
with one warning !!
Fork of testing_rtc by
Embed:
(wiki syntax)
Show/hide line numbers
rtc_tst.cpp
00001 #include "rtc_tst.h" 00002 #include "string.h" 00003 00004 SPI spi(PTD6, PTD7, PTD5); // mosi, miso, sclk 00005 DigitalOut cs(D9); 00006 00007 00008 char* Getts() 00009 { 00010 00011 // Setup the spi for 8 bit data, high steady state clock, 00012 // second edge capture, with a 1MHz clock rate 00013 spi.format(8,3); 00014 spi.frequency(1000000); 00015 00016 cs = 0; 00017 spi.write(0x80); // set write register to seconds 00018 spi.write(0x00); // send value of zero 00019 00020 //cs=1; 00021 //cs=0; 00022 spi.write(0x81); 00023 spi.write(0x00); //write reg to minutes 00024 00025 //cs=1; 00026 //cs=0; 00027 00028 spi.write(0x82); 00029 spi.write(0x15); //write reg to hours (00-23) 00030 00031 spi.write(0x83); 00032 spi.write(0x07); //write reg to day (1-7) 00033 00034 spi.write(0x84); 00035 spi.write(0x20); //write reg to date(01-31) 00036 00037 spi.write(0x85); 00038 spi.write(0x12); //write reg to mnth(01-12) 00039 00040 spi.write(0x86); 00041 spi.write(0x12); //write reg to year(00-99) 00042 00043 cs=1; 00044 // Receive the contents of the seconds register 00045 00046 00047 00048 00049 cs=0; 00050 spi.write(0x00); // set read register to seconds 00051 int seconds = spi.write(0x00); // read the value by sending dummy byte 00052 00053 //cs=1; 00054 //cs=0; 00055 00056 spi.write(0x01); 00057 int minutes =spi.write(0x01); 00058 00059 //cs=1; 00060 //cs=0; 00061 00062 spi.write(0x02); 00063 int hours =spi.write(0x01); 00064 00065 spi.write(0x03); 00066 int day =spi.write(0x01); 00067 00068 spi.write(0x04); 00069 int date =spi.write(0x01); 00070 00071 spi.write(0x05); 00072 int month =spi.write(0x01); 00073 00074 spi.write(0x06); 00075 int year =spi.write(0x01); 00076 00077 //This cs=1 is to make the chipselect line high to "deselect" the slave in our case RTC 00078 cs = 1; 00079 00080 //This printf function is to check the timestamp function in the terminal output 00081 printf("timestamp as = %X : %X : %X : %X : %X : %X : %X\n\r", hours,minutes,seconds,day,date,month,year); 00082 00083 00084 printf("b4 returning tmpstmp is : %s\n",getname(year,month,date,day,hours,minutes,seconds)); 00085 return(getname(year,month,date,day,hours,minutes,seconds)); 00086 00087 00088 } 00089 00090 00091 00092 00093 00094 00095 char* getname(int year1,int month1,int date1,int day1,int hours1,int minutes1,int seconds1) 00096 { 00097 year1= hexint(year1); 00098 month1=hexint(month1); 00099 date1=hexint(date1); 00100 day1=hexint(day1); 00101 hours1=hexint(hours1); 00102 minutes1=hexint(minutes1); 00103 seconds1=hexint(seconds1); 00104 /*char y[3]=getstr(year); 00105 char m[3]=getstr(month); 00106 char dat[3]=getstr(date); 00107 char da[3]=getstr(day); 00108 char h[3]=getstr(hours); 00109 char mi[3]=getstr(minutes); 00110 char s[3]=getstr(seconds);*/ 00111 char time[15]; 00112 sprintf(time,"%d%d%d%d%d%d%d",year1,month1,date1,day1,hours1,minutes1,seconds1); 00113 return(time); 00114 00115 } 00116 00117 int hexint(int a) 00118 { 00119 a=(a/16)*10+(a%16); 00120 return a; 00121 }
Generated on Sun Jul 17 2022 05:07:27 by
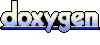