DHCP enabled. 00276 */ 00277 WiconnectResult getDhcpEnabled(bool *enabledPtr); 00278 00279 /** 00280 * @ingroup api_network_settings 00281 * 00282 * @brief Set static IP settings 00283 */ 00284 WiconnectResult setIpSettings(uint32_t ip, uint32_t netmask, uint32_t gateway); 00285 00286 /** 00287 * @ingroup api_network_settings 00288 * 00289 * @brief Set static IP settings (with string parameters) 00290 */ 00291 WiconnectResult setIpSettings(const char* ip, const char* netmask, const char* gateway); 00292 00293 /** 00294 * @ingroup api_network_settings 00295 * 00296 * @brief Get network IP settings 00297 */ 00298 WiconnectResult getIpSettings(uint32_t *ip, uint32_t *netmask, uint32_t *gateway); 00299 00300 /** 00301 * @ingroup api_network_settings 00302 * 00303 * @brief Set static DNS Address 00304 */ 00305 WiconnectResult setDnsAddress(uint32_t dnsAddress); 00306 00307 /** 00308 * @ingroup api_network_settings 00309 * 00310 * @brief Get the static DNS address 00311 */ 00312 WiconnectResult getDnsAddress(uint32_t *dnsAddress); 00313 00314 /** 00315 * @ingroup api_network_settings 00316 * 00317 * @note This method is only supported in blocking mode. 00318 * 00319 * @brief Return the current IP address of the module if possible, else 00320 * return 0.0.0.0 00321 * @param[in] buffer Optional, buffer to IP address string. If omitted, 00322 * the IP address string is stored in a local static buffer (this is non-reentrant!) 00323 */ 00324 const char* getIpAddress(char *buffer ALLOW_NULL_STRING_BUFFER); 00325 00326 00327 // ------------------------------------------------------------------------ 00328 00329 00330 /** 00331 * @ingroup conversion_util 00332 * 00333 * @brief Convert string to IP address 00334 */ 00335 static bool strToIp(const char *str, uint32_t *intPtr); 00336 00337 /** 00338 * @ingroup conversion_util 00339 * 00340 * @brief Convert IP address to string 00341 */ 00342 static const char* ipToStr(uint32_t ip, char *ipStrBuffer ALLOW_NULL_STRING_BUFFER); 00343 00344 00345 /** 00346 * @ingroup conversion_util 00347 * 00348 * @brief Convert @ref NetworkStatus to string 00349 */ 00350 static const char* networkStatusToStr(NetworkStatus status); 00351 00352 /** 00353 * @ingroup conversion_util 00354 * 00355 * @brief Convert @ref NetworkJoinResult to string 00356 */ 00357 static const char* networkJoinResultToStr(NetworkJoinResult joinResult); 00358 00359 /** 00360 * @ingroup conversion_util 00361 * 00362 * @brief Convert @ref NetworkSignalStrength to string 00363 */ 00364 static const char* signalStrengthToStr(NetworkSignalStrength signalStrenth); 00365 00366 /** 00367 * @ingroup conversion_util 00368 * 00369 * @brief Convert RSSI (in dBm) to @ref NetworkSignalStrength 00370 */ 00371 static NetworkSignalStrength rssiToSignalStrength(int rssi); 00372 00373 /** 00374 * @ingroup conversion_util 00375 * 00376 * @brief Convert string to @ref NetworkSecurity 00377 */ 00378 static NetworkSecurity strToNetworkSecurity(const char *str); 00379 00380 /** 00381 * @ingroup conversion_util 00382 * 00383 * @brief Convert @ref NetworkSecurity to string 00384 */ 00385 static const char* networkSecurityToStr(NetworkSecurity security); 00386 00387 /** 00388 * @ingroup conversion_util 00389 * 00390 * @brief Convert string @ref Ssid 00391 */ 00392 static bool strToSsid(const char *str, Ssid *ssid); 00393 00394 /** 00395 * @ingroup conversion_util 00396 * 00397 * @brief Convert @ref Ssid to string 00398 */ 00399 static const char* ssidToStr(const Ssid *ssid, char *ssidStrBuffer ALLOW_NULL_STRING_BUFFER); 00400 00401 /** 00402 * @ingroup conversion_util 00403 * 00404 * @brief Convert string @ref MacAddress 00405 */ 00406 static bool strToMacAddress(const char *str, MacAddress *macAddress); 00407 00408 /** 00409 * @ingroup conversion_util 00410 * 00411 * @brief Convert @ref MacAddress to string 00412 */ 00413 static const char* macAddressToStr(const MacAddress *macAddress, char *macStrBuffer ALLOW_NULL_STRING_BUFFER); 00414 00415 protected: 00416 NetworkInterface(Wiconnect *wiconnect); 00417 00418 WiconnectResult processScanResults(char *resultStr, ScanResultList &resultList); 00419 00420 #ifdef WICONNECT_ASYNC_TIMER_ENABLED 00421 Callback completeHandler; 00422 PeriodicTimer monitorTimer; 00423 00424 void webSetupStatusMonitor(); 00425 void webSetupStatusCheckCallback(WiconnectResult result, void *arg1, void *arg2); 00426 00427 void joinStatusMonitor(); 00428 void joinStatusCheckCallback(WiconnectResult result, void *arg1, void *arg2); 00429 00430 //void scanCompleteCallback(WiconnectResult result, void *arg1, void *arg2); 00431 #endif 00432 00433 private: 00434 Wiconnect *wiconnect; 00435 }; 00436 00437 }
Generated on Tue Jul 12 2022 17:35:58 by
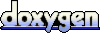