test
Dependencies: ST_INTERFACES X_NUCLEO_COMMON
vl53l0x_class.h
00001 /******************************************************************************* 00002 Copyright © 2016, STMicroelectronics International N.V. 00003 All rights reserved. 00004 00005 Redistribution and use in source and binary forms, with or without 00006 modification, are permitted provided that the following conditions are met: 00007 * Redistributions of source code must retain the above copyright 00008 notice, this list of conditions and the following disclaimer. 00009 * Redistributions in binary form must reproduce the above copyright 00010 notice, this list of conditions and the following disclaimer in the 00011 documentation and/or other materials provided with the distribution. 00012 * Neither the name of STMicroelectronics nor the 00013 names of its contributors may be used to endorse or promote products 00014 derived from this software without specific prior written permission. 00015 00016 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00017 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, AND 00019 NON-INFRINGEMENT OF INTELLECTUAL PROPERTY RIGHTS ARE DISCLAIMED. 00020 IN NO EVENT SHALL STMICROELECTRONICS INTERNATIONAL N.V. BE LIABLE FOR ANY 00021 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00022 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00023 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00024 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00025 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00026 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00027 *****************************************************************************/ 00028 00029 #ifndef __VL53L0X_CLASS_H 00030 #define __VL53L0X_CLASS_H 00031 00032 00033 #ifdef _MSC_VER 00034 # ifdef VL53L0X_API_EXPORTS 00035 # define VL53L0X_API __declspec(dllexport) 00036 # else 00037 # define VL53L0X_API 00038 # endif 00039 #else 00040 # define VL53L0X_API 00041 #endif 00042 00043 00044 /* Includes ------------------------------------------------------------------*/ 00045 #include "mbed.h" 00046 #include "RangeSensor.h" 00047 #include "DevI2C.h" 00048 00049 #include "vl53l0x_def.h" 00050 #include "vl53l0x_platform.h" 00051 #include "stmpe1600_class.h" 00052 00053 00054 /** 00055 * The device model ID 00056 */ 00057 #define IDENTIFICATION_MODEL_ID 0x000 00058 00059 00060 #define STATUS_OK 0x00 00061 #define STATUS_FAIL 0x01 00062 00063 00064 #define VL53L0X_OsDelay(...) wait_ms(2) // 2 msec delay. can also use wait(float secs)/wait_us(int) 00065 00066 #ifdef USE_EMPTY_STRING 00067 #define VL53L0X_STRING_DEVICE_INFO_NAME "" 00068 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS0 "" 00069 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS1 "" 00070 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS2 "" 00071 #define VL53L0X_STRING_DEVICE_INFO_NAME_ES1 "" 00072 #define VL53L0X_STRING_DEVICE_INFO_TYPE "" 00073 00074 /* PAL ERROR strings */ 00075 #define VL53L0X_STRING_ERROR_NONE "" 00076 #define VL53L0X_STRING_ERROR_CALIBRATION_WARNING "" 00077 #define VL53L0X_STRING_ERROR_MIN_CLIPPED "" 00078 #define VL53L0X_STRING_ERROR_UNDEFINED "" 00079 #define VL53L0X_STRING_ERROR_INVALID_PARAMS "" 00080 #define VL53L0X_STRING_ERROR_NOT_SUPPORTED "" 00081 #define VL53L0X_STRING_ERROR_RANGE_ERROR "" 00082 #define VL53L0X_STRING_ERROR_TIME_OUT "" 00083 #define VL53L0X_STRING_ERROR_MODE_NOT_SUPPORTED "" 00084 #define VL53L0X_STRING_ERROR_BUFFER_TOO_SMALL "" 00085 #define VL53L0X_STRING_ERROR_GPIO_NOT_EXISTING "" 00086 #define VL53L0X_STRING_ERROR_GPIO_FUNCTIONALITY_NOT_SUPPORTED "" 00087 #define VL53L0X_STRING_ERROR_CONTROL_INTERFACE "" 00088 #define VL53L0X_STRING_ERROR_INVALID_COMMAND "" 00089 #define VL53L0X_STRING_ERROR_DIVISION_BY_ZERO "" 00090 #define VL53L0X_STRING_ERROR_REF_SPAD_INIT "" 00091 #define VL53L0X_STRING_ERROR_NOT_IMPLEMENTED "" 00092 00093 #define VL53L0X_STRING_UNKNOW_ERROR_CODE "" 00094 00095 00096 00097 /* Range Status */ 00098 #define VL53L0X_STRING_RANGESTATUS_NONE "" 00099 #define VL53L0X_STRING_RANGESTATUS_RANGEVALID "" 00100 #define VL53L0X_STRING_RANGESTATUS_SIGMA "" 00101 #define VL53L0X_STRING_RANGESTATUS_SIGNAL "" 00102 #define VL53L0X_STRING_RANGESTATUS_MINRANGE "" 00103 #define VL53L0X_STRING_RANGESTATUS_PHASE "" 00104 #define VL53L0X_STRING_RANGESTATUS_HW "" 00105 00106 00107 /* Range Status */ 00108 #define VL53L0X_STRING_STATE_POWERDOWN "" 00109 #define VL53L0X_STRING_STATE_WAIT_STATICINIT "" 00110 #define VL53L0X_STRING_STATE_STANDBY "" 00111 #define VL53L0X_STRING_STATE_IDLE "" 00112 #define VL53L0X_STRING_STATE_RUNNING "" 00113 #define VL53L0X_STRING_STATE_UNKNOWN "" 00114 #define VL53L0X_STRING_STATE_ERROR "" 00115 00116 00117 /* Device Specific */ 00118 #define VL53L0X_STRING_DEVICEERROR_NONE "" 00119 #define VL53L0X_STRING_DEVICEERROR_VCSELCONTINUITYTESTFAILURE "" 00120 #define VL53L0X_STRING_DEVICEERROR_VCSELWATCHDOGTESTFAILURE "" 00121 #define VL53L0X_STRING_DEVICEERROR_NOVHVVALUEFOUND "" 00122 #define VL53L0X_STRING_DEVICEERROR_MSRCNOTARGET "" 00123 #define VL53L0X_STRING_DEVICEERROR_SNRCHECK "" 00124 #define VL53L0X_STRING_DEVICEERROR_RANGEPHASECHECK "" 00125 #define VL53L0X_STRING_DEVICEERROR_SIGMATHRESHOLDCHECK "" 00126 #define VL53L0X_STRING_DEVICEERROR_TCC "" 00127 #define VL53L0X_STRING_DEVICEERROR_PHASECONSISTENCY "" 00128 #define VL53L0X_STRING_DEVICEERROR_MINCLIP "" 00129 #define VL53L0X_STRING_DEVICEERROR_RANGECOMPLETE "" 00130 #define VL53L0X_STRING_DEVICEERROR_ALGOUNDERFLOW "" 00131 #define VL53L0X_STRING_DEVICEERROR_ALGOOVERFLOW "" 00132 #define VL53L0X_STRING_DEVICEERROR_RANGEIGNORETHRESHOLD "" 00133 #define VL53L0X_STRING_DEVICEERROR_UNKNOWN "" 00134 00135 /* Check Enable */ 00136 #define VL53L0X_STRING_CHECKENABLE_SIGMA_FINAL_RANGE "" 00137 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_RATE_FINAL_RANGE "" 00138 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_REF_CLIP "" 00139 #define VL53L0X_STRING_CHECKENABLE_RANGE_IGNORE_THRESHOLD "" 00140 00141 /* Sequence Step */ 00142 #define VL53L0X_STRING_SEQUENCESTEP_TCC "" 00143 #define VL53L0X_STRING_SEQUENCESTEP_DSS "" 00144 #define VL53L0X_STRING_SEQUENCESTEP_MSRC "" 00145 #define VL53L0X_STRING_SEQUENCESTEP_PRE_RANGE "" 00146 #define VL53L0X_STRING_SEQUENCESTEP_FINAL_RANGE "" 00147 #else 00148 #define VL53L0X_STRING_DEVICE_INFO_NAME "VL53L0X cut1.0" 00149 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS0 "VL53L0X TS0" 00150 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS1 "VL53L0X TS1" 00151 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS2 "VL53L0X TS2" 00152 #define VL53L0X_STRING_DEVICE_INFO_NAME_ES1 "VL53L0X ES1 or later" 00153 #define VL53L0X_STRING_DEVICE_INFO_TYPE "VL53L0X" 00154 00155 /* PAL ERROR strings */ 00156 #define VL53L0X_STRING_ERROR_NONE \ 00157 "No Error" 00158 #define VL53L0X_STRING_ERROR_CALIBRATION_WARNING \ 00159 "Calibration Warning Error" 00160 #define VL53L0X_STRING_ERROR_MIN_CLIPPED \ 00161 "Min clipped error" 00162 #define VL53L0X_STRING_ERROR_UNDEFINED \ 00163 "Undefined error" 00164 #define