USBHost library with fixes
Dependencies: mbed-rtos FATFileSystem
WANDongle.cpp
00001 /* Copyright (c) 2010-2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "USBHostConf.h" 00020 00021 #ifdef USBHOST_3GMODULE 00022 00023 #define __DEBUG__ 0 00024 #ifndef __MODULE__ 00025 #define __MODULE__ "WANDongle.cpp" 00026 #endif 00027 00028 #include "dbg.h" 00029 #include <stdint.h> 00030 #include "rtos.h" 00031 00032 #include "WANDongle.h" 00033 #include "WANDongleInitializer.h" 00034 00035 WANDongle::WANDongle() : m_pInitializer(NULL), m_serialCount(0), m_totalInitializers(0) 00036 { 00037 host = USBHost::getHostInst(); 00038 init(); 00039 } 00040 00041 00042 bool WANDongle::connected() { 00043 return dev_connected; 00044 } 00045 00046 bool WANDongle::tryConnect() 00047 { 00048 //FIXME should run on USB thread 00049 00050 USB_DBG("Trying to connect device"); 00051 00052 if (dev_connected) { 00053 return true; 00054 } 00055 00056 m_pInitializer = NULL; 00057 00058 for (int i = 0; i < MAX_DEVICE_CONNECTED; i++) 00059 { 00060 if ((dev = host->getDevice(i)) != NULL) 00061 { 00062 m_pInitializer = NULL; //Will be set in setVidPid callback 00063 00064 USB_DBG("Enumerate"); 00065 int ret = host->enumerate(dev, this); 00066 if(ret) 00067 { 00068 return false; 00069 } 00070 00071 USB_DBG("Device has VID:%04x PID:%04x", dev->getVid(), dev->getPid()); 00072 00073 if(m_pInitializer) //If an initializer has been found 00074 { 00075 USB_DBG("m_pInitializer=%p", m_pInitializer); 00076 USB_DBG("m_pInitializer->getSerialVid()=%04x", m_pInitializer->getSerialVid()); 00077 USB_DBG("m_pInitializer->getSerialPid()=%04x", m_pInitializer->getSerialPid()); 00078 if ((dev->getVid() == m_pInitializer->getSerialVid()) && (dev->getPid() == m_pInitializer->getSerialPid())) 00079 { 00080 USB_DBG("The dongle is in virtual serial mode"); 00081 host->registerDriver(dev, 0, this, &WANDongle::init); 00082 m_serialCount = m_pInitializer->getSerialPortCount(); 00083 if( m_serialCount > WANDONGLE_MAX_SERIAL_PORTS ) 00084 { 00085 m_serialCount = WANDONGLE_MAX_SERIAL_PORTS; 00086 } 00087 for(int j = 0; j < m_serialCount; j++) 00088 { 00089 USB_DBG("Connecting serial port #%d", j+1); 00090 USB_DBG("Ep %p", m_pInitializer->getEp(dev, j, false)); 00091 USB_DBG("Ep %p", m_pInitializer->getEp(dev, j, true)); 00092 m_serial[j].connect( dev, m_pInitializer->getEp(dev, j, false), m_pInitializer->getEp(dev, j, true) ); 00093 } 00094 00095 USB_DBG("Device connected"); 00096 00097 dev_connected = true; 00098 00099 00100 return true; 00101 } 00102 else if ((dev->getVid() == m_pInitializer->getMSDVid()) && (dev->getPid() == m_pInitializer->getMSDPid())) 00103 { 00104 USB_DBG("Vodafone K3370 dongle detected in MSD mode"); 00105 //Try to switch 00106 if( m_pInitializer->switchMode(dev) ) 00107 { 00108 USB_DBG("Switched OK"); 00109 return false; //Will be connected on a next iteration 00110 } 00111 else 00112 { 00113 USB_ERR("Could not switch mode"); 00114 return false; 00115 } 00116 } 00117 } //if() 00118 } //if() 00119 } //for() 00120 return false; 00121 } 00122 00123 bool WANDongle::disconnect() 00124 { 00125 dev_connected = false; 00126 for(int i = 0; i < WANDONGLE_MAX_SERIAL_PORTS; i++) 00127 { 00128 m_serial[i].disconnect(); 00129 } 00130 return true; 00131 } 00132 00133 int WANDongle::getDongleType() 00134 { 00135 if( m_pInitializer != NULL ) 00136 { 00137 return m_pInitializer->getType(); 00138 } 00139 else 00140 { 00141 return WAN_DONGLE_TYPE_UNKNOWN; 00142 } 00143 } 00144 00145 IUSBHostSerial& WANDongle::getSerial(int index) 00146 { 00147 return m_serial[index]; 00148 } 00149 00150 int WANDongle::getSerialCount() 00151 { 00152 return m_serialCount; 00153 } 00154 00155 //Private methods 00156 void WANDongle::init() 00157 { 00158 m_pInitializer = NULL; 00159 dev_connected = false; 00160 for(int i = 0; i < WANDONGLE_MAX_SERIAL_PORTS; i++) 00161 { 00162 m_serial[i].init(host); 00163 } 00164 } 00165 00166 00167 /*virtual*/ void WANDongle::setVidPid(uint16_t vid, uint16_t pid) 00168 { 00169 WANDongleInitializer* initializer; 00170 00171 for(int i = 0; i < m_totalInitializers; i++) 00172 { 00173 initializer = m_Initializers[i]; 00174 USB_DBG("initializer=%p", initializer); 00175 USB_DBG("initializer->getSerialVid()=%04x", initializer->getSerialVid()); 00176 USB_DBG("initializer->getSerialPid()=%04x", initializer->getSerialPid()); 00177 if ((dev->getVid() == initializer->getSerialVid()) && (dev->getPid() == initializer->getSerialPid())) 00178 { 00179 USB_DBG("The dongle is in virtual serial mode"); 00180 m_pInitializer = initializer; 00181 break; 00182 } 00183 else if ((dev->getVid() == initializer->getMSDVid()) && (dev->getPid() == initializer->getMSDPid())) 00184 { 00185 USB_DBG("Dongle detected in MSD mode"); 00186 m_pInitializer = initializer; 00187 break; 00188 } 00189 initializer++; 00190 } //for 00191 if(m_pInitializer) 00192 { 00193 m_pInitializer->setVidPid(vid, pid); 00194 } 00195 } 00196 00197 /*virtual*/ bool WANDongle::parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol) //Must return true if the interface should be parsed 00198 { 00199 if(m_pInitializer) 00200 { 00201 return m_pInitializer->parseInterface(intf_nb, intf_class, intf_subclass, intf_protocol); 00202 } 00203 else 00204 { 00205 return false; 00206 } 00207 } 00208 00209 /*virtual*/ bool WANDongle::useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir) //Must return true if the endpoint will be used 00210 { 00211 if(m_pInitializer) 00212 { 00213 return m_pInitializer->useEndpoint(intf_nb, type, dir); 00214 } 00215 else 00216 { 00217 return false; 00218 } 00219 } 00220 00221 00222 bool WANDongle::addInitializer(WANDongleInitializer* pInitializer) 00223 { 00224 if (m_totalInitializers >= WANDONGLE_MAX_INITIALIZERS) 00225 return false; 00226 m_Initializers[m_totalInitializers++] = pInitializer; 00227 return true; 00228 } 00229 00230 WANDongle::~WANDongle() 00231 { 00232 for(int i = 0; i < m_totalInitializers; i++) 00233 delete m_Initializers[i]; 00234 } 00235 00236 #endif /* USBHOST_3GMODULE */
Generated on Thu Jul 14 2022 04:27:36 by
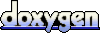