VL53L0X_STRING_ERROR_INVALID_PARAMS \ 00165 "Invalid parameters error" 00166 #define VL53L0X_STRING_ERROR_NOT_SUPPORTED \ 00167 "Not supported error" 00168 #define VL53L0X_STRING_ERROR_RANGE_ERROR \ 00169 "Range error" 00170 #define VL53L0X_STRING_ERROR_TIME_OUT \ 00171 "Time out error" 00172 #define VL53L0X_STRING_ERROR_MODE_NOT_SUPPORTED \ 00173 "Mode not supported error" 00174 #define VL53L0X_STRING_ERROR_BUFFER_TOO_SMALL \ 00175 "Buffer too small" 00176 #define VL53L0X_STRING_ERROR_GPIO_NOT_EXISTING \ 00177 "GPIO not existing" 00178 #define VL53L0X_STRING_ERROR_GPIO_FUNCTIONALITY_NOT_SUPPORTED \ 00179 "GPIO funct not supported" 00180 #define VL53L0X_STRING_ERROR_INTERRUPT_NOT_CLEARED \ 00181 "Interrupt not Cleared" 00182 #define VL53L0X_STRING_ERROR_CONTROL_INTERFACE \ 00183 "Control Interface Error" 00184 #define VL53L0X_STRING_ERROR_INVALID_COMMAND \ 00185 "Invalid Command Error" 00186 #define VL53L0X_STRING_ERROR_DIVISION_BY_ZERO \ 00187 "Division by zero Error" 00188 #define VL53L0X_STRING_ERROR_REF_SPAD_INIT \ 00189 "Reference Spad Init Error" 00190 #define VL53L0X_STRING_ERROR_NOT_IMPLEMENTED \ 00191 "Not implemented error" 00192 00193 #define VL53L0X_STRING_UNKNOW_ERROR_CODE \ 00194 "Unknown Error Code" 00195 00196 00197 00198 /* Range Status */ 00199 #define VL53L0X_STRING_RANGESTATUS_NONE "No Update" 00200 #define VL53L0X_STRING_RANGESTATUS_RANGEVALID "Range Valid" 00201 #define VL53L0X_STRING_RANGESTATUS_SIGMA "Sigma Fail" 00202 #define VL53L0X_STRING_RANGESTATUS_SIGNAL "Signal Fail" 00203 #define VL53L0X_STRING_RANGESTATUS_MINRANGE "Min Range Fail" 00204 #define VL53L0X_STRING_RANGESTATUS_PHASE "Phase Fail" 00205 #define VL53L0X_STRING_RANGESTATUS_HW "Hardware Fail" 00206 00207 00208 /* Range Status */ 00209 #define VL53L0X_STRING_STATE_POWERDOWN "POWERDOWN State" 00210 #define VL53L0X_STRING_STATE_WAIT_STATICINIT \ 00211 "Wait for staticinit State" 00212 #define VL53L0X_STRING_STATE_STANDBY "STANDBY State" 00213 #define VL53L0X_STRING_STATE_IDLE "IDLE State" 00214 #define VL53L0X_STRING_STATE_RUNNING "RUNNING State" 00215 #define VL53L0X_STRING_STATE_UNKNOWN "UNKNOWN State" 00216 #define VL53L0X_STRING_STATE_ERROR "ERROR State" 00217 00218 00219 /* Device Specific */ 00220 #define VL53L0X_STRING_DEVICEERROR_NONE "No Update" 00221 #define VL53L0X_STRING_DEVICEERROR_VCSELCONTINUITYTESTFAILURE \ 00222 "VCSEL Continuity Test Failure" 00223 #define VL53L0X_STRING_DEVICEERROR_VCSELWATCHDOGTESTFAILURE \ 00224 "VCSEL Watchdog Test Failure" 00225 #define VL53L0X_STRING_DEVICEERROR_NOVHVVALUEFOUND \ 00226 "No VHV Value found" 00227 #define VL53L0X_STRING_DEVICEERROR_MSRCNOTARGET \ 00228 "MSRC No Target Error" 00229 #define VL53L0X_STRING_DEVICEERROR_SNRCHECK \ 00230 "SNR Check Exit" 00231 #define VL53L0X_STRING_DEVICEERROR_RANGEPHASECHECK \ 00232 "Range Phase Check Error" 00233 #define VL53L0X_STRING_DEVICEERROR_SIGMATHRESHOLDCHECK \ 00234 "Sigma Threshold Check Error" 00235 #define VL53L0X_STRING_DEVICEERROR_TCC \ 00236 "TCC Error" 00237 #define VL53L0X_STRING_DEVICEERROR_PHASECONSISTENCY \ 00238 "Phase Consistency Error" 00239 #define VL53L0X_STRING_DEVICEERROR_MINCLIP \ 00240 "Min Clip Error" 00241 #define VL53L0X_STRING_DEVICEERROR_RANGECOMPLETE \ 00242 "Range Complete" 00243 #define VL53L0X_STRING_DEVICEERROR_ALGOUNDERFLOW \ 00244 "Range Algo Underflow Error" 00245 #define VL53L0X_STRING_DEVICEERROR_ALGOOVERFLOW \ 00246 "Range Algo Overlow Error" 00247 #define VL53L0X_STRING_DEVICEERROR_RANGEIGNORETHRESHOLD \ 00248 "Range Ignore Threshold Error" 00249 #define VL53L0X_STRING_DEVICEERROR_UNKNOWN \ 00250 "Unknown error code" 00251 00252 /* Check Enable */ 00253 #define VL53L0X_STRING_CHECKENABLE_SIGMA_FINAL_RANGE \ 00254 "SIGMA FINAL RANGE" 00255 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_RATE_FINAL_RANGE \ 00256 "SIGNAL RATE FINAL RANGE" 00257 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_REF_CLIP \ 00258 "SIGNAL REF CLIP" 00259 #define VL53L0X_STRING_CHECKENABLE_RANGE_IGNORE_THRESHOLD \ 00260 "RANGE IGNORE THRESHOLD" 00261 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_RATE_MSRC \ 00262 "SIGNAL RATE MSRC" 00263 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_RATE_PRE_RANGE \ 00264 "SIGNAL RATE PRE RANGE" 00265 00266 /* Sequence Step */ 00267 #define VL53L0X_STRING_SEQUENCESTEP_TCC "TCC" 00268 #define VL53L0X_STRING_SEQUENCESTEP_DSS "DSS" 00269 #define VL53L0X_STRING_SEQUENCESTEP_MSRC "MSRC" 00270 #define VL53L0X_STRING_SEQUENCESTEP_PRE_RANGE "PRE RANGE" 00271 #define VL53L0X_STRING_SEQUENCESTEP_FINAL_RANGE "FINAL RANGE" 00272 #endif /* USE_EMPTY_STRING */ 00273 00274 00275 00276 00277 00278 /* sensor operating modes */ 00279 typedef enum 00280 { 00281 range_single_shot_polling=1, 00282 range_continuous_polling, 00283 range_continuous_interrupt, 00284 range_continuous_polling_low_threshold, 00285 range_continuous_polling_high_threshold, 00286 range_continuous_polling_out_of_window, 00287 range_continuous_interrupt_low_threshold, 00288 range_continuous_interrupt_high_threshold, 00289 range_continuous_interrupt_out_of_window, 00290 }OperatingMode; 00291 00292 /** default device address */ 00293 #define DEFAULT_DEVICE_ADDRESS 0x52 /* (8-bit) */ 00294 00295 /* Classes -------------------------------------------------------------------*/ 00296 /** Class representing a VL53L0 sensor component 00297 */ 00298 class VL53L0X : public RangeSensor 00299 { 00300 public: 00301 /** Constructor 00302 * @param[in] &i2c device I2C to be used for communication 00303 * @param[in] &pin_gpio1 pin Mbed InterruptIn PinName to be used as component GPIO_1 INT 00304 * @param[in] DevAddr device address, 0x29 by default 00305 */ 00306 VL53L0X(DevI2C &i2c, DigitalOut &pin, PinName pin_gpio1, uint8_t DevAddr=DEFAULT_DEVICE_ADDRESS) : RangeSensor(), dev_i2c(i2c), gpio0(&pin) 00307 { 00308 MyDevice.I2cDevAddr =DevAddr; 00309 MyDevice.comms_type =1; // VL53L0X_COMMS_I2C 00310 MyDevice.comms_speed_khz =400; 00311 Device=&MyDevice; 00312 expgpio0=NULL; 00313 if (pin_gpio1 != NC) { gpio1Int = new InterruptIn(pin_gpio1); } 00314 else { gpio1Int = NULL; } 00315 } 00316 00317 /** Constructor 2 (STMPE1600DigiOut) 00318 * @param[in] i2c device I2C to be used for communication 00319 * @param[in] &pin Gpio Expander STMPE1600DigiOut pin to be used as component GPIO_0 CE 00320 * @param[in] pin_gpio1 pin Mbed InterruptIn PinName to be used as component GPIO_1 INT 00321 * @param[in] device address, 0x29 by default 00322 */ 00323 VL53L0X(DevI2C &i2c, STMPE1600DigiOut &pin, PinName pin_gpio1, uint8_t DevAddr=DEFAULT_DEVICE_ADDRESS) : RangeSensor(), dev_i2c(i2c), expgpio0(&pin) 00324 { 00325 MyDevice.I2cDevAddr =DevAddr; 00326 MyDevice.comms_type =1; // VL53L0X_COMMS_I2C 00327 MyDevice.comms_speed_khz =400; 00328 Device=&MyDevice; 00329 gpio0=NULL; 00330 if (pin_gpio1 != NC) { gpio1Int = new InterruptIn(pin_gpio1); } 00331 else { gpio1Int = NULL; } 00332 } 00333 00334 /** Destructor 00335 */ 00336 virtual ~VL53L0X(){ 00337 if (gpio1Int != NULL) delete gpio1Int; 00338 } 00339 /* warning: VL53L0X class inherits from GenericSensor, RangeSensor and LightSensor, that haven`t a destructor. 00340 The warning should request to introduce a virtual destructor to make sure to delete the object */ 00341 00342 /*** Interface Methods ***/ 00343 /*** High level API ***/ 00344 /** 00345 * @brief PowerOn the sensor 00346 * @return void 00347 */ 00348 /* turns on the sensor */ 00349 void VL53L0X_On(void) 00350 { 00351 if (gpio0) 00352 *gpio0 = 1; 00353 else if (expgpio0) 00354 *expgpio0 = 1; 00355 wait_ms(1); 00356 } 00357 00358 /** 00359 * @brief PowerOff the sensor 00360 * @return void 00361 */ 00362 /* turns off the sensor */ 00363 void VL53L0X_Off(void) 00364 { 00365 if (gpio0) 00366 *gpio0 = 0; 00367 else if (expgpio0) 00368 *expgpio0 = 0; 00369 wait_ms(1); 00370 } 00371 00372 /** 00373 * @brief Initialize the sensor with default values 00374 * @return 0 on Success 00375 */ 00376 int InitSensor(uint8_t NewAddr); 00377 00378 /** 00379 * @brief Start the measure indicated by operating mode 00380 * @param[in] operating_mode specifies requested measure 00381 * @param[in] fptr specifies call back function must be !NULL in case of interrupt measure 00382 * @return 0 on Success 00383 */ 00384 int StartMeasurement(OperatingMode operating_mode, void (*fptr)(void)); 00385 00386 /** 00387 * @brief Get results for the measure indicated by operating mode 00388 * @param[in] operating_mode specifies requested measure results 00389 * @param[out] Data pointer to the MeasureData_t structure to read data in to 00390 * @return 0 on Success 00391 */ 00392 int GetMeasurement(OperatingMode operating_mode, VL53L0X_RangingMeasurementData_t *Data); 00393 00394 /** 00395 * @brief Stop the currently running measure indicate by operating_mode 00396 * @param[in] operating_mode specifies requested measure to stop 00397 * @return 0 on Success 00398 */ 00399 int StopMeasurement(OperatingMode operating_mode); 00400 00401 /** 00402 * @brief Interrupt handling func to be called by user after an INT is occourred 00403 * @param[in] opeating_mode indicating the in progress measure 00404 * @param[out] Data pointer to the MeasureData_t structure to read data in to 00405 * @return 0 on Success 00406 */ 00407 int HandleIRQ(OperatingMode operating_mode, VL53L0X_RangingMeasurementData_t *Data); 00408 00409 /** 00410 * @brief Enable interrupt measure IRQ 00411 * @return 0 on Success 00412 */ 00413 void EnableInterruptMeasureDetectionIRQ(void) 00414 { 00415 if (gpio1Int != NULL) gpio1Int->enable_irq(); 00416 } 00417 00418 /** 00419 * @brief Disable interrupt measure IRQ 00420 * @return 0 on Success 00421 */ 00422 void DisableInterruptMeasureDetectionIRQ(void) 00423 { 00424 if (gpio1Int != NULL) gpio1Int->disable_irq(); 00425 } 00426 /*** End High level API ***/ 00427 00428 /** 00429 * @brief Attach a function to call when an interrupt is detected, i.e. measurement is ready 00430 * @param[in] fptr pointer to call back function to be called whenever an interrupt occours 00431 * @return 0 on Success 00432 */ 00433 void AttachInterruptMeasureDetectionIRQ(void (*fptr)(void)) 00434 { 00435 if (gpio1Int != NULL) gpio1Int->rise(fptr); 00436 } 00437 00438 /** 00439 * @brief Check the sensor presence 00440 * @return 1 when device is present 00441 */ 00442 unsigned Present() 00443 { 00444 // return Device->Present; 00445 return 1; 00446 } 00447 00448 /** Wrapper functions */ 00449 /** @defgroup api_init Init functions 00450 * @brief API init functions 00451 * @ingroup api_hl 00452 * @{ 00453 */ 00454 /** 00455 * @brief Wait for device booted after chip enable (hardware standby) 00456 * @par Function Description 00457 * After Chip enable Application you can also simply wait at least 1ms to ensure device is ready 00458 * @warning After device chip enable (gpio0) de-asserted user must wait gpio1 to get asserted (hardware standby). 00459 * or wait at least 400usec prior to do any low level access or api call . 00460 * 00461 * This function implements polling for standby but you must ensure 400usec from chip enable passed\n 00462 * @warning if device get prepared @a VL53L0X_Prepare() re-using these function can hold indefinitely\n 00463 * 00464 * @param void 00465 * @return 0 on success 00466 */ 00467 int WaitDeviceBooted() 00468 { 00469 return VL53L0X_WaitDeviceBooted(Device); 00470 // return 1; 00471 } 00472 00473 /** 00474 * 00475 * @brief One time device initialization 00476 * 00477 * To be called once and only once after device is brought out of reset (Chip enable) and booted see @a VL6180x_WaitDeviceBooted() 00478 * 00479 * @par Function Description 00480 * When not used after a fresh device "power up" or reset, it may return @a #CALIBRATION_WARNING 00481 * meaning wrong calibration data may have been fetched from device that can result in ranging offset error\n 00482 * If application cannot execute device reset or need to run VL6180x_InitData multiple time 00483 * then it must ensure proper offset calibration saving and restore on its own 00484 * by using @a VL6180x_GetOffsetCalibrationData() on first power up and then @a VL6180x_SetOffsetCalibrationData() all all subsequent init 00485 * 00486 * @param void 00487 * @return 0 on success, @a #CALIBRATION_WARNING if failed 00488 */ 00489 virtual int Init(void * NewAddr) 00490 { 00491 return VL53L0X_DataInit(Device); 00492 } 00493 00494 /** 00495 * @brief Configure GPIO1 function and set polarity. 00496 * @par Function Description 00497 * To be used prior to arm single shot measure or start continuous mode. 00498 * 00499 * The function uses @a VL6180x_SetupGPIOx() for setting gpio 1. 00500 * @warning changing polarity can generate a spurious interrupt on pins. 00501 * It sets an interrupt flags condition that must be cleared to avoid polling hangs. \n 00502 * It is safe to run VL6180x_ClearAllInterrupt() just after. 00503 * 00504 * @param IntFunction The interrupt functionality to use one of :\n 00505 * @a #GPIOx_SELECT_OFF \n 00506 * @a #GPIOx_SELECT_GPIO_INTERRUPT_OUTPUT 00507 * @param ActiveHigh The interrupt line polarity see ::IntrPol_e 00508 * use @a #INTR_POL_LOW (falling edge) or @a #INTR_POL_HIGH (rising edge) 00509 * @return 0 on success 00510 */ 00511 int SetupGPIO1(uint8_t InitFunction, int ActiveHigh) 00512 { 00513 // return VL6180x_SetupGPIO1(Device, InitFunction, ActiveHigh); 00514 return 1; 00515 } 00516 00517 /** 00518 * @brief Prepare device for operation 00519 * @par Function Description 00520 * Does static initialization and reprogram common default settings \n 00521 * Device is prepared for new measure, ready single shot ranging or ALS typical polling operation\n 00522 * After prepare user can : \n 00523 * @li Call other API function to set other settings\n 00524 * @li Configure the interrupt pins, etc... \n 00525 * @li Then start ranging or ALS operations in single shot or continuous mode 00526 * 00527 * @param void 00528 * @return 0 on success 00529 */ 00530 int Prepare() 00531 { 00532 // taken from rangingTest() in vl53l0x_SingleRanging_Example.c 00533 VL53L0X_Error Status = VL53L0X_ERROR_NONE; 00534 uint32_t refSpadCount; 00535 uint8_t isApertureSpads; 00536 uint8_t VhvSettings; 00537 uint8_t PhaseCal; 00538 00539 if(Status == VL53L0X_ERROR_NONE) 00540 { 00541 //printf ("Call of VL53L0X_StaticInit\n"); 00542 Status = VL53L0X_StaticInit(Device); // Device Initialization 00543 } 00544 00545 if(Status == VL53L0X_ERROR_NONE) 00546 { 00547 //printf ("Call of VL53L0X_PerformRefCalibration\n"); 00548 Status = VL53L0X_PerformRefCalibration(Device, 00549 &VhvSettings, &PhaseCal); // Device Initialization 00550 } 00551 00552 if(Status == VL53L0X_ERROR_NONE) 00553 { 00554 //printf ("Call of VL53L0X_PerformRefSpadManagement\n"); 00555 Status = VL53L0X_PerformRefSpadManagement(Device, 00556 &refSpadCount, &isApertureSpads); // Device Initialization 00557 // printf ("refSpadCount = %d, isApertureSpads = %d\n", refSpadCount, isApertureSpads); 00558 } 00559 00560 return Status; 00561 } 00562 00563 /** 00564 * @brief Start continuous ranging mode 00565 * 00566 * @details End user should ensure device is in idle state and not already running 00567 * @return 0 on success 00568 */ 00569 int RangeStartContinuousMode() 00570 { 00571 // return VL6180x_RangeStartContinuousMode(Device); 00572 return 1; 00573 } 00574 00575 /** 00576 * @brief Start single shot ranging measure 00577 * 00578 * @details End user should ensure device is in idle state and not already running 00579 * @return 0 on success 00580 */ 00581 int RangeStartSingleShot() 00582 { 00583 // return VL6180x_RangeStartSingleShot(Device); 00584 return 1; 00585 } 00586 00587 /** 00588 * @brief Set maximum convergence time 00589 * 00590 * @par Function Description 00591 * Setting a low convergence time can impact maximal detectable distance. 00592 * Refer to VL6180x Datasheet Table 7 : Typical range convergence time. 00593 * A typical value for up to x3 scaling is 50 ms 00594 * 00595 * @param MaxConTime_msec 00596 * @return 0 on success. <0 on error. >0 for calibration warning status 00597 */ 00598 int RangeSetMaxConvergenceTime(uint8_t MaxConTime_msec) 00599 { 00600 // return VL6180x_RangeSetMaxConvergenceTime(Device, MaxConTime_msec); 00601 return 1; 00602 } 00603 00604 /** 00605 * @brief Single shot Range measurement in polling mode. 00606 * 00607 * @par Function Description 00608 * Kick off a new single shot range then wait for ready to retrieve it by polling interrupt status \n 00609 * Ranging must be prepared by a first call to @a VL6180x_Prepare() and it is safer to clear very first poll call \n 00610 * This function reference VL6180x_PollDelay(dev) porting macro/call on each polling loop, 00611 * but PollDelay(dev) may never be called if measure in ready on first poll loop \n 00612 * Should not be use in continuous mode operation as it will stop it and cause stop/start misbehaviour \n 00613 * \n This function clears Range Interrupt status , but not error one. For that uses @a VL6180x_ClearErrorInterrupt() \n 00614 * This range error is not related VL6180x_RangeData_t::errorStatus that refer measure status \n 00615 * 00616 * @param pRangeData Will be populated with the result ranging data @a VL6180x_RangeData_t 00617 * @return 0 on success , @a #RANGE_ERROR if device reports an error case in it status (not cleared) use 00618 * 00619 * \sa ::VL6180x_RangeData_t 00620 */ 00621 int RangePollMeasurement(VL53L0X_RangingMeasurementData_t *pRangeData) 00622 { 00623 // return VL6180x_RangePollMeasurement(Device, pRangeData); 00624 return 1; 00625 } 00626 00627 /** 00628 * @brief Check for measure readiness and get it if ready 00629 * 00630 * @par Function Description 00631 * Using this function is an alternative to @a VL6180x_RangePollMeasurement() to avoid polling operation. This is suitable for applications 00632 * where host CPU is triggered on a interrupt (not from VL6180X) to perform ranging operation. In this scenario, we assume that the very first ranging 00633 * operation is triggered by a call to @a VL6180x_RangeStartSingleShot(). Then, host CPU regularly calls @a VL6180x_RangeGetMeasurementIfReady() to 00634 * get a distance measure if ready. In case the distance is not ready, host may get it at the next call.\n 00635 * 00636 * @warning 00637 * This function does not re-start a new measurement : this is up to the host CPU to do it.\n 00638 * This function clears Range Interrupt for measure ready , but not error interrupts. For that, uses @a VL6180x_ClearErrorInterrupt() \n 00639 * 00640 * @param pRangeData Will be populated with the result ranging data if available 00641 * @return 0 when measure is ready pRange data is updated (untouched when not ready), >0 for warning and @a #NOT_READY if measurement not yet ready, <0 for error @a #RANGE_ERROR if device report an error, 00642 */ 00643 int RangeGetMeasurementIfReady(VL53L0X_RangingMeasurementData_t *pRangeData) 00644 { 00645 // return VL6180x_RangeGetMeasurementIfReady(Device, pRangeData); 00646 return 1; 00647 } 00648 00649 /** 00650 * @brief Retrieve range measurements set from device 00651 * 00652 * @par Function Description 00653 * The measurement is made of range_mm status and error code @a VL6180x_RangeData_t \n 00654 * Based on configuration selected extra measures are included. 00655 * 00656 * @warning should not be used in continuous if wrap around filter is active \n 00657 * Does not perform any wait nor check for result availability or validity. 00658 *\sa VL6180x_RangeGetResult for "range only" measurement 00659 * 00660 * @param pRangeData Pointer to the data structure to fill up 00661 * @return 0 on success 00662 */ 00663 int RangeGetMeasurement(VL53L0X_RangingMeasurementData_t *pRangeData) 00664 { 00665 // return VL6180x_RangeGetMeasurement(Device, pRangeData); 00666 return 1; 00667 } 00668 00669 /** 00670 * @brief Get ranging result and only that 00671 * 00672 * @par Function Description 00673 * Unlike @a VL6180x_RangeGetMeasurement() this function only retrieves the range in millimeter \n 00674 * It does any required up-scale translation\n 00675 * It can be called after success status polling or in interrupt mode \n 00676 * @warning these function is not doing wrap around filtering \n 00677 * This function doesn't perform any data ready check! 00678 * 00679 * @param pRange_mm Pointer to range distance 00680 * @return 0 on success 00681 */ 00682 virtual int GetDistance(uint32_t *piData) 00683 { 00684 int status=0; 00685 VL53L0X_RangingMeasurementData_t pRangingMeasurementData; 00686 00687 status=StartMeasurement(range_single_shot_polling, NULL); 00688 if (!status) { 00689 status=GetMeasurement(range_single_shot_polling, &pRangingMeasurementData); 00690 } 00691 if (pRangingMeasurementData.RangeStatus == 0) { 00692 // we have a valid range. 00693 *piData = pRangingMeasurementData.RangeMilliMeter; 00694 } 00695 else { 00696 *piData = 0; 00697 status = VL53L0X_ERROR_RANGE_ERROR; 00698 } 00699 StopMeasurement(range_single_shot_polling); 00700 return status; 00701 } 00702 00703 /** 00704 * @brief Configure ranging interrupt reported to application 00705 * 00706 * @param ConfigGpioInt Select ranging report\n select one (and only one) of:\n 00707 * @a #CONFIG_GPIO_INTERRUPT_DISABLED \n 00708 * @a #CONFIG_GPIO_INTERRUPT_LEVEL_LOW \n 00709 * @a #CONFIG_GPIO_INTERRUPT_LEVEL_HIGH \n 00710 * @a #CONFIG_GPIO_INTERRUPT_OUT_OF_WINDOW \n 00711 * @a #CONFIG_GPIO_INTERRUPT_NEW_SAMPLE_READY 00712 * @return 0 on success 00713 */ 00714 int RangeConfigInterrupt(uint8_t ConfigGpioInt) 00715 { 00716 // return VL6180x_RangeConfigInterrupt(Device, ConfigGpioInt); 00717 return 1; 00718 } 00719 00720 /** 00721 * @brief Return ranging error interrupt status 00722 * 00723 * @par Function Description 00724 * Appropriate Interrupt report must have been selected first by @a VL6180x_RangeConfigInterrupt() or @a VL6180x_Prepare() \n 00725 * 00726 * Can be used in polling loop to wait for a given ranging event or in interrupt to read the trigger \n 00727 * Events triggers are : \n 00728 * @a #RES_INT_STAT_GPIO_LOW_LEVEL_THRESHOLD \n 00729 * @a #RES_INT_STAT_GPIO_HIGH_LEVEL_THRESHOLD \n 00730 * @a #RES_INT_STAT_GPIO_OUT_OF_WINDOW \n (RES_INT_STAT_GPIO_LOW_LEVEL_THRESHOLD|RES_INT_STAT_GPIO_HIGH_LEVEL_THRESHOLD) 00731 * @a #RES_INT_STAT_GPIO_NEW_SAMPLE_READY \n 00732 * 00733 * @sa IntrStatus_t 00734 * @param pIntStatus Pointer to status variable to update 00735 * @return 0 on success 00736 */ 00737 int RangeGetInterruptStatus(uint8_t *pIntStatus) 00738 { 00739 // return VL6180x_RangeGetInterruptStatus(Device, pIntStatus); 00740 return 1; 00741 } 00742 00743 /** 00744 * @brief Low level ranging and ALS register static settings (you should call @a VL6180x_Prepare() function instead) 00745 * 00746 * @return 0 on success 00747 */ 00748 int StaticInit() 00749 { 00750 // return VL6180x_StaticInit(Device); 00751 return 1; 00752 } 00753 00754 /** 00755 * @brief Wait for device to be ready (before a new ranging command can be issued by application) 00756 * @param MaxLoop Max Number of i2c polling loop see @a #msec_2_i2cloop 00757 * @return 0 on success. <0 when fail \n 00758 * @ref VL6180x_ErrCode_t::TIME_OUT for time out \n 00759 * @ref VL6180x_ErrCode_t::INVALID_PARAMS if MaxLop<1 00760 */ 00761 int RangeWaitDeviceReady(int MaxLoop ) 00762 { 00763 // return VL6180x_RangeWaitDeviceReady(Device, MaxLoop); 00764 return 1; 00765 } 00766 00767 /** 00768 * @brief Program Inter measurement period (used only in continuous mode) 00769 * 00770 * @par Function Description 00771 * When trying to set too long time, it returns #INVALID_PARAMS 00772 * 00773 * @param InterMeasTime_msec Requires inter-measurement time in msec 00774 * @return 0 on success 00775 */ 00776 int RangeSetInterMeasPeriod(uint32_t InterMeasTime_msec) 00777 { 00778 // return VL6180x_RangeSetInterMeasPeriod(Device, InterMeasTime_msec); 00779 return 1; 00780 } 00781 00782 /** 00783 * @brief Set device ranging scaling factor 00784 * 00785 * @par Function Description 00786 * The ranging scaling factor is applied on the raw distance measured by the device to increase operating ranging at the price of the precision. 00787 * Changing the scaling factor when device is not in f/w standby state (free running) is not safe. 00788 * It can be source of spurious interrupt, wrongly scaled range etc ... 00789 * @warning __This function doesns't update high/low threshold and other programmed settings linked to scaling factor__. 00790 * To ensure proper operation, threshold and scaling changes should be done following this procedure: \n 00791 * @li Set Group hold : @a VL6180x_SetGroupParamHold() \n 00792 * @li Get Threshold @a VL6180x_RangeGetThresholds() \n 00793 * @li Change scaling : @a VL6180x_UpscaleSetScaling() \n 00794 * @li Set Threshold : @a VL6180x_RangeSetThresholds() \n 00795 * @li Unset Group Hold : @a VL6180x_SetGroupParamHold() 00796 * 00797 * @param scaling Scaling factor to apply (1,2 or 3) 00798 * @return 0 on success when up-scale support is not configured it fail for any 00799 * scaling than the one statically configured. 00800 */ 00801 int UpscaleSetScaling(uint8_t scaling) 00802 { 00803 // return VL6180x_UpscaleSetScaling(Device, scaling); 00804 return 1; 00805 } 00806 00807 /** 00808 * @brief Get current ranging scaling factor 00809 * 00810 * @return The current scaling factor 00811 */ 00812 int UpscaleGetScaling() 00813 { 00814 // return VL6180x_UpscaleGetScaling(Device); 00815 return 1; 00816 } 00817 00818 /** 00819 * @brief Get the maximal distance for actual scaling 00820 * @par Function Description 00821 * Do not use prior to @a VL6180x_Prepare() or at least @a VL6180x_InitData() 00822 * 00823 * Any range value more than the value returned by this function is to be considered as "no target detected" 00824 * or "no target in detectable range" \n 00825 * @warning The maximal distance depends on the scaling 00826 * 00827 * @return The maximal range limit for actual mode and scaling 00828 */ 00829 uint16_t GetUpperLimit() 00830 { 00831 // return VL6180x_GetUpperLimit(Device); 00832 return 1; 00833 } 00834 00835 /** 00836 * @brief Apply low and high ranging thresholds that are considered only in continuous mode 00837 * 00838 * @par Function Description 00839 * This function programs low and high ranging thresholds that are considered in continuous mode : 00840 * interrupt will be raised only when an object is detected at a distance inside this [low:high] range. 00841 * The function takes care of applying current scaling factor if any.\n 00842 * To be safe, in continuous operation, thresholds must be changed under "group parameter hold" cover. 00843 * Group hold can be activated/deactivated directly in the function or externally (then set 0) 00844 * using /a VL6180x_SetGroupParamHold() function. 00845 * 00846 * @param low Low threshold in mm 00847 * @param high High threshold in mm 00848 * @param SafeHold Use of group parameters hold to surround threshold programming. 00849 * @return 0 On success 00850 */ 00851 int RangeSetThresholds(uint16_t low, uint16_t high, int SafeHold) 00852 { 00853 // return VL6180x_RangeSetThresholds(Device, low, high, SafeHold); 00854 return 1; 00855 } 00856 00857 /** 00858 * @brief Get scaled high and low threshold from device 00859 * 00860 * @par Function Description 00861 * Due to scaling factor, the returned value may be different from what has been programmed first (precision lost). 00862 * For instance VL6180x_RangeSetThresholds(dev,11,22) with scale 3 00863 * will read back 9 ((11/3)x3) and 21 ((22/3)x3). 00864 * 00865 * @param low scaled low Threshold ptr can be NULL if not needed 00866 * @param high scaled High Threshold ptr can be NULL if not needed 00867 * @return 0 on success, return value is undefined if both low and high are NULL 00868 * @warning return value is undefined if both low and high are NULL 00869 */ 00870 int RangeGetThresholds(uint16_t *low, uint16_t *high) 00871 { 00872 // return VL6180x_RangeGetThresholds(Device, low, high); 00873 return 1; 00874 } 00875 00876 /** 00877 * @brief Set ranging raw thresholds (scaling not considered so not recommended to use it) 00878 * 00879 * @param low raw low threshold set to raw register 00880 * @param high raw high threshold set to raw register 00881 * @return 0 on success 00882 */ 00883 int RangeSetRawThresholds(uint8_t low, uint8_t high) 00884 { 00885 // return VL6180x_RangeSetRawThresholds(Device, low, high); 00886 return 1; 00887 } 00888 00889 /** 00890 * @brief Set Early Convergence Estimate ratio 00891 * @par Function Description 00892 * For more information on ECE check datasheet 00893 * @warning May return a calibration warning in some use cases 00894 * 00895 * @param FactorM ECE factor M in M/D 00896 * @param FactorD ECE factor D in M/D 00897 * @return 0 on success. <0 on error. >0 on warning 00898 */ 00899 int RangeSetEceFactor(uint16_t FactorM, uint16_t FactorD) 00900 { 00901 // return VL6180x_RangeSetEceFactor(Device, FactorM, FactorD); 00902 return 1; 00903 } 00904 00905 /** 00906 * @brief Set Early Convergence Estimate state (See #SYSRANGE_RANGE_CHECK_ENABLES register) 00907 * @param enable State to be set 0=disabled, otherwise enabled 00908 * @return 0 on success 00909 */ 00910 int RangeSetEceState(int enable) 00911 { 00912 // return VL6180x_RangeSetEceState(Device, enable); 00913 return 1; 00914 } 00915 00916 /** 00917 * @brief Set activation state of the wrap around filter 00918 * @param state New activation state (0=off, otherwise on) 00919 * @return 0 on success 00920 */ 00921 int FilterSetState(int state) 00922 { 00923 // return VL6180x_FilterSetState(Device, state); 00924 return 1; 00925 } 00926 00927 /** 00928 * Get activation state of the wrap around filter 00929 * @return Filter enabled or not, when filter is not supported it always returns 0S 00930 */ 00931 int FilterGetState() 00932 { 00933 // return VL6180x_FilterGetState(Device); 00934 return 1; 00935 } 00936 00937 /** 00938 * @brief Set activation state of DMax computation 00939 * @param state New activation state (0=off, otherwise on) 00940 * @return 0 on success 00941 */ 00942 int DMaxSetState(int state) 00943 { 00944 // return VL6180x_DMaxSetState(Device, state); 00945 return 1; 00946 } 00947 00948 /** 00949 * Get activation state of DMax computation 00950 * @return Filter enabled or not, when filter is not supported it always returns 0S 00951 */ 00952 int DMaxGetState() 00953 { 00954 // return VL6180x_DMaxGetState(Device); 00955 return 1; 00956 } 00957 00958 /** 00959 * @brief Set ranging mode and start/stop measure (use high level functions instead : @a VL6180x_RangeStartSingleShot() or @a VL6180x_RangeStartContinuousMode()) 00960 * 00961 * @par Function Description 00962 * When used outside scope of known polling single shot stopped state, \n 00963 * user must ensure the device state is "idle" before to issue a new command. 00964 * 00965 * @param mode A combination of working mode (#MODE_SINGLESHOT or #MODE_CONTINUOUS) and start/stop condition (#MODE_START_STOP) \n 00966 * @return 0 on success 00967 */ 00968 int RangeSetSystemMode(uint8_t mode) 00969 { 00970 // return VL6180x_RangeSetSystemMode(Device, mode); 00971 return 1; 00972 } 00973 00974 /** @} */ 00975 00976 /** @defgroup api_ll_range_calibration Ranging calibration functions 00977 * @brief Ranging calibration functions 00978 * @ingroup api_ll 00979 * @{ 00980 */ 00981 /** 00982 * @brief Get part to part calibration offset 00983 * 00984 * @par Function Description 00985 * Should only be used after a successful call to @a VL6180x_InitData to backup device nvm value 00986 * 00987 * @return part to part calibration offset from device 00988 */ 00989 int8_t GetOffsetCalibrationData() 00990 { 00991 // return VL6180x_GetOffsetCalibrationData(Device); 00992 return 1; 00993 } 00994 00995 /** 00996 * Set or over-write part to part calibration offset 00997 * \sa VL6180x_InitData(), VL6180x_GetOffsetCalibrationData() 00998 * @param offset Offset 00999 */ 01000 void SetOffsetCalibrationData(int8_t offset) 01001 { 01002 // return VL6180x_SetOffsetCalibrationData(Device, offset); 01003 return; 01004 } 01005 01006 /** 01007 * @brief Set Cross talk compensation rate 01008 * 01009 * @par Function Description 01010 * It programs register @a #SYSRANGE_CROSSTALK_COMPENSATION_RATE 01011 * 01012 * @param Rate Compensation rate (9.7 fix point) see datasheet for details 01013 * @return 0 on success 01014 */ 01015 int SetXTalkCompensationRate(uint16_t Rate) 01016 { 01017 // return VL6180x_SetXTalkCompensationRate(Device, Rate); 01018 return 1; 01019 } 01020 /** @} */ 01021 01022 /** 01023 * @brief Set new device i2c address 01024 * 01025 * After completion the device will answer to the new address programmed. 01026 * 01027 * @sa AN4478: Using multiple VL6180X's in a single design 01028 * @param NewAddr The new i2c address (7bit) 01029 * @return 0 on success 01030 */ 01031 int SetDeviceAddress(int NewAddr) 01032 { 01033 int status; 01034 01035 status=VL53L0X_SetDeviceAddress(Device, NewAddr); 01036 if(!status) 01037 Device->I2cDevAddr =NewAddr; 01038 return status; 01039 01040 // return 1; 01041 } 01042 01043 /** 01044 * @brief Fully configure gpio 0/1 pin : polarity and functionality 01045 * 01046 * @param pin gpio pin 0 or 1 01047 * @param IntFunction Pin functionality : either #GPIOx_SELECT_OFF or #GPIOx_SELECT_GPIO_INTERRUPT_OUTPUT (refer to #SYSTEM_MODE_GPIO1 register definition) 01048 * @param ActiveHigh Set active high polarity, or active low see @a ::IntrPol_e 01049 * @return 0 on success 01050 */ 01051 int SetupGPIOx(int pin, uint8_t IntFunction, int ActiveHigh) 01052 { 01053 // return VL6180x_SetupGPIOx(Device, pin, IntFunction, ActiveHigh); 01054 return 1; 01055 } 01056 01057 /** 01058 * @brief Set interrupt pin polarity for the given GPIO 01059 * 01060 * @param pin Pin 0 or 1 01061 * @param active_high select active high or low polarity using @ref IntrPol_e 01062 * @return 0 on success 01063 */ 01064 int SetGPIOxPolarity(int pin, int active_high) 01065 { 01066 // return VL6180x_SetGPIOxPolarity(Device, pin, active_high); 01067 return 1; 01068 } 01069 01070 /** 01071 * Select interrupt functionality for the given GPIO 01072 * 01073 * @par Function Description 01074 * Functionality refer to @a SYSTEM_MODE_GPIO0 01075 * 01076 * @param pin Pin to configure 0 or 1 (gpio0 or gpio1)\nNote that gpio0 is chip enable at power up ! 01077 * @param functionality Pin functionality : either #GPIOx_SELECT_OFF or #GPIOx_SELECT_GPIO_INTERRUPT_OUTPUT (refer to #SYSTEM_MODE_GPIO1 register definition) 01078 * @return 0 on success 01079 */ 01080 int SetGPIOxFunctionality(int pin, uint8_t functionality) 01081 { 01082 // return VL6180x_SetGPIOxFunctionality(Device, pin, functionality); 01083 return 1; 01084 } 01085 01086 /** 01087 * #brief Disable and turn to Hi-Z gpio output pin 01088 * 01089 * @param pin The pin number to disable 0 or 1 01090 * @return 0 on success 01091 */ 01092 int DisableGPIOxOut(int pin) 01093 { 01094 // return VL6180x_DisableGPIOxOut(Device, pin); 01095 return 1; 01096 } 01097 01098 /** @} */ 01099 01100 /** @defgroup api_ll_intr Interrupts management functions 01101 * @brief Interrupts management functions 01102 * @ingroup api_ll 01103 * @{ 01104 */ 01105 01106 /** 01107 * @brief Get all interrupts cause 01108 * 01109 * @param status Ptr to interrupt status. You can use @a IntrStatus_t::val 01110 * @return 0 on success 01111 */ 01112 int GetInterruptStatus(uint8_t *status) 01113 { 01114 // return VL6180x_GetInterruptStatus(Device, status); 01115 return 1; 01116 } 01117 01118 /** 01119 * @brief Clear given system interrupt condition 01120 * 01121 * @par Function Description 01122 * Clear given interrupt cause by writing into register #SYSTEM_INTERRUPT_CLEAR register. 01123 * @param dev The device 01124 * @param IntClear Which interrupt source to clear. Use any combinations of #INTERRUPT_CLEAR_RANGING , #INTERRUPT_CLEAR_ALS , #INTERRUPT_CLEAR_ERROR. 01125 * @return 0 On success 01126 */ 01127 int ClearInterrupt(uint8_t IntClear) 01128 { 01129 // return VL6180x_ClearInterrupt(Device, IntClear ); 01130 return 1; 01131 } 01132 01133 /** 01134 * @brief Clear error interrupt 01135 * 01136 * @param dev The device 01137 * @return 0 On success 01138 */ 01139 #define VL6180x_ClearErrorInterrupt(dev) VL6180x_ClearInterrupt(dev, INTERRUPT_CLEAR_ERROR) 01140 01141 /** 01142 * @brief Clear All interrupt causes (als+range+error) 01143 * 01144 * @param dev The device 01145 * @return 0 On success 01146 */ 01147 #define VL6180x_ClearAllInterrupt(dev) VL6180x_ClearInterrupt(dev, INTERRUPT_CLEAR_ERROR|INTERRUPT_CLEAR_RANGING|INTERRUPT_CLEAR_ALS) 01148 01149 01150 private: 01151 /* api.h functions */ 01152 VL53L0X_Error VL53L0X_DataInit(VL53L0X_DEV Dev); 01153 VL53L0X_Error VL53L0X_GetOffsetCalibrationDataMicroMeter(VL53L0X_DEV Dev, int32_t *pOffsetCalibrationDataMicroMeter); 01154 VL53L0X_Error VL53L0X_SetOffsetCalibrationDataMicroMeter(VL53L0X_DEV Dev, 01155 int32_t OffsetCalibrationDataMicroMeter); 01156 VL53L0X_Error VL53L0X_GetDeviceParameters(VL53L0X_DEV Dev, 01157 VL53L0X_DeviceParameters_t *pDeviceParameters); 01158 VL53L0X_Error VL53L0X_GetDeviceMode(VL53L0X_DEV Dev, 01159 VL53L0X_DeviceModes *pDeviceMode); 01160 VL53L0X_Error VL53L0X_GetInterMeasurementPeriodMilliSeconds(VL53L0X_DEV Dev, 01161 uint32_t *pInterMeasurementPeriodMilliSeconds); 01162 VL53L0X_Error VL53L0X_GetXTalkCompensationRateMegaCps(VL53L0X_DEV Dev, 01163 FixPoint1616_t *pXTalkCompensationRateMegaCps); 01164 VL53L0X_Error VL53L0X_GetLimitCheckValue(VL53L0X_DEV Dev, uint16_t LimitCheckId, 01165 FixPoint1616_t *pLimitCheckValue); 01166 VL53L0X_Error VL53L0X_GetLimitCheckEnable(VL53L0X_DEV Dev, uint16_t LimitCheckId, 01167 uint8_t *pLimitCheckEnable); 01168 VL53L0X_Error VL53L0X_GetWrapAroundCheckEnable(VL53L0X_DEV Dev, 01169 uint8_t *pWrapAroundCheckEnable); 01170 VL53L0X_Error VL53L0X_GetMeasurementTimingBudgetMicroSeconds(VL53L0X_DEV Dev, 01171 uint32_t *pMeasurementTimingBudgetMicroSeconds); 01172 VL53L0X_Error VL53L0X_GetSequenceStepEnables(VL53L0X_DEV Dev, 01173 VL53L0X_SchedulerSequenceSteps_t *pSchedulerSequenceSteps); 01174 VL53L0X_Error sequence_step_enabled(VL53L0X_DEV Dev, 01175 VL53L0X_SequenceStepId SequenceStepId, uint8_t SequenceConfig, 01176 uint8_t *pSequenceStepEnabled); 01177 VL53L0X_Error VL53L0X_GetVcselPulsePeriod(VL53L0X_DEV Dev, 01178 VL53L0X_VcselPeriod VcselPeriodType, uint8_t *pVCSELPulsePeriodPCLK); 01179 VL53L0X_Error VL53L0X_GetDeviceInfo(VL53L0X_DEV Dev, 01180 VL53L0X_DeviceInfo_t *pVL53L0X_DeviceInfo); 01181 VL53L0X_Error VL53L0X_StaticInit(VL53L0X_DEV Dev); 01182 VL53L0X_Error VL53L0X_GetMeasurementDataReady(VL53L0X_DEV Dev, 01183 uint8_t *pMeasurementDataReady); 01184 VL53L0X_Error VL53L0X_GetInterruptMaskStatus(VL53L0X_DEV Dev, 01185 uint32_t *pInterruptMaskStatus); 01186 VL53L0X_Error VL53L0X_ClearInterruptMask(VL53L0X_DEV Dev, uint32_t InterruptMask); 01187 VL53L0X_Error VL53L0X_PerformSingleRangingMeasurement(VL53L0X_DEV Dev, 01188 VL53L0X_RangingMeasurementData_t *pRangingMeasurementData); 01189 VL53L0X_Error VL53L0X_SetDeviceMode(VL53L0X_DEV Dev, VL53L0X_DeviceModes DeviceMode); 01190 VL53L0X_Error VL53L0X_PerformSingleMeasurement(VL53L0X_DEV Dev); 01191 VL53L0X_Error VL53L0X_StartMeasurement(VL53L0X_DEV Dev); 01192 VL53L0X_Error VL53L0X_CheckAndLoadInterruptSettings(VL53L0X_DEV Dev, 01193 uint8_t StartNotStopFlag); 01194 VL53L0X_Error VL53L0X_GetInterruptThresholds(VL53L0X_DEV Dev, 01195 VL53L0X_DeviceModes DeviceMode, FixPoint1616_t *pThresholdLow, 01196 FixPoint1616_t *pThresholdHigh); 01197 VL53L0X_Error VL53L0X_GetRangingMeasurementData(VL53L0X_DEV Dev, 01198 VL53L0X_RangingMeasurementData_t *pRangingMeasurementData); 01199 VL53L0X_Error VL53L0X_GetXTalkCompensationEnable(VL53L0X_DEV Dev, 01200 uint8_t *pXTalkCompensationEnable); 01201 VL53L0X_Error VL53L0X_WaitDeviceBooted(VL53L0X_DEV Dev); 01202 VL53L0X_Error VL53L0X_PerformRefCalibration(VL53L0X_DEV Dev, uint8_t *pVhvSettings, 01203 uint8_t *pPhaseCal); 01204 VL53L0X_Error VL53L0X_PerformRefSpadManagement(VL53L0X_DEV Dev, 01205 uint32_t *refSpadCount, uint8_t *isApertureSpads); 01206 VL53L0X_Error VL53L0X_SetDeviceAddress(VL53L0X_DEV Dev, uint8_t DeviceAddress); 01207 VL53L0X_Error VL53L0X_SetGpioConfig(VL53L0X_DEV Dev, uint8_t Pin, 01208 VL53L0X_DeviceModes DeviceMode, VL53L0X_GpioFunctionality Functionality, 01209 VL53L0X_InterruptPolarity Polarity); 01210 VL53L0X_Error VL53L0X_GetFractionEnable(VL53L0X_DEV Dev, uint8_t *pEnabled); 01211 VL53L0X_Error VL53L0X_SetSequenceStepEnable(VL53L0X_DEV Dev, 01212 VL53L0X_SequenceStepId SequenceStepId, uint8_t SequenceStepEnabled); 01213 VL53L0X_Error VL53L0X_SetMeasurementTimingBudgetMicroSeconds(VL53L0X_DEV Dev, 01214 uint32_t MeasurementTimingBudgetMicroSeconds); 01215 VL53L0X_Error VL53L0X_SetLimitCheckEnable(VL53L0X_DEV Dev, uint16_t LimitCheckId, 01216 uint8_t LimitCheckEnable); 01217 VL53L0X_Error VL53L0X_SetLimitCheckValue(VL53L0X_DEV Dev, uint16_t LimitCheckId, 01218 FixPoint1616_t LimitCheckValue); 01219 VL53L0X_Error VL53L0X_StopMeasurement(VL53L0X_DEV Dev); 01220 VL53L0X_Error VL53L0X_GetStopCompletedStatus(VL53L0X_DEV Dev, 01221 uint32_t *pStopStatus); 01222 VL53L0X_Error VL53L0X_SetVcselPulsePeriod(VL53L0X_DEV Dev, 01223 VL53L0X_VcselPeriod VcselPeriodType, uint8_t VCSELPulsePeriod); 01224 01225 /* api_core.h functions */ 01226 VL53L0X_Error VL53L0X_get_info_from_device(VL53L0X_DEV Dev, uint8_t option); 01227 VL53L0X_Error VL53L0X_device_read_strobe(VL53L0X_DEV Dev); 01228 VL53L0X_Error VL53L0X_get_measurement_timing_budget_micro_seconds(VL53L0X_DEV Dev, 01229 uint32_t *pMeasurementTimingBudgetMicroSeconds); 01230 VL53L0X_Error VL53L0X_get_vcsel_pulse_period(VL53L0X_DEV Dev, 01231 VL53L0X_VcselPeriod VcselPeriodType, uint8_t *pVCSELPulsePeriodPCLK); 01232 uint8_t VL53L0X_decode_vcsel_period(uint8_t vcsel_period_reg); 01233 uint32_t VL53L0X_decode_timeout(uint16_t encoded_timeout); 01234 uint32_t VL53L0X_calc_timeout_us(VL53L0X_DEV Dev, 01235 uint16_t timeout_period_mclks, 01236 uint8_t vcsel_period_pclks); 01237 uint32_t VL53L0X_calc_macro_period_ps(VL53L0X_DEV Dev, uint8_t vcsel_period_pclks); 01238 VL53L0X_Error VL53L0X_measurement_poll_for_completion(VL53L0X_DEV Dev); 01239 VL53L0X_Error VL53L0X_load_tuning_settings(VL53L0X_DEV Dev, 01240 uint8_t *pTuningSettingBuffer); 01241 VL53L0X_Error VL53L0X_get_pal_range_status(VL53L0X_DEV Dev, 01242 uint8_t DeviceRangeStatus, 01243 FixPoint1616_t SignalRate, 01244 uint16_t EffectiveSpadRtnCount, 01245 VL53L0X_RangingMeasurementData_t *pRangingMeasurementData, 01246 uint8_t *pPalRangeStatus); 01247 VL53L0X_Error VL53L0X_calc_sigma_estimate(VL53L0X_DEV Dev, 01248 VL53L0X_RangingMeasurementData_t *pRangingMeasurementData, 01249 FixPoint1616_t *pSigmaEstimate, 01250 uint32_t *pDmax_mm); 01251 VL53L0X_Error VL53L0X_get_total_signal_rate(VL53L0X_DEV Dev, 01252 VL53L0X_RangingMeasurementData_t *pRangingMeasurementData, 01253 FixPoint1616_t *ptotal_signal_rate_mcps); 01254 VL53L0X_Error VL53L0X_get_total_xtalk_rate(VL53L0X_DEV Dev, 01255 VL53L0X_RangingMeasurementData_t *pRangingMeasurementData, 01256 FixPoint1616_t *ptotal_xtalk_rate_mcps); 01257 uint32_t VL53L0X_calc_timeout_mclks(VL53L0X_DEV Dev, 01258 uint32_t timeout_period_us, 01259 uint8_t vcsel_period_pclks); 01260 uint32_t VL53L0X_isqrt(uint32_t num); 01261 VL53L0X_Error VL53L0X_calc_dmax( 01262 VL53L0X_DEV Dev, 01263 FixPoint1616_t totalSignalRate_mcps, 01264 FixPoint1616_t totalCorrSignalRate_mcps, 01265 FixPoint1616_t pwMult, 01266 uint32_t sigmaEstimateP1, 01267 FixPoint1616_t sigmaEstimateP2, 01268 uint32_t peakVcselDuration_us, 01269 uint32_t *pdmax_mm); 01270 VL53L0X_Error VL53L0X_set_measurement_timing_budget_micro_seconds(VL53L0X_DEV Dev, 01271 uint32_t MeasurementTimingBudgetMicroSeconds); 01272 VL53L0X_Error get_sequence_step_timeout(VL53L0X_DEV Dev, 01273 VL53L0X_SequenceStepId SequenceStepId, 01274 uint32_t *pTimeOutMicroSecs); 01275 VL53L0X_Error set_sequence_step_timeout(VL53L0X_DEV Dev, 01276 VL53L0X_SequenceStepId SequenceStepId, 01277 uint32_t TimeOutMicroSecs); 01278 uint16_t VL53L0X_encode_timeout(uint32_t timeout_macro_clks); 01279 VL53L0X_Error VL53L0X_set_vcsel_pulse_period(VL53L0X_DEV Dev, 01280 VL53L0X_VcselPeriod VcselPeriodType, uint8_t VCSELPulsePeriodPCLK); 01281 uint8_t VL53L0X_encode_vcsel_period(uint8_t vcsel_period_pclks); 01282 01283 /* api_calibration.h functions */ 01284 VL53L0X_Error VL53L0X_apply_offset_adjustment(VL53L0X_DEV Dev); 01285 VL53L0X_Error VL53L0X_get_offset_calibration_data_micro_meter(VL53L0X_DEV Dev, 01286 int32_t *pOffsetCalibrationDataMicroMeter); 01287 VL53L0X_Error VL53L0X_set_offset_calibration_data_micro_meter(VL53L0X_DEV Dev, 01288 int32_t OffsetCalibrationDataMicroMeter); 01289 VL53L0X_Error VL53L0X_perform_ref_spad_management(VL53L0X_DEV Dev, 01290 uint32_t *refSpadCount, 01291 uint8_t *isApertureSpads); 01292 VL53L0X_Error VL53L0X_perform_ref_calibration(VL53L0X_DEV Dev, 01293 uint8_t *pVhvSettings, uint8_t *pPhaseCal, uint8_t get_data_enable); 01294 VL53L0X_Error VL53L0X_perform_vhv_calibration(VL53L0X_DEV Dev, 01295 uint8_t *pVhvSettings, const uint8_t get_data_enable, 01296 const uint8_t restore_config); 01297 VL53L0X_Error VL53L0X_perform_single_ref_calibration(VL53L0X_DEV Dev, 01298 uint8_t vhv_init_byte); 01299 VL53L0X_Error VL53L0X_ref_calibration_io(VL53L0X_DEV Dev, uint8_t read_not_write, 01300 uint8_t VhvSettings, uint8_t PhaseCal, 01301 uint8_t *pVhvSettings, uint8_t *pPhaseCal, 01302 const uint8_t vhv_enable, const uint8_t phase_enable); 01303 VL53L0X_Error VL53L0X_perform_phase_calibration(VL53L0X_DEV Dev, 01304 uint8_t *pPhaseCal, const uint8_t get_data_enable, 01305 const uint8_t restore_config); 01306 VL53L0X_Error enable_ref_spads(VL53L0X_DEV Dev, 01307 uint8_t apertureSpads, 01308 uint8_t goodSpadArray[], 01309 uint8_t spadArray[], 01310 uint32_t size, 01311 uint32_t start, 01312 uint32_t offset, 01313 uint32_t spadCount, 01314 uint32_t *lastSpad); 01315 void get_next_good_spad(uint8_t goodSpadArray[], uint32_t size, 01316 uint32_t curr, int32_t *next); 01317 uint8_t is_aperture(uint32_t spadIndex); 01318 VL53L0X_Error enable_spad_bit(uint8_t spadArray[], uint32_t size, 01319 uint32_t spadIndex); 01320 VL53L0X_Error set_ref_spad_map(VL53L0X_DEV Dev, uint8_t *refSpadArray); 01321 VL53L0X_Error get_ref_spad_map(VL53L0X_DEV Dev, uint8_t *refSpadArray); 01322 VL53L0X_Error perform_ref_signal_measurement(VL53L0X_DEV Dev, 01323 uint16_t *refSignalRate); 01324 VL53L0X_Error VL53L0X_set_reference_spads(VL53L0X_DEV Dev, 01325 uint32_t count, uint8_t isApertureSpads); 01326 01327 /* api_strings.h functions */ 01328 VL53L0X_Error VL53L0X_get_device_info(VL53L0X_DEV Dev, 01329 VL53L0X_DeviceInfo_t *pVL53L0X_DeviceInfo); 01330 VL53L0X_Error VL53L0X_check_part_used(VL53L0X_DEV Dev, 01331 uint8_t *Revision, 01332 VL53L0X_DeviceInfo_t *pVL53L0X_DeviceInfo); 01333 01334 /* Read function of the ID device */ 01335 virtual int ReadID(uint8_t *id); 01336 01337 VL53L0X_Error WaitMeasurementDataReady(VL53L0X_DEV Dev); 01338 VL53L0X_Error WaitStopCompleted(VL53L0X_DEV Dev); 01339 01340 /* Write and read functions from I2C */ 01341 01342 VL53L0X_Error VL53L0X_WrByte(VL53L0X_DEV dev, uint8_t index, uint8_t data); 01343 VL53L0X_Error VL53L0X_WrWord(VL53L0X_DEV dev, uint8_t index, uint16_t data); 01344 VL53L0X_Error VL53L0X_WrDWord(VL53L0X_DEV dev, uint8_t index, uint32_t data); 01345 VL53L0X_Error VL53L0X_RdByte(VL53L0X_DEV dev, uint8_t index, uint8_t *data); 01346 VL53L0X_Error VL53L0X_RdWord(VL53L0X_DEV dev, uint8_t index, uint16_t *data); 01347 VL53L0X_Error VL53L0X_RdDWord(VL53L0X_DEV dev, uint8_t index, uint32_t *data); 01348 VL53L0X_Error VL53L0X_UpdateByte(VL53L0X_DEV dev, uint8_t index, uint8_t AndData, uint8_t OrData); 01349 01350 VL53L0X_Error VL53L0X_WriteMulti(VL53L0X_DEV Dev, uint8_t index, uint8_t *pdata, uint32_t count); 01351 VL53L0X_Error VL53L0X_ReadMulti(VL53L0X_DEV Dev, uint8_t index, uint8_t *pdata, uint32_t count); 01352 01353 VL53L0X_Error VL53L0X_I2CWrite(uint8_t dev, uint8_t index, uint8_t *data, uint16_t number_of_bytes); 01354 VL53L0X_Error VL53L0X_I2CRead(uint8_t dev, uint8_t index, uint8_t *data, uint16_t number_of_bytes); 01355 01356 VL53L0X_Error VL53L0X_PollingDelay(VL53L0X_DEV Dev); /* usually best implemented as a real function */ 01357 01358 int IsPresent() 01359 { 01360 int status; 01361 01362 status=ReadID((uint8_t *)MyDevice.I2cDevAddr ); 01363 if(status) 01364 VL53L0X_ErrLog("Failed to read ID device. Device not present!\n\r"); 01365 return status; 01366 } 01367 int StopRangeMeasurement(OperatingMode operating_mode); 01368 int GetRangeMeas(OperatingMode operating_mode, VL53L0X_RangingMeasurementData_t *Data); 01369 int RangeSetLowThreshold(uint16_t threshold); 01370 int RangeSetHighThreshold(uint16_t threshold); 01371 int GetRangeError(VL53L0X_RangingMeasurementData_t *Data, VL53L0X_RangingMeasurementData_t RangeData); 01372 int RangeMeasPollSingleShot(); 01373 int RangeMeasPollContinuousMode(); 01374 int RangeMeasIntContinuousMode(void (*fptr)(void)); 01375 01376 01377 VL53L0X_DeviceInfo_t DeviceInfo; 01378 01379 /* IO Device */ 01380 DevI2C &dev_i2c; 01381 /* Digital out pin */ 01382 DigitalOut *gpio0; 01383 /* GPIO expander */ 01384 STMPE1600DigiOut *expgpio0; 01385 /* Measure detection IRQ */ 01386 InterruptIn *gpio1Int; 01387 /* Device data */ 01388 VL53L0X_Dev_t MyDevice; 01389 VL53L0X_DEV Device; 01390 }; 01391 01392 01393 #endif /* _VL53L0X_CLASS_H_ */ 01394 01395
Generated on Wed Jul 13 2022 06:23:43 by
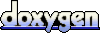